Is Lazy Loading Hurting Your Application's Performance?
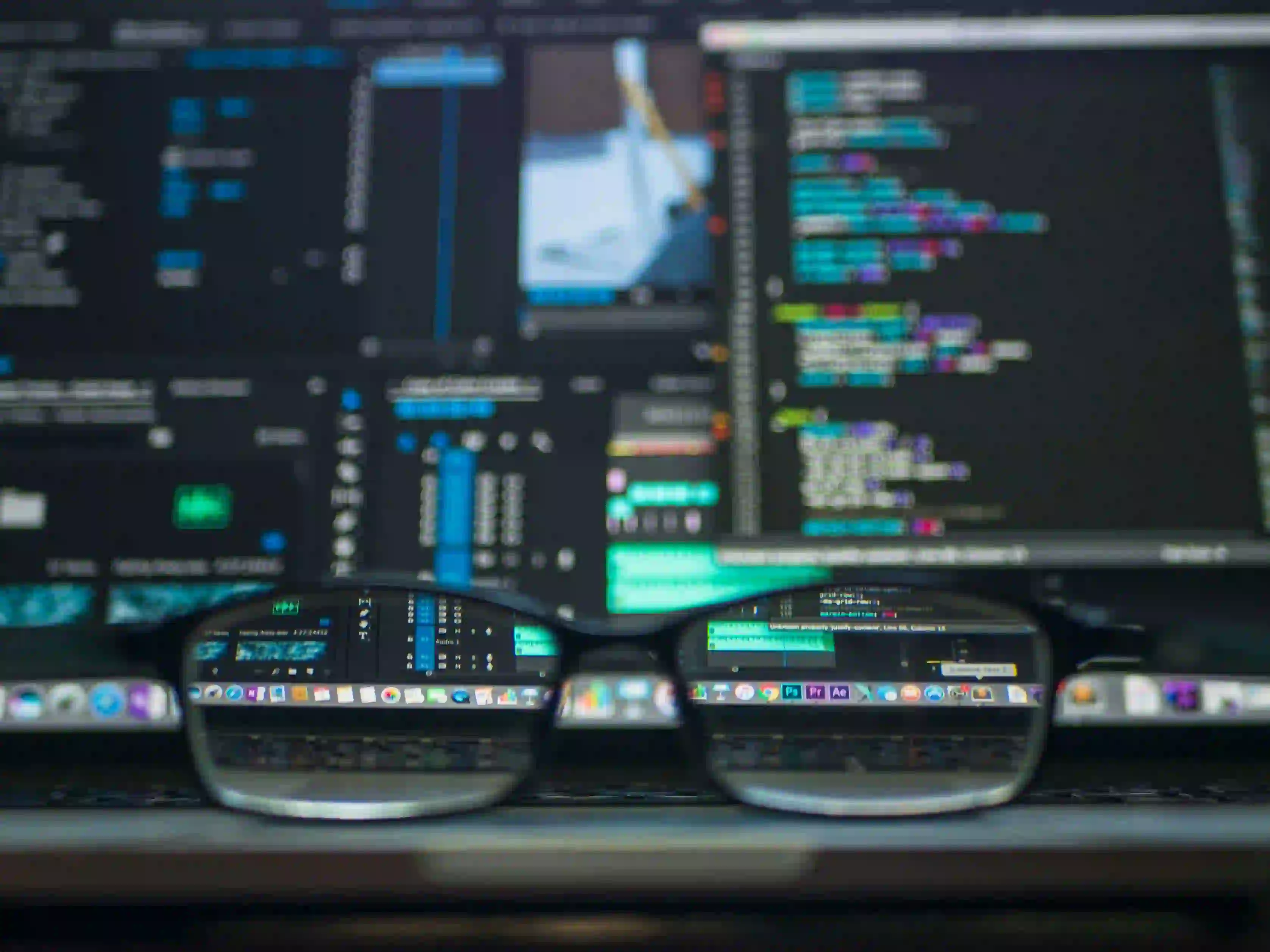
Is Lazy Loading Hurting Your Application's Performance?
Lazy loading is a design pattern commonly used in programming. The idea is to defer the initialization of an object until its value is actually needed. In the world of Java, lazy loading helps improve the perceived performance of applications, especially those that manage large data sets or require significant resource allocation.
However, despite its advantages, lazy loading can sometimes hurt your application’s performance if not implemented properly. This blog post will explore lazy loading, its benefits, and potential pitfalls. Along the way, we’ll provide clear, actionable coding examples and explanations.
What is Lazy Loading?
