Protecting Your Java Web App's Email Links from Spam Attacks
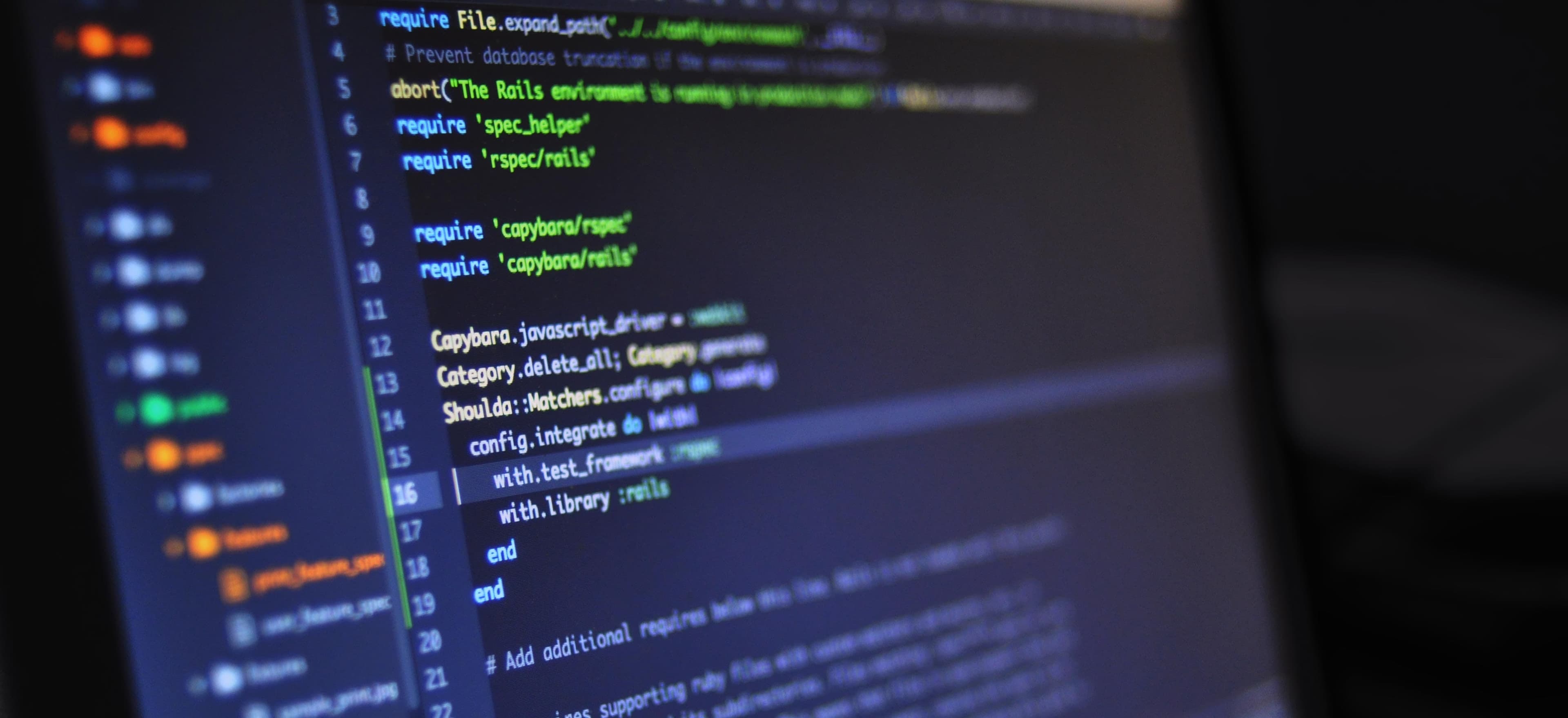
- Published on
Protecting Your Java Web App's Email Links from Spam Attacks
In the era of digital communication, email remains one of the most important channels for interaction. Unfortunately, it is also a prime target for spammers. If you have a Java web application, protecting your email links should be a priority. In this post, we’ll delve into practical strategies to secure email links from spam attacks while maintaining usability for your users.
Why Shield Your Email Links?
