Common Pitfalls in Architecting Large Java Projects
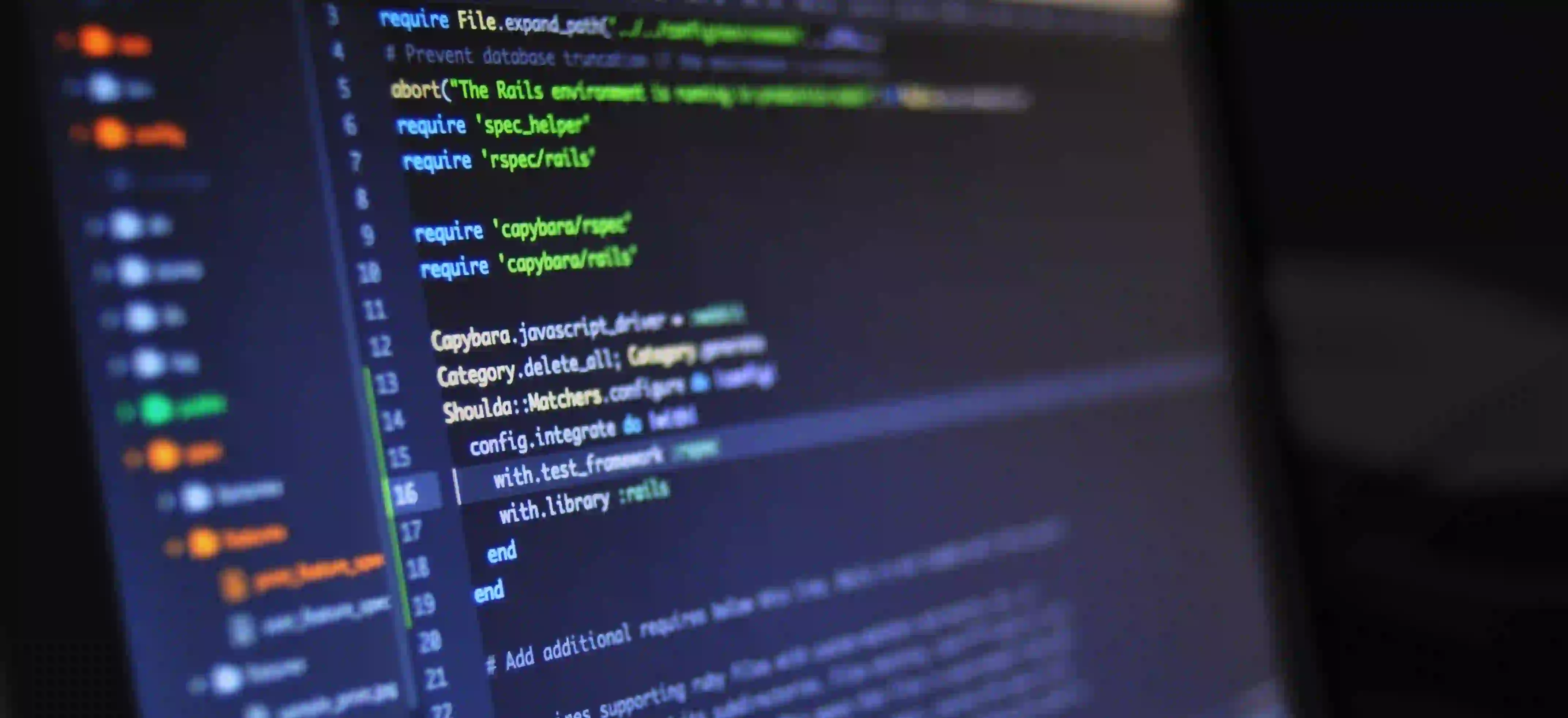
Common Pitfalls in Architecting Large Java Projects
Architecting large Java projects can be an exhilarating yet daunting task. As the complexity grows, so do the challenges. Many developers and teams face common pitfalls that can impede their progress, lead to technical debt, and create scalability issues. In this post, we will discuss these pitfalls, present best practices to avoid them, and provide code snippets to illustrate key concepts.
Table of Contents
- Lack of Clear Requirements
- Choosing the Wrong Technology Stack
- Ignoring Modularity and Code Reusability
- Poor Exception Handling
- Not Implementing Proper Testing Strategies
- Over-Engineering
- Neglecting Performance Considerations
- Summary and Conclusion
1. Lack of Clear Requirements
One of the most significant pitfalls in architecting large Java projects is the lack of clear requirements. Insufficient or vague requirements lead to scope creep, bugs, and rework. The consequences can ripple through the entire project, affecting timelines and morale.
