Common Pitfalls in Basic Android Game Development
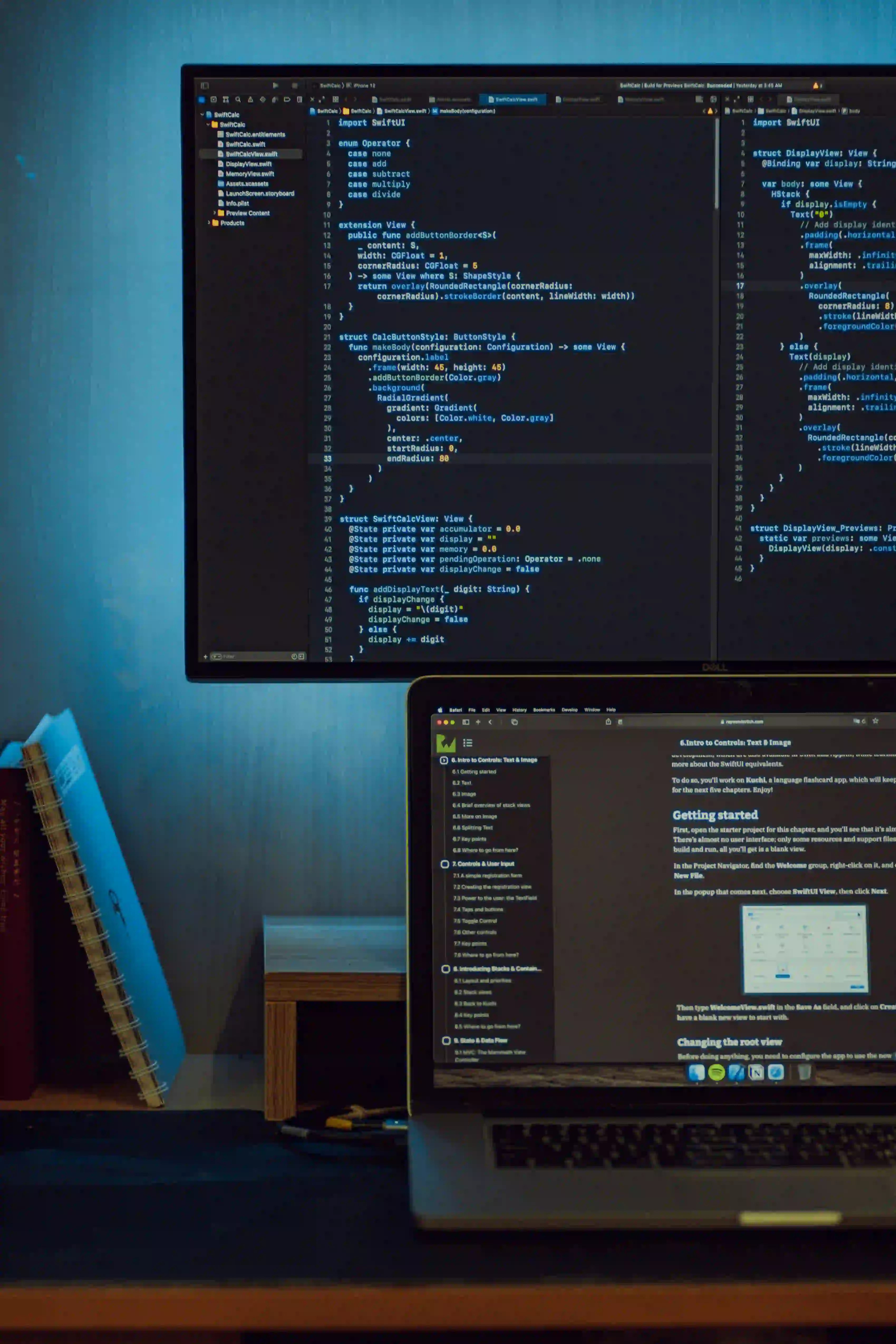
Common Pitfalls in Basic Android Game Development
Android game development is an exciting venture that blends creativity with coding skills. However, amidst the thrill of creating immersive experiences, developers—especially beginners—often stumble upon common pitfalls that can impact their projects. In this blog post, we’ll explore these pitfalls, providing insights on how to avoid them. Let’s dive in!
1. Neglecting Game Design Principles
One of the first steps in game development is to establish solid game design principles. Ignoring these can lead to a poor user experience.
Why It Matters
Game design principles encompass elements like balance, challenge, context, and flow. Failing to consider them can result in games that are either too easy or overly complex.
