Choosing the Right Microservices Framework for Your Project
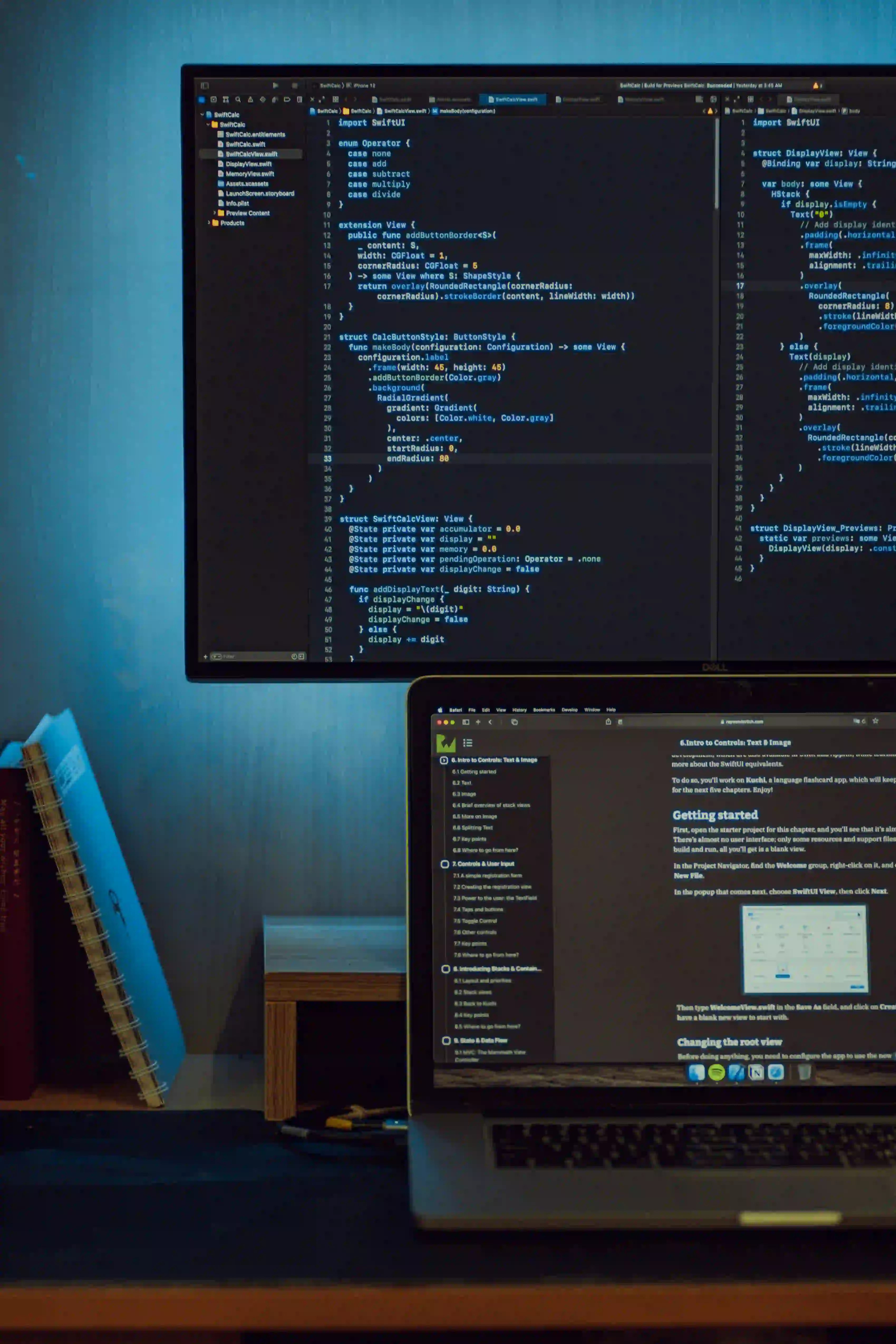
Choosing the Right Microservices Framework for Your Project
In today’s fast-paced technology landscape, microservices architecture has emerged as a leading model for developing scalable and flexible applications. Companies ranging from startups to tech giants have adopted this architecture to enhance their development processes. However, the key to effective microservices lies in selecting the right framework. This blog post discusses the key considerations when choosing a microservices framework and outlines some popular options in the industry.
What are Microservices?
Microservices are a software development technique that structures an application as a collection of loosely coupled services. This architecture allows teams to develop, deploy, and scale services independently, ultimately leading to a more robust and agile software ecosystem.
Key Benefits of Microservices:
- Scalability: Independently scalable services can optimize resource use.
