Java Strategies for Building Resilient Promo Code Engines
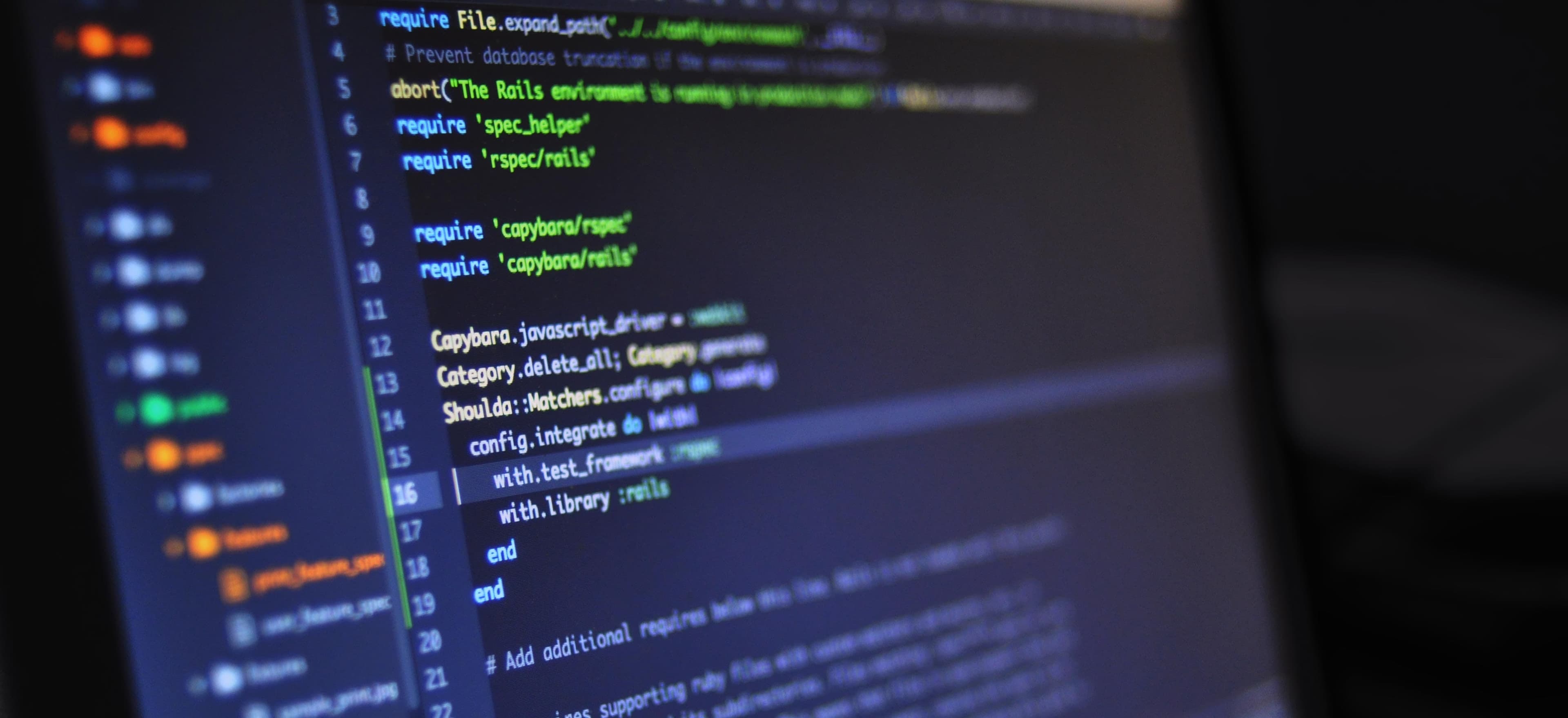
- Published on
Java Strategies for Building Resilient Promo Code Engines
Building a promo code engine poses unique challenges, particularly in ensuring reliability and scalability. In this blog post, we'll delve into several Java strategies for designing a resilient promo code engine, ideal for eCommerce platforms and businesses. From handling varying code structures to giving insights into user behavior, we’ll cover everything you need to know to create a robust system.
Understanding Promo Code Engines
A promo code engine is designed to validate coupon codes, apply discounts, and manage the overall discount strategy for a business. It often needs to support multiple conditions, including expiration dates, usage limits, and varying discount structures.
Key Components of a Promo Code Engine
- Validation: Check if the provided code is valid.
- Rules Engine: Apply the discount rules associated with the promo code.
- Logging & Monitoring: Track usage and performance.
- Integration: Interact seamlessly with the eCommerce platform.
In the following sections, we will layer more strategies and insights on each of these components using Java.
Validation Strategies
To start, let’s address the importance of a robust validation mechanism. A promo code may need to be validated against various criteria:
- It must be active and not expired.
