Diving into Java: Tackling Asynchronous Challenges in Java
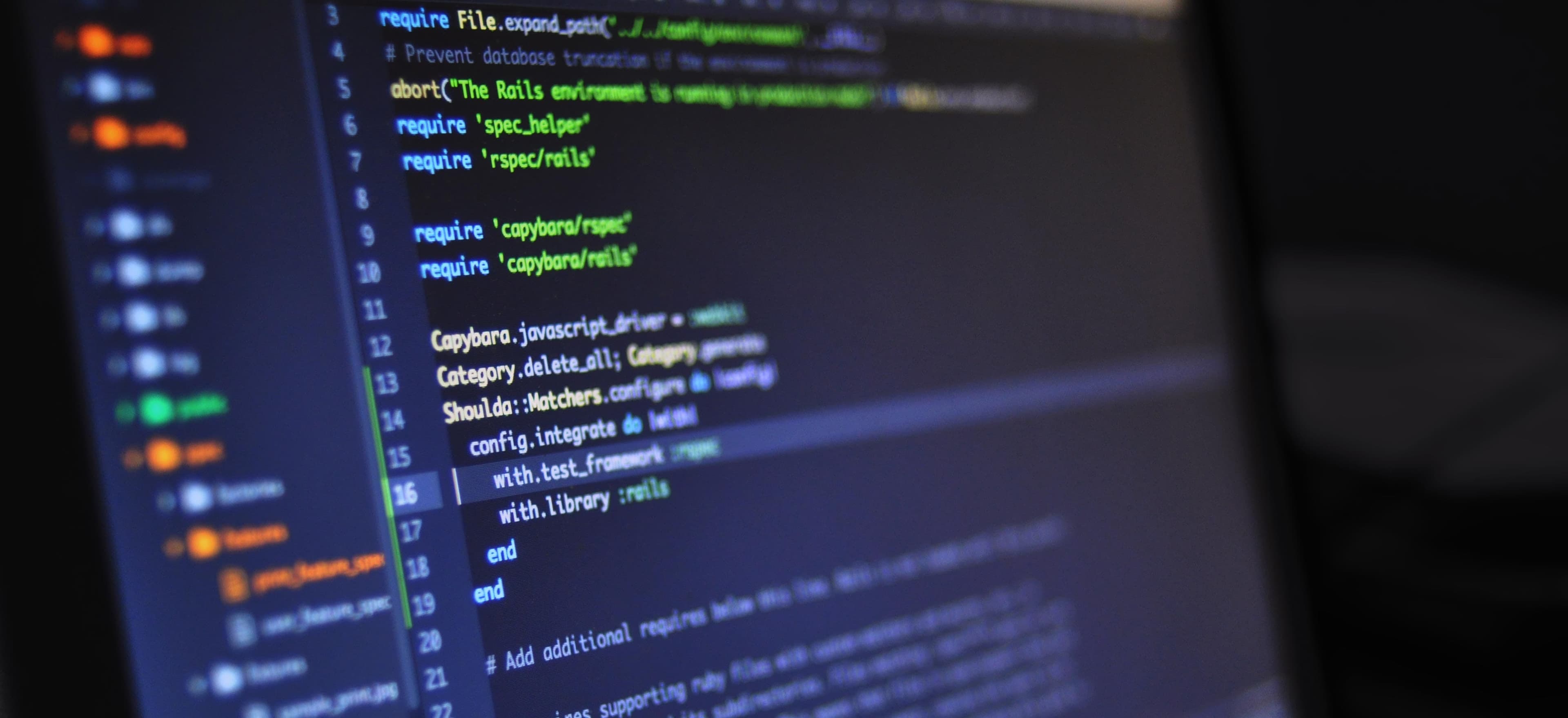
- Published on
Diving into Java: Tackling Asynchronous Challenges in Java
As Java developers, we often find ourselves navigating through complex applications that demand high performance and responsiveness. When it comes to handling asynchronous tasks, Java offers various paradigms and tools. In this blog post, we will explore the asynchronous programming landscape in Java, tackle some common challenges, and provide effective solutions using modern approaches.
Understanding Asynchronous Programming
Asynchronous programming allows a program to perform tasks concurrently, without blocking the main flow of execution. This model is beneficial in scenarios like network requests, file I/O operations, or lengthy computations, where we don't want to keep users waiting.
Before we dive deeper, it's worth noting that asynchronous programming isn't unique to Java. In JavaScript, for example, managing asynchronous flows can lead to challenges like "callback hell." If you're keen to learn more about that topic, check out this article on Unraveling Callback Hell: Mastering Async JS & Promises which provides a comprehensive overview.
Popular Approaches to Asynchronous Programming in Java
Java has evolved tremendously in handling asynchronous code. Let's review some of the popular approaches.
1. Threads
The most straightforward way to handle asynchronous tasks in Java is using threads. By creating separate threads for tasks, we can let them run while the main program continues executing.
