Java Strategies for Handling Asynchronous Testing in Cypress
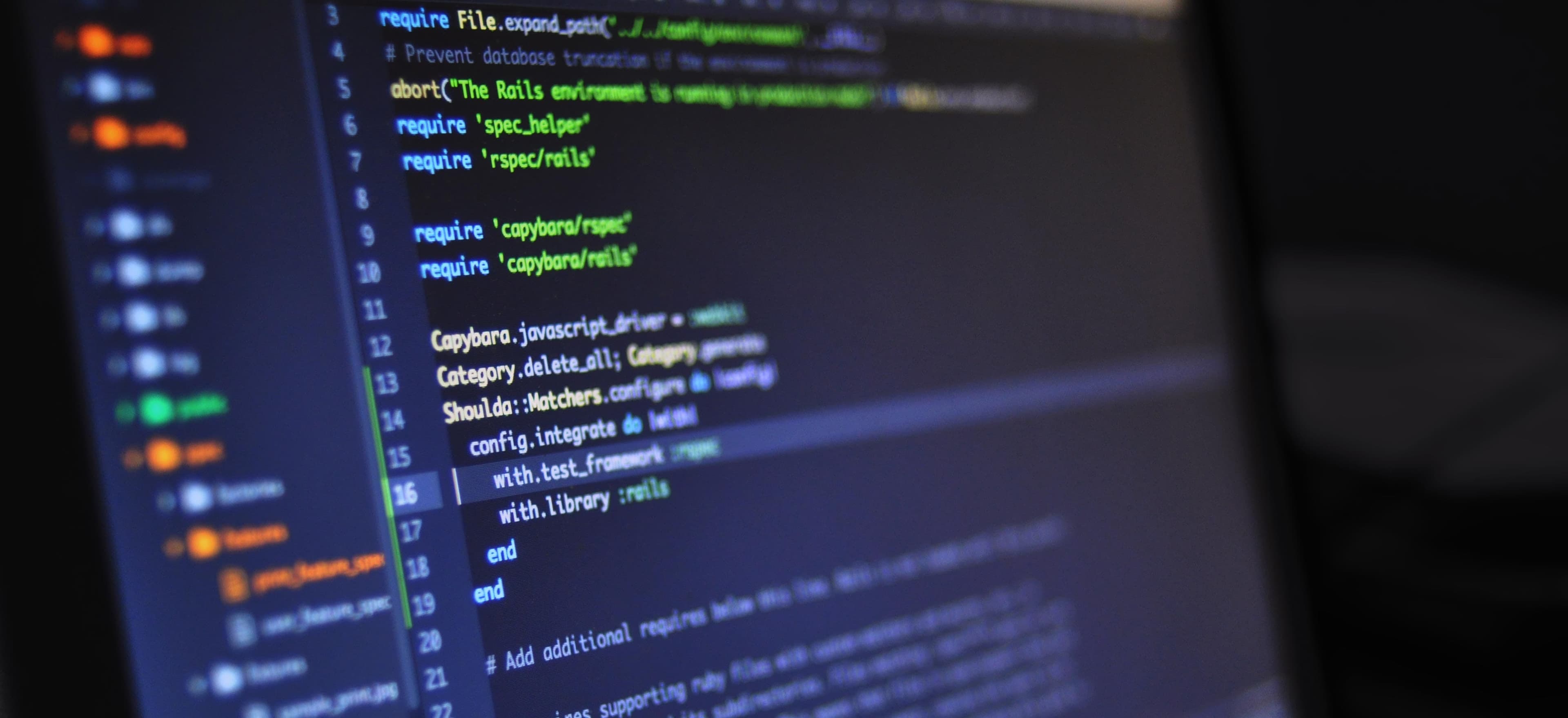
- Published on
Java Strategies for Handling Asynchronous Testing in Cypress
When it comes to testing web applications, ensuring the reliability of asynchronous operations can be particularly challenging. This is where tools like Cypress shine. Known for its ability to handle complex front-end testing, Cypress focuses on real-time testing, making it a favorite among developers. While most resources focus on JavaScript-based testing strategies, integrating Java into your workflow can further streamline your testing strategy, especially in large applications where UI tests are crucial.
In this blog post, we will explore various Java strategies to enhance asynchronous testing in Cypress. We will also refer to an insightful resource titled "Mastering Cypress: Tackling Asynchronous Testing Issues" available at tech-snags.com/articles/mastering-cypress-asynchronous-testing for a deeper understanding of some asynchronous challenges in testing.
Understanding Asynchronous Testing
Asynchronous testing deals with actions that are performed independently of the main program flow. In web applications, this is so common that failing to test these scenarios can lead to major issues in production. Typically, AJAX requests, API calls, and dynamic DOM updates are areas where asynchronicity manifests.
