Overcoming OpenHub Framework Integration Challenges
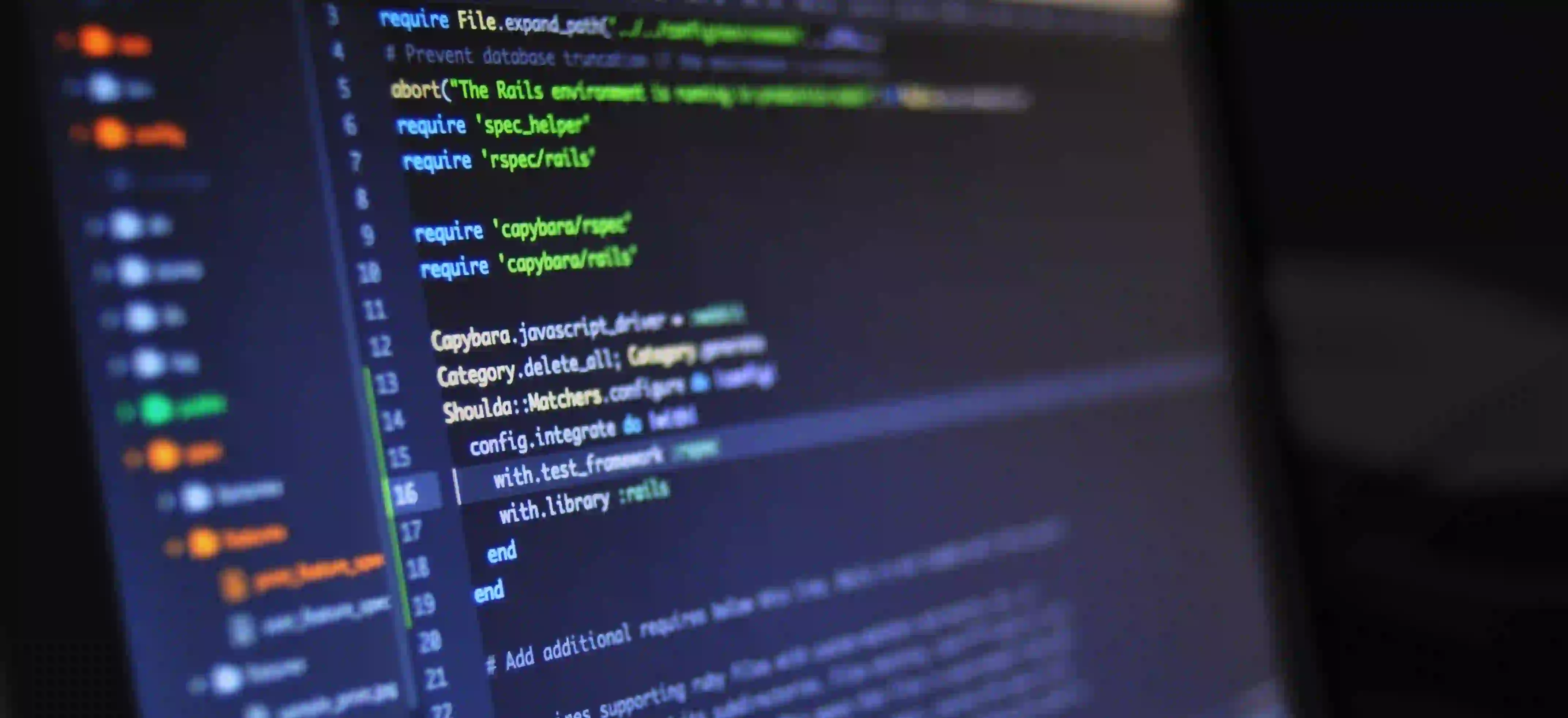
Overcoming OpenHub Framework Integration Challenges
In the ever-evolving world of software development, integration frameworks stand as critical components that streamline workflow, enhance productivity, and foster collaboration. One such framework is OpenHub. It offers a comprehensive approach to manage large amounts of APIs and services efficiently. However, like any powerful tool, integrating OpenHub into your existing systems can present various challenges. In this blog post, we will explore common pitfalls in OpenHub integration and provide practical solutions to overcome them.
Understanding OpenHub Framework
Before diving into the challenges, it's essential to understand the fundamentals of OpenHub. OpenHub is an architecture designed to facilitate the integration of disparate systems through a unified interface. By offering a flexible way to connect, transform, and manage data, OpenHub allows developers to create robust applications that leverage multiple services seamlessly.
