Taming Asynchronous Java Code: Avoiding Callback Chaos
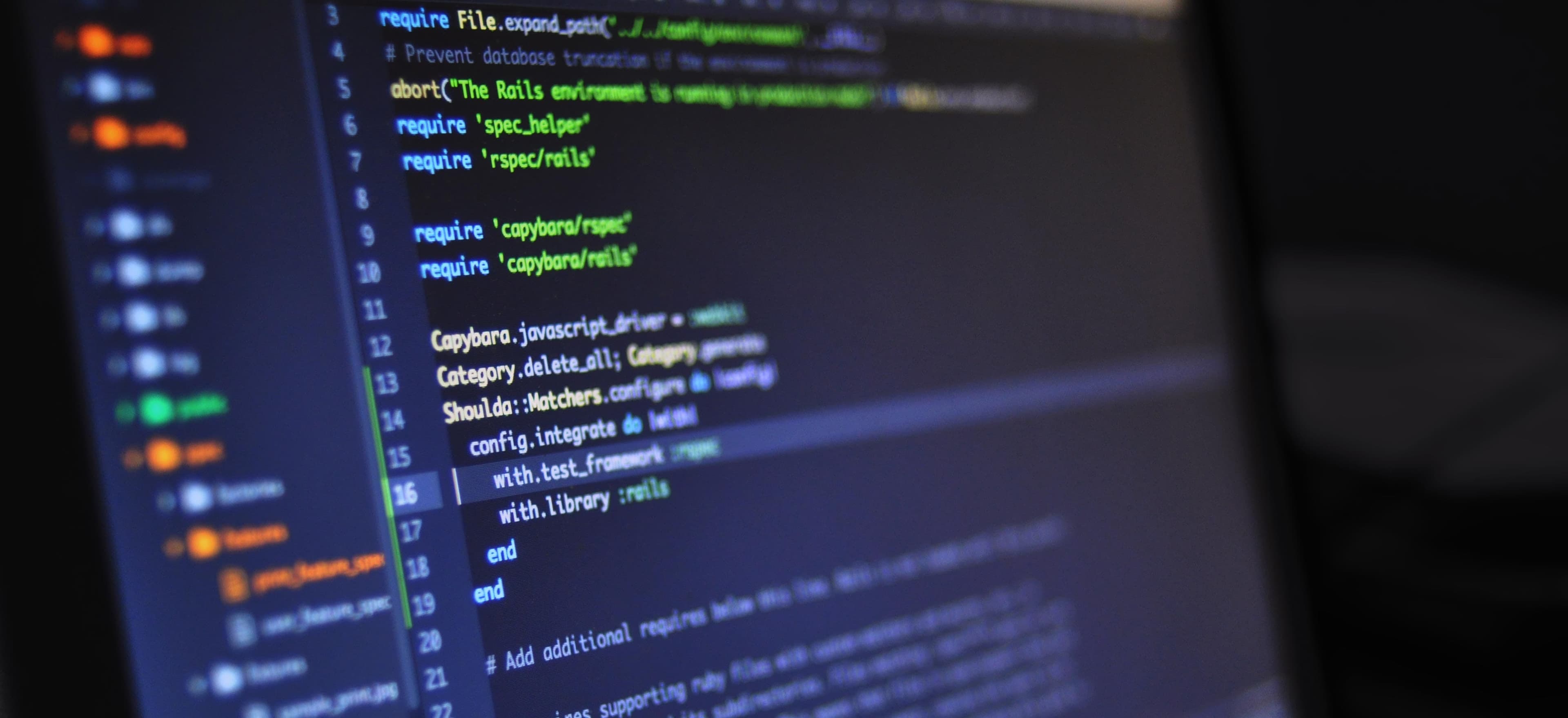
- Published on
Taming Asynchronous Java Code: Avoiding Callback Chaos
In modern programming, especially web development, asynchronous operations are crucial for building efficient and responsive applications. However, when poorly managed, these operations can lead to what developers often refer to as "callback hell." This phenomenon can result in code that is deeply nested and difficult to read or maintain. Today, we will explore how to manage asynchronous programming in Java effectively, drawing on techniques that can help you avoid callback chaos.
Understanding Asynchronous Programming in Java
Asynchronous programming allows for the execution of tasks in a non-blocking manner. This means that the program can continue executing while waiting for an operation like reading a file or making an HTTP request to complete. This model is especially important in environments such as web servers, where blocking I/O operations can severely degrade performance.
