Efficiently Scanning Large DynamoDB Tables with Java
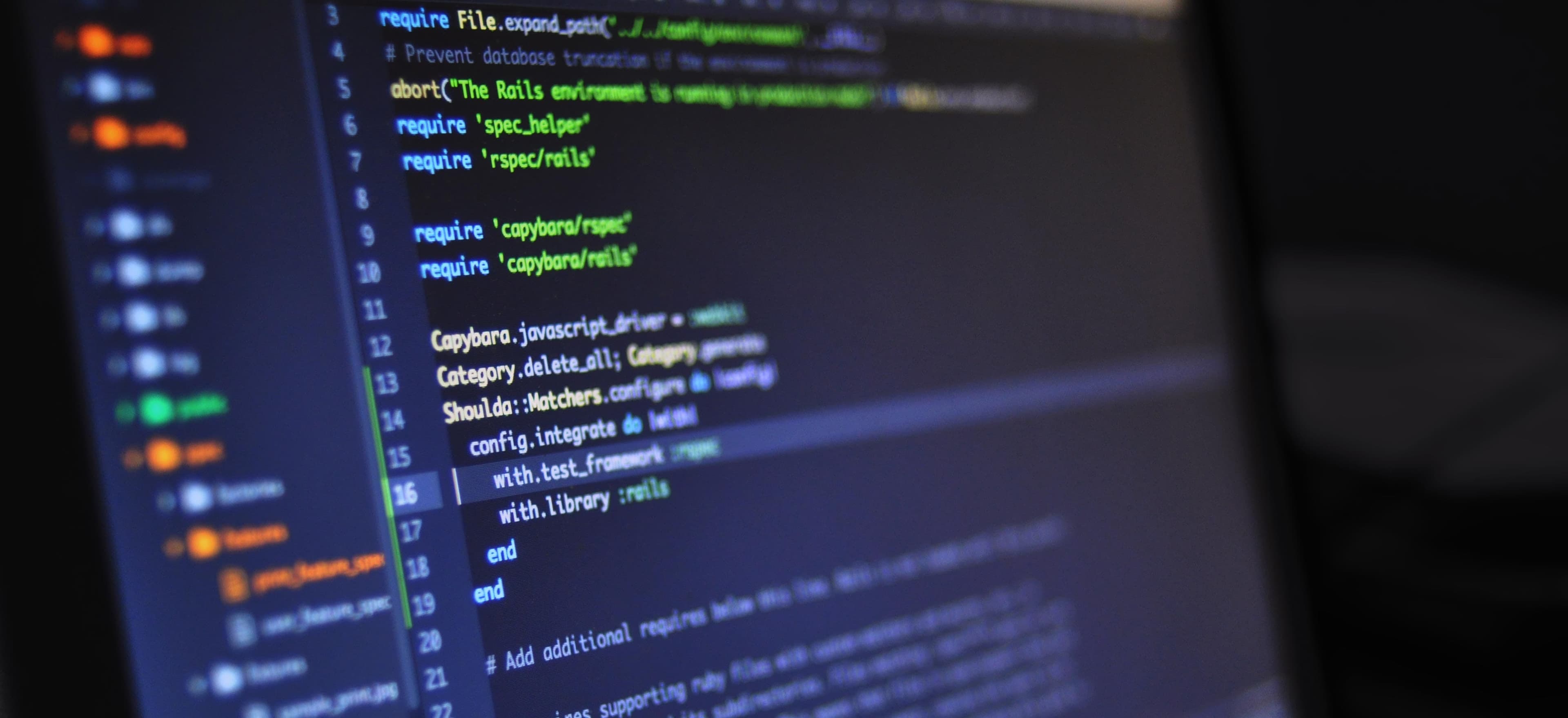
- Published on
Efficiently Scanning Large DynamoDB Tables with Java
Amazon DynamoDB is a highly scalable NoSQL database service that provides fast and predictable performance with seamless scalability. While it offers various access patterns, developers often face challenges when dealing with large tables, especially when it comes to efficient scanning.
In this blog post, we’ll explore how to efficiently scan large DynamoDB tables using Java. We will discuss the nuances of scanning, avoiding bottlenecks, and applying best practices to ensure optimal performance. We will also provide relevant code snippets and explanations to deepen your understanding.
Understanding DynamoDB Scans
Before diving into the implementation, let’s briefly discuss what scanning is in the context of DynamoDB. A scan operation examines every item in the table and returns all data attributes by default. This can lead to performance degradation if not managed properly, especially for tables with large volumes of data.
Key Considerations for Scanning
-
Read Capacity Units (RCUs): Scanning is expensive in terms of RCUs. Each item scanned consumes RCUs based on item size. Please refer to the AWS documentation on pricing for more insights.
