Mastering Java for Cost-Efficient App Development
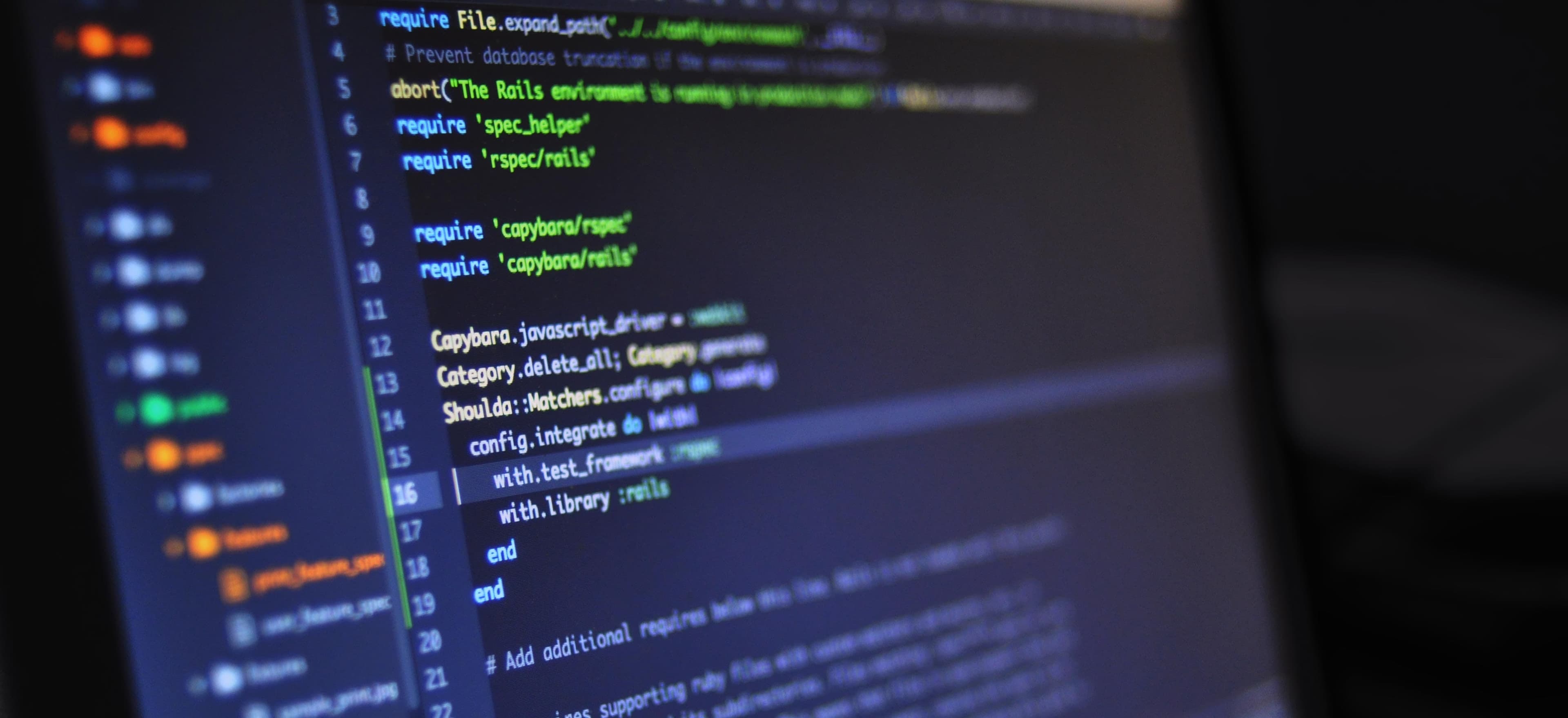
- Published on
Mastering Java for Cost-Efficient App Development
Java has consistently been one of the most popular programming languages, beloved by developers for its versatility and robustness. If you're aiming to develop applications in a cost-efficient manner, you’re in the right place. In this blog post, we’re going to dive into key concepts of Java development while keeping your budget in mind. Whether you are a budding developer or a seasoned professional, understanding Java’s ecosystem can lead to smarter, more economical decisions.
Why Choose Java for App Development?
Java is a powerful, platform-independent language that offers several advantages:
-
Cross-Platform Compatibility: Thanks to the Java Virtual Machine (JVM), Java applications can run on any device that supports the JVM. This characteristic minimizes the need for multiple versions of the same application for different platforms, ultimately saving time and cost.
-
Rich Ecosystem: Java's extensive libraries and frameworks facilitate quicker development. Libraries like Spring and Hibernate can accelerate the building process, often saving you countless hours and resources.
-
Strong Community Support: With a large and active community, finding help, resources, and libraries is easy. It’s also worth mentioning that a plethora of tutorials and forums are available, making it easier to overcome hurdles without incurring additional costs.
