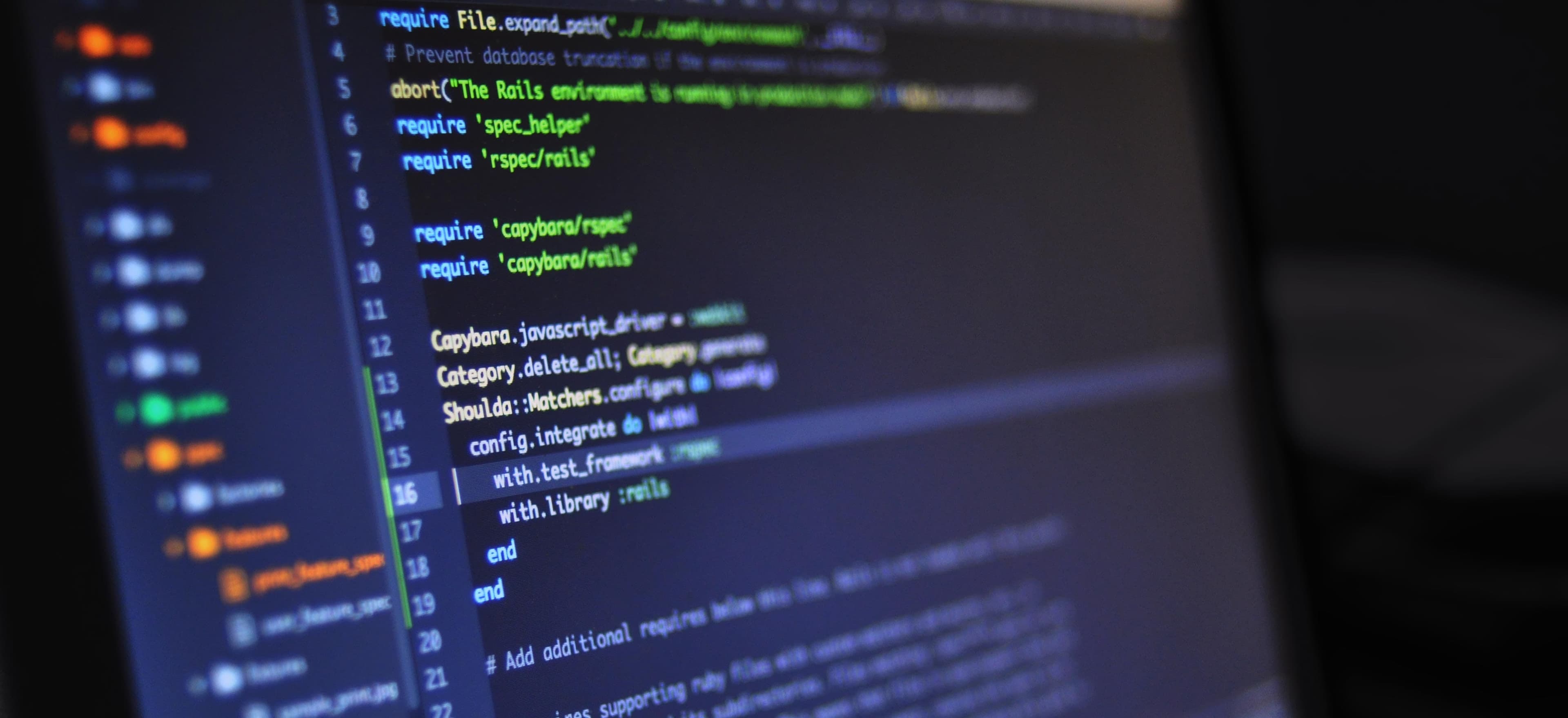
- Published on
Protect Your Java App's Mailto Links from Spam Attackers
Email is a vital communication tool used in web and app development, and for many Java applications, providing users with a way to contact you directly through mailto links is essential. However, these links are prime targets for spammers. In these evolving times of technology, ensuring that your mailto links remain shielded from spam bots is as important as ever. In this blog post, we'll explore methods to defend your Java application’s mailto links from spam attackers while diving deep into code snippets, providing clear explanations of why each step is critical.