- It should have available usage limits.
- The associated terms should match the user’s cart.
Here's a sample Java method for validating a promo code:
public boolean isPromoCodeValid(PromoCode promoCode, Cart cart) {
// Check if the promo code is active
if (!promoCode.isActive()) {
return false;
}
// Check if it has expired
if (promoCode.getExpirationDate().isBefore(LocalDate.now())) {
return false;
}
// Check usage limits
if (promoCode.getUsageCount() >= promoCode.getMaxUsage()) {
return false;
}
// Additional checks can be added here
return true;
}
Explanation of the Code
- Active Check: We ensure that the promo code is active by calling
promoCode.isActive()
. - Expiration Check: The expiration date is compared against the current date.
- Usage Limits: We compare how many times the promo code has been used against the maximum allowed usage.
By incorporating comprehensive checks, we ensure that the correct information reaches the users.
Rules Engine Configuration
Next, let's dive into implementing a rules engine that applies the discounts based on the promo code characteristics. Depending on your needs, you might want to implement different discount strategies, such as percentage off, fixed amount off, or even buy-one-get-one-free (BOGO).
public double applyDiscount(PromoCode promoCode, Cart cart) {
double discount = 0;
switch (promoCode.getDiscountType()) {
case PERCENTAGE:
discount = cart.getTotalAmount() * (promoCode.getDiscountValue() / 100);
break;
case FIXED_AMOUNT:
discount = promoCode.getDiscountValue();
break;
case BOGO:
// BOGO logic here
discount = applyBogoDiscount(cart, promoCode);
break;
default:
throw new IllegalArgumentException("Invalid discount type");
}
return discount > cart.getTotalAmount() ? cart.getTotalAmount() : discount;
}
Why Use a Rules Engine?
- Flexibility: Supporting multiple discount strategies helps meet diverse marketing needs.
- User Experience: A seamless implementation contributes to an improved shopping experience.
Adding more discount types in the future will be straightforward. Just extend the switch statement.
Logging and Monitoring
To create a resilient promo code engine, logging is crucial. Keeping track of how promo codes are applied helps you understand user behavior. Here is how you might implement basic logging:
public void logPromoCodeUsage(PromoCode promoCode, User user) {
// Sample logging implementation, ideally should go to a logging framework
System.out.println("User " + user.getId() + " applied promo code " + promoCode.getCode() + " on " + LocalDateTime.now());
// Here you might integrate with a real logging library, for instance, Log4j or SLF4J.
}
The Importance of Monitoring
- Performance Metrics: Understanding which promo codes perform well helps in future promotions.
- User Behavior: Insights can help tailor future marketing strategies and identify user preferences.
For further exploration on promotional strategies, check the article on Survive the Savings: Promo Codes for Doomsday Preppers. Although it addresses doomsday prepper needs, the strategies for promotional codes can be universally applied.
Integration with eCommerce Systems
Integration with existing eCommerce systems is vital. Your promo code engine must interact effectively with your product catalog, user database, and checkout process.
Here’s a simple example of how you might handle promo code validation during checkout:
public double checkout(Cart cart, String promoCodeInput) {
double discount = 0;
PromoCode promoCode = promoCodeRepository.findByCode(promoCodeInput);
if (promoCode != null && isPromoCodeValid(promoCode, cart)) {
discount = applyDiscount(promoCode, cart);
logPromoCodeUsage(promoCode, cart.getUser());
}
return cart.getTotalAmount() - discount;
}
Explanation of Checkout Process
- Promo Code Retrieval: You retrieve the promo code from the repository with
findByCode(promoCodeInput)
. - Validation and Discount Application: We validate and apply the discount if valid.
- Logging: The usage is logged post-application for further analytics.
Integrating your promo code engine with your checkout process ensures fluent and effective discount application.
Best Practices for Resilient Promo Code Engines
- Modular Design: Utilize a clean architecture to separate concerns—validation, application, logging.
- Unit Testing: Validate each component's functionality independently.
- Scalable Database: Ensure that your database can handle high loads during sales traffic.
- User Feedback Loop: Actively seek user feedback on your promo code functionality for continuous improvement.
Lessons Learned
Building a resilient promo code engine in Java involves meticulous attention to validation, rule application, logging, and integration practices. The strategies outlined in this guide are foundational to creating an effective and reliable system.
By implementing a focused approach, your promo codes won't just be mere marketing tools; they'll become powerful assets that enhance user experience and drive conversions. As you explore these strategies, remember that adaptability and continuous learning are vital components of your development journey.
Utilize these principles to cultivate an engine that can withstand the tides of market demands and user expectations, ensuring robust functionality today and tomorrow.
Checkout our other articles