Common Spring Boot Misconfigurations and How to Fix Them
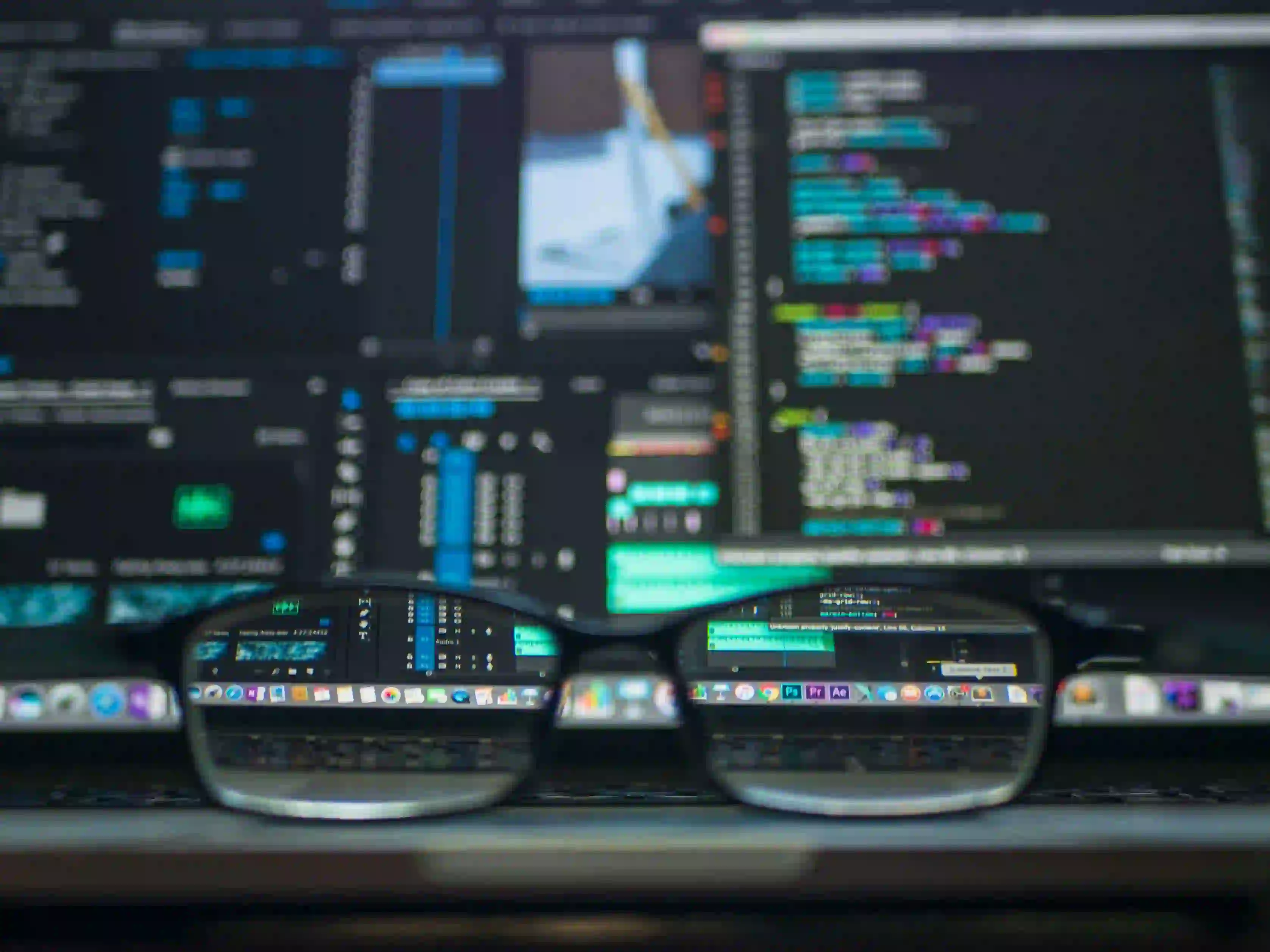
Common Spring Boot Misconfigurations and How to Fix Them
Spring Boot has rapidly become one of the most popular frameworks for building Java applications, thanks to its ease of use and ability to simplify the configuration process. However, even though it streamlines many aspects of application development, there are still common misconfigurations that developers encounter. In this post, we'll explore these misconfigurations and provide solutions to help you avoid the pitfalls.
