Preventing External Links from Slowing Down Your Java App
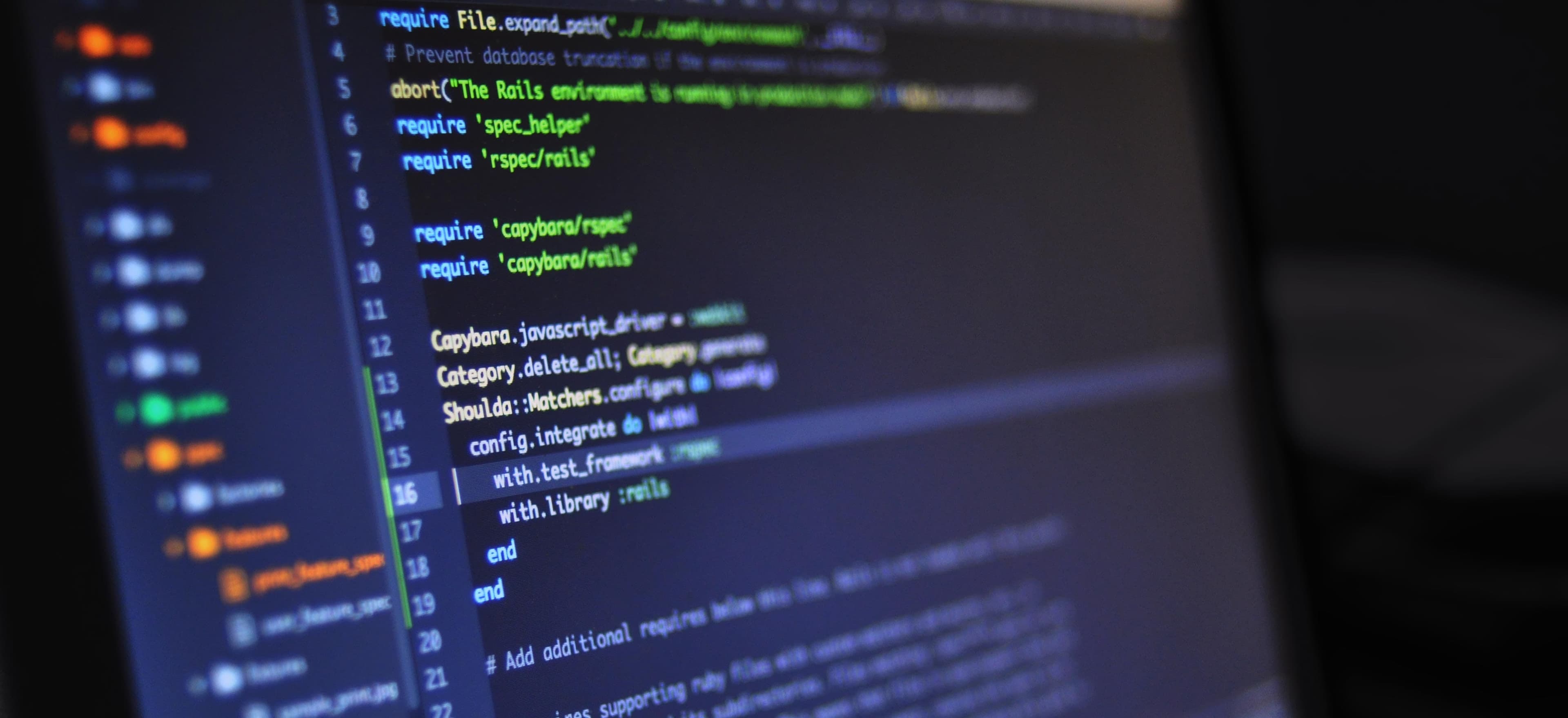
- Published on
Preventing External Links from Slowing Down Your Java App
In the era of digital connectivity, Java applications often rely on external resources. This can enhance functionality, improve user experience, and accelerate application development. However, it introduces a potential issue: external links can slow down your application and disrupt user engagement. This blog post will explore strategies to prevent external links from hampering your Java app's performance while also providing actionable insights relative to the guidelines in the article How to Stop External Links from Disrupting Your Site's Load.
