Mastering Java Event Notifications: Common Pitfalls to Avoid
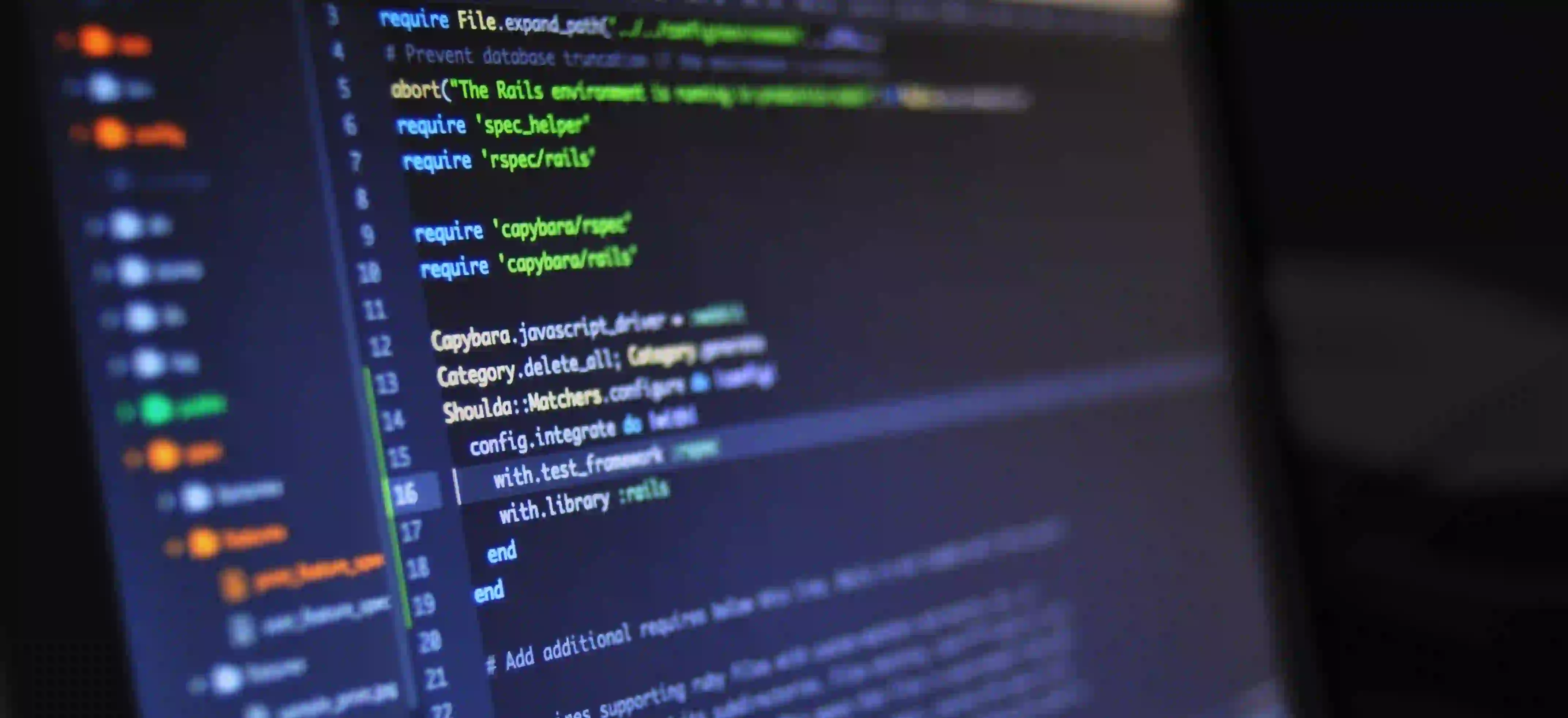
Mastering Java Event Notifications: Common Pitfalls to Avoid
Java is renowned for its robustness, scalability, and community-driven libraries. Among its many strengths is the observer pattern, an integral approach to building applications with event-driven designs. However, even seasoned developers can encounter pitfalls when implementing Java event notifications. This article will delve into the intricacies of Java event notifications, illuminate frequent mistakes, and guide you on your way to mastery.
Table of Contents
- Understanding Java Event Notifications
- Common Pitfalls in Event Management
- Best Practices for Handling Events
- Example of Java Event Notifications
- Conclusion
Understanding Java Event Notifications
At its core, Java event notifications allow objects to communicate with one another when changes occur. This is typically accomplished using interfaces and listener classes. Here's a breakdown of how this process works:
-
Event Source: This is the object that generates an event. For example, a button click or a change in a data model.
-
Event Listener: This interface defines the method(s) to be implemented for handling events.
-
Event Object: This object contains information about the event itself (like the source of the event and its type).
By decoupling event producers from consumers, developers can create flexible and maintainable applications.
Common Pitfalls in Event Management
Even though Java provides a solid framework for managing events, mistakes can hinder performance and lead to complex bugs. Here are some common pitfalls to avoid:
1. Neglecting to Remove Listeners
Forgetting to unregister event listeners can lead to memory leaks. If an event listener remains active after its source is no longer needed, the garbage collector cannot reclaim memory, resulting in increased memory usage.
Solution: Always clean up listeners once they are no longer needed.
