Why Selection Sort Fails with Large Datasets in Java
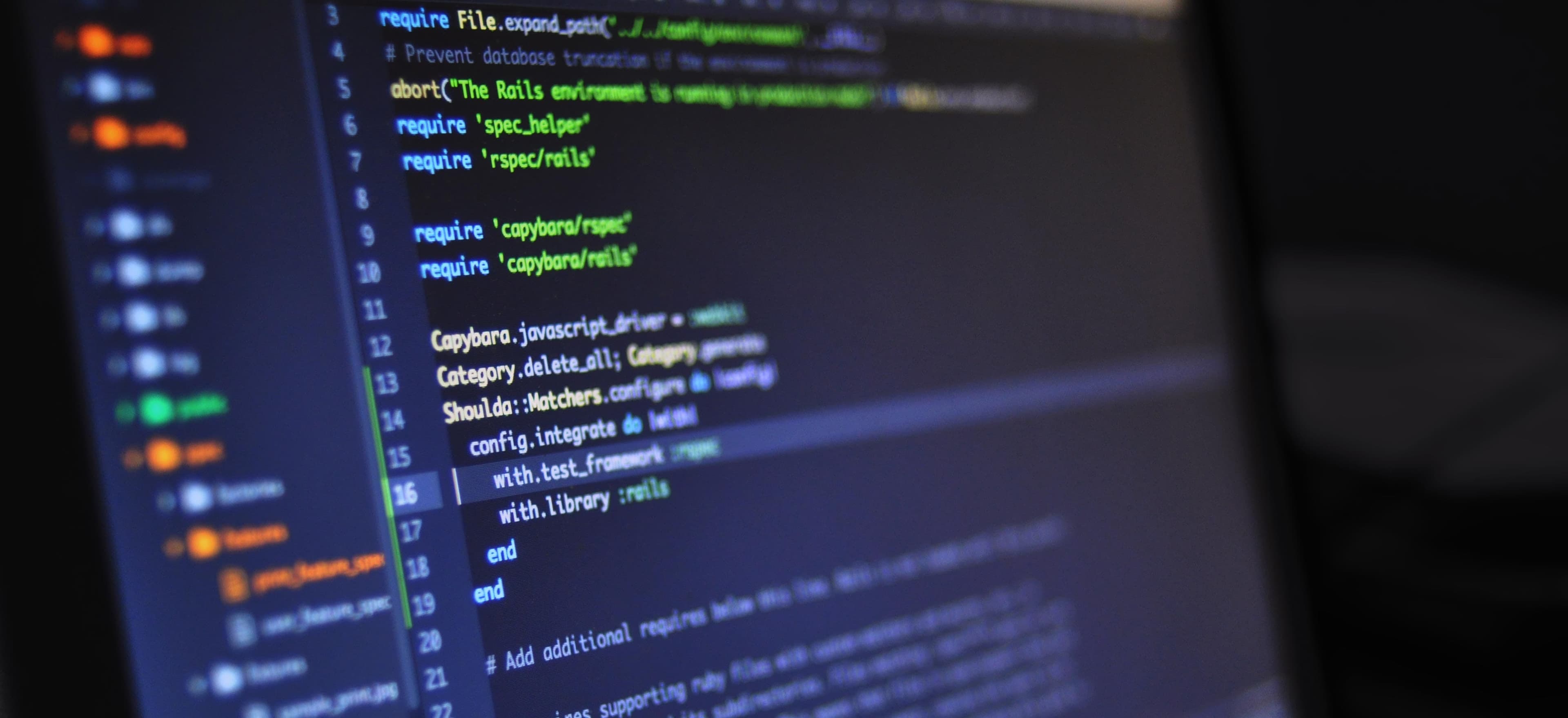
- Published on
Why Selection Sort Fails with Large Datasets in Java
When it comes to sorting algorithms, selection sort is often one of the first algorithms taught in computer science courses. Its implementation simplicity and ease of understanding make it a favored choice for beginners. However, as you dive deeper into sorting techniques, it becomes clear that selection sort is grossly inefficient for large datasets. In this post, we will discuss the mechanics of selection sort, provide Java implementation, and highlight why it falls short with larger datasets.
What is Selection Sort?
Selection sort is a comparison-based sorting algorithm that works by repeatedly selecting the smallest (or largest, depending on sorting order) element from an unsorted portion of the array and moving it to the beginning.
How Selection Sort Works
Here's a step-by-step breakdown of selection sort:
- Find the Minimum: Start at the first element, scan through the list to find the smallest value.
