Common Pitfalls When Using Enums in Spring Data JPA
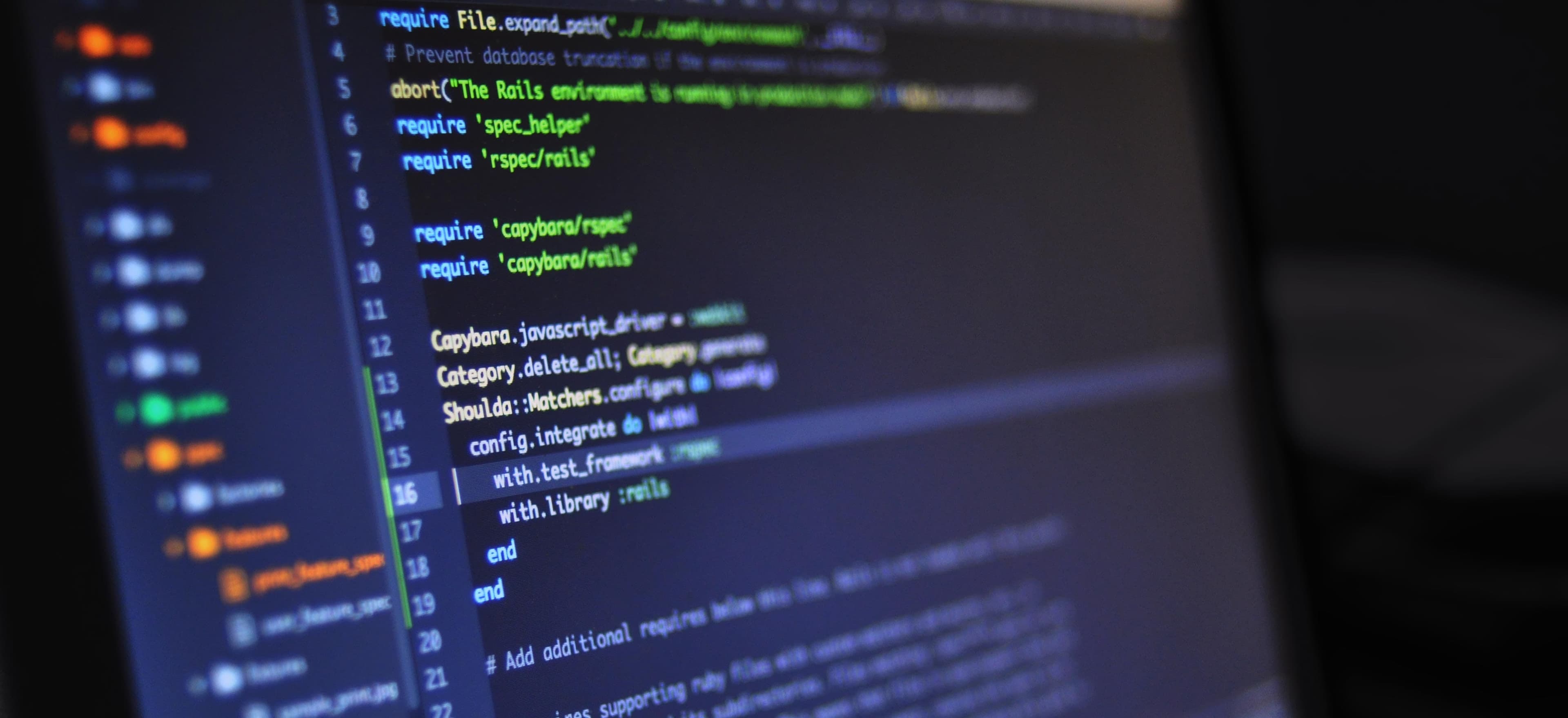
- Published on
Common Pitfalls When Using Enums in Spring Data JPA
Enums are a powerful feature in Java, allowing developers to define a finite set of constants. When it comes to Spring Data JPA, using enums can be beneficial—offering type safety and enhanced readability. However, there are several common pitfalls that developers may encounter when using enums in this context. In this blog post, we will explore these pitfalls and provide practical solutions for navigating them effectively.
