Reducing GC Pressure: Why Primitives Matter in Java
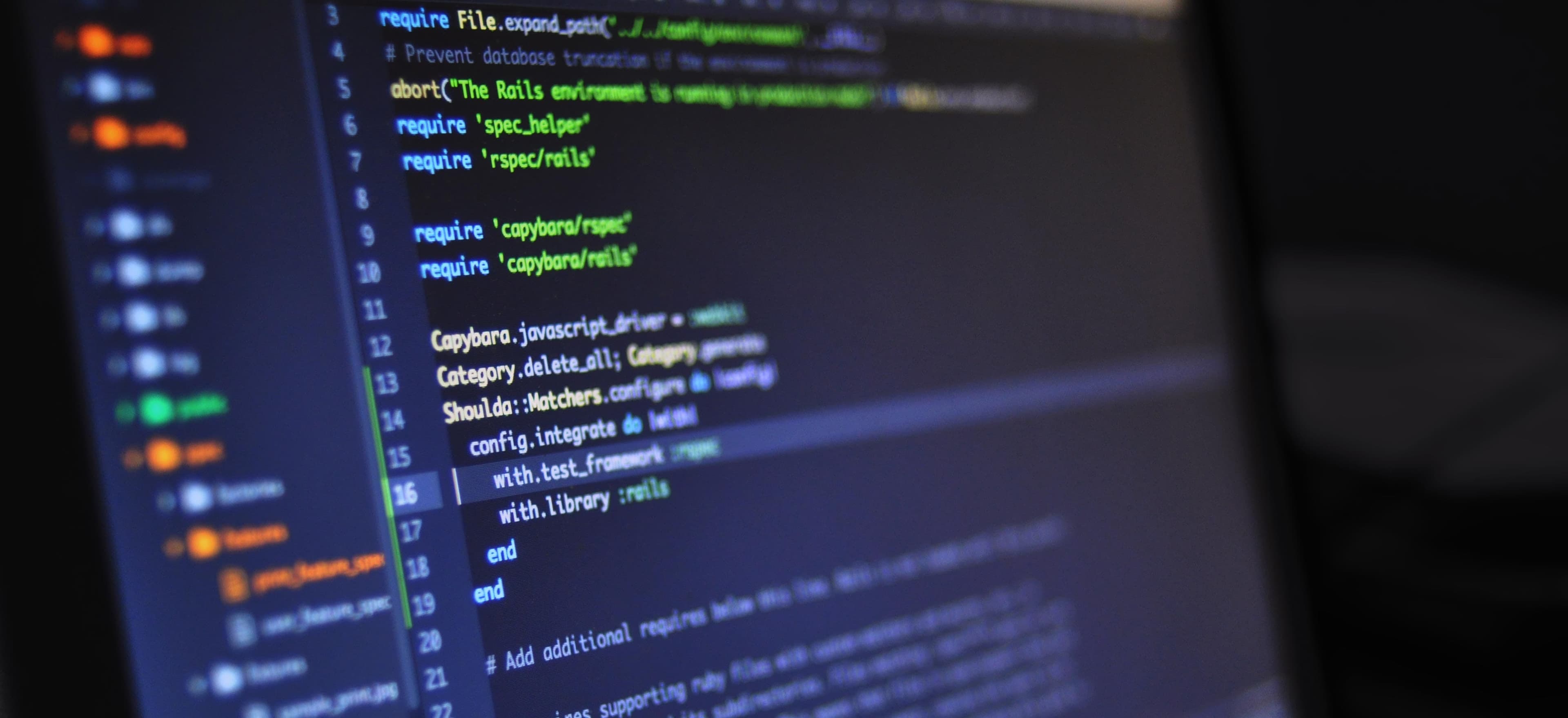
- Published on
Reducing GC Pressure: Why Primitives Matter in Java
Java developers often face the challenge of optimizing performance, especially in long-running applications. One important area to consider is garbage collection (GC) pressure. Understanding GC pressure can significantly impact performance by reducing pauses and improving throughput. In this post, we will delve into why primitives matter and how they can help reduce GC pressure in Java applications.
What is GC Pressure?
