Java in Web Development: Choosing the Right Path
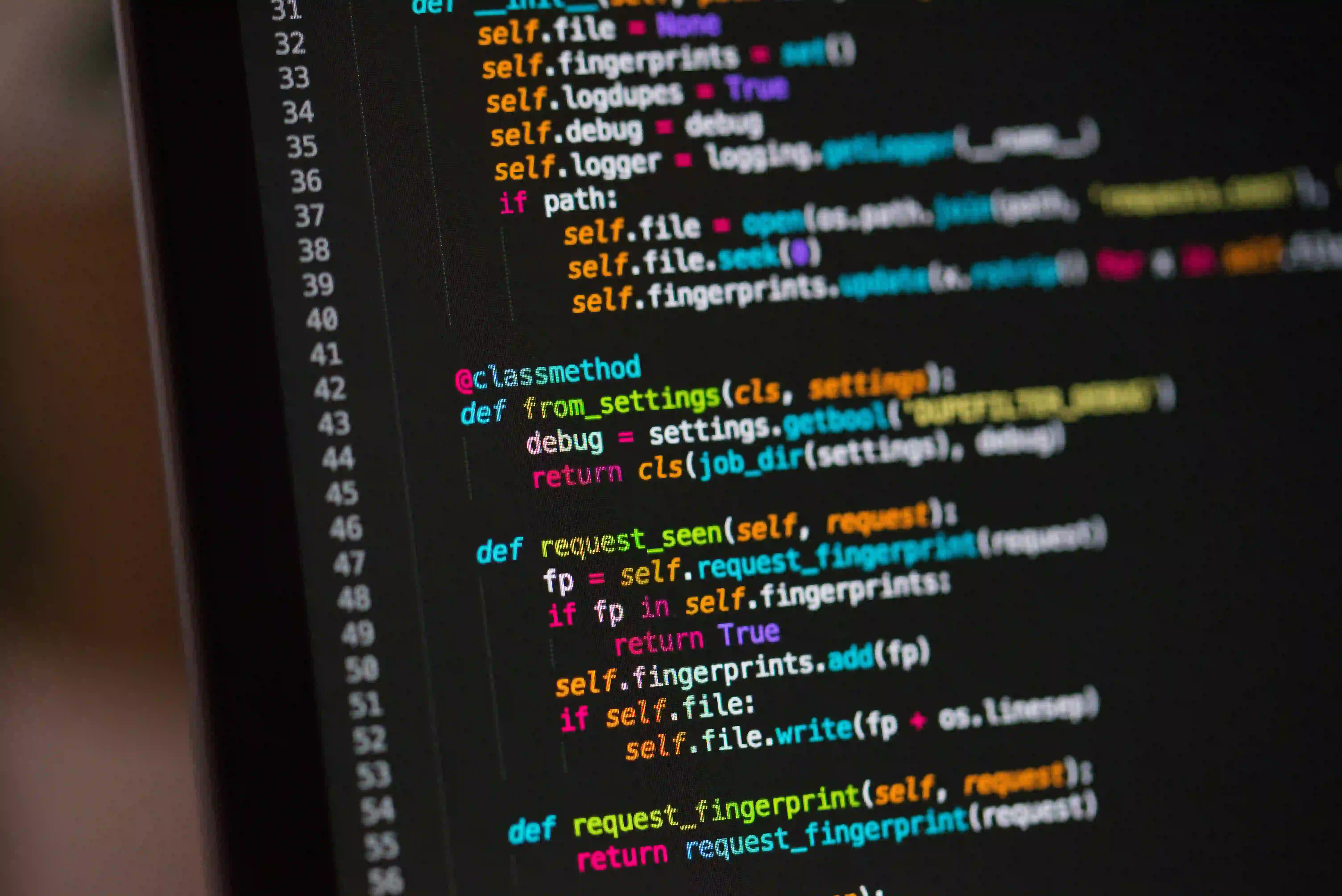
Java in Web Development: Choosing the Right Path
Java has been a prominent player in the realm of web development for decades. With its "Write Once, Run Anywhere" mantra, the language has made a name for itself due to its portability, scalability, and robust community support. If you're considering a career in web development, you might be wondering where Java fits compared to other programming languages. This article will guide you through the essential aspects of using Java in web development, helping you make an informed decision about your career path.
