Common Errors When Creating Your First Spring Boot REST API
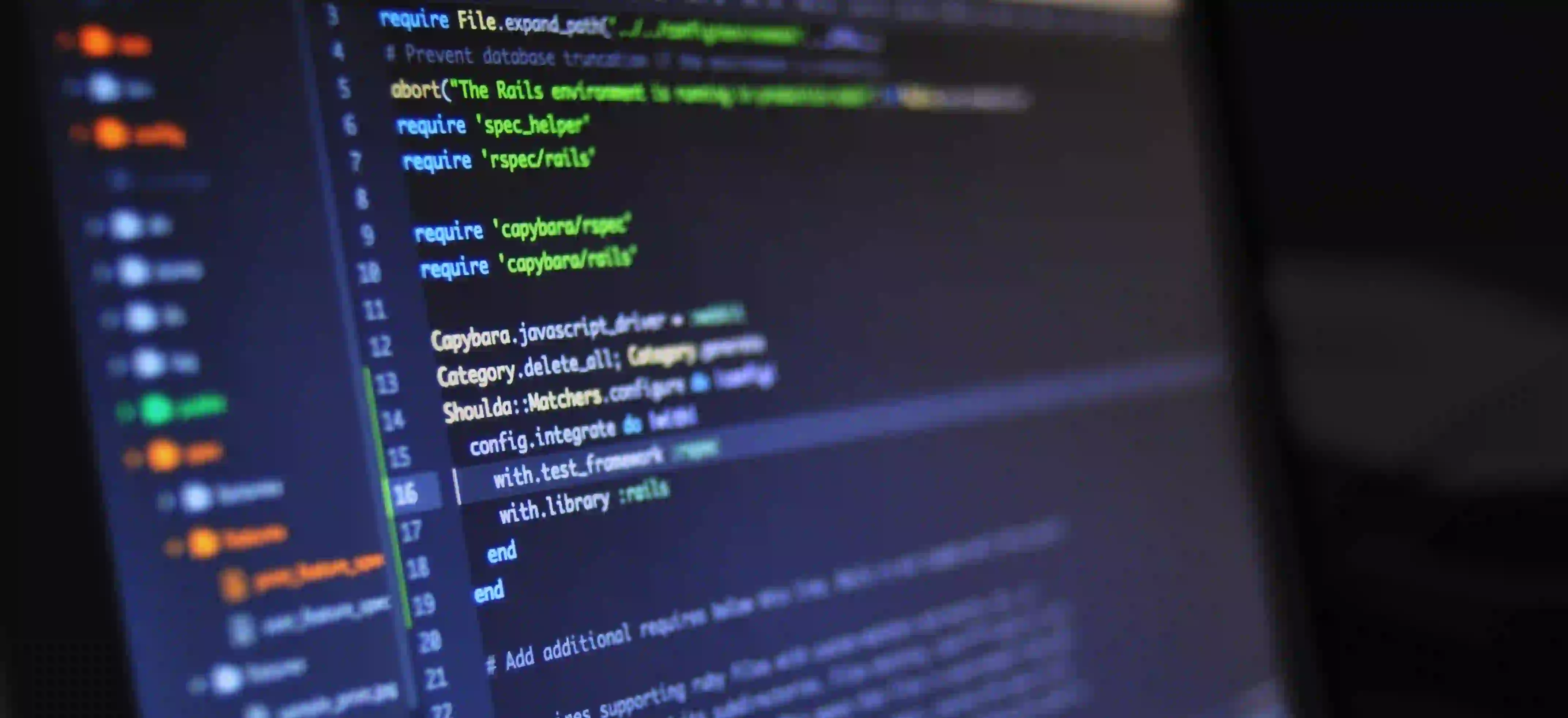
Common Errors When Creating Your First Spring Boot REST API
Creating your first Spring Boot REST API is an exciting journey, but it can also be fraught with pitfalls if you're not careful. Spring Boot simplifies the process of developing Java applications, but certain common errors can trip up new developers. In this post, we will explore these errors, how to avoid them, and best practices for building a robust REST API.
