Understanding When to Choose Code or Architecture Refactoring
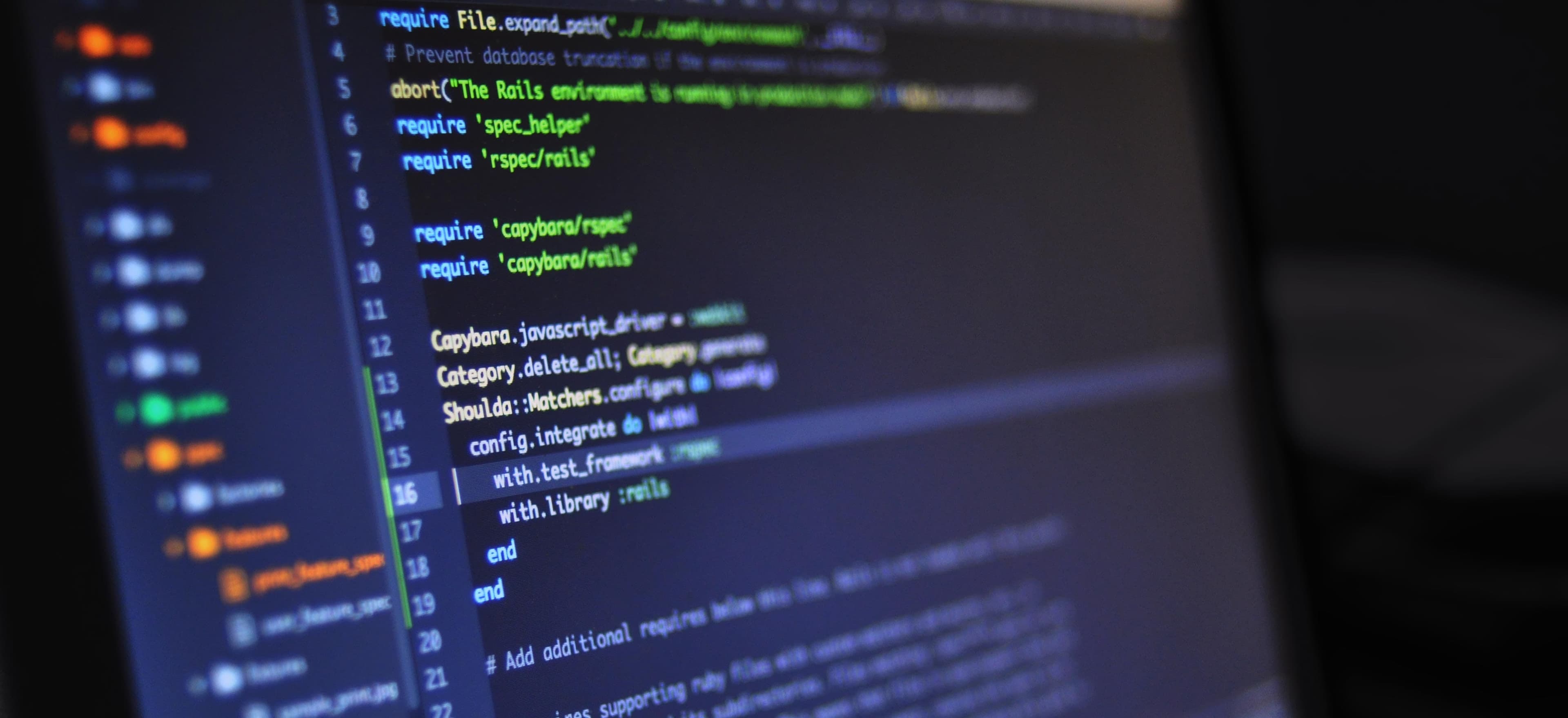
- Published on
Understanding When to Choose Code or Architecture Refactoring
Software development is an ever-evolving field. As projects grow, teams frequently face the dilemma of whether to refactor code or refactor the architecture of their applications. Understanding how and when to choose between these two vital practices can significantly affect the long-term maintainability and adaptability of software systems.
In this blog post, we will explore the definitions and differences between code refactoring and architecture refactoring. We will also provide insights into the scenarios when one may be preferred over the other, punctuated with practical examples.
What is Code Refactoring?
Code refactoring is the process of restructuring existing code without changing its external behavior. Its primary goal is to improve the non-functional attributes of the code, such as readability, maintainability, and performance.
