Selecting the Perfect UUID for Your Lucene Index
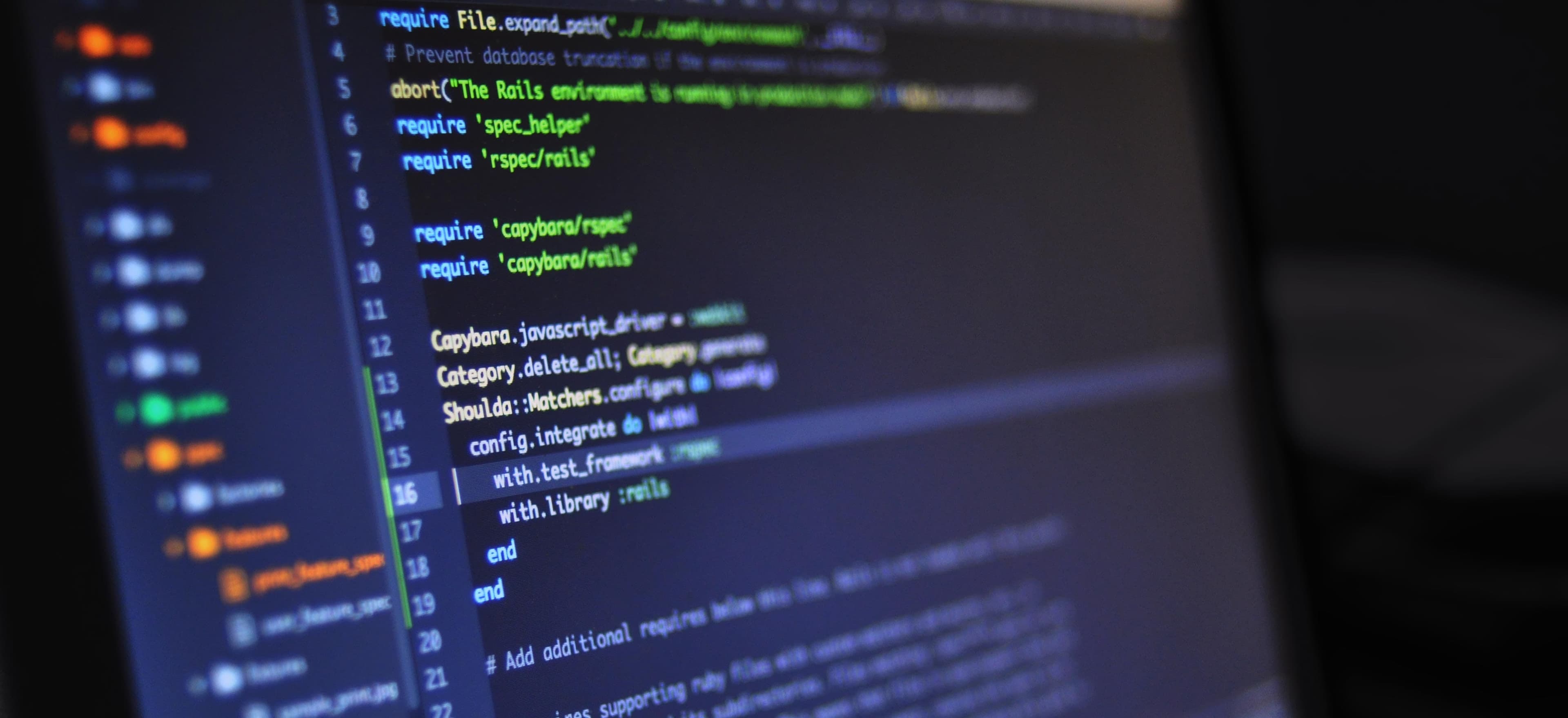
- Published on
Selecting the Perfect UUID for Your Lucene Index
In the world of software development, particularly when it comes to managing and organizing data, Unique Identifiers (UUIDs) play an integral role. They allow us to identify objects uniquely, preventing conflicts and ensuring consistency across different systems. When working with Lucene, a powerful search library in Java, the selection of a proper UUID for your index documents can significantly impact performance and data integrity. In this post, we'll explore the best practices for selecting UUIDs for your Lucene index, diving deep into the 'why' and 'how' with some illustrative code examples along the way.
What is a UUID?
A UUID, or Universally Unique Identifier, is a 128-bit label used for information in computer systems. It is intended to enable distributed systems to uniquely identify information without significant coordination. UUIDs are commonly represented in hexadecimal format and are usually generated according to specific algorithms. They are often favored over traditional integer-based keys, especially in distributed databases or systems like Lucene.
