Troubleshooting OkHttpClient in WildFly 9: Common Pitfalls
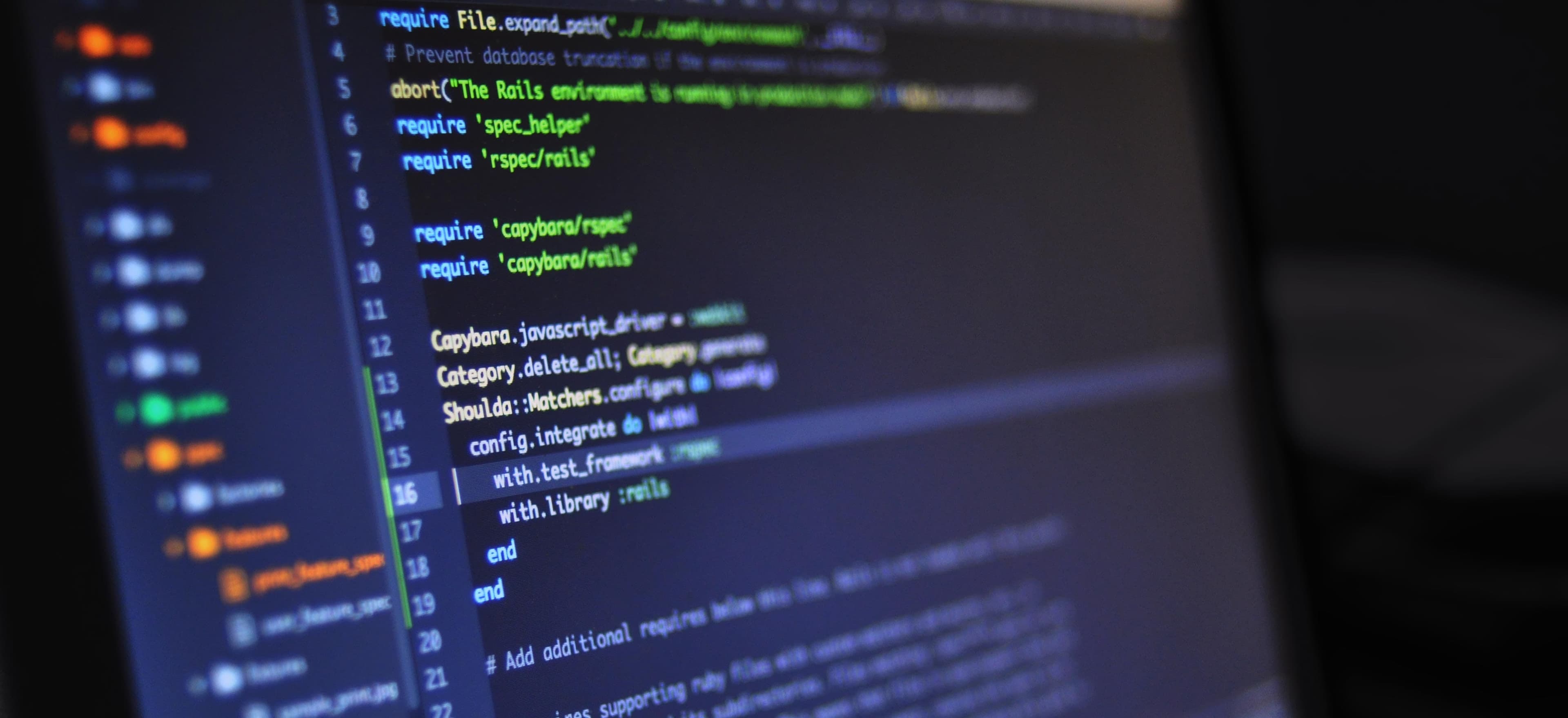
- Published on
Troubleshooting OkHttpClient in WildFly 9: Common Pitfalls
OkHttp is a popular HTTP client for Java applications, well-known for its efficiency and robust capabilities. When running an application that utilizes OkHttpClient on a server like WildFly 9, developers often encounter issues that can hinder their progress. This blog post will address common pitfalls one may face when integrating OkHttpClient into a WildFly 9 environment and offer plausible solutions.
Understanding OkHttpClient and WildFly Setup
Before diving into troubleshooting, it's crucial to understand the stack you're working with. WildFly is an application server providing a runtime environment for your Java applications, while OkHttpClient allows for seamless API communication over HTTP/HTTPS.
