Navigating Common Pitfalls in Software Development Mastery
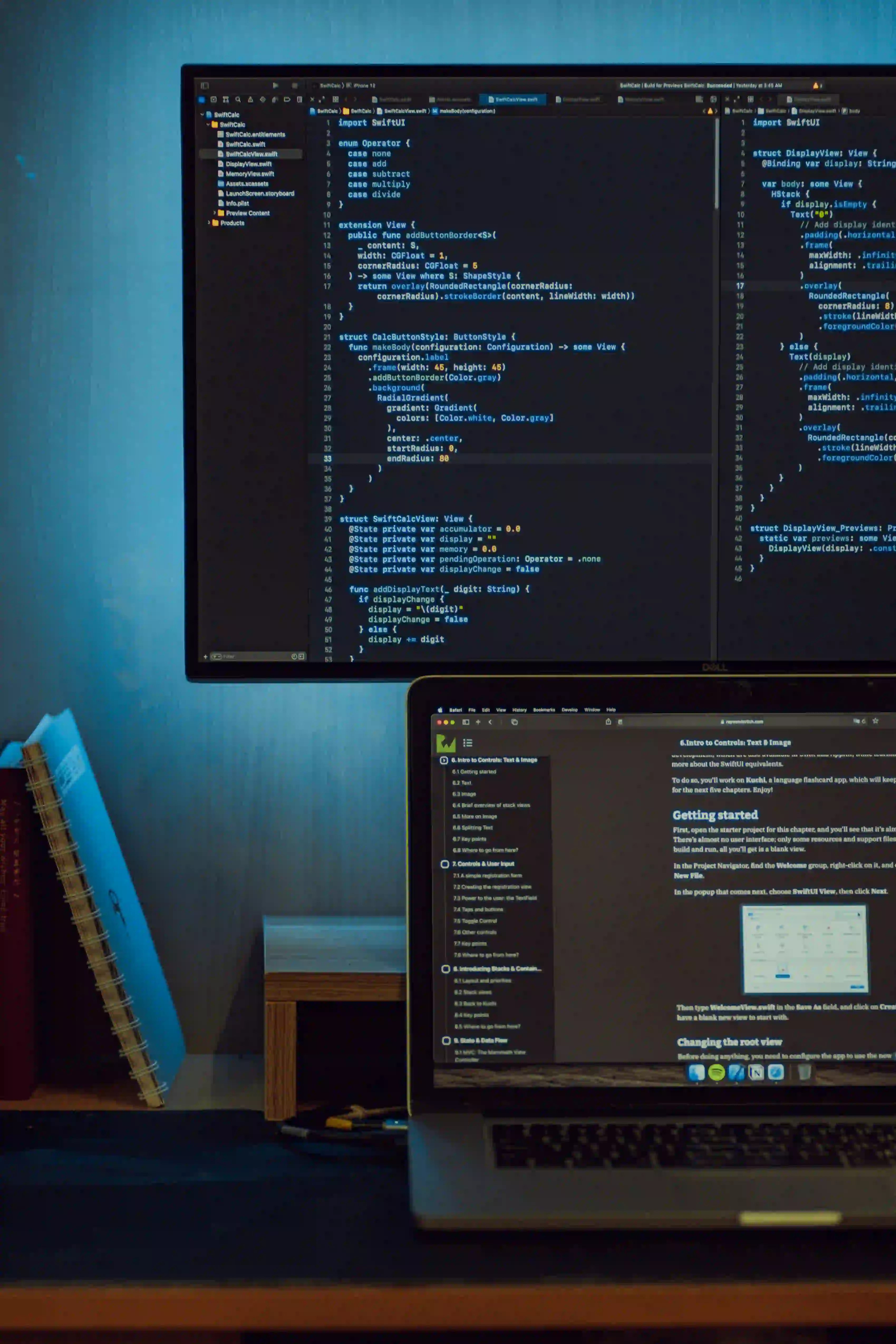
Navigating Common Pitfalls in Software Development Mastery
Software development is an ever-evolving field that demands not just technical skills but also critical thinking, creativity, and adaptability. As developers, we often face various challenges that can hinder progress. Mastering these pitfalls is essential for both novice and experienced developers. In this blog post, we'll explore common obstacles in software development, with a specific focus on Java programming. We will provide code snippets, explanations, and valuable resources to help you navigate these challenges effectively.