1. Incorrect Data Source Configuration
Problem: A common mistake for newcomers is misconfiguring the data source settings in application.properties
or application.yml
. This can lead to connection failures or runtime exceptions.
Solution: Double-check your database connection properties. Here is a typical configuration for a MySQL database in application.properties
:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=myuser
spring.datasource.password=mypassword
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
Explanation
- Spring DataSource: The
spring.datasource.url
specifies the database's location. - Credentials: Ensure that your username and password are correct.
- Driver Class: Always include the driver class name to avoid issues during startup.
For additional security, consider storing sensitive configuration properties in a separate file or using Spring Cloud Config to manage these values centrally.
2. Not Using Profiles Effectively
Problem: Many developers neglect Spring profiles, which can lead to issues when deploying applications across different environments.
Solution: Utilize profiles to manage environment-specific configurations. Create different property files like application-dev.properties
for development and application-prod.properties
for production.
Example:
# application-prod.properties
spring.datasource.url=jdbc:mysql://prod-db-server:3306/proddb
spring.datasource.username=produser
spring.datasource.password=prodpassword
Explanation
Profiles allow you to maintain environment-specific configurations effortlessly. Make sure to activate the profile needed by specifying it at runtime:
java -jar myapp.jar --spring.profiles.active=prod
3. Mismanaged Dependencies
Problem: Additionally, having a bloated pom.xml
or build.gradle
can lead to performance issues and version conflicts.
Solution: Carefully manage your dependencies and ensure that you only include those necessary for your application. For instance, if you are not using Spring Web, avoid adding spring-boot-starter-web
.
Example for Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
Explanation
Use only the starter dependencies that are pertinent to your project. This will not only reduce your application size but also minimize security vulnerabilities associated with unused libraries.
4. Ignoring Proper Logging
Problem: Improper logging configurations can lead to incomplete logs or an overwhelming amount of log data.
Solution: Configure logging strategies properly in your application.properties
file. Use appropriate logging levels for different environments.
Example:
logging.level.root=WARN
logging.level.org.springframework.web=DEBUG
Explanation
By adjusting the log levels, developers can avoid cluttering logs with unnecessary data during production and gather more detailed logs during development. You can also integrate ELK Stack for advanced logging capabilities.
5. Poor Exception Handling
Problem: Clients are often subjected to unhelpful error messages due to inadequate exception handling.
Solution: Implement a global exception handler using @ControllerAdvice
. This allows you to manage exceptions uniformly across the application.
Example:
@RestControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFoundException(ResourceNotFoundException ex) {
ErrorResponse errorResponse = new ErrorResponse("Resource not found", ex.getMessage());
return new ResponseEntity<>(errorResponse, HttpStatus.NOT_FOUND);
}
}
Explanation
The @ExceptionHandler
annotation allows you to capture specific exceptions and return a standardized response. This way, your API users will receive informative error messages instead of raw stack traces.
6. Not Enabling CORS
Problem: Frontend applications often face issues when trying to communicate with a Spring Boot backend because of Cross-Origin Resource Sharing (CORS).
Solution: Enable CORS in your Spring Boot application. You can do this by adding a configuration class.
Example:
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("http://localhost:3000");
}
}
Explanation
The addCorsMappings
method allows you to specify origins that are permitted to communicate with your backend, enhancing security while maintaining flexibility.
7. Failing to Use Spring Boot Actuator
Problem: Developers sometimes overlook Spring Boot Actuator, which provides invaluable insights into application performance and health.
Solution: Include Actuator in your dependencies and enable necessary endpoints for monitoring.
Example for Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Explanation
Actuator exposes various metrics and health checks that can help monitor your application. After you add this to your project, ensure you secure it properly by configuring access rules.
management.endpoints.web.exposure.include=health,info
Closing Remarks
Spring Boot is powerful, but it requires careful configuration and attention to detail to reap its full benefits. By avoiding these common misconfigurations, you can enhance your application's performance, maintainability, and security.
For further reading, consider exploring the official Spring Boot documentation or other community resources like Spring Guides.
If you've encountered any other issues in Spring Boot, or have any tips and tricks to share, please leave a comment below!