Lazy loading can be understood as a technique used to delay the loading of an object or resource until it is needed. In Java, lazy loading is often applied in contexts such as data retrieval, UI components, and even model associations in frameworks like Hibernate.
Benefits of Lazy Loading:
- Reduced Initial Load Time: Loading data only when necessary can significantly reduce the time it takes for your application to start and become interactable.
- Reduced Memory Footprint: Since objects are created only when needed, this can lead to lower memory usage.
- Improved User Experience: By providing faster initial feedback to the user, applications appear more responsive and engaging.
Despite these advantages, lazy loading can also introduce some challenges that you should be aware of.
When Lazy Loading Can Hurt Performance
-
Repeated Lazy Loads: Continuously accessing a lazily-loaded field can lead to performance degradation. If the data needs to be loaded multiple times, the overhead can be higher than loading it all at once.
-
Increased Complexity: Code that uses lazy loading can become complex and harder to maintain. Debugging can be challenging if loading logic is scattered across many classes.
-
Concurrency Issues: In a multi-threaded environment, lazy loading can lead to potential thread safety issues if not handled correctly.
Lazy Loading Example
Let’s illustrate lazy loading with a practical example in Java. Suppose we have a class representing a user account that stores user data. Instead of loading all user attributes upfront, we can lazily load attributes as needed.
public class UserAccount {
private String userId;
private LazyLoadedData lazyLoadedData;
public UserAccount(String userId) {
this.userId = userId;
}
public LazyLoadedData getLazyLoadedData() {
if (lazyLoadedData == null) {
// Load data only when accessed
lazyLoadedData = loadDataFromDatabase(userId);
}
return lazyLoadedData;
}
private LazyLoadedData loadDataFromDatabase(String userId) {
// Simulate database logic
System.out.println("Loading data for user: " + userId);
return new LazyLoadedData(userId);
}
}
Why This Works
In this example, we delay loading LazyLoadedData
until getLazyLoadedData()
is invoked. If the data is never needed, it will not be retrieved, so memory and processing resources are conserved.
Potential Drawbacks
Now, let's consider the implications if this method is called multiple times in quick succession.
public class Main {
public static void main(String[] args) {
UserAccount account = new UserAccount("user123");
// Multiple accesses to the lazy-loaded data
account.getLazyLoadedData();
account.getLazyLoadedData();
account.getLazyLoadedData();
}
}
You would see "Loading data for user: user123" printed to the console only once. While beautiful in its efficiency, consider how it scales. If loadDataFromDatabase
is a resource-intensive call, repeated accesses could still become a bottleneck.
Optimizing Lazy Loading
To address the potential pitfalls of lazy loading, consider these strategies:
-
Cache Results: As shown in the example above, once data is loaded, store it to prevent re-access until necessary.
-
Batch Loading: Instead of loading data object-by-object or on each access, consider loading in batches whenever possible.
-
Eager Loading Where Appropriate: Use lazy loading primarily when necessary. For some critical data, load it upfront. This requires a balance of when to load eagerly vs. lazily.
Example of Eager vs. Lazy Loading
Let's compare eager loading with lazy loading using a database example.
// Eager loading example
public class EagerUser {
private String userId;
private List<Post> posts; // Load all posts immediately
public EagerUser(String userId) {
this.userId = userId;
posts = loadPosts(userId); // Eagerly fetching posts
}
}
// Lazy loading example from above
public class LazyUser {
private String userId;
private Lazy<List<Post>> posts; // Load posts on first access
public LazyUser(String userId) {
this.userId = userId;
this.posts = Lazy.of(() -> loadPosts(userId));
}
}
The Choice Between Eager and Lazy Loading
Using eager loading ensures that all necessary data is available immediately, avoiding potential delays in data access later. However, if the user may not need all data immediately, lazy loading is more efficient.
Deciding which to use hinges on application requirements, user behavior, network conditions, and data size and access patterns.
Lessons Learned
Lazy loading is a powerful tool in a Java developer's arsenal, particularly when managing resource-intensive processes. However, care must be taken in its implementation to avoid pitfalls that hinder application performance.
Always evaluate when to embrace lazy loading versus eager loading, based on your specific use cases. The balance between performance, maintainability, and user experience is key to creating successful applications.
For further reading on lazy loading in various contexts, you can explore articles on Hibernate Lazy Loading, which dives deeply into Lazy Loading for databases, or Spring Data JPA, which discusses its use in Spring applications.
By keeping lazy loading's benefits and drawbacks top of mind, developers can make informed choices that ultimately serve the application's strategic objectives. Happy coding!