Solving Asynchronous Testing Problems in Java Frameworks
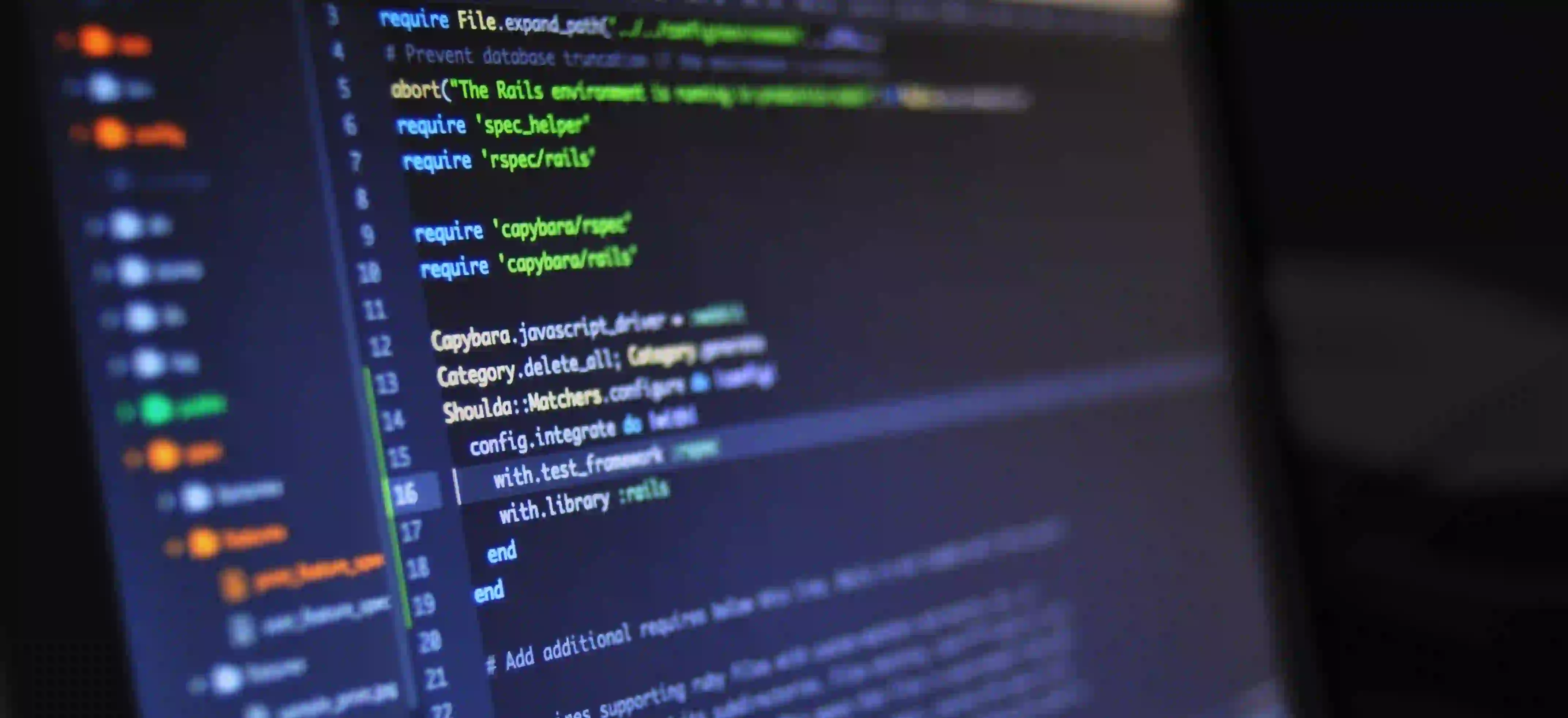
Solving Asynchronous Testing Problems in Java Frameworks
As more applications move towards asynchronous programming paradigms, particularly with the rise of microservices and reactive programming, the implications for testing these applications grow in complexity. Java, being a versatile and widely-used language, offers a number of frameworks that support asynchronous operations. In this blog post, we will explore asynchronous testing challenges in Java frameworks and provide solutions to address them effectively.
Understanding Asynchronous Programming
Before diving into testing strategies, it's crucial to understand what asynchronous programming entails. In simple terms, asynchronous programming allows multiple operations to be executed concurrently. This can improve the efficiency and responsiveness of applications, particularly those that perform I/O operations, such as fetching data from a remote server.
However, this concurrency introduces challenges in testing because the order of operations is not guaranteed, making it difficult to assert outcomes reliably.
Common Challenges in Asynchronous Testing
-
Race Conditions: When two or more threads access shared data simultaneously, inconsistency may arise. Detecting race conditions during testing can be tough since they may occur intermittently.
-
Non-Determinism: Due to the nature of asynchronous calls, tests may produce different outcomes on different runs, making it challenging to establish solid test cases.