Advantages of Using UUIDs
- Uniqueness: Unlike auto-incremented integers, UUIDs are generated independently, which means they are unique across space and time.
- Distributed Systems: In microservices and distributed architectures, UUIDs prevent conflicts resulting from concurrent data generation.
- Non-Sequential: UUIDs are not predictable, providing an additional layer of abstraction.
- Scalability: UUIDs scale better when partitioning data across different nodes or services.
Choosing the Right UUID Version
UUIDs come in several versions, each with different characteristics. Understanding these can aid in your selection process.
- Version 1: Based on the time and the node (usually the MAC address). Great for traceability but not suitable for privacy.
- Version 3: Detereministic, generates a UUID from a namespace and a name using MD5 hashing.
- Version 4: Randomly generated. It’s generally the most commonly used type due to its straightforward implementation and low chance of collision.
- Version 5: Similar to version 3 but uses SHA-1 hashing instead.
For most applications, Version 4 provides a good balance between simplicity and uniqueness.
Implementing UUIDs in a Lucene Index
Integrating UUIDs into a Lucene index not only simplifies search and retrieval but also enhances data integrity across the indexing process. Here's an example of how to implement UUIDs within your Lucene indexing flow.
1. Add the UUID Field
You need to include the UUID in your Lucene Document. Here’s how to create a document with a UUID field:
import org.apache.lucene.document.Document;
import org.apache.lucene.document.StringField;
import org.apache.lucene.index.IndexWriter;
import java.util.UUID;
public void addDocument(IndexWriter indexWriter, String content) {
try {
// Generate a new random UUID
String uuid = UUID.randomUUID().toString();
// Create a new document
Document document = new Document();
document.add(new StringField("id", uuid, Field.Store.YES)); // Storing the UUID in the index
document.add(new StringField("content", content, Field.Store.YES));
// Write the document to the index
indexWriter.addDocument(document);
} catch (IOException e) {
e.printStackTrace();
}
}
Why Use StringField for UUID?
- Field.Store.YES: This means the UUID value will be stored and retrievable later.
- StringField: This is a suitable type since we are working with unique identifiers that do not require full-text searching.
Having a UUID helps in retrieving documents directly by their identifiers, ensuring that each document's identity is clear and intact.
2. Searching Documents by UUID
When it comes to searching documents, being able to find by UUID ensures quick access. Below's a simplified example of how to create a query based on a UUID.
import org.apache.lucene.index.IndexReader;
import org.apache.lucene.search.IndexSearcher;
import org.apache.lucene.search.Query;
import org.apache.lucene.search.TermQuery;
import org.apache.lucene.store.Directory;
import org.apache.lucene.store.RAMDirectory;
public Document getDocumentByUUID(IndexSearcher searcher, String uuid) {
try {
Query query = new TermQuery(new Term("id", uuid)); // Querying the UUID field
TopDocs results = searcher.search(query, 1); // Limit the search to 1 document
if (results.totalHits.value > 0) {
int docId = results.scoreDocs[0].doc;
return searcher.doc(docId);
}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
Why Is This Implementation Effective?
- Efficient Access: By using UUID as search criteria, you can directly retrieve documents without scanning the entire index, making it faster and less resource-consuming.
- Scalability: Even as the index grows, the UUID approach maintains efficiency.
Common Pitfalls to Avoid
While implementing UUIDs with Lucene, there are a few common mistakes developers make:
- Not Storing the UUID: Ensure you set
Field.Store.YES
to retrieve UUID after indexing. - Using Non-Random UUIDs for Security: If your application has privacy concerns, avoid using Version 1 UUIDs as they can expose the machine’s MAC address.
- Neglecting Exception Handling: Always consider IOException in your indexing and searching methods for robustness.
Final Thoughts
Selecting the perfect UUID for your Lucene index is crucial for maintaining data integrity and performance in distributed systems. The advantages of UUIDs outweigh the complexities involved, particularly when considering the scalability and uniqueness they offer.
By using the right version and appropriately implementing UUIDs in your code, you can enhance both the structure of your indexed data and the performance of your search operations.
For more insights into UUID usage in Java, feel free to check out this detailed guide on UUIDs.
Similarly, for an in-depth look at the Lucene library and its capabilities, refer to the official Apache Lucene documentation.
Remember, the wise use of UUIDs can transform the way your application handles data significantly. Happy coding!