Struggling with Mockito? Tips for Mocking Autowired Fields!
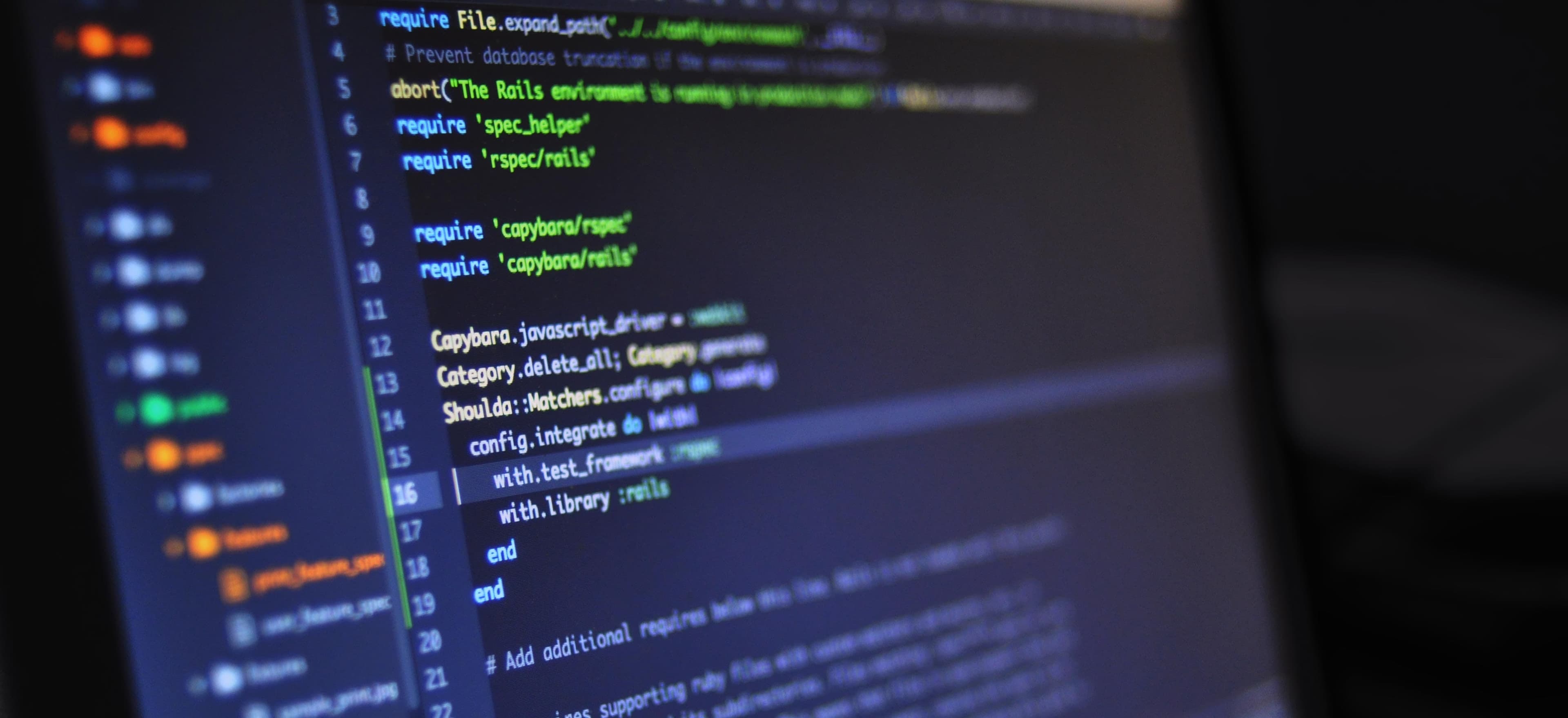
- Published on
Struggling with Mockito? Tips for Mocking Autowired Fields!
Mockito is one of the most popular frameworks for mocking in Java unit tests. It's lightweight, easy to use, and can significantly enhance your testing strategy. However, when it comes to mocking @Autowired
fields in Spring components, developers can often find themselves in a quagmire. In this blog post, we will walk through the best practices for mocking autowired fields with Mockito.
