Preventing External Link Issues in Your Java Web App
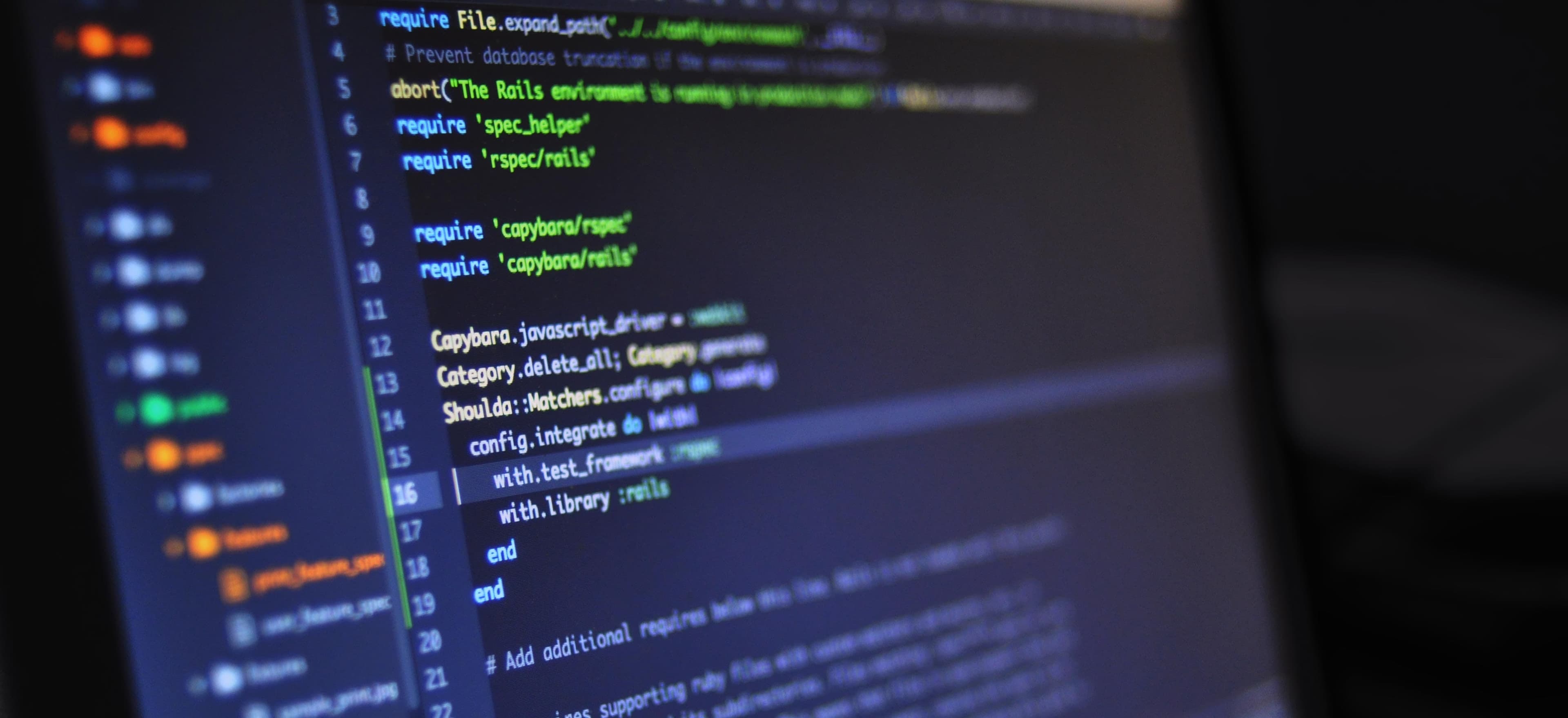
- Published on
Preventing External Link Issues in Your Java Web App
In the ever-evolving world of web development, maintaining a seamless user experience is fundamental. One common pitfall that developers encounter involves external links that can disrupt site performance. In this blog post, we will explore how to prevent external link issues in your Java web applications, highlighting practical solutions and best practices you can implement today.
Understanding External Links
What Are External Links?
External links are hyperlinks that point to domains other than your own. While they can provide valuable resources for users, unchecked external links may lead to slower page load times and negatively affect search engine optimization (SEO).