Examples of Code Refactoring
Here is a simple Java snippet that demonstrates a code refactoring case. Imagine you have a method that calculates the area of a rectangle.
public double calculateArea(double width, double height) {
return width * height;
}
While this method is simple, it can benefit from clearer naming:
public double calculateRectangleArea(double width, double height) {
return width * height;
}
Why Refactor Code?
- Improve Readability: Using clear method names makes the code easier to read and understand.
- Enhance Maintainability: Organized and clean code is easier to maintain, enabling the team to onboard new developers smoothly.
- Increase Testability: Refactored code often adheres better to principles like Single Responsibility, making it easier to unit test.
What is Architecture Refactoring?
Architecture refactoring refers to the process of changing the overall structure and design of the software system. Unlike code refactoring, this can involve significant changes, impacting components, modules, and interactions between systems.
Examples of Architecture Refactoring
Consider a microservices architecture where services are tightly coupled. Refactoring towards a more decoupled architecture can improve scalability and resilience.
Imagine a scenario where you’re looking to separate your user authentication into its own microservice. This refactoring allows for individual scaling and focused development efforts:
// Old structure with tight coupling
public class UserService {
private final Database database; // Direct connection to DB
public UserService(Database database) {
this.database = database;
}
public void registerUser(User user) {
// Logic for registering user
}
}
// New structure separating auth with a microservice
public class AuthService {
public void authenticateUser(String username, String password) {
// Logic for authenticating user
}
}
public class UserService {
private final AuthService authService;
public UserService(AuthService authService) {
this.authService = authService;
}
public void registerUser(User user) {
// Logic for user registration
}
}
Why Refactor Architecture?
- Scalability: Decoupling services enables a system to scale more efficiently as loads change.
- Improved Fault Isolation: Issues in one microservice won't necessarily affect others, leading to better overall system reliability.
- Future Growth: A well-structured architecture allows for easier implementation of new features and integrations.
Determining When to Refactor Code or Architecture
Choosing between code refactoring and architecture refactoring depends on various factors. Here are some guidelines and situations that may lead you toward one or the other.
Indicators for Code Refactoring
-
Technical Debt: When a codebase is cluttered with "quick solutions," it is often time to refactor. If you notice repetitive patterns, unclear naming conventions, or duplicate logic, it is time to clean up the code.
-
Code Smells: Common signs include long methods, large classes, and excessive parameters. Refactoring can eliminate these smells, making the codebase cleaner.
-
Frequent Bugs: If the code is constantly breaking or producing unexpected results, it may signify that the complexity needs to be tackled through refactoring.
Indicators for Architecture Refactoring
-
Performance Bottlenecks: If your application is experiencing latency due to its architecture (i.e., all requests go through a monolith), it may require refactoring towards a more efficient design.
-
Tight Coupling: Applications with too much interdependence between components are difficult to modify. Refactoring the architecture can offer better modularity and decrease the coupling.
-
Rapidly Changing Business Needs: If your application or business model is evolving quickly, redesigning the architecture could provide the flexibility and responsiveness required.
Best Practices for Refactoring
Regardless of whether you opt for code or architecture refactoring, certain best practices should be followed:
Plan Carefully
Always identify the specific issues you are addressing. Have a clear goal for your refactoring.
Backward Compatibility
Make changes gradually and ensure that they don't impact existing functionality. This practice prevents disruptions for users and other dependent projects.
Tests, Tests, Tests
Implement unit tests before and after the refactoring process. This ensures that no feature is broken after your changes.
Document Changes
Keep documentation up to date so that your team knows the rationale behind changes. This documentation is invaluable for future developers encountering your code.
Wrapping Up
Refactoring is a critical component of maintaining and evolving software systems. Differentiating between code and architecture refactoring can help you address the right issues effectively.
When teams focus on code refactoring, they ensure the code is clean and maintainable. When they turn to architecture refactoring, they pave the way for sustainable growth in a changing business landscape.
By observing the indicators outlined in this article, developers and architects can make informed decisions that benefit both the development process and the end-users.
For more resources on software architecture and best practices, consider checking out Martin Fowler's website or the Microservices.io site by Chris Richardson, which offers a wealth of knowledge on architecture refactoring.
Happy coding!
Checkout our other articles