What is Spring Boot?
Before diving into the common errors, let’s establish a foundation. Spring Boot is an extension of the Spring framework, designed to simplify the development of new applications. It eliminates much of the boilerplate code and configuration that developers often face. For those you who want to learn more, consider reading Spring Boot Documentation.
Starting with Spring Boot
When you set out to create a REST API, you typically start by setting up a Spring Boot project. You can use the Spring Initializr to bootstrap a new application. Select your dependencies such as Spring Web, Spring Data JPA, and H2 Database (for this example).
Common Errors
1. Missing Dependencies
One of the most common errors when creating a Spring Boot application is missing dependencies. If Spring Web is not included, your application won’t handle HTTP requests.
Solution
Make sure you have the necessary dependencies in your pom.xml
or build.gradle
. For example, your pom.xml
should include:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
This dependency includes everything you need to build a web application, including RESTful services.
2. Invalid or Missing Annotations
Using the wrong annotations can lead to various issues. One common mistake is forgetting to annotate your main application class with @SpringBootApplication
.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
Why This Matters
The @SpringBootApplication
annotation is a composite of several other annotations that enable component scanning, auto-configuration, and more. If you omit it, your application won’t work correctly.
3. Incorrect URL Mapping
Another common issue is incorrect URL mapping in your controller. The @RequestMapping
or @GetMapping
annotations should be correctly set up to handle the incoming HTTP requests.
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MyController {
@GetMapping("/api/hello")
public String sayHello() {
return "Hello, World!";
}
}
Debugging Tips
- Check if the mapping annotations are pointing to the correct URL.
- Always ensure that the HTTP method (GET, POST, PUT, DELETE) you are using in your application corresponds with the client request.
4. Missing or Incorrect Response Entity
Returning the wrong type of object or not specifying the correct response entity can result in client-side errors.
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
@RestControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception ex) {
return new ResponseEntity<>("Internal Server Error", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Explanation
Using ResponseEntity
allows you to customize the HTTP response, including status codes and response bodies. Proper error handling enhances the user experience by providing meaningful feedback.
5. CORS Issues
Another characteristic stumbling block involves Cross-Origin Resource Sharing (CORS). If your API is hosted on a different port than your frontend application, you might encounter CORS errors when the frontend tries to access your API.
Solution
To enable CORS, you can add a configuration class:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/api/**").allowedOrigins("http://localhost:3000");
}
}
Why CORS Matters
CORS headers allow for secure cross-origin requests. By configuring it correctly, you make your API accessible to the required domains while preserving security standards.
6. Invalid JSON Response
When building a REST API, your JSON responses should match the expected format. Mismatches can lead to deserialization errors on the client side.
import com.fasterxml.jackson.annotation.JsonProperty;
public class User {
private String name;
private int age;
public User(@JsonProperty("name") String name, @JsonProperty("age") int age) {
this.name = name;
this.age = age;
}
// Getters and setters
}
Debugging JSON Issues
- Use Postman or similar tools to test your API responses.
- Utilize JSON validation tools to confirm that your output adheres to the expected standards.
7. Poor Documentation
When building an API, failing to document your endpoints and their respective input/output types can lead to confusion for developers consuming your API.
Best Practice
Use tools like Swagger for API documentation. By integrating Swagger with Spring Boot, you can automatically generate interactive API documentation.
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
Benefits
Having Swagger UI will allow developers to explore your API easily and understand how to interact with it efficiently.
The Bottom Line
Building your first Spring Boot REST API can be a rewarding experience, filled with opportunities to learn and grow as a developer. Be mindful of these common errors, and leverage the solutions detailed in this blog post. With awareness and preparation, you'll be well on your way to creating high-quality REST APIs.
For further reading, you might find these resources helpful:
- Spring Boot Reference Documentation
- RESTful Web Services with Spring Boot
- Understanding Spring’s RestTemplate
Happy coding!