When OkHttpClient is deployed to WildFly, several factors can impact its performance, including dependency conflicts, configuration errors, and networking issues. Thus, knowing where to look during troubleshooting can save you considerable time.
Key Factors to Consider
- Dependency Management: Ensure that the correct versions of OkHttp and its dependencies are packaged with your application.
- JNDI Configuration: Properly configure the Java Naming and Directory Interface (JNDI) for connectivity.
- Thread Management: Understand how OkHttp manages threads, as mismanagement can lead to threading issues within the WildFly environment.
Common Pitfall #1: Dependency Conflicts
The Problem
One of the most prevalent issues comes from dependency conflicts. WildFly contains its set of libraries, and if your application bundles different versions of libraries (like OkHttp's), conflicts can arise.
The Solution
Make use of Maven or Gradle to manage your dependencies effectively. To ensure you are using the correct version, always use the exclusion feature to omit transitive dependencies that may conflict with WildFly's existing libraries.
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>4.9.1</version>
<exclusions>
<exclusion>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
</exclusion>
</exclusions>
</dependency>
Here, we exclude an older version of JUnit that might be included transitively. Always check for library compatibility when troubleshooting.
Relevant Resource
For a detailed guide on managing dependencies with Maven, visit the Maven Dependency Management.
Common Pitfall #2: Improper JNDI Configuration
The Problem
JNDI is critical when your application accesses resources like databases or message queues. A misconfigured JNDI resource can lead to failures in establishing connections.
The Solution
Ensure you define your JNDI resources accurately in the standalone.xml
configuration file or through the WildFly management console. Below is an example of how to configure a JNDI resource.
<resource-local>
<jndi-name>java:jboss/datasources/MyDS</jndi-name>
<connection-url>jdbc:mysql://localhost:3306/mydb</connection-url>
<driver>mysql</driver>
<user-name>username</user-name>
<password>password</password>
</resource-local>
After defining your JNDI resource, access it in your Java code:
Context ctx = new InitialContext();
DataSource ds = (DataSource) ctx.lookup("java:/jboss/datasources/MyDS");
This will ensure that your application can obtain the necessary data source efficiently.
Common Pitfall #3: Connection Timeouts
The Problem
In a cloud environment or clustered services, network issues can arise, resulting in connection timeouts. Developers often misconfigure OkHttpClient's timeouts.
The Solution
Tuning the timeout settings of OkHttpClient can enhance reliability. Here’s an example of configuring connection and read timeout settings:
OkHttpClient client = new OkHttpClient.Builder()
.connectTimeout(30, TimeUnit.SECONDS) // Maximum time to establish a connection
.readTimeout(30, TimeUnit.SECONDS) // Maximum time to read response data
.writeTimeout(30, TimeUnit.SECONDS) // Maximum time to write data
.build();
This setup provides ample time for network operations and prevents premature disconnections.
Why It Matters
Setting adequate timeout values ensures that transient issues do not lead to failing your application. By tuning these settings, you create resilience against fluctuating network conditions.
Common Pitfall #4: SSL Configuration Issues
The Problem
SSL handshake failures can occur when the server and client are misconfigured or incompatible. WildFly may enforce stricter security protocols that your OkHttpClient application does not handle.
The Solution
Ensure that your OkHttpClient has the proper SSL configuration. Here’s how you can achieve this:
OkHttpClient client = new OkHttpClient.Builder()
.sslSocketFactory(sslSocketFactory, trustManager)
.hostnameVerifier((hostname, session) -> true) // Notes: This is not secure
.build();
In this example, replace the sslSocketFactory
and trustManager
with your properly configured classes. Always aim to replace the hostname verification mechanism to enhance security.
Why You Should Care
Proper SSL handling prevents man-in-the-middle attacks and ensures the integrity of data exchanged between your application and external services.
To Wrap Things Up
Troubleshooting OkHttpClient within a WildFly 9 environment can feel overwhelming at first. However, understanding the common pitfalls—dependency management, JNDI configurations, connection timeouts, and SSL issues—can significantly mitigate challenges.
Remember to stay up-to-date on best practices for both OkHttp and WildFly through official documentation and community forums. These resources will help you avoid common mistakes and streamline your development process.
By implementing the solutions discussed, you can ensure smoother integration of OkHttpClient with WildFly, positioning your application for success. If you have additional tips or questions, feel free to leave them in the comments below!
Checkout our other articles