What are Enums in Java?
Enums, or enumerations, are a special Java type used to define collections of constants. They help in creating an understandable set of named values. For example:
public enum OrderStatus {
PENDING,
SHIPPED,
DELIVERED,
CANCELLED
}
Why Use Enums in Spring Data JPA?
Enums provide a clearer representation of the state within your application or the database. Since database fields often represent a limited number of values, embedding enums correlates naturally and avoids using generic strings or integers. Enums lead to safer code and fewer human errors.
The Common Pitfalls
1. Storing Enum as a String vs. Ordinal
One of the primary pitfalls is determining whether to store your enum as a string or an ordinal. When defining in JPA, using @Enumerated
can dictate how your enum is persisted.
Using the ordinal for storage means your enum values are represented by integers, which may lead to inconsistency if you change the order of enum constants in the future. That’s why it's recommended to store them as strings.
Example:
@Entity
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Enumerated(EnumType.STRING)
private OrderStatus status;
// getters and setters
}
Using EnumType.STRING
is a solid approach as it enhances readability in the database, making it clearer what values are being used.
2. Enum Compatibility with Database Values
When the database is populated manually or through some external processes, ensure that the values stored in the database are consistent with your Enum definitions in Java. If the database contains an unexpected value, a java.lang.IllegalArgumentException
can be thrown when fetching data.
To avoid this pitfall, you might consider implementing a custom converter using JPA's @Converter
annotation.
Example:
@Converter(autoApply = true)
public class OrderStatusConverter implements AttributeConverter<OrderStatus, String> {
@Override
public String convertToDatabaseColumn(OrderStatus status) {
return status != null ? status.name() : null;
}
@Override
public OrderStatus convertToEntityAttribute(String dbStatus) {
return dbStatus != null ? OrderStatus.valueOf(dbStatus) : null;
}
}
This converter handles both the conversion from the entity to the database and vice versa, thus ensuring compatibility with unexpected values.
3. Enums and Relationships
When using enums in entities that also have relationships (e.g., OneToMany or ManyToOne), ensure that the relationship does not get affected by the enum's presence. In certain cases, especially when using cascade operations, you may encounter persistence issues.
For instance, do not use an enum for defining a relationship; instead, treat enums as simple attributes. This separation ensures that any enum-related operation does not impact your entity relationships adversely.
4. Enum Type Handling in Queries
Spring Data JPA allows for querying based on enums, but developers must be cautious about their handling. An often overlooked area is the construction of query expressions involving enums.
When fetching data based on enum values, ensure you are using the correct enum reference. If you incorrectly reference the enum fields directly, you may create unexpected runtime errors.
Example Query:
public interface OrderRepository extends JpaRepository<Order, Long> {
List<Order> findByStatus(OrderStatus status);
}
In this example, beware of passing the wrong type when calling the method as it could lead to data type mismatch.
5. Performance Consideration
Depending on the storage type (string vs. ordinal), there can be performance considerations. Enums stored as strings might lead to increased storage overhead and performance issues on larger datasets. It’s crucial to analyze the trade-offs based on the specific requirements of the application.
Best Practices for Using Enums in Spring Data JPA
- Always store enums as strings unless you have compelling reasons to use ordinals.
- Implement converters for better compatibility and handling of database values.
- Separate enum use from relationships to avoid complications.
- Validate enum usage in queries to prevent runtime errors.
- Analyze performance implications when working with enums and large datasets.
My Closing Thoughts on the Matter
While enums can greatly enhance the readability and maintainability of Java applications and Spring Data JPA integrations, inherent pitfalls exist that developers should be wary of. By understanding these common issues—such as appropriate storage mechanisms, database compatibility, and query handling—developers can leverage enums effectively while minimizing the chances of errors.
For more detailed information on Spring Data JPA and its features, refer to the official Spring Data JPA Documentation and explore additional Enum handling techniques here.
By implementing the best practices outlined in this blog post, you can effectively utilize enums within your application while circumventing potential pitfalls that may emerge along the way. Happy coding!