Why Avoid Callback Hell?
-
Readability: Deeply nested callbacks make code difficult to read and understand. When reading through code that looks like an inverted pyramid, it becomes challenging to follow the logic.
-
Maintainability: As code grows, maintaining it becomes increasingly complicated. Fixing bugs or adding features may require navigating through layers of nested callbacks.
-
Error Handling: Error handling becomes cumbersome when it is embedded deeply within callback structures. It is prone to missing errors thrown in outer callbacks, leading to unhandled exceptions.
To illustrate these points, let’s consider a simple Java example using traditional callback functions.
public void fetchData(final Callback callback) {
httpRequest.send("GET", "https://api.example.com/data", new Callback() {
@Override
public void onResponse(Data data) {
processData(data, new Callback() {
@Override
public void onProcessed(ProcessedData processedData) {
callback.onComplete(processedData);
}
});
}
});
}
In this code snippet, each asynchronous operation leads to a new level of nested callbacks. The deeper we go, the more complex it becomes. Now, let’s explore more structured approaches to asynchronous programming in Java that can help tame callback chaos.
Approaches to Avoid Callback Hell
1. Promises
Promises serve as an elegant solution to handling asynchronous operations. They represent a value that may be available now, or in the future, or never. With the introduction of frameworks like CompletableFuture in Java 8 and later, we can manage async programming with greater ease.
Example: CompletableFuture
import java.util.concurrent.CompletableFuture;
public void fetchDataAsync() {
CompletableFuture.supplyAsync(() -> httpRequest.send("GET", "https://api.example.com/data"))
.thenApply(data -> processData(data))
.thenAccept(processedData -> {
// Handle completed data
System.out.println("Data processed: " + processedData);
})
.exceptionally(ex -> {
// Error handling
System.err.println("Error occurred: " + ex.getMessage());
return null;
});
}
Why Use CompletableFuture?
- Chaining: It allows for chaining multiple asynchronous operations without deeply nesting callbacks.
- Clarity: The flow of operations is much clearer, enabling easier debugging and maintenance.
- Error Handling: The
exceptionally
method provides a dedicated space for error handling, making your code cleaner and more robust.
2. Reactive Programming
Reactive programming is another paradigm to handle asynchronous data streams and events. This model empowers developers to respond to changes and integrate asynchronized processes seamlessly.
In Java, you can utilize the Reactor library or RxJava to implement reactive programming concepts.
Example: Using Reactor
import reactor.core.publisher.Mono;
public void fetchDataReactor() {
Mono<Data> dataMono = Mono.fromCallable(() -> httpRequest.send("GET", "https://api.example.com/data"));
dataMono
.map(data -> processData(data))
.subscribe(
processedData -> System.out.println("Data processed: " + processedData),
error -> System.err.println("Error occurred: " + error.getMessage())
);
}
Why Choose Reactive Programming?
- Non-Blocking: It inherently supports non-blocking operations, leading to better resource utilization especially in high-throughput systems.
- Composability: The functional style allows for a clean and composable way to handle data transformations, making your code more flexible.
Best Practices for Asynchronous Java Programming
-
Prefer Higher-Level Abstractions: Use CompletableFuture or reactive programming libraries to manage async logic instead of raw callbacks.
-
Keep Your Methods Small: Break complex logic into smaller methods for better readability and maintainability. This also helps localize errors.
-
Clear Error Handling: Always consider how errors will be handled and ensure that your error-handling logic is as clean as your success logic.
-
Test Your Code: As with all programming patterns, testing is key. Be sure to write unit tests for asynchronous code to ensure it behaves as expected.
The Bottom Line
Asynchronous programming in Java doesn't have to result in callback chaos. By adopting modern practices such as using CompletableFuture or exploring reactive programming, you can maintain clear and effective code. These tools provide not only cleaner structures but also promote better error handling strategies, making the development process more efficient.
If you want to delve deeper into asynchronous programming techniques and their challenges, consider checking out this insightful article on JavaScript: Unraveling Callback Hell: Mastering Async JS & Promises. Understanding how asynchronous logic works in one language can often provide valuable insights into tackling similar challenges in another.
By following these strategies and principles, you can master the art of asynchronous programming in Java, elevating your code quality while taming asynchronous chaos effectively. Happy coding!
Checkout our other articles