Avoiding the Pitfall
- Prototype Early: Create simple prototypes to test your concepts before investing significant time in development. Tools like Unity and Godot offer prototyping features that facilitate this process.
Example Code Snippet
// A simple prototype setup for a game character in Android
public class Character {
private int health;
private int attackPoints;
public Character(int health, int attackPoints) {
this.health = health;
this.attackPoints = attackPoints;
}
// Method to attack another character
public void attack(Character opponent) {
opponent.takeDamage(attackPoints);
}
public void takeDamage(int damage) {
health -= damage;
if (health <= 0) {
// Character is defeated
System.out.println("Character defeated!");
}
}
}
The code snippet exemplifies a simple character system. Using clear methods for attack and damage helps ensure the gameplay is straightforward and easy to understand.
2. Overcomplicating the Code
Beginners often fall into the trap of writing complex code with unnecessary features. While it can be tempting to showcase programming skills, clarity and simplicity should be prioritized.
Why It Matters
Clear and maintainable code improves collaboration, debugging, and future updates, essential for ongoing game development.
Avoiding the Pitfall
- Keep It Simple: Follow the KISS (Keep It Simple, Stupid) principle. Break down tasks into smaller, manageable pieces, focusing on writing clean, readable code.
Example Code Snippet
// Example of a simple game loop
public void gameLoop() {
while (isRunning) {
updateGameState();
renderGraphics();
checkInput();
}
}
private void updateGameState() {
// Logic to update game entities
}
private void renderGraphics() {
// Logic to draw the game frame
}
private void checkInput() {
// Logic to capture player input
}
This snippet illustrates a classic game loop. The methods compartmentalize functionality, making the code easier to maintain and understand.
3. Ignoring Performance Optimization
Performance is one of the cornerstones of a successful Android game. New developers may overlook optimization, leading to laggy gameplay experiences.
Why It Matters
Performance issues can frustrate players and lead to negative reviews. An optimized game runs smoothly, enhancing player engagement.
Avoiding the Pitfall
- Profile Your Game: Use tools like Android Profiler, included in Android Studio, to pinpoint performance bottlenecks and optimize accordingly.
Example Code Snippet
// Lazy loading of resources
public class ResourceLoader {
private HashMap<String, Bitmap> images = new HashMap<>();
public Bitmap loadImage(String filePath) {
if (!images.containsKey(filePath)) {
images.put(filePath, BitmapFactory.decodeFile(filePath));
}
return images.get(filePath);
}
}
This example demonstrates lazy loading, only loading resources when needed. By caching already loaded images, it reduces memory consumption and increases performance.
4. Failing to Test Across Devices
The diversity of Android devices can create unique challenges. Relying solely on an emulator may overlook issues specific to certain hardware or display configurations.
Why It Matters
Testing on multiple devices ensures a wider range of users can enjoy a consistent experience.
Avoiding the Pitfall
- Use Device Labs or Emulators: Leverage tools like Firebase Test Lab for comprehensive testing across various devices and screen sizes.
5. Lack of Version Control
New developers often skip version control practices. This oversight can lead to loss of code, particularly when making changes or debugging.
Why It Matters
Version control systems (VCS) like Git enable developers to track changes, collaborate with others, and roll back to previous code states when needed.
Avoiding the Pitfall
- Set Up Git Early: Initiate a Git repository from the start of your project, and commit your changes regularly. Tools like GitHub or GitLab can facilitate collaboration and provide backup.
Example Command Usage
# Initialize a new Git repository
git init
# Add all files to the repository
git add .
# Commit changes with a message
git commit -m "Initial commit: Set up game skeleton"
6. Inadequate Monetization Planning
While many developers create games for fun, considering monetization strategies early in development is crucial when aiming for profitability.
Why It Matters
Monetization strategies dictate how you will sustain your game. Ignoring this can lead to missed opportunities for revenue.
Avoiding the Pitfall
- Research Monetization Options: Familiarize yourself with various monetization models—such as freemium, ads, and in-app purchases. Choose one that aligns with your game’s design and target audience.
For further insight into monetization strategies, check out AdMob by Google, which provides various ad monetization options for mobile applications.
To Wrap Things Up
Navigating Android game development can be challenging, but recognizing these common pitfalls will empower developers to create engaging and high-performing games. Emphasizing solid game design, writing clear code, optimizing performance, thoroughly testing across devices, utilizing version control, and planning for monetization will set the stage for success.
Developing a game is a journey filled with learning opportunities. So, don't be disheartened by challenges—embrace them, and continually strive to learn and improve. Happy coding!
This post provides practical tips to help beginner Android developers navigate potential pitfalls. For further reading on Android development, refer to the official Android Developer Guide.