1. Why Java?
Java is not merely a programming language; it is a powerful ecosystem. It offers a diverse set of frameworks, tools, and libraries that can fulfill various development needs. Here are some compelling reasons to consider Java for web development:
a. Platform Independence
Java applications can run on any device that has a Java Virtual Machine (JVM) installed. This feature makes it excellent for building cross-platform web applications.
b. Scalability
Java handles high loads effortlessly. If you expect your web application to grow in terms of users and data, Java is a reliable choice.
c. Security Features
Security is paramount in web applications. Java offers built-in security features, making it easy to build secure applications.
d. Strong Community Support
Java has been around since 1995. There is an immense community of developers ready to assist you, along with extensive documentation available.
e. Rich Development Tools
Java offers a variety of Integrated Development Environments (IDEs), such as IntelliJ IDEA and Eclipse, which make coding effective and less error-prone.
2. Java Frameworks for Web Development
Java boasts a variety of frameworks that streamline web application development. Below are some of the most popular:
a. Spring Framework
Spring is a powerful framework that provides comprehensive infrastructure support for developing Java applications. One of its key features is its inversion of control (IoC) container, which helps manage object creation and dependency injection.
Here’s an example of how to define a simple Spring REST controller:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
Why use Spring?
Spring enables reduced boilerplate code and promotes a clean architectural approach through its dependency injection system.
b. JavaServer Faces (JSF)
JSF is a component-based framework that simplifies the development of user interfaces for Java web applications. It allows developers to create reusable UI components.
This example shows how to create a simple JSF page:
<h:form>
<h:inputText value="#{bean.name}" />
<h:commandButton value="Submit" action="#{bean.submit}" />
</h:form>
Why use JSF?
JSF is ideal for complex UIs because it allows for greater reusability of components, making your code cleaner and easier to manage.
c. Play Framework
The Play Framework enables asynchronous web applications in Java with a focus on scalability. It simplifies the development of modern web applications using a reactive model.
A simple Play Controller may look like this:
import play.mvc.*;
public class HomeController extends Controller {
public Result index() {
return ok("Hello from Play Framework!");
}
}
Why use Play?
If you prefer building responsive applications with minimal configuration, the Play Framework is designed for modern web development practices.
3. Best Practices for Java Web Development
If you decide to delve into Java web development, adhering to best practices is vital. Here are some guidelines you should consider:
a. Understand MVC Architecture
The Model-View-Controller (MVC) design pattern is a powerful architectural pattern commonly used in Java web applications. It separates the application into three interconnected components, allowing you to manage complexity effectively.
b. Use Build Tools
Make use of build automation tools like Maven or Gradle. These tools help manage project dependencies and streamline the build process.
c. Embrace Testing
Automated testing is crucial for maintaining code quality. Use JUnit or TestNG for unit testing your Java applications to ensure your code behaves as expected.
d. Write Clean Code
Readability matters. Use descriptive variable names, maintain proper indentation, and comment complex sections to make your code understandable.
4. Comparison with Other Languages
While Java excels in many areas, it is also essential to consider alternative languages and frameworks in web development. Languages like JavaScript, Python, and Ruby have gained popularity due to their ease of use and speed of development.
For instance, JavaScript is ubiquitous in web development primarily for client-side scripting, while Python with Django is favored for rapid application development.
Want to learn more about the differences in web-development career options? Check out this article: Web Dev or Web Design? Unraveling the Career Dilemma.
5. Conclusion
Java remains a strong contender in the landscape of web development. Whether it's the enterprise-level applications or flexible frameworks like Spring and JSF, Java provides a robust toolkit for ambitious developers. Use the resources available, keep fostering your skills, and engage with the community to stay updated.
If you choose the Java path, you'll not only acquire a valuable skill set but also join a global community of developers who are continually pushing the boundaries of what's possible.
So, is Java right for your web development career? The answer lies in your specific goals, interests, and the type of applications you want to build. Take the plunge into the Java ecosystem and discover what it offers!
This blog post is designed to be SEO-optimized while providing an engaging and informative view on Java in web development. Whether you are a beginner or looking to specialize further, the insights offered here can guide you effectively.