Garbage Collection in Java is a process that automatically manages memory, freeing up space by removing objects that are no longer in use. Although GC simplifies memory management, it comes with its challenges. GC pressure refers to the frequency and intensity of garbage collection events that the JVM (Java Virtual Machine) must handle. High GC pressure can lead to frequent stop-the-world pauses, resulting in poor application performance.
Understanding Primitives vs. Objects
In Java, data types are categorized into two groups: primitives and objects. Primitives (e.g., int, char, double) represent simple values and are stored directly in memory. Objects, in contrast, are instances of classes, which can hold both data and methods.
Why Choose Primitives?
-
Memory Efficiency: Primitives consume less memory compared to objects. For example, an
int
occupies 4 bytes, while anInteger
object, which wraps anint
, takes at least 16 bytes due to additional object overhead. -
Faster Access: Accessing primitive types is generally faster. Since they are stored directly in the memory stack, fetching a primitive value is quicker than dereferencing an object which may involve multiple memory lookups.
-
Reduced GC Load: Using primitives reduces the number of objects that the garbage collector has to manage. This decreases the frequency of garbage collection cycles and mitigation of pauses.
Examples Solving GC Pressure with Primitives
Example 1: Array of Primitives
When creating arrays, prefer primitives over their wrapper types. Let’s demonstrate this with a simple example:
// Using an array of primitives
int[] primitiveArray = new int[1000000]; // 4 bytes each (Total: 4MB)
// Using an array of their wrapper objects
Integer[] objectArray = new Integer[1000000]; // 16 bytes each (Total: 16MB)
In the example above, the memory footprint of the primitive array is significantly smaller than that of the Integer
object array. When GC attempts to reclaim memory, it will find fewer allocations with the primitive array.
Example 2: Collection Optimization
Consider a case where you need to store a list of numbers:
// Using the wrapper class
List<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
// Using primitive encapsulation with arrays
int[] primitiveNumbers = new int[3];
primitiveNumbers[0] = 1;
primitiveNumbers[1] = 2;
primitiveNumbers[2] = 3;
Here, replacing the Integer
objects with an array of int
not only saves memory but also streamlines processing since fewer objects are created. If your application frequently manipulates these numbers, the performance benefit becomes more pronounced.
When to Use Objects
It is essential to recognize when you might need to use objects instead of primitives. Here are a few considerations:
- Nullability: Wrapper classes can be null, whereas primitives cannot. If you need to represent "no value," consider using wrapper types.
- Java Collections Framework: Many of the collections work with objects, thus necessitating the use of wrapper classes. But often, you can create more memory-efficient custom structures for primitive data.
For more insights into Java collections, you can read Oracle's Java Collections Framework.
Performance Tips for Reducing GC Pressure
To further reduce GC pressure in your Java applications, consider implementing these performance tips:
-
Use Primitive Types Where Possible: Embrace primitives whenever feasible. As seen, the performance benefits are considerable—especially in high-volume applications.
-
Avoid Autoboxing: Autoboxing occurs when Java automatically converts primitives to their corresponding wrapper classes. This hidden conversion can lead to unnecessary object creation and, consequently, higher GC pressure.
-
Limit Object Creation: The more objects you create, the more GC has to manage. Look for opportunities to reuse objects or use object pools where appropriate.
-
Monitor and Tune GC Settings: Utilize GC tools to monitor garbage collection processes, allowing you to fine-tune JVM parameters. Classes such as
G1
orZGC
offer different tuning approaches that may suit your application’s needs. -
Use Efficient Data Structures: Select data structures based on access patterns and characteristics. For instance,
ArrayList
is more memory-efficient thanLinkedList
when managing primitive types since it uses a single array and avoids node overhead.
Closing the Chapter
Reducing GC pressure in Java applications is crucial for achieving optimal performance. By understanding the advantages of using primitives over objects, developers can create more efficient memory management strategies.
The choice between primitives and objects is critical. Primitives are lightweight, faster, and reduce the memory overhead, leading to lower GC pressure. As we continue to build complex applications, leveraging primitives where appropriate and understanding their impacts on garbage collection will contribute to more responsive and efficient systems.
For developers looking to dive deeper into Java performance optimization, I recommend checking out Effective Java by Joshua Bloch. This book provides invaluable insights and best practices for Java programming.
Start by evaluating your applications and identify where you can reduce GC pressure by embracing primitives. Optimize for performance, and your users will appreciate the smoother experience!
Checkout our other articles