-
Robust Security Features: Java is designed with security in mind, featuring strong memory management and built-in antivirus capabilities, reducing potential vulnerabilities that might otherwise lead to costly breaches.
Key Concepts to Master for Cost-Efficient Development
Understanding certain core concepts in Java will not only streamline your development process but also help reduce long-term costs.
1. Object-Oriented Programming (OOP)
Java is inherently an object-oriented language. Mastering OOP is crucial because it encourages reusable, modular code, which can significantly reduce ongoing maintenance costs.
Example: Creating a Basic Class
public class Car {
private String color;
private String model;
public Car(String color, String model) {
this.color = color;
this.model = model;
}
public void displayDetails() {
System.out.println("Car model: " + model + ", Color: " + color);
}
}
In this snippet, we define a Car
class with properties and a method to display details. By creating classes, you can encapsulate related functionalities, making your codebase easier to manage.
2. Effective Exception Handling
Good exception handling ensures that your applications run smoothly, providing a better user experience. This can lead to fewer error reports and reduced troubleshooting costs.
Example: Handling Exceptions
public void readFile(String fileName) {
try {
FileReader fileReader = new FileReader(fileName);
BufferedReader bufferedReader = new BufferedReader(fileReader);
String line;
while ((line = bufferedReader.readLine()) != null) {
System.out.println(line);
}
bufferedReader.close();
} catch (IOException e) {
System.err.println("File not found or unable to read: " + e.getMessage());
}
}
The code above illustrates responsive error handling to avoid application crashes. Handling exceptions gracefully provides stability and enhances user satisfaction, saving you costs related to support and debugging.
3. Use of Design Patterns
Learning and utilizing design patterns can greatly enhance the maintainability of your code and improve your team's productivity.
Example: Singleton Pattern
public class DatabaseConnection {
private static DatabaseConnection instance;
private DatabaseConnection() {
// Private constructor prevents instantiation from outside
}
public static synchronized DatabaseConnection getInstance() {
if (instance == null) {
instance = new DatabaseConnection();
}
return instance;
}
}
The Singleton pattern ensures that a class has only one instance, offering a global point of access. This saves resources by preventing multiple instantiations, thereby reducing memory consumption and potential errors.
Frameworks and Tools for Cost-Efficiency
Choosing the right frameworks can significantly enhance development efficiency while optimizing operational costs. Here are some essential frameworks to consider:
-
Spring Framework: Spring simplifies Java development with Dependency Injection and Aspect-Oriented Programming, enhancing modularity and testability. Utilizing Spring also decreases the time spent on boilerplate code.
-
Hibernate: For data management, Hibernate streamlines interaction with databases, allowing for easier querying and making it less error-prone. This can save costs associated with data management tasks.
-
Maven: Automating the build process with Maven reduces manual errors and ensures consistency across different environments, ultimately decreasing deployment time.
-
IntelliJ IDEA or Eclipse: Powerful Integrated Development Environments (IDEs) like IntelliJ and Eclipse can help catch errors during development, leading to better code quality and reduced debug times.
Cost-Saving Practices
Besides leveraging Java's features and libraries, applying cost-saving practices is crucial:
-
Code Reusability: Write reusable components. This practice minimizes code duplication and enhances maintainability.
-
Documentation: Always comment your code and provide documentation. This practice reduces onboarding time for new team members and is beneficial for future development.
-
Unit Testing: Implement test-driven development (TDD). Writing tests ensures your code works as intended, preventing regression bugs that can increase support costs.
-
Open Source Libraries: Use open-source libraries wherever possible. These can save you development time and costs while leveraging community support for improvements.
Real-World Example: Cost-Efficient Java Application Development
Consider a scenario where you decide to develop a simple e-commerce application. By applying the above practices, you could structure your application using a microservices architecture with Spring Boot. This can lead to independent deployments, faster development cycles, and lower long-term maintenance costs.
Moreover, if you decide to implement a loyalty program, you could refer to existing frameworks that provide utilities for such functionality. Instead of building from scratch, this can dramatically reduce both time and financial resources.
Bringing It All Together
Mastering Java not only opens doors to a rewarding career but also equips you with the tools to create cost-efficient applications. By leveraging Java’s OOP principles, effective exception handling, design patterns, and choosing the right frameworks, you are poised to develop applications that not only meet users’ needs but also operate within budget constraints.
For more cost-saving strategies, especially if you’re interested in optimizing resources in unpredictable times, check out the article “Survive the Savings: Promo Codes for Doomsday Preppers” at youvswild.com/blog/survive-savings-promo-codes-doomsday-preppers. The insights provided there can help you make informed decisions that lead to a financially savvy development approach.
By focusing on these essential Java skills and practices, you'll be well on your way to mastering the art of cost-efficient app development!
Checkout our other articles