Balancing Mineral Content in Java-Based Water Purification Systems
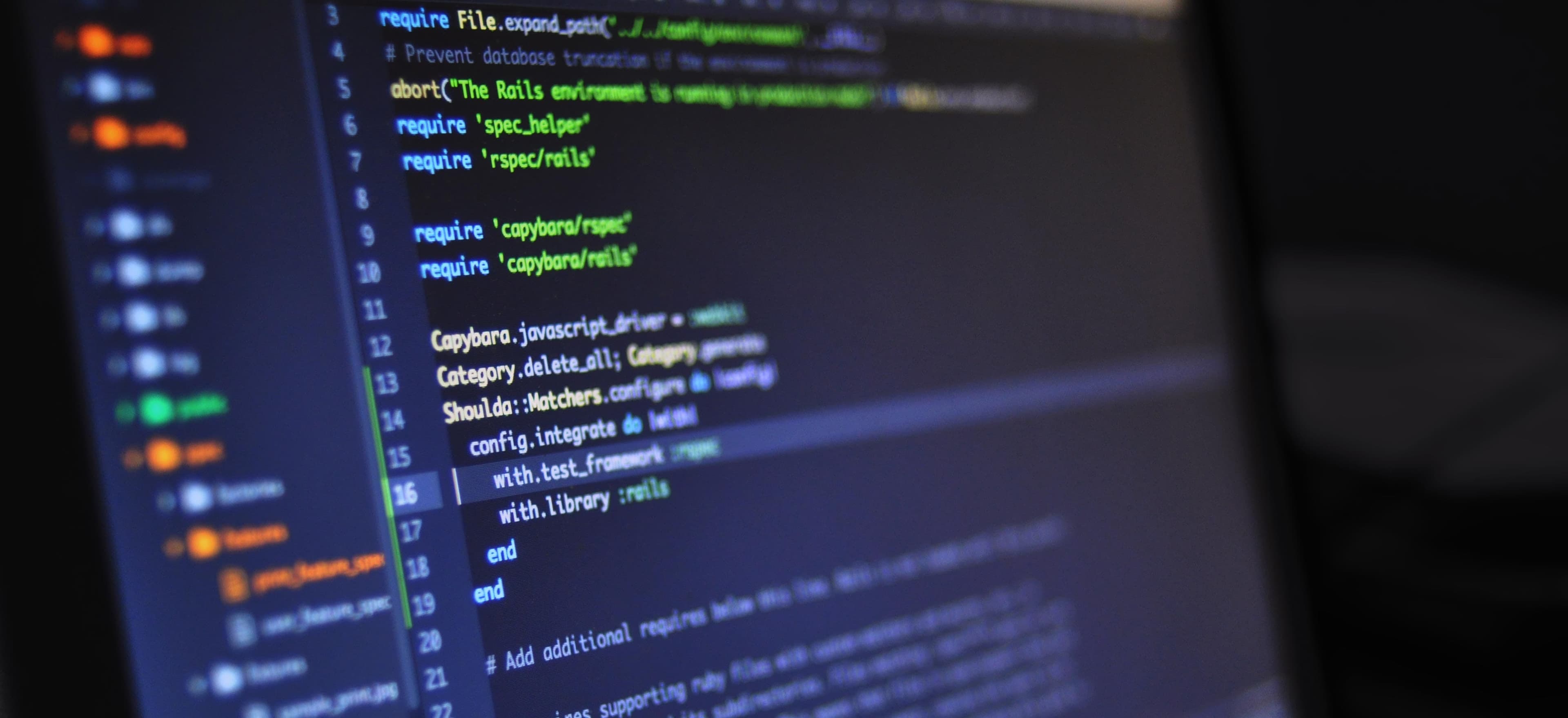
- Published on
Balancing Mineral Content in Java-Based Water Purification Systems
Water purification is crucial for ensuring the health of consumers. A primary concern among consumers is how water filters, particularly those like the Berkey filter, affect the mineral content of water. If you want to delve deeper, you can check out the article titled Does Your Berkey Filter Strip Water of Essential Minerals?. In this blog post, we'll explore how to design a Java-based application for managing and balancing mineral content in water purification systems.
Understanding the Importance of Mineral Content
Water isn't just H2O; it contains vital minerals such as calcium, magnesium, and potassium that are essential for health. Many home water purification devices remove these beneficial minerals, which raises concerns among consumers. Thus, striking a balance between clean water and mineral retention is essential.
Why Build a Java Application for Mineral Management?
- Customization: A Java application allows for creating personalized settings based on local water quality and consumer preferences.
