Understanding the Hidden Costs of Software Development Change
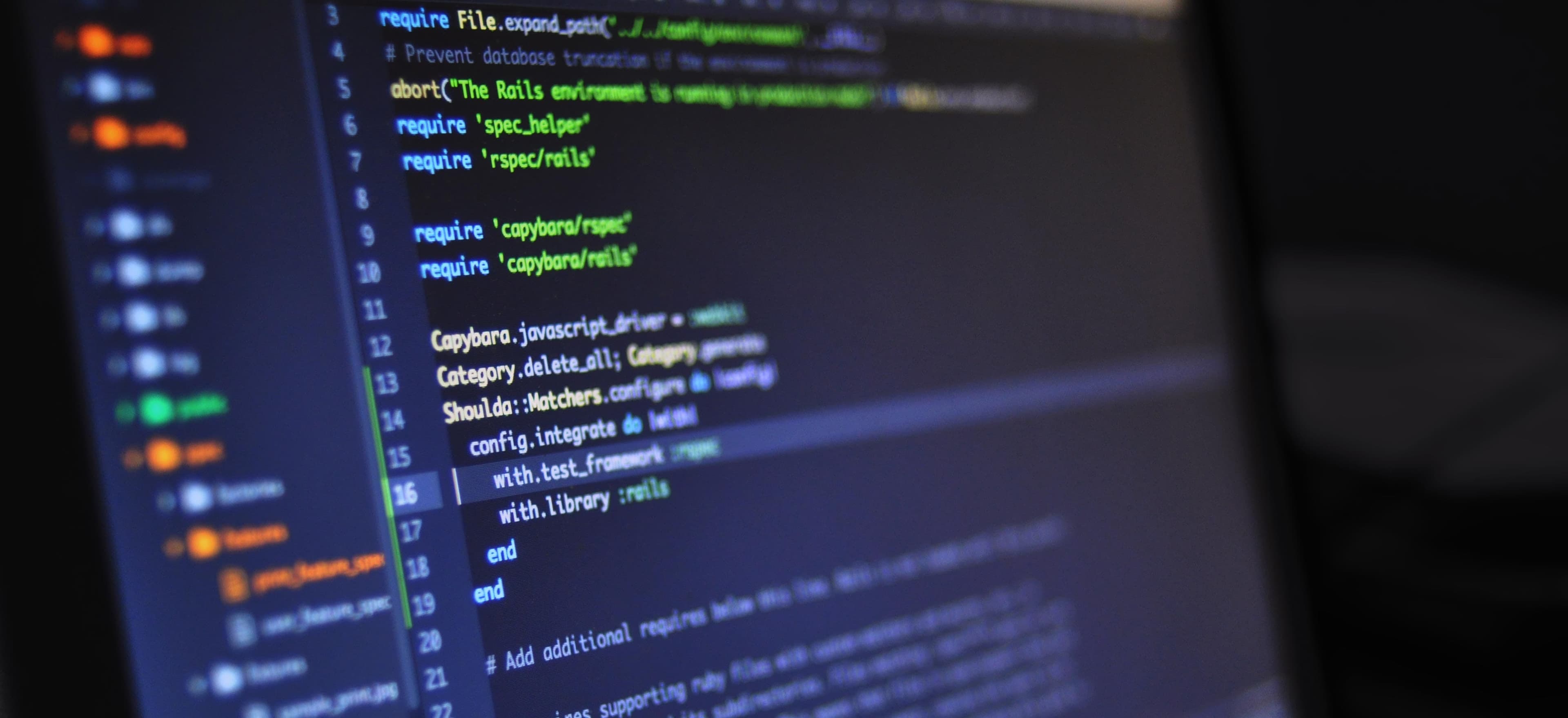
- Published on
Understanding the Hidden Costs of Software Development Change
Software development is a complex process, rife with challenges that extend beyond just writing code. As projects evolve, varying requirements often lead to changes that introduce hidden costs. Understanding these costs is crucial for developers, project managers, and stakeholders to mitigate risks and ensure efficient project delivery. This post explores the hidden costs of software development changes and offers strategies to manage them effectively.
The Nature of Software Changes
Software development is inherently dynamic. Requirements can shift based on user feedback, business needs, or technological advancements. While changes can enhance the final product, they often come at a price. The Project Management Institute estimates that nearly 70% of all projects experience scope changes during their lifecycle.