Understanding @Autowired in Spring
Before we dive into mocking, it's important to understand what @Autowired
does. This Spring annotation allows Spring to automatically resolve and inject collaborating beans into your application context. It can significantly simplify the configuration of complex interactions.
However, when testing components that depend on autowired beans, you typically want to isolate the unit under test. This is where Mockito comes in.
Step 1: Setup Your Testing Environment
To start using Mockito with Spring, ensure you have the necessary dependencies in your pom.xml
if you're using Maven:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>4.0.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
If you're using Gradle, your build.gradle
would resemble this:
testImplementation 'org.mockito:mockito-core:4.0.0'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
Step 2: Use Mockito Annotations
Mockito provides several annotations to help simplify your test setup. You will primarily use @Mock
, @InjectMocks
, and @RunWith(MockitoJUnitRunner.class)
.
Here's how we can set this up:
import org.junit.jupiter.api.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class MyServiceTest {
@Mock
private CollaboratingService collaboratingService; // The autowired field we want to mock
@InjectMocks
private MyService myService; // The class under test
@BeforeEach
public void init() {
MockitoAnnotations.openMocks(this); // Initializes mocks
}
@Test
public void testCollaboratingService() {
// Arrange
when(collaboratingService.someMethod()).thenReturn("mocked response");
// Act
String response = myService.useCollaboratingService();
// Assert
assertEquals("mocked response", response);
}
}
Why Use @InjectMocks
?
Using @InjectMocks
tells Mockito to instantiate the class under test (MyService
) and inject the mocks created with @Mock
(CollaboratingService
) into it. This way, you can simulate dependencies without relying on the actual implementations.
Step 3: Avoid the Spring Context
In many cases, you'll want to avoid loading the entire Spring context for unit tests. Using the @Mock
and @InjectMocks
annotations enables you to create lightweight tests. However, if you need to run tests against the Spring context, you can use @SpringBootTest
as showcased earlier.
If you don’t need the Spring features for your tests, you can simply annotate the test class with @ExtendWith(MockitoExtension.class)
instead. Here’s an example:
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.junit.jupiter.MockitoExtension;
@ExtendWith(MockitoExtension.class)
public class MyServiceTest {
@Mock
private CollaboratingService collaboratingService;
@InjectMocks
private MyService myService;
@Test
public void testCollaboratingService() {
when(collaboratingService.someMethod()).thenReturn("mocked response");
String response = myService.useCollaboratingService();
assertEquals("mocked response", response);
}
}
Why Prefer Mockito Extension?
Using @ExtendWith(MockitoExtension.class)
reduces the overhead of having a full Spring context, thus speeding up your tests significantly. Make sure to only use this approach if your service under test does not require the full Spring setup.
Step 4: Handling Final Classes
Sometimes, you might run into the issue of final classes. By default, Mockito cannot mock final classes. To enable this, you need to add the Mockito inline dependency to your project:
For Maven:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-inline</artifactId>
<version>4.0.0</version>
<scope>test</scope>
</dependency>
And for Gradle:
testImplementation 'org.mockito:mockito-inline:4.0.0'
Step 5: Using the Mocked Beans
Once you have set up your mocks, you can control their behavior and verify interactions. The when(...).thenReturn(...)
pattern allows you to specify the behavior of your mocked beans. For example, consider the method useCollaboratingService()
in your MyService
class that calls a method from collaboratingService
.
public String useCollaboratingService() {
return collaboratingService.someMethod();
}
Why Mock Its Dependencies?
Mocking the dependencies allows you to focus on testing only the behavior of the unit under test. This leads to more robust tests and helps avoid unintended side effects from actual implementations.
The Closing Argument
Mocking @Autowired
fields in Spring using Mockito is easy once you understand the basic principles. By following a structured approach—setting up your testing environment, using Mockito annotations, bypassing the Spring context when possible, and handling final classes—you can create clean, efficient unit tests.
For continued learning, you might want to refer to the official Mockito Documentation for more advanced uses. Also, consider looking over the Spring Testing Documentation to help deepen your understanding of integrating testing strategies in Spring applications.
By following these tips, you will become more proficient in writing unit tests for your Spring applications, helping you catch bugs early, leading to better software quality. Happy testing!
Checkout our other articles