Understanding the Impact of External Links
External links are hyperlinks that direct users to a domain outside your own application. While they may serve legitimate purposes, such as API calls or third-party libraries, they come with risks. Delays in response times from these external sites can result in slower load times for your application, directly impacting user satisfaction and application performance.
The Connection Between Performance and User Experience
In the realm of web applications, speed is paramount. Studies show that users abandon a page if it takes longer than a few seconds to load. A Java application that depends heavily on responsive external resources can experience significant lag, resulting in a negative experience. Therefore, addressing the performance impact of external links is essential for maintaining user engagement.
Key Strategies to Optimize External Link Usage
1. Asynchronous Calls
One of the most effective ways to manage external links is to use asynchronous calls. This approach prevents the main execution thread from being blocked while waiting for external resources.
import java.util.concurrent.CompletableFuture;
public class AsyncExample {
public String fetchDataFromExternalApi(String url) {
return CompletableFuture.supplyAsync(() -> {
// Simulate a delay for the external API call
try {
Thread.sleep(2000); // Simulated delay
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Data fetched from " + url;
}).join(); // This will wait for the completion
}
}
Why This Works: Using CompletableFuture
allows your application to continue working on other tasks while waiting for the data to be fetched, significantly improving the user experience.
2. Caching Responses
Caching is another excellent strategy to reduce the dependency on external links. By storing previously fetched data, your application will use the cache instead, avoiding the need to make repeated external calls.
import java.util.HashMap;
import java.util.Map;
public class CachingExample {
private Map<String, String> cache = new HashMap<>();
public String fetchData(String url) {
// Check cache before fetching
if (cache.containsKey(url)) {
return cache.get(url);
}
// Simulate fetching data
String fetchedData = "Fetched Data from " + url;
cache.put(url, fetchedData); // Store it in cache
return fetchedData;
}
}
Why This Works: By using a caching mechanism, you reduce the number of requests made to external links, which can drastically improve load times. This is particularly useful for data that does not change frequently.
3. Using Local Resources When Possible
Incorporating local resources reduces your dependency on external links, thereby cutting down on potential delays.
public class LocalResourceExample {
public String getLocalData() {
return "Local Data Engineered!";
}
}
Why This Works: By relying on resources stored locally within your application, you eliminate external call latencies altogether. Whenever possible, consider using local resources for better efficiency.
4. Utilizing Load Balancing
Implementing load balancers can help optimize requests to external links by distributing traffic across multiple servers, significantly improving response times.
import java.util.List;
import java.util.Random;
public class LoadBalancer {
private List<String> servers;
public LoadBalancer(List<String> servers) {
this.servers = servers;
}
public String getServer() {
Random rand = new Random();
return servers.get(rand.nextInt(servers.size())); // Randomly choose a server
}
}
Why This Works: Load balancing reduces the load on any single server and can lead to faster response times since users are directed to the most responsive/available server.
5. Monitoring and Optimization
Continuous monitoring helps you assess which external links are causing delays. You can leverage tools like APM (Application Performance Management) tools such as New Relic or Dynatrace.
public class MonitoringExample {
public void monitorPerformance() {
// Integrate monitoring tools here
}
}
Why This Works: By identifying performance bottlenecks, you can make informed decisions about what to optimize or replace, potentially leading to significant performance improvements.
My Closing Thoughts on the Matter
Managing external resources effectively is crucial for creating responsive Java applications. By adopting techniques such as asynchronous programming, caching, using local resources, and implementing load balancing, you can enhance the performance and reliability of your application.
This blog also emphasizes the value of continuous monitoring to ensure your application performs optimally as external dependencies evolve. For more guidance on handling external links, be sure to check out the insightful article How to Stop External Links from Disrupting Your Site's Load.
Further Reading
- Explore more on Java Best Practices for performance tuning.
- Understanding CORS for secure linking to external resources.
By continuously optimizing your Java application and being mindful of external link usage, you can ensure a seamless user experience that keeps your audience engaged.