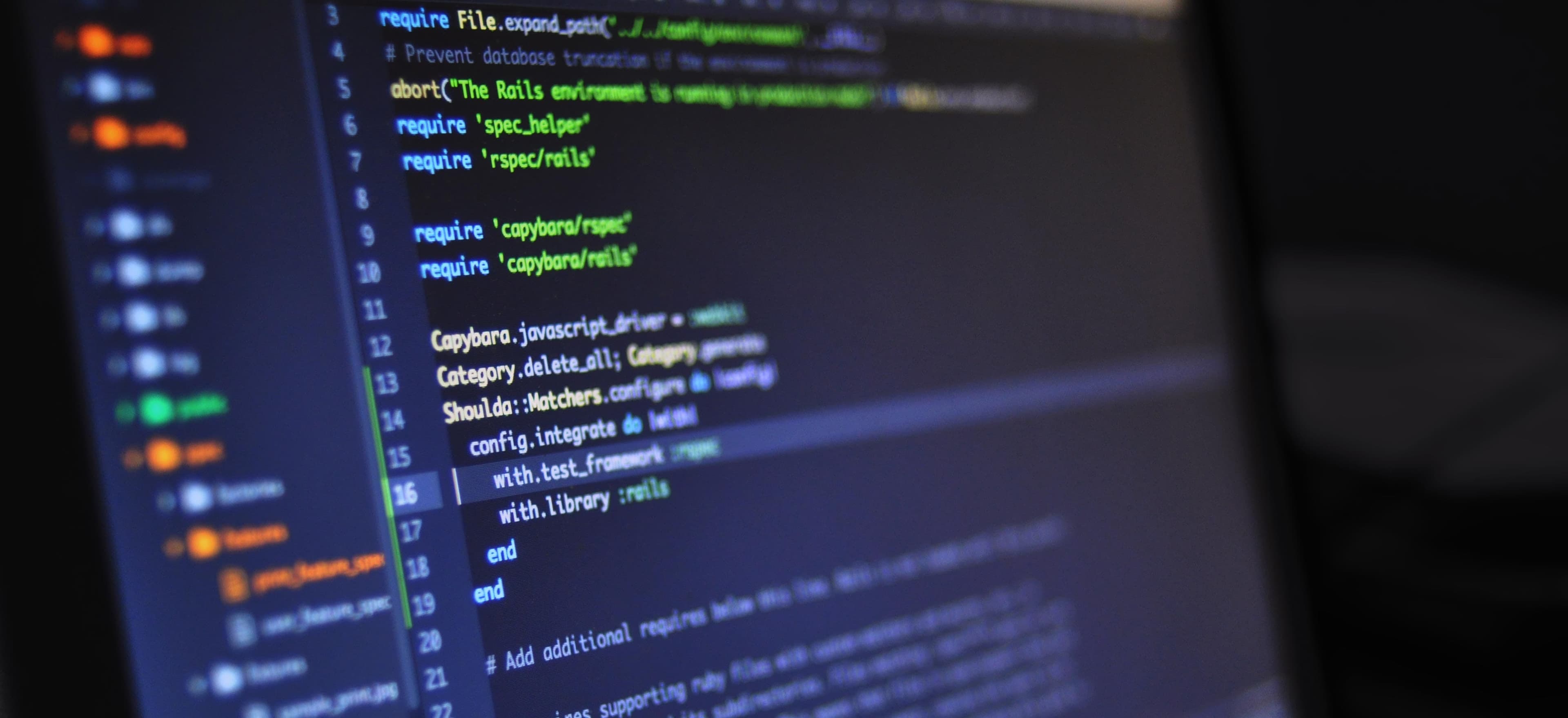
- Published on
Protecting Your Java Web App's Mailto Links from Spammers
In the digital age, protecting user data has become a paramount concern for web developers. One of the often-overlooked vulnerabilities in web applications is the exposure of email addresses via mailto links. These links can easily be harvested by spammers, leading to unwanted email solicitations that can seriously impact user experience and privacy. If you're building a Java web application, you're in luck. Today, we're going to discuss effective strategies to guard your mailto links against spam.
Why Mailto Links are Vulnerable
Mailto links serve as an easy and convenient method for users to contact you via email. However, as simple as they are, mailto links can provide spammers with a pathway directly to your users' inboxes. When web crawlers scrape your website, identifying these mailto links can be as easy as scanning HTML markup, leading to your email being added to spam lists.
