Overcoming Common Challenges in Spring Boot with AngularJS
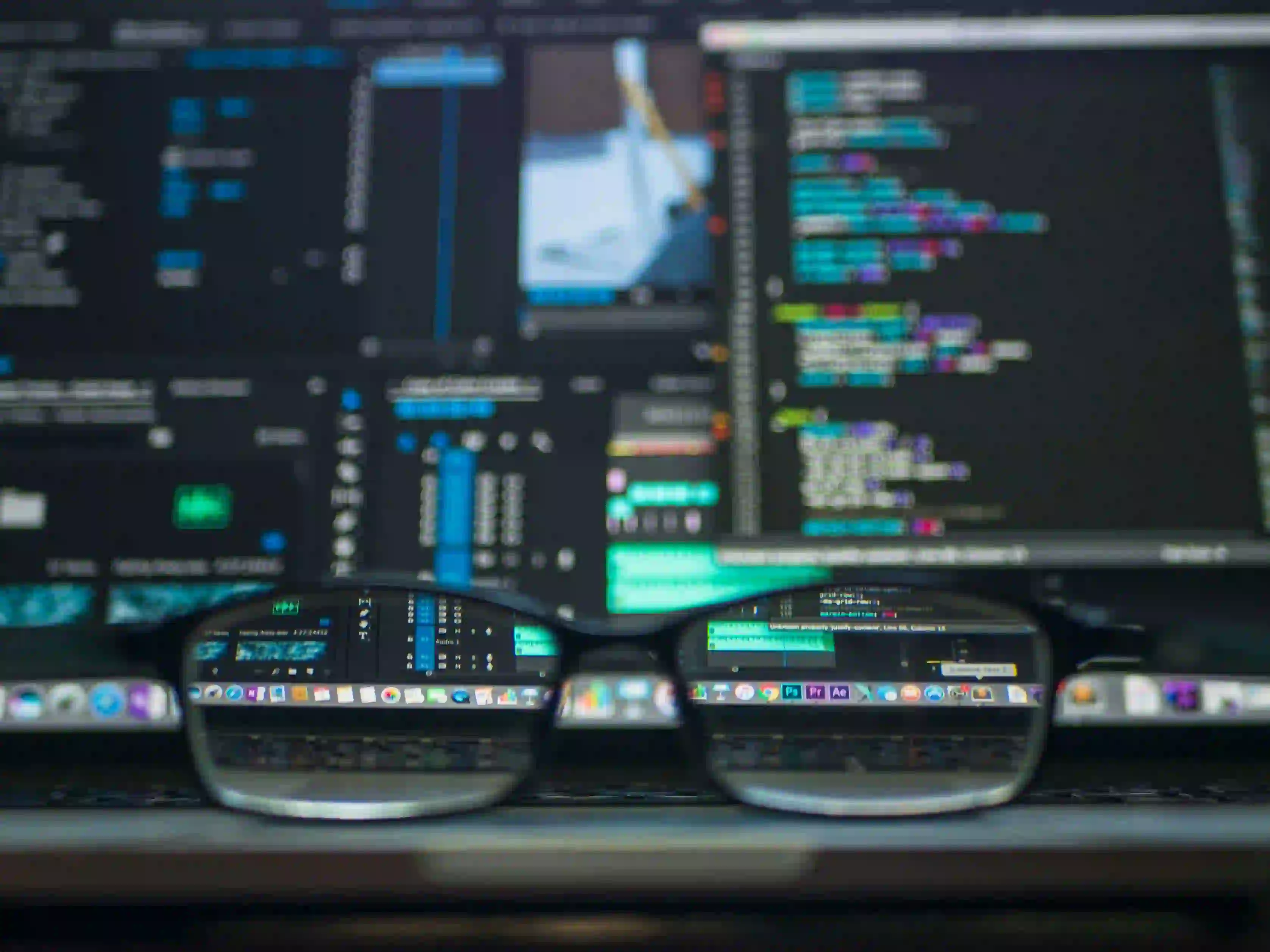
Overcoming Common Challenges in Spring Boot with AngularJS
In today's web development landscape, integrating a robust back-end framework like Spring Boot with a powerful front-end JavaScript framework such as AngularJS can result in a highly performant web application. However, developers often face several challenges during this integration process. This blog post explores these obstacles and offers practical solutions, ensuring smooth communication between the server and the client.
