Optimizing Resource Management with Java: A Prepper's Guide
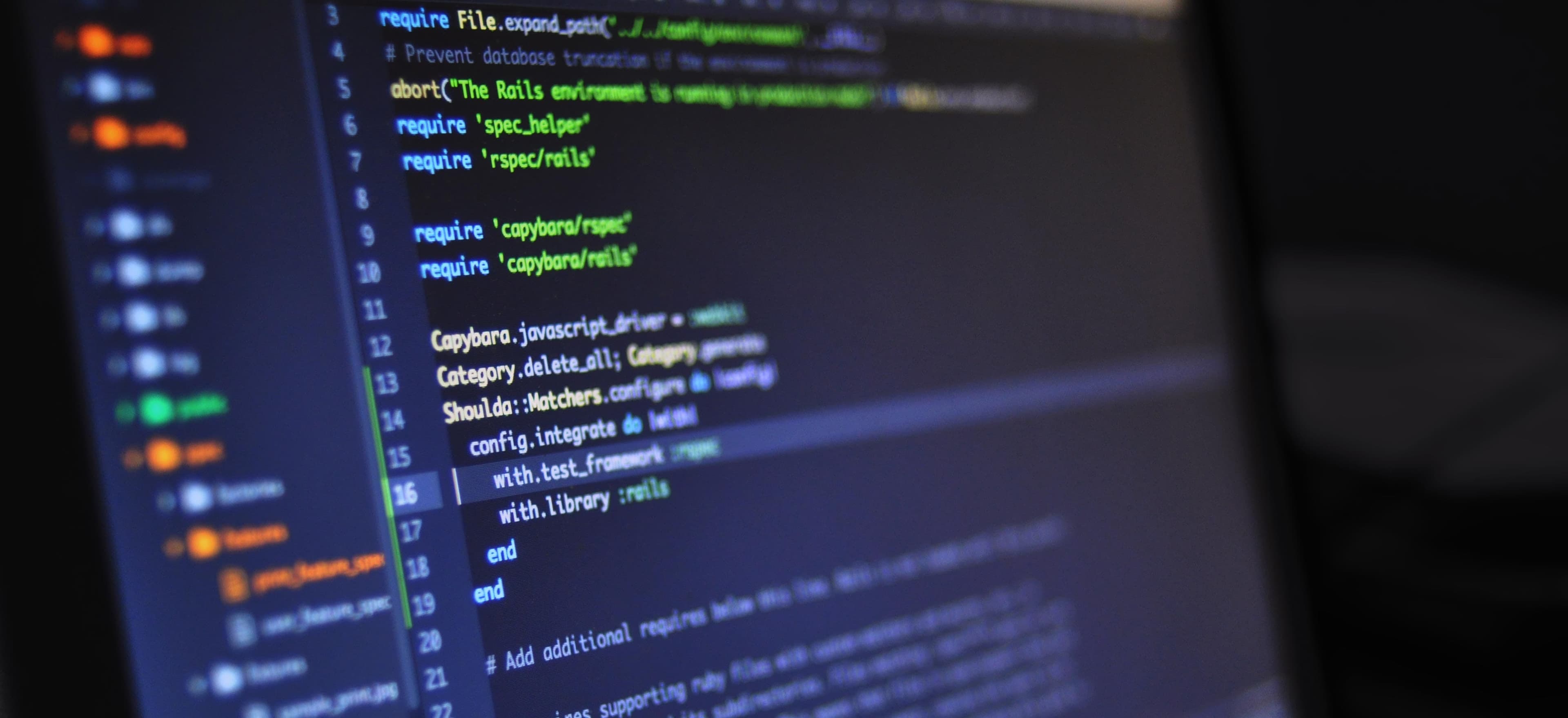
- Published on
Optimizing Resource Management with Java: A Prepper's Guide
In an unpredictable world, efficient resource management becomes paramount. Whether you are a doomsday prepper or simply someone looking to manage resources effectively, mastering Java can significantly enhance your toolkit. In this guide, we will explore how to use Java to optimize resource management, draw parallels to prepping strategies, and discuss coding best practices that ensure your applications run smoothly.
Understanding Resource Management
Before diving into Java specifics, let's clarify what resource management entails. Resource management involves the strategic deployment of resources—be it time, money, materials, or human capital—to achieve specific objectives. For instance, as highlighted in the article Survive the Savings: Promo Codes for Doomsday Preppers, preparing for emergencies can benefit from smart spending and resource optimization.
Why Use Java for Resource Management?
Java is a versatile, high-level programming language known for its portability, scalability, and strong community support. These attributes make Java an excellent choice for developing resource management systems. Here are some reasons why Java is particularly suited for this task:
- Platform Independence: Java applications can run on any device with a Java Virtual Machine (JVM). This is crucial for accessing resources in diverse environments.