- Data Analysis: You can analyze the mineral content dynamically, offering consumers insights into how their water quality changes.
- User-Friendly Interface: With Java's powerful graphical libraries, you can design a user-friendly interface for monitoring mineral levels.
Setting Up Your Development Environment
Before diving into code, ensure you have set up your Java development environment. You'll need:
- Java Development Kit (JDK): Make sure you download the latest version from the Oracle website.
- Integrated Development Environment (IDE): While any text editor can be used, IDEs like IntelliJ IDEA or Eclipse offer great features for Java development.
Building the Application
Step 1: Project Structure
A good project structure can enhance code maintainability. Here is a simple directory structure:
WaterPurificationSystem/
├── src/
│ ├── Main.java
│ └── MineralManager.java
├── resources/
│ └── waterData.json
└── README.md
Step 2: Creating the MineralManager Class
The MineralManager
class will handle all mineral-related operations. This class will read data, calculate mineral content, and suggest improvements.
// MineralManager.java
import org.json.JSONObject;
import java.nio.file.Files;
import java.nio.file.Paths;
public class MineralManager {
private double calcium; // mg/L
private double magnesium; // mg/L
private double potassium; // mg/L
// Constructor
public MineralManager(String jsonData) {
parseJsonData(jsonData);
}
// Method to parse JSON data
private void parseJsonData(String jsonData) {
JSONObject jsonObject = new JSONObject(jsonData);
this.calcium = jsonObject.getDouble("calcium");
this.magnesium = jsonObject.getDouble("magnesium");
this.potassium = jsonObject.getDouble("potassium");
}
// Method to calculate total mineral content
public double calculateTotalMinerals() {
return calcium + magnesium + potassium;
}
// Display mineral content
public void displayMineralContent() {
System.out.println("Calcium: " + calcium + " mg/L");
System.out.println("Magnesium: " + magnesium + " mg/L");
System.out.println("Potassium: " + potassium + " mg/L");
}
}
Commentary on the Code
-
JSON Parsing: The
parseJsonData
method efficiently extracts mineral data from a JSON object. The actual data can be stored in a file namedwaterData.json
in the resources folder. This makes it easy to update data without altering the code. -
Modular Design: This design allows for easy expansion. If you decide to include more minerals, simply modify the
MineralManager
class without affecting other components.
Step 3: Creating the Main Application Class
The Main
class will serve as the entry point for our application.
// Main.java
import java.nio.file.Files;
import java.nio.file.Paths;
public class Main {
public static void main(String[] args) {
try {
// Read data from JSON file
String jsonData = new String(Files.readAllBytes(Paths.get("resources/waterData.json")));
MineralManager mineralManager = new MineralManager(jsonData);
// Display mineral content
mineralManager.displayMineralContent();
double totalMinerals = mineralManager.calculateTotalMinerals();
System.out.println("Total Mineral Content: " + totalMinerals + " mg/L");
} catch (Exception e) {
System.err.println("Error reading file: " + e.getMessage());
}
}
}
Commentary on the Code
-
File Handling: The application reads data from a file, promoting separation of concerns. It has better maintainability, making it easier to update mineral levels.
-
Error Handling: The
try-catch
block ensures that any IO exceptions are handled gracefully, which is critical when working with file systems.
Step 4: Data File Example
You can create a JSON file named waterData.json
in the resources
folder. This file might look like this:
{
"calcium": 50.0,
"magnesium": 30.0,
"potassium": 2.5
}
Step 5: Testing the Application
Run the application from your IDE or command line. It should display the mineral content based on the data provided in the JSON file. Modify the values in the waterData.json
file to test how the application reacts to different mineral levels.
Recommendations for Further Improvements
While this basic application provides a baseline for managing mineral content, there are several enhancements that can be implemented:
-
Graphical User Interface (GUI): Consider using JavaFX or Swing to create a user-friendly interface for users.
-
Database Integration: For a more robust solution, integrate a database to store historical mineral data for analysis.
-
Alerts and Notifications: Enable alerts if the mineral levels fall below or exceed recommended guidelines.
-
Web API: Create a RESTful API to allow other applications to interface with your water purification system.
Wrapping Up
By leveraging Java to build a water purification management system, we can help consumers make informed decisions about their water quality and mineral content. Understanding how systems like the Berkey filter affect mineral levels is pivotal. For further insights on this topic, consider reading Does Your Berkey Filter Strip Water of Essential Minerals?.
This Java application can serve as a foundational step in developing more sophisticated solutions that prioritize both the cleanliness of drinking water and the retention of essential minerals. Happy coding!
Checkout our other articles