// Example demonstrating unregistration of listeners
class ButtonEventSource {
private final List<ButtonClickListener> listeners = new ArrayList<>();
public void addListener(ButtonClickListener listener) {
listeners.add(listener);
}
public void removeListener(ButtonClickListener listener) {
listeners.remove(listener);
}
public void clickButton() {
for (ButtonClickListener listener : listeners) {
listener.onClick(); // Notify listeners
}
}
}
In this example, removeListener
ensures that we can unregister listeners, thus preventing memory leaks.
2. Overusing Event Objects
Passing too much information in an event object can lead to performance bottlenecks. Long-lived event objects consume more memory, and the overhead from processing them can slow applications down.
Solution: Keep your event object lightweight. Include only essential data.
class UserEvent {
private final String username;
public UserEvent(String username) {
this.username = username;
}
public String getUsername() {
return username;
}
}
Here, the UserEvent
class contains only what's necessary: the username. This facilitates quick handling.
3. Ignoring Thread Safety
In a multithreaded environment, event listeners can be fired asynchronously. If your event source and listeners aren’t thread-safe, you may face race conditions, which can lead to erratic application behavior.
Solution: Use synchronization mechanisms or concurrent data structures.
// Using synchronized for thread safety
public synchronized void clickButton() {
for (ButtonClickListener listener : listeners) {
listener.onClick();
}
}
The synchronized
keyword ensures that only one thread can execute the clickButton
method at a time, preserving consistent behavior.
4. Excessive Listener Calls
If not managed correctly, an event can trigger multiple listeners in succession. This can lead to performance issues, especially if listeners perform I/O operations or extensive computations.
Solution: Implement throttling for event notifications if possible.
5. Not Implementing Typed Listeners
Using generic listener types can lead to runtime errors. If a listener is designed for a specific event, ensure that it strictly handles that type.
Solution: Use generics to define listener capabilities clearly.
interface ButtonClickListener {
void onClick(UserEvent event);
}
By defining ButtonClickListener
with a specific event type, we ensure that each listener only interacts with the intended event data.
Best Practices for Handling Events
Now that we've discussed the common pitfalls, let's explore best practices to implement Java event notifications effectively:
1. Follow a Consistent Naming Convention
Ensure that all events follow a naming convention that makes their purpose clear. For instance, prefixing method names with "on" (like onButtonClick
) clarifies the listener’s role.
2. Use Weak References
To avoid memory leaks, consider using WeakReference
for listener registration. This allows the garbage collector to reclaim memory if the listener goes out of scope.
class ButtonEventSource {
private final List<WeakReference<ButtonClickListener>> listeners = new ArrayList<>();
public void addListener(ButtonClickListener listener) {
listeners.add(new WeakReference<>(listener));
}
}
Using WeakReference
ensures that if there are no strong references to the listener, it can be garbage collected.
3. Document Event Flow
Clear documentation on how your events flow through the application is crucial. This will help new developers understand the codebase and reduce the chances of misuse.
4. Keep Event Handling Lightweight
Event handling should be quick. Avoid lengthy operations within event handling methods. Instead, consider queuing tasks or processing them asynchronously.
5. Modularize Event Logic
If your application grows complex, consider separating event-related logic into different modules. This promotes separation of concerns and makes maintenance easier.
Example of Java Event Notifications
Now that we have insights into pitfalls and best practices let’s tie everything together with a complete example.
import java.util.ArrayList;
import java.util.List;
import java.lang.ref.WeakReference;
// Event class
class UserEvent {
private final String username;
public UserEvent(String username) {
this.username = username;
}
public String getUsername() {
return username;
}
}
// Listener interface
interface UserEventListener {
void onUserEvent(UserEvent event);
}
// Event source
class UserEventSource {
private final List<WeakReference<UserEventListener>> listeners = new ArrayList<>();
public void addListener(UserEventListener listener) {
listeners.add(new WeakReference<>(listener));
}
public void removeListener(UserEventListener listener) {
listeners.removeIf(ref -> ref.get() == listener);
}
public void notifyUserEvent(String username) {
UserEvent event = new UserEvent(username);
for (WeakReference<UserEventListener> ref : listeners) {
UserEventListener listener = ref.get();
if (listener != null) {
listener.onUserEvent(event);
}
}
}
}
// Example implementation of listener
class UserEventFeedback implements UserEventListener {
@Override
public void onUserEvent(UserEvent event) {
System.out.println("User event triggered: " + event.getUsername());
}
}
// Driver code
public class EventDemo {
public static void main(String[] args) {
UserEventSource userEventSource = new UserEventSource();
UserEventFeedback userEventFeedback = new UserEventFeedback();
userEventSource.addListener(userEventFeedback);
userEventSource.notifyUserEvent("Alice");
// Clean up listeners after use to avoid memory leaks
userEventSource.removeListener(userEventFeedback);
}
}
Commentary
In this example, UserEventSource
manages user events by notifying registered UserEventListener
s. The use of WeakReference
for the listener ensures that even if the listener is no longer needed, it won't hold a strong reference, avoiding memory leaks.
Key Takeaways
Mastering Java event notifications can significantly enhance your application's responsiveness and architecture. By being aware of common pitfalls like memory leaks or thread safety issues and adhering to best practices, you can create a robust event-driven application.
As you implement your event handling, keep the advice shared in this article in mind. Continuous learning and refining your approach will greatly enhance your development skills in Java.
For further reading, you can check the official Java documentation or explore the Observer pattern in more depth here.
Embrace the power of event notifications and watch your applications thrive!