Turning your attention to email links, a common practice involves using "mailto" links. These clickable email addresses make it easy for users to connect via email. However, they can be crawled by automated bots, leading to spam. Thus, the question arises: how do we effectively shield these from spammers while ensuring our users can still send emails without obstacles?
Understanding the Email Link Problem
When you use a standard "mailto" link:
<a href="mailto:example@mail.com">Email Us</a>
Anyone can scrape this link from your page using a bot. This exposes your email address to unsolicited contact and spam emails.
Reference to Existing Solutions
It's essential to stay informed and learn from innovative techniques applied by others in the field. For example, the article titled "Shield Your Mailto Links from Spammers with JavaScript" discusses various effective methods of safeguarding email links. You can find it here for more insights on utilizing JavaScript for email protection.
Using Java to Protect Email Links
Method 1: Encoding Email Addresses
One practical approach is to encode your email addresses in a way that is not readable by bots. This can be achieved by breaking the address into segments. Here’s how you can implement it in Java:
Example Code Snippet
public class EmailEncoder {
public static String encodeEmail(String email) {
StringBuilder encodedEmail = new StringBuilder();
for (char c : email.toCharArray()) {
if (Character.isLetterOrDigit(c)) {
encodedEmail.append(c);
} else {
// Convert special characters to HTML entities (e.g., '@' = '@')
encodedEmail.append("&#").append((int) c).append(';');
}
}
return encodedEmail.toString();
}
public static void main(String[] args) {
String email = "example@mail.com";
System.out.println(encodeEmail(email));
}
}
Why Use This Approach?
By encoding the email, you make it harder for automated bots to recognize it. In our example, the '@' character is converted to its respective HTML entity. Browsers will render the encoded email correctly, allowing users to click it effortlessly, while bots struggle to decode it.
Method 2: Using a Java Servlet
Another effective approach is to serve email links dynamically from your backend. By generating email addresses on the server-side, you can minimize exposure. Here’s how you might implement a Java Servlet for this purpose.
Example Servlet
@WebServlet("/emailLink")
public class EmailLinkServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String email = "example@mail.com"; // fetch from a secure location or configure appropriately
response.setContentType("text/plain");
response.getWriter().write(email);
}
}
Why a Servlet?
Using a Servlet adds a layer of protection. The email link is requested from the server, and bots won’t see it in the HTML source code. Instead, they'll only encounter a route they cannot access or interpret—enhancing overall security.
Utilizing JavaScript as an Additional Layer
While Java offers robust server-side solutions, combining these with client-side techniques may enhance your defenses even further. As mentioned in the previously referenced article, JavaScript can obfuscate email addresses. Here’s a simple implementation.
Example JavaScript Code
<script>
function encodeEmail(email) {
return email.replace(/@/, ' [at] ').replace(/\./g, ' [dot] ');
}
document.addEventListener('DOMContentLoaded', function() {
var emailAddress = document.createElement('a');
emailAddress.href = 'mailto:example@mail.com';
emailAddress.textContent = encodeEmail('example@mail.com');
document.getElementById('email-container').appendChild(emailAddress);
});
</script>
<div id="email-container"></div>
Why Use JavaScript Here?
This JavaScript snippet converts the email address into a user-friendly format for display purposes while still creating a functional "mailto" link. Spambots may fail to recognize the address, as they typically look for the "@" symbol and a dot, making your email less vulnerable.
Testing the Solutions
After implementing your Java and JavaScript solutions, comprehensive testing is crucial. Ensure the email links function as expected in all browsers and devices. You might consider using browser console tools to simulate bot scraping behavior and evaluate how effective your solutions have been.
Final Thoughts
In summary, protecting your Java web application's email links from spam attacks is vital for maintaining a clean inbox. Employing a combination of encoding techniques, server-side solutions, and client-side JavaScript can significantly mitigate risks.
It’s not just about functionality; it’s about user experience and security. By following the methods discussed in this post and utilizing the insights shared from the referenced article, you can create a robust defense against unwanted spam attacks.
For more insights on ensuring email link safety, refer to the article "Shield Your Mailto Links from Spammers with JavaScript" here.
Stay vigilant and proactive in safeguarding your Java web application’s email interactions!
Checkout our other articles