Table of Contents
- Understanding the Spring Boot and AngularJS Ecosystem
- Challenge 1: CORS Issues
- Challenge 2: API Versioning
- Challenge 3: Data Binding
- Challenge 4: Error Handling
- Challenge 5: Security
- Conclusion
Understanding the Spring Boot and AngularJS Ecosystem
Spring Boot is a powerful Java-based framework that simplifies the setup and development of new Spring applications. It is designed to get you up and running quickly with minimal fuss. On the other hand, AngularJS is a structural framework for dynamic web applications. It allows developers to extend HTML attributes using directives, resulting in a more functional and interactive user interface.
Linking Both Frameworks
Together, these frameworks can provide a streamlined development experience. However, integrating them effectively requires attention to various challenges that may come up along the way.
Challenge 1: CORS Issues
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers. It restricts web pages from making requests to a different domain than the one from which they originated. When your AngularJS app attempts to access APIs on a different port (or server) than where it is hosted, you may run into CORS-related issues.
Solution
To resolve CORS problems, you need to configure your Spring Boot application to allow requests from your AngularJS app's domain. Below is an example demonstrating how to enable CORS:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://localhost:4200") // Replace with your Angular app URL
.allowedMethods("GET", "POST", "PUT", "DELETE", "OPTIONS")
.allowedHeaders("*");
}
}
Why This Matters
This configuration allows requests from your AngularJS app running on http://localhost:4200
to access your Spring Boot application, thereby resolving common CORS issues.
Challenge 2: API Versioning
As your application grows, you will likely need to implement new endpoints or make changes to the existing ones. Proper API versioning helps maintain backward compatibility for clients that are already using older versions of your API.
Solution
In Spring Boot, you can version your APIs in multiple ways. A simple yet effective method is to use URL versioning. Here is an example:
@RestController
@RequestMapping("/api/v1/users") // Versioned endpoint
public class UserController {
@GetMapping
public List<User> getAllUsers() {
return userService.findAll();
}
}
Why This Matters
By adopting versioning practices, you can seamlessly introduce breaking changes while providing uninterrupted service to your users who rely on legacy APIs.
Challenge 3: Data Binding
Data binding is crucial in AngularJS that allows developers to synchronize data between the model and the view. However, problems can arise when data is fetched from a Spring Boot application, especially when the response format does not align with Angular's expectations.
Solution
Make sure your Spring Boot application returns JSON data in a format that can be easily consumed by Angular:
@GetMapping("/api/users")
public ResponseEntity<List<User>> getUsers() {
List<User> users = userService.findAll();
return new ResponseEntity<>(users, HttpStatus.OK);
}
Why This Matters
Returning a well-structured JSON response allows AngularJS to bind the data directly, improving the overall user experience and reducing the complexity of data manipulation.
Challenge 4: Error Handling
Inevitably, errors will occur. Handling them effectively on both client and server applications is essential. From incorrect inputs to failure in service calls, you want graceful degradation of service.
Solution
In Spring Boot, you can implement global error handling using the @ControllerAdvice
annotation:
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleAllExceptions(Exception ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Why This Matters
This allows your application to respond uniformly when errors occur, enabling the front end to handle these errors gracefully and inform users about the issues.
Challenge 5: Security
No web application is complete without a proper security mechanism. With AngularJS communicating with Spring Boot, you need to ensure that your data and endpoints are protected against malicious attacks.
Solution
Leveraging Spring Security with JWT (JSON Web Token) is a standard approach. Below is an example configuration for securing your API:
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/api/v1/auth/**").permitAll() // Public endpoints
.anyRequest().authenticated(); // Protected endpoints
}
}
Why This Matters
This method allows you to secure specific endpoints while ensuring that public routes are accessible without authentication, thus balancing usability with security.
The Bottom Line
Integrating Spring Boot with AngularJS for web application development provides ample opportunities for building scalable and maintainable applications. By proactively addressing common challenges such as CORS, API versioning, data binding, error handling, and security, you can create a seamless experience for both developers and users.
Learning and overcoming these challenges not only enhances your technical skills but also equips you with the knowledge needed to tackle future projects with confidence. For more resources on Spring Boot and AngularJS integration, you can explore the official Spring Boot Documentation and the AngularJS Official Guide.
By understanding these fundamental aspects, you'll be well on your way to mastering the integration of these two powerful frameworks. Happy coding!