-
Timeouts: Asynchronous operations can sometimes take longer than expected. Setting appropriate timeouts for tests can save headaches down the line.
-
Complexity of Assertions: Traditional assertions may not work properly when dealing with asynchronous operations. Special handling is required to ensure that values are checked appropriately.
Given these challenges, let's look at how to implement effective testing strategies in popular Java frameworks.
Testing Asynchronous Code with JUnit and CompletableFuture
JUnit is one of the most popular testing frameworks for Java, and it works quite well with asynchronous programming, especially when combined with the CompletableFuture
class.
Example with CompletableFuture
Let's consider a simple service that fetches user details from a remote API asynchronously.
import java.util.concurrent.CompletableFuture;
public class UserService {
public CompletableFuture<User> getUserAsync(String userId) {
return CompletableFuture.supplyAsync(() -> {
// Simulated delay to mimic network latency
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return new User(userId, "John Doe");
});
}
}
class User {
private String userId;
private String name;
public User(String userId, String name) {
this.userId = userId;
this.name = name;
}
public String getUserId() { return userId; }
public String getName() { return name; }
// hashCode, equals, other methods...
}
In the code above, getUserAsync
simulates an asynchronous call to fetch user details. The use of CompletableFuture
allows us to easily work with the result once it's ready.
Unit Testing the Asynchronous Code
To test this asynchronous method, we can use JUnit's assertTimeout
capability, allowing us to specify a maximum duration to complete the test.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
public class UserServiceTest {
private final UserService userService = new UserService();
@Test
void testGetUserAsync() {
CompletableFuture<User> userFuture = userService.getUserAsync("123");
User user = assertTimeoutPreemptively(java.time.Duration.ofSeconds(2), () -> {
return userFuture.join(); // block to get the result
});
assertNotNull(user);
assertEquals("123", user.getUserId());
assertEquals("John Doe", user.getName());
}
@Test
void testGetUserAsyncWithException() {
User user = userService.getUserAsync("invalidId").exceptionally(ex -> {
return new User("unknown", "Unknown User");
}).join();
assertNotNull(user);
assertEquals("unknown", user.getUserId());
assertEquals("Unknown User", user.getName());
}
}
Commentary on the Code
-
join()
: This method allows you to retrieve the result of theCompletableFuture
. Be cautious: if the computation threw an exception,join()
will rethrow it wrapped in aCompletionException
. -
assertTimeoutPreemptively
: This is used to ensure that our test does not hang indefinitely. If the asynchronous call takes longer than the specified duration, the test will fail. -
Handling Exceptions: The use of
exceptionally
helps manage any errors gracefully, providing a fallback user object in case of failure.
These patterns not only effectively manage asynchronous outcomes but also ensure our tests are robust and reliable.
Advanced Testing with Reactive Streams
In scenarios where your application leverages reactive programming, frameworks like Project Reactor or RxJava come into play, which creates streams of data that can be processed asynchronously.
Example With Project Reactor
Consider using the Mono
type in Project Reactor to deal with asynchronous data fetching:
import reactor.core.publisher.Mono;
public class ReactiveUserService {
public Mono<User> getUserReactive(String userId) {
return Mono.fromCallable(() -> {
Thread.sleep(1000); // Simulate delay
return new User(userId, "John Doe");
});
}
}
Testing Reactive Streams with StepVerifier
For testing purpose, StepVerifier
in Project Reactor allows you to assert expectations on the sequence of emitted items.
import org.junit.jupiter.api.Test;
import reactor.test.StepVerifier;
public class ReactiveUserServiceTest {
private final ReactiveUserService userService = new ReactiveUserService();
@Test
void testGetUserReactive() {
StepVerifier.create(userService.getUserReactive("123"))
.expectNextMatches(user -> {
assertEquals("123", user.getUserId());
assertEquals("John Doe", user.getName());
return true;
})
.expectComplete()
.verify();
}
}
Key Points in Reactive Testing
-
StepVerifier.create
: This initializes aStepVerifier
for the providedMono
orFlux
. It's an essential tool in verifying the reactive pipeline. -
expectNextMatches
: Using this method allows you to check against the emitted object while also executing additional assertions.
By allowing for a clear testing strategy in a reactive setting, we can ensure our asynchronous operations behave as expected.
Closing Remarks
Asynchronous programming in Java offers numerous benefits, especially in enhancing application performance. However, it presents unique challenges when it comes to testing these asynchronous behaviors. Utilizing modern Java frameworks like JUnit and Spring’s Project Reactor, you can structure your tests to handle asynchronous calls effectively.
For additional insights about tackling asynchronous testing issues, consider reviewing the existing article titled Mastering Cypress: Tackling Asynchronous Testing Issues. It contains valuable strategies which, though focused on JavaScript frameworks, can inspire your approach in the Java ecosystem.
By implementing the strategies discussed above, you're well on your way to mastering asynchronous testing in Java, enabling you to deliver efficient and robust applications confidently.