Best Practice: Engage stakeholders early and regularly to gather and clarify requirements. Use tools like JIRA or Trello to track and manage requirements effectively.
2. Choosing the Wrong Technology Stack
Java offers a plethora of frameworks and libraries, such as Spring, Hibernate, and Java EE. However, choosing the wrong technology stack can hinder development and limit scalability.
Why It Matters: The technology stack should align with project requirements, team expertise, and long-term maintainability.
// Example: Choosing Spring Boot for microservices
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Commentary: In this example, Spring Boot simplifies the setup for a microservices-based architecture, significantly reducing boilerplate code. This choice supports agile development and allows quick iterations, critical for larger projects.
3. Ignoring Modularity and Code Reusability
A monolithic approach can lead to tightly coupled components, making the codebase harder to manage and scale. Ignoring modularity can also hinder code reuse and lead to duplication.
Best Practice: Embrace principles like Dependency Injection and Design Patterns to promote modularity.
// Example: Dependency Injection using Spring
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
Commentary: By using Spring's dependency injection, you decouple the UserService
from the UserRepository
. This flexibility makes testing easier and enhances code reuse across other components.
4. Poor Exception Handling
In large projects, having a robust exception handling mechanism is critical. Developers often overlook this aspect, leading to unhandled exceptions that can crash the entire application.
Why It Matters: A comprehensive exception handling strategy can improve user experience and make debugging easier.
// Example: Centralized exception handling using @ControllerAdvice
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
@ResponseStatus(HttpStatus.NOT_FOUND)
public String handleResourceNotFound(ResourceNotFoundException ex) {
return ex.getMessage();
}
}
Commentary: Here, we define a global exception handler using Spring's @ControllerAdvice
. This approach ensures that any ResourceNotFoundException
is caught and handled gracefully, providing a better user experience.
5. Not Implementing Proper Testing Strategies
Testing is often deprioritized in the rush to deliver features, leading to unstable applications. A solid testing strategy is crucial, encompassing unit tests, integration tests, and end-to-end tests.
Best Practice: Introduce automated testing early in the development cycle. Use frameworks like JUnit and Mockito for unit testing.
// Example: Unit testing with JUnit and Mockito
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
void testGetUser() {
UserRepository mockUserRepo = mock(UserRepository.class);
UserService userService = new UserService(mockUserRepo);
User user = new User("John");
when(mockUserRepo.findById(1)).thenReturn(user);
assertEquals("John", userService.getUser(1).getName());
}
}
Commentary: This snippet demonstrates a unit test for the UserService
class using Mockito to mock dependencies. By testing early and often, you can catch bugs before they propagate throughout the system.
6. Over-Engineering
While aiming for a robust architecture, it's easy to fall into the trap of over-engineering. Adding unnecessary complexity can confuse team members and slow down development.
Best Practice: Stick to the KISS (Keep It Simple, Stupid) principle. Opt for solutions that meet current needs without excessive abstraction.
7. Neglecting Performance Considerations
Performance should be a priority from day one. Developers often overlook profiling and optimizing the application until it becomes a significant issue.
Best Practice: Use profiling tools such as VisualVM or YourKit early in the development cycle to identify bottlenecks.
// Example: Lazy Loading in Hibernate
@Entity
public class User {
@OneToMany(fetch = FetchType.LAZY)
private Set<Order> orders;
}
Commentary: By using FetchType.LAZY
, we avoid unnecessary data loading, which can improve performance, especially in applications with large datasets.
8. Summary and Conclusion
Architecting large Java projects is a multifaceted endeavor filled with both challenges and opportunities. By recognizing common pitfalls such as vague requirements, poor technology choices, lack of modularity, and neglecting testing, you can enhance the robustness and maintainability of your applications.
To avoid these pitfalls, prioritize clear communication, choose a fitting technology stack, and embrace good design practices. Furthermore, invest in performance optimizations and thorough testing to create a deployable application that performs well under load.
For more insights into Java architecture best practices, consider checking out the Spring Framework documentation and resources on design patterns that can significantly enhance your development workflow.
By adopting these strategies, you’ll not only avoid common pitfalls but also lay a strong foundation for a successful Java project that can grow and adapt to future needs. Happy coding!