Understanding the Risks
Spam bots scour the web looking for email addresses to add to their distributions lists. When they find a mailto link, they can automate sending thousands of unwanted emails. This not only clutters your inbox but can also harm your domain reputation.
The Traditional Approach: Using Mailto Links Directly
Many developers implement mailto links directly into their HTML like this:
<a href="mailto:yourname@example.com">Contact Us</a>
While this approach seems straightforward, it's akin to inviting spammers right through your front door. To mitigate this risk, we must be more strategic.
A Better Solution: JavaScript Obfuscation
Understanding the Concept
Obfuscating a mailto link via JavaScript can effectively shield your email from being harvested by spam bots. Rather than inserting the email address directly into the HTML, we can use JavaScript to dynamically generate the mailto link. This method keeps the direct email from appearing in the HTML source code, significantly reducing the chances of spam bots capturing it.
Implementation in Java
Let's illustrate how you can obfuscate a mailto link using a simple Java example. First, we will set up a basic servlet that generates the email link dynamically.
Step 1: Create a Servlet
Here's how to create a servlet that handles the mailto functionality:
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet("/contact")
public class EmailContactServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
// Construct email link
String name = "yourname";
String domain = "example.com";
String email = name + "@" + domain;
out.println("<html><body>");
out.println("<a href=\"mailto:" + email + "\">Contact Us</a>");
out.println("</body></html>");
}
}
Why This Approach Works
-
Dynamic Email Generation: By constructing the email using string concatenation in the servlet, we make it harder for simple bots to identify the email address immediately.
-
Server-Side Execution: The email is only generated when a user makes a request to the servlet, meaning the direct email address never appears in the client-side source code.
Further Protection: Using JavaScript
We can amplify the spam protection by implementing a client-side JavaScript solution to obscure the email link completely. Here’s how to enhance our earlier servlet:
Step 2: Add JavaScript to Obfuscate Further
You can add the following code to your servlet's output:
<script>
var name = "yourname";
var domain = "example.com";
var email = name + "@" + domain;
document.write('<a href="mailto:' + email + '">Contact Us</a>');
</script>
The Complete Servlet Code
Bringing it all together, here’s how the servlet could look:
@WebServlet("/contact")
public class EmailContactServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<script>");
out.println("var name = 'yourname';");
out.println("var domain = 'example.com';");
out.println("var email = name + '@' + domain;");
out.println("document.write('<a href=\"mailto:' + email + '\">Contact Us</a>');");
out.println("</script>");
out.println("</body></html>");
}
}
Why Use Client-Side JavaScript as Well?
-
Layers of Protection: Even if bots were to scrape your servlet's HTML output, they would find it difficult to decipher the email since it’s generated dynamically.
-
User Friendliness: This method maintains ease of use for human visitors, while providing numerous barriers to malicious bots.
Consider Alternative Methods
It's also prudent to consider additional anti-spam measures. For example, you could implement CAPTCHA to verify if a user is human before allowing them to access your contact information. This adds yet another layer of protection against unwanted spam emails.
Monitoring and Maintenance
Whenever you implement security features, it’s critical to monitor their effectiveness. Regularly check your email inbox to assess if spam levels are diminishing. Stay informed about new methods spammers deploy and stay ahead by periodically adjusting your strategies.
Key Takeaways
Protecting your Java app's mailto links from spam attackers is vital in ensuring seamless user interactions and maintaining your domain reputation. Implementing techniques such as server-side email generation and client-side obfuscation can dramatically reduce your spam vulnerability.
For tips on how to further protect your web app from spammers, check out our related article on Shield Your Mailto Links from Spammers with JavaScript.
By employing these strategies, not only do you enhance your application's security, but you also offer your users a smooth and harassment-free communication channel. So, take action today, and keep your inbox free from spam!
Checkout our other articles