Example:
public class AsyncThreadExample {
public static void main(String[] args) {
Thread thread = new Thread(() -> {
for (int i = 1; i <= 5; i++) {
System.out.println("Thread Task " + i);
try {
Thread.sleep(500); // Simulating a long-running task
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
});
thread.start();
// Main thread continues its execution
System.out.println("Main thread is not blocked.");
}
}
Why this code? This simple example demonstrates how to leverage threads to perform tasks without hindering the execution of the main program. It's a basic introduction, but the thread management can become complex with synchronization and state management, leading to potential pitfalls.
2. Executor Framework
Java's Executor framework provides a higher-level abstraction for managing threads, which can offer better flexibility and control.
Example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ExecutorServiceExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(3);
for (int i = 1; i <= 5; i++) {
final int taskId = i;
executor.submit(() -> {
System.out.println("Executing task " + taskId);
try {
Thread.sleep(1000); // Simulating a long-running task
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.println("Finished task " + taskId);
});
}
executor.shutdown();
System.out.println("All tasks submitted.");
}
}
Why this code? The ExecutorService
manages a pool of threads, making it easier to handle multiple concurrent tasks. It offers better resource management compared to manually handling threads, giving developers a more scalable way to execute long-running tasks.
3. CompletableFuture
Introduced in Java 8, CompletableFuture
provides a powerful way to manage asynchronous computations. It allows you to write non-blocking code, handle results, and compose asynchronous tasks.
Example:
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture.supplyAsync(() -> {
// Simulating a long-running operation
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Result from Async Task";
})
.thenAccept(result -> System.out.println("Received: " + result));
System.out.println("This prints before the async task completes!");
// Wait for the async task to complete (for demo purposes)
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Why this code? The use of CompletableFuture
illustrates how to initiate an asynchronous task and handle the result without blocking the main flow. It enables more readable and maintainable asynchronous code.
4. Reactive Programming
For applications requiring more complex asynchronous flows, reactive programming paradigms using libraries like Project Reactor or RxJava can be quite powerful. They embrace an event-driven approach and deal with streams of data.
Example:
import reactor.core.publisher.Flux;
public class ReactiveExample {
public static void main(String[] args) {
Flux.just("A", "B", "C")
.map(String::toLowerCase)
.subscribe(System.out::println);
}
}
Why this code? Reactive programming with libraries like Reactor allows for seamless data transformations and handling of asynchronous streams in a highly declarative manner. This approach can help in developing efficient and scalable applications.
Handling Common Asynchronous Challenges
While working with async programming in Java, you may encounter challenges such as error handling, task cancellation, and coordinating multiple tasks. Here are some strategies to address common issues.
Error Handling
Error handling in asynchronous tasks can be particularly tricky. Java's CompletableFuture
simplifies this aspect with methods like handle()
and exceptionally()
.
Example:
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
throw new RuntimeException("Error occurred!");
}).exceptionally(ex -> {
System.out.println("Handling error: " + ex.getMessage());
return "Error handled";
});
Why this code? This snippet handles exceptions thrown during asynchronous operations, ensuring robust error management.
Task Cancellation
You can cancel ongoing tasks using controls in Java’s Executor framework. However, be cautious as cancellation is not always straightforward.
Example:
ExecutorService executor = Executors.newSingleThreadExecutor();
Future<?> future = executor.submit(() -> {
while (!Thread.currentThread().isInterrupted()) {
// Do some work
}
});
future.cancel(true); // Attempt to cancel the task
executor.shutdown();
Why this code? Here, we demonstrate how to cancel an ongoing task cleanly and safely to avoid leaving abandoned threads.
Coordinating Multiple Tasks
When working with multiple asynchronous tasks that depend on each other, it’s vital to maintain order and dependencies. The allOf()
method in CompletableFuture
can help you wait for multiple futures to complete.
Example:
CompletableFuture<Void> future1 = CompletableFuture.runAsync(() -> {
// Task 1
});
CompletableFuture<Void> future2 = CompletableFuture.runAsync(() -> {
// Task 2
});
CompletableFuture<Void> combinedFuture = CompletableFuture.allOf(future1, future2);
combinedFuture.join(); // Wait for both tasks to complete
Why this code? This example demonstrates how to synchronize multiple asynchronous tasks effectively, allowing developers to manage dependencies easily.
The Bottom Line
Java's flexibility in handling asynchronous programming can significantly enhance the performance and responsiveness of applications. From basic thread management to advanced concepts like reactive programming, understanding these concepts is crucial for every Java developer.
As we further engage with async paradigms, it's essential to continuously learn and adapt, especially when bridging with different programming languages and their approaches, like JavaScript as highlighted in the article on Unraveling Callback Hell: Mastering Async JS & Promises.
Invest the time to understand these paradigms, explore their use cases, and experiment with the code samples provided. As you gain experience, your proficiency in asynchronously tackling challenges in Java will undoubtedly rise, positioning you as a competent developer in an ever-evolving landscape. Happy coding!
Checkout our other articles