Mastering Single Character Input in Java: The Common Pitfall
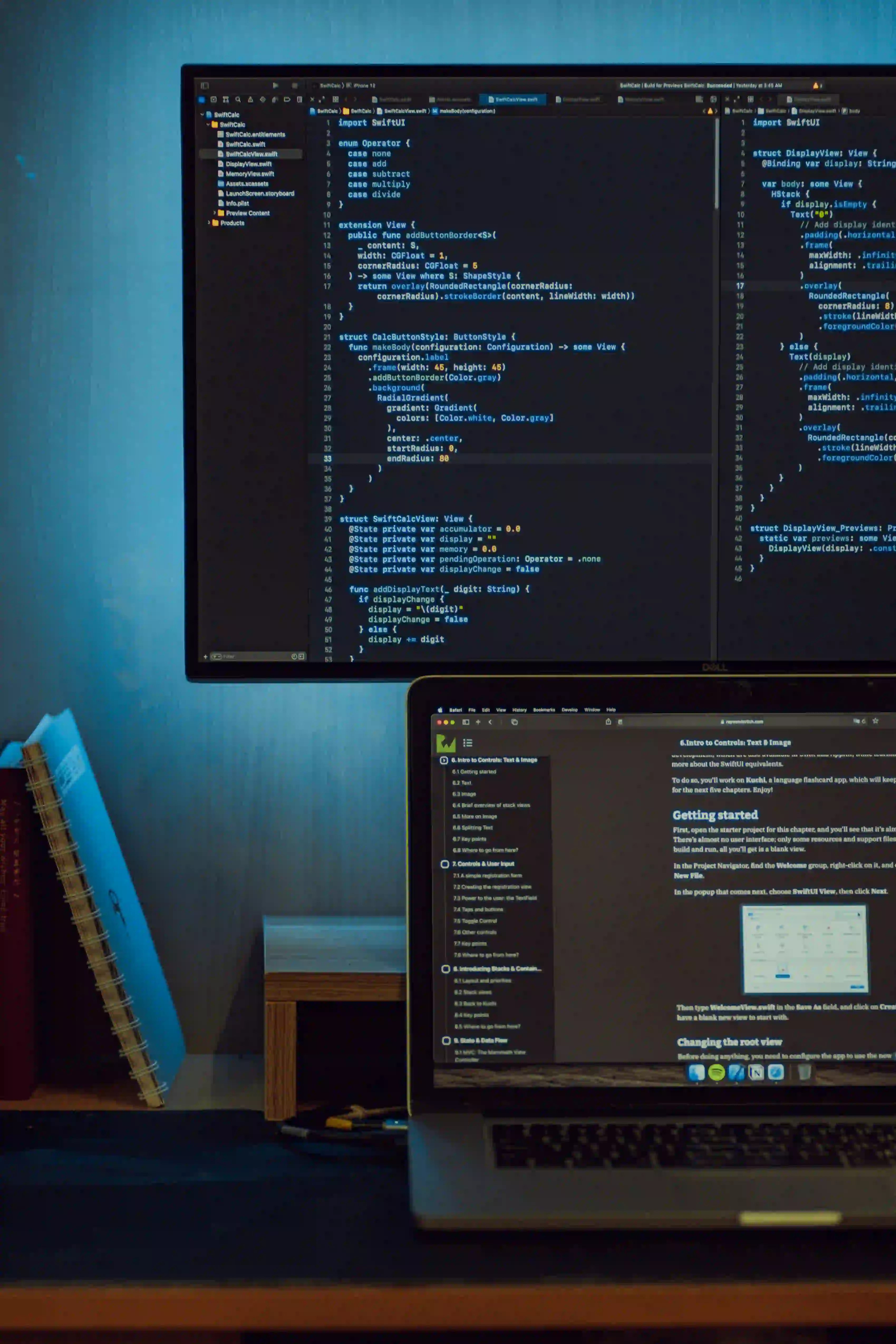
Mastering Single Character Input in Java: The Common Pitfall
When it comes to reading user inputs in Java, developers often run into common pitfalls that can lead to unexpected behaviors and bugs. One such area of confusion lies in capturing single character inputs from the user. In this blog post, we will delve into the intricacies of handling single character input in Java, pinpointing common mistakes and presenting effective solutions.