Types of Changes
- Functional Changes: Alterations that affect how the software behaves or interacts with users.
- Non-Functional Changes: Adjustments related to performance, security, scalability, or reliability.
- Regulatory Changes: Modifications necessitated by compliance with laws or standards.
These changes can be managed but not without a thorough understanding of their implications.
Hidden Costs of Change
The hidden costs associated with changes in software development span several key areas:
1. Development Time
Changes in requirements inevitably lead to additional development time. Whether adding new features or modifying existing ones, developers need time to implement these changes correctly. This can slow the overall timeline of the project.
Example:
// Existing feature to calculate and display the user’s balance
public class Account {
private double balance;
public double getBalance() {
return balance;
}
}
// Change: need to include a method to calculate interest on the balance
public double calculateInterest(double rate) {
return balance * rate;
}
In the example above, the change to include interest calculation adds complexity to the Account
class, requiring developers to alter existing behavior and thoroughly test new logic.
2. Testing
Every change demands extensive testing to ensure that it functions as expected and does not inadvertently break existing features. This includes:
- Unit Testing: Verifying the change in isolation.
- Integration Testing: Ensuring the code works as part of the whole system.
- User Acceptance Testing: Getting feedback from end-users.
Testing consumes resources. If the change is substantial, QA teams may need to rerun entire test suites.
3. Documentation
Updated requirements necessitate changes in project documentation. This includes:
- Code comments
- API documentation
- User manuals
Neglecting documentation may lead to knowledge gaps for new team members or future developers maintaining the software.
4. Training and Support
Whenever new functionalities are added, training is often required for end-users and support teams. This entails:
- Developing training materials
- Conducting training sessions
- Offering ongoing support to troubleshoot user issues
This can stretch budgets and delay timelines further if not planned properly.
5. Technical Debt
Changes often introduce technical debt. This term refers to the implied cost of future rework caused by choosing the easy solution now instead of the better approach that would take longer.
For example, when hastily implementing a change, developers might write quick, unoptimized code that needs to be revisited later for cleanup. Ignoring this scenic route can lead to an unmanageable codebase, hindering future development efforts.
Example:
// Quick fix to display user information
public void displayUserInfo(User user) {
System.out.println("User Info: " + user.name + "; Age: " + user.age);
// No null-checks or error handling; risks NullPointerException
}
In the future, the above method could lead to runtime exceptions if the user
object isn't properly validated.
Strategies to Manage Hidden Costs
-
Agile Development Practices: Embrace Agile methodologies such as Scrum or Kanban, which facilitate iterative development. Regular reviews and feedback loops help accommodate change while managing scope effectively.
-
Version Control: Utilize systems like Git to manage code variations and rollback changes if necessary. This helps in tracing back modifications and understanding impacts.
-
Code Reviews: Establish a culture of peer review to spot potential issues early in the design phase. This practice can lead to improvements in coding standards and reduce the need for costly rework.
-
Documentation Standards: Ensure that documentation is an integral part of the development process. Adopting a "Documentation-First" mindset can significantly reduce future costs associated with onboarding and support.
-
Testing Automation: Invest in automated testing frameworks to run tests efficiently with every build. Tools like JUnit for Java can simplify writing and executing test cases.
Example of a Simple JUnit Test
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class AccountTest {
private Account account = new Account();
@Test
public void testCalculateInterest() {
account.setBalance(1000);
assertEquals(100.0, account.calculateInterest(0.1), 0.01); // Check interest calculation
}
}
By automating testing, you’re more likely to catch issues earlier, reducing problems associated with late-stage changes.
Lessons Learned
Understanding the hidden costs of software development changes is essential for adapting to the realities of the development process. By acknowledging these costs and implementing effective management strategies, teams can reduce the financial and temporal impact of changes.
In a landscape where software is frequently updated and expanded, being proactive about managing change leads to better outcomes, increased satisfaction among users, and, ultimately, a more successful product.
For those interested in agile practices to enhance their development process, check out Scrum Guide for more insights and best practices.
Additional Resources
- Continuous Integration and Continuous Deployment
- Understanding Agile
With an informed approach to managing changes, you can transform potential setbacks into opportunities for improvement. Let's embrace change as a stepping stone for innovation in the software development realm.
Checkout our other articles