- Swap: Swap it with the value at the starting index.
- Repeat: Move the starting index one position to the right and repeat the process for the remaining unsorted portion of the list.
Selection Sort Implementation in Java
Below is a simple Java implementation of the selection sort algorithm:
public class SelectionSort {
public static void selectionSort(int[] array) {
int n = array.length;
// Traverse through all array elements
for (int i = 0; i < n - 1; i++) {
int minIndex = i; // Index of the minimum element
// Find the minimum element in the unsorted portion of the array
for (int j = i + 1; j < n; j++) {
if (array[j] < array[minIndex]) {
minIndex = j; // Update minIndex if a smaller element is found
}
}
// Swap the found minimum element with the first element
if (minIndex != i) {
swap(array, i, minIndex); // Only swap if necessary
}
}
}
// Method to swap two elements in the array
private static void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
// Helper method for displaying the array
public static void printArray(int[] array) {
for (int num : array) {
System.out.print(num + " ");
}
System.out.println();
}
public static void main(String[] args) {
int[] data = {64, 25, 12, 22, 11};
System.out.println("Original array:");
printArray(data);
selectionSort(data);
System.out.println("Sorted array:");
printArray(data);
}
}
Why Does Selection Sort Fail with Large Datasets?
The appeal of selection sort lies in its simplicity, but its performance drastically diminishes as the dataset size grows. Here's why:
1. Time Complexity
The time complexity of selection sort is O(n^2), where n is the number of elements in the array. This is because:
- The outer loop runs n times.
- The inner loop runs approximately n times for each iteration of the outer loop.
This results in a total of around n(n-1)/2 comparisons, which is clearly impractical for large datasets.
Comparison with Other Algorithms
For context, consider faster algorithms like Merge Sort or Quick Sort:
- Merge Sort: O(n log n)
- Quick Sort: Average O(n log n)
These algorithms generally have consistently better performance characteristics, especially as the dataset size increases.
2. Swaps
While the number of swaps in selection sort is fewer when compared to some other algorithms more than n swaps are still must be made. This is due to the number of comparisons made; each time a smaller index is found, a swap is executed. With larger arrays, swaps can eat into performance as well.
3. Poor Cache Performance
Selection sort exhibits poor cache performance due to its access pattern. Algorithms that work better with caching, such as Merge Sort and Heap Sort, are preferred for large datasets. These algorithms can efficiently access contiguous memory, reducing time spent accessing memory and improving performance.
4. Not Stable
Selection sort is not a stable sorting algorithm. This means that if two elements are equal, their original order may not be preserved in the sorted array. Stability can be crucial in cases where additional sorting order matters or when the data has secondary keys.
Sample Performance Analysis
Let’s run a simple experiment to measure the execution time of selection sort versus a more efficient sorting algorithm, such as Java's built-in Arrays.sort()
:
import java.util.Arrays;
import java.util.Random;
public class SortPerformanceTest {
public static void main(String[] args) {
Random rand = new Random();
int size = 10000; // Change this number to test with different sizes
int[] array = new int[size];
// Populate array with random integers
for (int i = 0; i < size; i++) {
array[i] = rand.nextInt(100000);
}
// Clone the original array for fair comparison
int[] arrayForSelectionSort = array.clone();
// Measure Selection Sort Time
long startTime = System.nanoTime();
SelectionSort.selectionSort(arrayForSelectionSort);
long endTime = System.nanoTime();
System.out.println("Selection Sort Time (ns): " + (endTime - startTime));
// Measure Arrays.sort() Time
long startTime2 = System.nanoTime();
Arrays.sort(array);
long endTime2 = System.nanoTime();
System.out.println("Arrays.sort Time (ns): " + (endTime2 - startTime2));
}
}
Final Thoughts
In conclusion, while selection sort has its educational benefits, it is fundamentally unfit for large datasets due to its O(n^2) time complexity, execution inefficiencies, poor cache utilization, and lack of stability. For larger datasets, you would be better off using algorithms designed for efficiency and performance.
If you're interested in diving deeper into sorting algorithms and improving your code performance, consider learning about more efficient algorithms like Quick Sort, Merge Sort, and Heap Sort. For an even more robust reference, check resources like GeeksforGeeks or dive into Java's Collections Framework for built-in options that are optimized for performance.
By understanding both the strengths and weaknesses of each sorting algorithm, you'll be better equipped to choose the right one for your particular use case. Happy coding!
Checkout our other articles