1. Lack of Understanding Requirements
One of the most significant pitfalls in software development is inadequate comprehension of project requirements. This can lead to a series of misunderstandings and erroneous implementations.
The Importance of Requirements Analysis
A well-defined set of requirements is the foundation of successful software projects. It dictates the scope, functionality, and user experience. Without clear requirements:
- Developers may create features that are unnecessary.
- Time and resources may be misallocated.
- Project deadlines can be easily missed.
Example:
Imagine you are tasked with developing a simple calculator application in Java, but the requirements state that the application must also support complex numbers.
public class Calculator {
public static ComplexNumber add(ComplexNumber a, ComplexNumber b) {
return new ComplexNumber(a.real + b.real, a.imaginary + b.imaginary);
}
// Additional implementation...
}
Why This Matters: Without a discussion with stakeholders regarding the need for complex number support, developers may miss critical functionality, leading to a product that does not meet user needs.
Resources for Further Reading:
- Understanding User Requirements
- Effective Communication in Software Development
2. Not Utilizing Version Control
Version control systems, such as Git, are indispensable in modern software development. Failing to utilize them can lead to disorganization and loss of code integrity.
The Power of Version Control
Version control provides several benefits:
- Ability to track changes and revert when necessary.
- Enables teamwork through branching and merging.
- Simplifies collaboration with distributed teams.
Example:
When using Git, it is crucial to commit changes regularly with descriptive messages.
git add .
git commit -m "Added method for adding complex numbers"
Why This Matters: Regular commits allow for better tracking of code evolution. If a bug appears, developers can review history and pinpoint where things went wrong.
Resources for Further Reading:
3. Ignoring Code Quality
Code quality is often an afterthought, yet it directly impacts maintainability, readability, and efficiency.
Why Code Quality Matters
High-quality code enhances:
- Collaboration among team members.
- Ease of debugging and testing.
- Future feature implementations.
Example:
In Java, using proper naming conventions can immensely improve code clarity.
public class Rectangle {
private double length;
private double width;
public double calculateArea() {
return length * width;
}
}
Why This Matters: Descriptive class and method names inform users about their purpose. When revisiting the code months later, developers can quickly grasp its functionality without deciphering unclear names.
Resources for Further Reading:
4. Failing to Write Tests
Writing tests is a vital aspect of ensuring code reliability and quality. Many developers skip this step due to time constraints or a lack of understanding.
The Significance of Testing
Tests help catch bugs before deployment and ensure that new features do not break existing functionality.
Example:
Using JUnit for unit testing in Java can illustrate the importance of automated tests.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class RectangleTest {
@Test
public void testCalculateArea() {
Rectangle rect = new Rectangle(5, 3);
assertEquals(15, rect.calculateArea());
}
}
Why This Matters: This test verifies that the calculateArea
method is functioning correctly. If the method implementation changes later, running this test will confirm whether the change impacted the functionality.
Resources for Further Reading:
5. Mismanagement of Dependencies
In Java, managing external libraries or dependencies can become cumbersome, especially in large projects. Ignoring dependency management can lead to conflicts, versioning issues, and bloated applications.
Why Dependency Management is Crucial
Proper dependency management ensures:
- Consistent builds.
- Avoidance of version conflicts.
- Easier upgrades and maintenance.
Example:
Using a build tool like Maven can simplify dependency management significantly.
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
Why This Matters: Declaring dependencies in pom.xml
enables Maven to manage them for you, including transitive dependencies. This minimizes potential issues that foreign libraries can introduce.
Resources for Further Reading:
- Maven Documentation
- Understanding Dependency Management
A Final Look
Mastering software development means recognizing and addressing common pitfalls along the journey. From understanding requirements and managing dependencies to ensuring high code quality and implementing robust tests, each step contributes to the overall success of a project.
Final Thoughts
Remember, the key to effective software development is not just to write code but to write intelligent, maintainable code that aligns with user needs. By avoiding these pitfalls, we pave the way for fulfilling software development experiences. Continue to learn, adapt and share with others as we all grow in our respective skills.
For continuous learning, consider engaging with fellow developers through communities such as Stack Overflow or joining forums related to Java programming.
Embrace these insights, and you will navigate the rocky waters of software development with confidence and expertise!