Why Single Character Input Can Be Tricky
At first glance, reading a single character seems straightforward. However, the nuances of Java's Scanner
class can catch developers off guard, particularly in how various input types are handled. Characters in Java can be represented by the char
data type, which makes them distinct from strings. Therefore, trying to read a character input that is naively implemented can often lead to surprises.
The Standard Approach: Using Scanner
Java's Scanner
class is one of the most frequently used methods for reading input. Below is a simple code snippet to read a single character input.
import java.util.Scanner;
public class SingleCharacterInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Please enter a single character: ");
String input = scanner.nextLine();
// Check if the input length is exactly 1
if (input.length() == 1) {
char characterInput = input.charAt(0);
System.out.println("You entered: " + characterInput);
} else {
System.out.println("Invalid input. Please enter exactly one character.");
}
scanner.close();
}
}
Code Commentary:
- Scanner Initialization: The
Scanner
object is created to read from the standard input stream. - Input Capture: A call to
nextLine()
captures the entire line as a string. - Input Validation: The length of the string is checked to ensure it's exactly 1.
- Character Extraction: Using
charAt(0)
, the character is extracted if the input is valid.
This code effectively validates the input, ensuring that only a single character is processed. However, there are pitfalls you need to be aware of.
Common Pitfall: Buffering Issues
Consider a scenario where the user needs to input multiple entries in succession. If developers are not cautious about clearing the input buffer, they might retrieve unexpected results. For instance, if the user inputs more than one character, only the first one is considered. If not handled properly, the remaining characters remain in the buffer for the next read.
Improved Practices for Multiple Inputs
To address this issue, here’s an enhanced version of the single character input code that performs better in scenarios involving multiple entries.
import java.util.Scanner;
public class ImprovedSingleCharacterInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Please enter a single character (or 'q' to quit): ");
String input = scanner.nextLine();
if (input.equals("q")) {
break; // Exit condition for the loop
}
if (input.length() == 1) {
char characterInput = input.charAt(0);
System.out.println("You entered: " + characterInput);
} else {
System.out.println("Invalid input. Please enter exactly one character.");
}
}
scanner.close();
}
}
Breakdown of the Code Changes:
- Loop for Continuity: The
while(true)
loop allows for continuous input until the user decides to quit by entering 'q'. - Exit Condition: A simple string comparison lets the user break out of the loop.
- Input Handling: Similar input validation ensures all inputs are handled correctly, reminding users of the requirement.
Working with Special Characters
Java allows the entry of special characters (like !
, @
, etc.), and it treats them as valid inputs. You can extend your validation logic to handle or restrict certain special characters depending on your application needs. For instance, you can utilize regular expressions for better control.
if (input.matches("[^a-zA-Z]")) {
System.out.println("Please enter an alphanumeric character.");
}
Why Regular Expressions?
Regular expressions offer powerful string manipulation solutions. By using matches
, you can validate the input against defined patterns efficiently. This can prevent unwanted characters from entering your system unnecessarily, making your application more stable and predictable.
Common Use Cases for Single Character Input
Understanding where and how to use single character input can significantly enhance your application's functionality. Here are a few practical use cases:
- Menu Navigation: In console applications, single character inputs can be used to navigate between options efficiently.
- Game Development: Capture user actions in real-time, like keystrokes for movement or actions.
- User Interactions: Collect single commands in applications that require instant responses, such as text-based games.
Wrapping Up
Handling single character input in Java might seem simple, but nuances can complicate the process. By comprehending the common pitfalls, utilizing tricks to manipulate the input buffer, and validating entries, developers can create robust applications. Always remember to consider user input in the wider context of your application to ensure it behaves predictably.
For more reading on handling user input in Java, the official Java documentation is an excellent resource. Additionally, if you wish to learn about character encoding and its importance in Java, check out details on Java Character Encoding.
With these insights and practices, you can master single character input in Java while avoiding the common pitfalls. Happy coding!