Example of a Simple Mailto Link
<a href="mailto:example@domain.com">Contact Us</a>
While this code provides a straightforward way for users to reach out, it poses a significant risk by exposing the email address directly to any spam bot that visits the page.
Strategies to Protect Mailto Links
To mitigate the risks associated with spam, developers can employ several methods. Let’s explore the most effective strategies you can use in your Java web application.
1. Obfuscation Techniques
Obfuscation is the process of making something obscure. With email addresses, this commonly involves breaking apart the email string into fragments so that algorithms designed to harvest email addresses can't easily recognize them.
Example: JavaScript Obfuscation
A commonly used technique involves JavaScript to dynamically create the email address:
<script>
var user = "example";
var domain = "domain.com";
var link = user + "@" + domain;
document.write('<a href="mailto:' + link + '">' + link + '</a>');
</script>
Why This Works: By using JavaScript to write the mailto link to the page, search engine bots that load the page just as text won't easily find the email address unless they can execute the JavaScript.
2. Use of Captchas
Integrating a Captcha method helps verify that a human, rather than a bot, is trying to access the mailto link.
Example: Simple Java Integration
Using Google’s reCAPTCHA in a Java web app can significantly reduce automated spam submissions:
- Include reCAPTCHA in your HTML form:
<form action="/sendMail" method="POST">
<input type="text" name="userMessage" placeholder="Your message">
<div class="g-recaptcha" data-sitekey="your-site-key"></div>
<button type="submit">Send</button>
</form>
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
- Java Servlet Handling:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String reCaptchaResponse = request.getParameter("g-recaptcha-response");
// Validate the reCAPTCHA response
boolean isHuman = validateCaptcha(reCaptchaResponse);
if (isHuman) {
// Proceed with sending email
} else {
// Handle the spammer case
}
}
private boolean validateCaptcha(String response) {
// Implement your validation logic
return true; // Placeholder
}
Why This Works: The Captcha ensures that only users capable of interacting in a human manner can access functionalities that utilize mailto links, greatly reducing spam emails.
3. Using Contact Forms
Redirecting users to a contact form instead of using mailto links can keep sensitive information hidden while providing a better user experience.
Example of a Contact Form
<form action="/sendMail" method="POST">
<input type="text" name="name" required placeholder="Your Name">
<input type="email" name="email" required placeholder="Your Email">
<textarea name="message" required placeholder="Your Message"></textarea>
<button type="submit">Send Message</button>
</form>
Why This Works: Contact forms do not expose email addresses directly. Instead, they route user messages through your server, where additional filtering and verification can occur.
Using Server-Side Validation
Regardless of the methods employed on the front end, ensuring robust server-side validation is critical.
Java Email Sending Logic
Here’s a simple Java code snippet that demonstrates how to handle incoming messages from the contact form.
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("name");
String email = request.getParameter("email");
String message = request.getParameter("message");
if(isValidEmail(email)) {
sendEmail(name, email, message);
response.getWriter().write("Email sent successfully.");
} else {
response.getWriter().write("Invalid email address.");
}
}
private boolean isValidEmail(String email) {
// Regex for validating email
String regex = "^[A-Za-z0-9+_.-]+@(.+)$";
return email.matches(regex);
}
private void sendEmail(String name, String email, String message) {
// Implement your email sending logic (SMTP configuration, etc.)
}
Why This Works: Server-side validation acts as a secondary layer of defense, filtering out potential spam and ensuring that only legitimate emails are processed.
Best Practices for Java Mailto Protection
-
Keep Email Addresses Hidden: Utilize JavaScript and server-side coding techniques to conceal email addresses.
-
Implement Robust Form Features: Use Captchas and validate each submission rigorously before processing.
-
Maintain Regular Updates: Ensure that libraries used for email sending and validation are regularly updated, keeping security tight.
-
User Education: Educate your users regarding phishing schemes and suspicious emails.
The Closing Argument
Protecting your Java web app's mailto links from spammers is not just a good practice—it's vital for ensuring user trust and security. The combination of obfuscation, Captcha methods, contact forms, and server-side validation can go a long way in keeping your users’ data safe.
For more detailed information on protecting mailto links with JavaScript, be sure to check out the article Shield Your Mailto Links from Spammers with JavaScript.
By implementing these strategies, you can effectively limit exposure to spam and create a better online experience for your users.
Checkout our other articles