- Robust Libraries: Java offers numerous libraries that aid in data manipulation, making it easier to track and manage resources.
- Concurrency Support: Java's built-in support for multithreading enhances performance, especially in applications that handle multiple operations simultaneously.
Key Concepts in Resource Management with Java
To effectively leverage Java for resource management, we should focus on several key concepts:
- Data Structures
- File I/O
- Concurrency
- Exception Handling
Let's explore each of these concepts in detail, using code snippets to illustrate their application.
1. Data Structures
Using the correct data structures is vital for optimizing resource management. For instance, if you're developing a resource tracker for prepper supplies, a HashMap can be useful to quickly access data.
import java.util.HashMap;
public class ResourceTracker {
private HashMap<String, Integer> resources;
public ResourceTracker() {
resources = new HashMap<>();
}
public void addResource(String resource, int quantity) {
resources.put(resource, resources.getOrDefault(resource, 0) + quantity);
}
public int getResourceQuantity(String resource) {
return resources.getOrDefault(resource, 0);
}
public void displayResources() {
for (String resource : resources.keySet()) {
System.out.println(resource + ": " + resources.get(resource));
}
}
}
Why Use a HashMap?
A HashMap is ideal here as it allows for constant-time complexity O(1) for the average case when accessing resources. In a situation requiring quick inspections of stock levels—important for preppers—it greatly enhances performance.
2. File I/O
Resource management often includes reading from and writing to files, whether it's logging resource usage or updating inventories. Java’s java.nio.file
package simplifies this process.
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.List;
public class ResourceFileManager {
private final String filePath;
public ResourceFileManager(String filePath) {
this.filePath = filePath;
}
public void saveResources(List<String> resourceData) {
try {
Files.write(Paths.get(filePath), resourceData);
} catch (IOException e) {
System.out.println("Error writing to file: " + e.getMessage());
}
}
public List<String> loadResources() {
try {
return Files.readAllLines(Paths.get(filePath));
} catch (IOException e) {
System.out.println("Error reading from file: " + e.getMessage());
return null;
}
}
}
Why Use java.nio.file
?
This package offers a more modern way to handle file I/O compared to older methods. It enhances performance and readability, making it suitable for high-volume resource management applications.
3. Concurrency
In resource management systems, especially those that deal with real-time updates, handling multiple threads is crucial. Java’s ExecutorService
allows you to manage concurrent tasks efficiently.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
public class ResourceUpdater {
private final ExecutorService executor;
public ResourceUpdater(int threads) {
executor = Executors.newFixedThreadPool(threads);
}
public void updateResource(String resource) {
executor.submit(() -> {
// Simulate updating resource logic
System.out.println("Updating: " + resource);
});
}
public void shutdown() {
executor.shutdown();
try {
executor.awaitTermination(10, TimeUnit.SECONDS);
} catch (InterruptedException e) {
System.out.println("Executor service interrupted.");
}
}
}
Why Use ExecutorService?
Managing threads manually can lead to complex code and potential performance issues. ExecutorService
abstracts this complexity, allowing developers to focus on logic without worrying about thread management.
4. Exception Handling
Robust resource management requires anticipating potential failures. Proper exception handling ensures your application remains reliable.
public class ResourceManager {
public void manageResource(String resource, int quantity) {
try {
if (quantity < 0) {
throw new IllegalArgumentException("Quantity cannot be negative.");
}
// Logic for managing resources
System.out.println("Managing resource: " + resource + ", quantity: " + quantity);
} catch (IllegalArgumentException e) {
System.out.println("Error managing resource: " + e.getMessage());
}
}
}
Why Handle Exceptions?
Exception handling not only prevents crashes but also enhances user experience. By providing clear error messages, users can troubleshoot issues without technical know-how.
Best Practices for Resource Management in Java
To ensure your resource management system is efficient, consider the following best practices:
- Modular Code: Break your application into smaller modules for better readability and maintainability.
- Optimize for Performance: Choose appropriate data structures and algorithms based on your use case.
- Regular Testing: Conduct unit and integration tests to catch bugs early.
- Documentation: Provide clear documentation to guide future users or developers.
Final Thoughts
Resource management is critical for anyone looking to maintain a sense of control, particularly in uncertain times. Java provides the tools and capabilities needed to build effective resource management systems. By leveraging data structures, file I/O, concurrency, and exception handling, you can create robust applications that optimize resource allocation.
Whether you're a doomsday prepper or just someone looking to manage resources more effectively, mastering Java can empower you. For more information on how to save during your prepping journey, check out the article Survive the Savings: Promo Codes for Doomsday Preppers.
Ready to start building your resource management application? Dive into Java, and remember—preparation is the key to survival. Happy coding!
Checkout our other articles