- Flexibility: Different technologies can be utilized for different services.
- Resilience: If one service fails, it does not cause the entire application to fail.
- Continuous Deployment: Smaller and frequent updates are possible, allowing for faster release cycles.
Key Criteria for Selecting a Microservices Framework
When searching for the right microservices framework, consider the following:
1. Language Support
Choose a framework that aligns with the programming language your team is most comfortable with. Popular languages for microservices development include:
- Java: Known for its portability and performance.
- Node.js: Offers a non-blocking I/O model, making it ideal for I/O-heavy applications.
2. Community and Support
A vibrant community provides extensive documentation, resources, and a support network. Popular frameworks tend to have robust communities that facilitate faster problem-solving.
3. Integration Capabilities
A framework should seamlessly integrate with CI/CD tools, databases, and other services. This integration is crucial for automated testing and deployment.
4. Scalability and Performance
Evaluate the framework’s ability to manage increased traffic and ensure fast response times.
5. Learning Curve
Consider the time your team will spend learning the framework. A complex framework may lead to delays in development.
Popular Microservices Frameworks
Here, we highlight some popular choices for microservices frameworks, specifically for Java and Node.js.
1. Spring Boot (Java)
Spring Boot is particularly popular in the Java ecosystem for developing microservices. Its convention-over-configuration philosophy allows developers to create production-ready applications with minimal setup.
Example Code:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Commentary:
@SpringBootApplication
: This annotation simplifies the configuration and setup by activating component scanning and enabling auto-configuration. It removes boilerplate code that developers would traditionally write for Java applications.SpringApplication.run()
: This method launches the application by creating the application context and initializing the Spring components.
Advantages of Spring Boot:
- Production Ready: It offers built-in tools for monitoring and managing applications.
- Dependency Management: Simplifies adding libraries and dependencies, facilitating integration with various systems.
For a more in-depth understanding of Spring Boot and its capabilities, visit the official Spring documentation.
2. Quarkus (Java)
Quarkus is a relatively newer framework tailored for Kubernetes. It was designed to optimize Java specifically for cloud-native development.
Example Code:
import javax.ws.rs.GET;
import javax.ws.rs.Path;
@Path("/hello")
public class GreetingResource {
@GET
public String hello() {
return "Hello, Quarkus!";
}
}
Commentary:
@Path("/hello")
: This annotation defines a REST endpoint at the path/hello
.- The method
hello()
returns a simple string response, demonstrating how to build a basic REST service quickly.
Advantages of Quarkus:
- Fast Boot Times: It offers blazingly fast startup times and low memory overhead.
- Kubernetes-Ready: Built with Kubernetes in mind, it provides seamless integration.
To explore more about Quarkus, visit the Quarkus website.
3. Express.js (Node.js)
Express.js is a minimalist web framework for Node.js and facilitates building web applications and APIs easily. It’s particularly effective for microservices due to its simplicity and flexibility.
Example Code:
const express = require('express');
const app = express();
app.get('/hello', (req, res) => {
res.send('Hello, Express!');
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
Commentary:
app.get()
: This method sets up a GET request endpoint at the/hello
path.res.send()
: Sends the response back to the client.
Advantages of Express.js:
- Fast Development: Rapid creation of web applications and APIs with lower overhead.
- Middleware Support: Allows for middleware to process requests before reaching the endpoint, providing high customization.
4. Micronaut (Java)
Micronaut is a modern JVM-based framework for building modular and easily testable microservices. It emphasizes the use of annotations to simplify configuration and dependency injection.
Example Code:
import io.micronaut.runtime.Micronaut;
public class Application {
public static void main(String[] args) {
Micronaut.run(Application.class);
}
}
Commentary:
Micronaut.run()
: This method initializes the application and prepares it for handling requests.
Advantages of Micronaut:
- Low Memory Footprint: Designed for microservices, enabling efficient resource utilization.
- Ahead-of-Time Compilation: Reduces startup time and improves performance.
The Bottom Line
Choosing the right microservices framework for your project can significantly influence your development process and the scalability of your application. Whether you lean toward Spring Boot for its robust features or Express.js for its minimalism, always align your choice with your team's strengths and project requirements.
Remember to consider the scalability, support, integration capabilities, and, most importantly, the learning curve of the framework you select. With microservices, the mantra "build small, fail fast" holds true—be prepared to adapt and evolve as your project scales.
For further reading on microservices architecture and how to implement it effectively, check Martin Fowler’s article on microservices.
By making an informed choice in your microservices framework, your project stands to benefit immensely in the long run. Happy coding!