Characteristics of Asynchronous Operations
- Non-blocking: They allow other operations to continue while waiting for the response.
- Timing-Dependent: The timing of the operations can be unpredictable.
- State Management: They frequently update application state, which can introduce complexity in tests.
Technology stacks like Java and JavaScript differ significantly in how they handle these operations. Java developers leveraging Cypress need to keep in mind how to orchestrate Java's synchronous nature with Cypress's asynchronous behavior.
Leveraging Java for Cypress Testing
In a typical scenario, you might have a Java backend with a Cypress frontend for testing JavaScript-heavy applications. Here's how you can utilize Java to handle asynchronous testing effectively:
1. Employ Behavioral Driven Development (BDD)
Implementing BDD can bridge the gap between your Java backend and Cypress tests. By leveraging frameworks like Cucumber-Gherkin in Java, you can define test scenarios in a human-readable format. This approach provides clarity on test cases, including those that involve asynchronous behavior.
Here's an example of how you can write a BDD scenario:
Feature: User Login
Scenario: Successfully logging in
Given the user navigates to the login page
When the user enters valid credentials
Then the user should be redirected to the dashboard
Using Cypress, you can then write test implementations that correspond to these scenarios:
Given('the user navigates to the login page', () => {
cy.visit('/login');
});
When('the user enters valid credentials', () => {
cy.get('input[name=username]').type('testuser');
cy.get('input[name=password]').type('password123');
cy.get('button[type=submit]').click();
});
Then('the user should be redirected to the dashboard', () => {
cy.url().should('include', '/dashboard');
});
2. Use Mocking and Stubbing
Mocking API responses in your Cypress tests can dramatically improve the reliability of tests. With tools like Nock for Node.js, you can simulate the Java backend, allowing you to control the asynchronous flow without relying on a live server.
it('should display user info after fetching from API', () => {
cy.intercept('GET', '/api/user', { fixture: 'user.json' }).as('getUser');
cy.visit('/profile');
cy.wait('@getUser');
cy.get('.user-name').should('contain', 'John Doe');
});
Using Cypress's built-in interception capabilities allows you to control the testing environment more firmly. The API calls can be mocked effectively, ensuring that tests remain fast and reliable.
3. Implement Timeouts Wisely
Cypress provides global timeouts for waiting on asynchronous commands. Sometimes, however, adjusting these timeouts can yield significant improvements in test stability. By default, the timeout is set to 4 seconds, but that can be changed depending on your application’s specs.
// Custom command with extended timeout
Cypress.Commands.add('waitForResponse', (timeout = 10000) => {
return cy.wait('@someRequestAlias', { timeout });
});
// Example of use
cy.intercept('GET', '/api/data').as('getData');
cy.visit('/some-page');
cy.waitForResponse(); // Waits up to 10 seconds
This is crucial when testing applications that may take longer to respond due to heavy load or real-time updates. Providing a flexible waiting strategy will reduce test flakiness.
4. Assert on AJAX Responses
Using the natural waiting capabilities of Cypress simplifies many tasks. The framework can automatically wait for elements to appear, which is fundamental when dealing with asynchronous operations.
Here's an illustrative example:
it('displays loading spinner until data arrives', () => {
cy.intercept('GET', '/api/items', { delay: 500 }).as('getItems');
cy.visit('/items');
cy.get('.loading').should('be.visible'); // Check if loading spinner shows
cy.wait('@getItems');
cy.get('.loading').should('not.exist'); // Check if it disappears
});
Assertions on loading states can clarify test intent and enhance user experience analysis, emphasizing the responsiveness of your application.
Best Practices for Java-Cypress Integration
- Keep Tests Isolated: Each test should be independent of others to simplify debugging and enhance reliability.
- Retain Clarity in Tests: Use descriptive names for tests and maintain a clean structure. This will aid both new developers and those revisiting old tests.
- Maintain a Feedback Loop: Building a continuous integration (CI) pipeline to execute your Cypress tests on every code change ensures that you catch issues early.
To Wrap Things Up
Navigating the complexities of asynchronous testing can be a daunting task, especially when integrating Java-based applications with JavaScript testing tools like Cypress. However, with a solid understanding of best practices, such as using BDD, leveraging mocking strategies, implementing suitable timeouts, and asserting effectively on AJAX responses, you can greatly enhance your testing workflow.
For a more in-depth look into tackling asynchronous testing issues using Cypress, consider exploring "Mastering Cypress: Tackling Asynchronous Testing Issues" at tech-snags.com/articles/mastering-cypress-asynchronous-testing. This article lays a foundation for better handle on asynchronous challenges, allowing you to form a comprehensive testing strategy.
Utilizing these Java strategies alongside Cypress can lead your team to achieve more effective, reliable, and scalable testing practices, ultimately enhancing your software development lifecycle. Happy testing!
Checkout our other articles