Key Features
- API Management: Centralized control over APIs.
- Data Transformation: Built-in capabilities to modify data structures.
- Scalability: Handles increasing loads with ease.
- Monitoring and Analytics: Track performance and usage metrics.
These features empower organizations to streamline their processes. However, the transition to OpenHub often comes with hurdles.
Common Integration Challenges
1. Legacy System Compatibility
One of the primary challenges developers face is integrating OpenHub with legacy systems. Many organizations run crucial business operations on outdated technology stacks that may not support modern protocols.
Solution: Gradual Migration
Consider a phased approach. Instead of complete overhauls, target specific functionalities for migration. This minimizes disruption while progressively transitioning to OpenHub.
Sample Code: Adapting Legacy Interfaces
public class LegacyAdapter {
private LegacyService legacyService;
public LegacyAdapter(LegacyService legacyService) {
this.legacyService = legacyService;
}
public DataModel fetchData() {
// Adapting legacy data to a modern format
LegacyData legacyData = legacyService.getData();
return transformToDataModel(legacyData);
}
private DataModel transformToDataModel(LegacyData legacyData) {
// Example transformation logic
return new DataModel(legacyData.getId(), legacyData.getName());
}
}
Why? This adapter pattern allows the legacy service to communicate within new frameworks without modifying the original system’s code.
2. Data Format Incompatibility
Different systems often operate on varying data formats, leading to inconsistencies and misinterpretations.
Solution: Standardized Data Formats
Implement a common data interchange format such as JSON or XML across your applications. OpenHub’s transformation capabilities can facilitate this process, ensuring all components speak the same language.
Sample Code: JSON Transformation
import com.fasterxml.jackson.databind.ObjectMapper;
public class DataTransformer {
private ObjectMapper objectMapper = new ObjectMapper();
public String toJson(DataModel dataModel) throws Exception {
// Serializing DataModel to JSON format
return objectMapper.writeValueAsString(dataModel);
}
public DataModel fromJson(String json) throws Exception {
// Deserializing JSON string back to DataModel
return objectMapper.readValue(json, DataModel.class);
}
}
Why? Using libraries such as Jackson simplifies JSON handling, making it easier to connect systems with different data formats.
3. Performance Bottlenecks
As you integrate with OpenHub, you may experience performance degradation, especially if the existing systems were not optimized for such changes.
Solution: Load Testing and Optimization
Conduct load testing to assess performance under stress conditions. Identify bottlenecks in the integration flow and apply optimizations such as caching or request batching.
Sample Code: Basic Caching Implementation
import java.util.HashMap;
import java.util.Map;
public class Cache {
private Map<String, DataModel> cacheStore = new HashMap<>();
public DataModel getCachedData(String key) {
return cacheStore.get(key);
}
public void putData(String key, DataModel data) {
cacheStore.put(key, data);
}
}
Why? Caching frequently accessed data can significantly reduce the number of API calls, resulting in quicker response times and reduced load on your servers.
4. Security Concerns
When integrating new frameworks, securing the data during integration is paramount. OpenHub, being a gateway for data flow, may become a target for malicious attacks.
Solution: Implement Robust Authentication
Utilize OAuth or JWT for securing API calls. By ensuring that only authorized clients can access your services, you mitigate the risks.
Sample Code: Token-Based Authentication
public class AuthService {
public String generateToken(User user) {
// Simplified token generation logic
return Jwts.builder()
.setSubject(user.getUsername())
.signWith(SignatureAlgorithm.HS256, "secretKey")
.compact();
}
public void authenticate(String token) {
// Validate the token
Claims claims = Jwts.parser()
.setSigningKey("secretKey")
.parseClaimsJws(token)
.getBody();
// Example of further processing
}
}
Why? Token-based security ensures that each request is validated, reducing the likelihood of unauthorized access to sensitive data.
The Closing Argument
Integrating OpenHub into existing systems can indeed present multiple challenges. From legacy compatibility issues to performance bottlenecks and security concerns, developers can find themselves navigating a complex landscape. However, the strategies outlined above—gradual migration, standardized data formats, performance testing, and robust security measures—will equip developers with effective tools to tackle these challenges head-on.
In the realm of software development, knowledge is power. Ensure you stay updated with the latest advancements by checking resources like OpenHub’s official documentation and Java's API documentation.
By embracing these integration strategies, your team can unlock the full potential of the OpenHub framework, leading your organization toward greater efficiency and innovation. Happy coding!