The Impact of External Links
When a user clicks on an external link, their browser must load content from another server. If that server is slow or unresponsive, it can create a lag on your website, leading to a frustrating user experience. This becomes even more critical in Java web applications, where performance is paramount.
As discussed in the article "How to Stop External Links from Disrupting Your Site's Load", managing these links effectively is crucial for overall site performance.
Best Practices for Handling External Links
To mitigate the risks associated with external links in your Java web applications, consider implementing the following best practices:
1. Use Asynchronous Loading
One of the most effective strategies for handling external links is to load them asynchronously. By doing so, your main webpage can load independently of external resources, enhancing speed and user experience.
Here’s a code snippet that demonstrates how you might implement this in a Java web application:
// Assuming you're using Java Servlet for handling requests
@WebServlet("/loadExternal")
public class ExternalLinkServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Simulating delay in loading an external resource
String jsonData = fetchExternalData();
response.setContentType("application/json");
response.getWriter().write(jsonData);
}
private String fetchExternalData() {
// Logic to fetch data asynchronously
// Placeholder for better integration with third-party APIs
return "{\"status\":\"data loaded\"}";
}
}
In the code above, the external data is fetched in a way that does not block the loading of the full page. This approach helps maintain a fast loading time on your web app, even when dealing with external resources.
2. Lazy Loading of External Resources
Another effective method is to lazy load external resources. Critical assets load first, and as the user scrolls or interacts with the page, non-critical resources are fetched.
Here’s an example using JavaScript:
<script>
document.addEventListener("DOMContentLoaded", function() {
const lazyLoad = () => {
const links = document.querySelectorAll(".external-link");
links.forEach(link => {
if (link.getBoundingClientRect().top < window.innerHeight) {
link.href = link.dataset.href; // Sets href when in view
link.classList.remove("external-link");
}
});
// Remove event listener if there are no more links to lazy load
if (links.length === 0) {
window.removeEventListener("scroll", lazyLoad);
}
};
window.addEventListener("scroll", lazyLoad);
lazyLoad(); // Initial check
});
</script>
<a class="external-link" data-href="https://externalresource.com/data">Load External Resource</a>
In this sample, the external link is only set when it comes into the viewport. This efficient method reduces initial load time and bandwidth usage.
3. Leverage Content Delivery Networks (CDNs)
If your Java web app performs extensive operations with external resources, consider using a CDN. CDNs cache external content nearby to users, decreasing load times significantly.
For example, when integrating a popular library, you can load it from a CDN:
<script src="https://cdn.jsdelivr.net/npm/jquery/dist/jquery.min.js"></script>
Using a CDN not only enhances speed but also relies on optimized servers, reducing downtime and providing a better experience for your users.
4. Implement Fallback Strategies
Suppose an external resource fails to load. In that case, you should implement fallback mechanisms to maintain functionality.
Below is an example of a fallback:
<script>
function loadScript(url, fallback) {
const script = document.createElement("script");
script.src = url;
script.onerror = () => {
console.log("Loading failed, using fallback.");
const fallbackScript = document.createElement("script");
fallbackScript.src = fallback;
document.body.appendChild(fallbackScript);
};
document.body.appendChild(script);
}
loadScript("https://externalresource.com/library.js", "local-library.js");
</script>
In this case, if the external script fails to load, the application gracefully falls back on a local resource, ensuring seamless user experience.
5. Monitor External Link Performance
Lastly, continuously monitoring the performance of external links is crucial. Tools like Google PageSpeed Insights and GTmetrix can help assess how these links affect your site and highlight areas for improvement.
Establishing regular checks can ensure that any slow or unresponsive links are addressed promptly, further enhancing user experience.
Bringing It All Together
Effectively managing external links in your Java web application is not only essential for performance but also indispensable for providing a satisfying user experience. By employing techniques such as asynchronous loading, lazy loading, leveraging CDNs, implementing fallback strategies, and consistently monitoring performance, you can prevent external link issues and keep your web application running smoothly.
For more insights and best practices on managing links and improving performance, be sure to check out How to Stop External Links from Disrupting Your Site's Load.
By taking a proactive approach and implementing the strategies outlined in this blog, you'll enhance your Java web app's reliability and user satisfaction, leading to higher engagement and better SEO results.
Checkout our other articles