-
Pagination: DynamoDB limits the number of items returned in a scan. By default, it returns up to 1 MB of data per request. Thus, handling pagination is crucial for large tables.
-
Filtering: Rather than retrieving all the data, applying filters to your scan can optimize performance, limiting the number of items processed.
Prerequisites
To get started, ensure you have the following:
- An AWS account with DynamoDB tables created.
- Java Development Kit (JDK) installed on your machine.
- AWS SDK for Java included in your project. If you are using Maven, add the following dependency:
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk-dynamodb</artifactId>
<version>1.12.269</version>
</dependency>
Setting Up the DynamoDB Client in Java
Before scanning data, let's set up a DynamoDB client instance. Here’s how to initialize the client:
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB;
import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder;
public class DynamoDBClient {
private final AmazonDynamoDB dynamoDB = AmazonDynamoDBClientBuilder.standard().build();
public AmazonDynamoDB getClient() {
return dynamoDB;
}
}
Explanation
- We are using the
AmazonDynamoDBClientBuilder
to configure our client. This method provides a simple yet effective way to connect to DynamoDB without any complicated configurations.
Efficient Scanning Implementation
Now let’s implement an efficient scan operation. Here’s a method that scans a DynamoDB table:
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB;
import com.amazonaws.services.dynamodbv2.document.DynamoDB;
import com.amazonaws.services.dynamodbv2.model.ScanRequest;
import com.amazonaws.services.dynamodbv2.model.ScanResult;
import java.util.HashMap;
import java.util.Map;
public class DynamoDBScan {
private final AmazonDynamoDB dynamoDB;
public DynamoDBScan(AmazonDynamoDB dynamoDB) {
this.dynamoDB = dynamoDB;
}
public void scanTable(String tableName) {
Map<String, AttributeValue> exclusiveStartKey = null;
boolean scannedEntireTable = false;
do {
ScanRequest scanRequest = new ScanRequest()
.withTableName(tableName)
.withExclusiveStartKey(exclusiveStartKey);
// Perform the scan operation
ScanResult scanResult = dynamoDB.scan(scanRequest);
// Process items
scanResult.getItems().forEach(item -> {
// Process each item here
System.out.println(item);
});
// Check if there are more items to be scanned
exclusiveStartKey = scanResult.getLastEvaluatedKey();
scannedEntireTable = exclusiveStartKey.isEmpty();
} while (!scannedEntireTable);
}
}
Explanation
- ScanRequest: This object is created with the specification of the table we want to scan.
- Pagination Handling: We utilize
exclusiveStartKey
to handle pagination by storing the last evaluated key returned by DynamoDB. - Scanning Loop: We scan in a loop until no further items are left to be processed.
Adding Filters to Your Scan
To further optimize the scan, we can apply filters that will reduce the number of items returned. Here's how you can modify the previous code to include a filter:
import com.amazonaws.services.dynamodbv2.model.ScanRequest;
import com.amazonaws.services.dynamodbv2.model.Condition;
import com.amazonaws.services.dynamodbv2.model.AttributeValue;
import com.amazonaws.services.dynamodbv2.model.ScanResult;
import java.util.HashMap;
import java.util.Map;
public class DynamoDBScanWithFilter {
private final AmazonDynamoDB dynamoDB;
public DynamoDBScanWithFilter(AmazonDynamoDB dynamoDB) {
this.dynamoDB = dynamoDB;
}
public void scanTableWithFilter(String tableName) {
Map<String, AttributeValue> exclusiveStartKey = null;
boolean scannedEntireTable = false;
do {
Map<String, Condition> scanFilter = new HashMap<>();
scanFilter.put("attributeName", new Condition().withComparisonOperator("CONTAINS")
.withAttributeValueList(new AttributeValue().withS("value")));
ScanRequest scanRequest = new ScanRequest()
.withTableName(tableName)
.withExclusiveStartKey(exclusiveStartKey)
.withScanFilter(scanFilter);
ScanResult scanResult = dynamoDB.scan(scanRequest);
// Process items
scanResult.getItems().forEach(item -> {
System.out.println(item);
});
exclusiveStartKey = scanResult.getLastEvaluatedKey();
scannedEntireTable = exclusiveStartKey.isEmpty();
} while (!scannedEntireTable);
}
}
Explanation
-
ScanFilter: By incorporating a scan filter, we can apply conditions for what should be included in the results. In this example, we check if an attribute contains a specific value.
-
This ultimately reduces the load on DynamoDB by avoiding unnecessary reads.
A Final Look
Scanning large DynamoDB tables can be daunting, but with proper strategies and code implementations, you can significantly enhance performance. We’ve covered the basics of scanning, handling pagination, and filtering items effectively.
For more complex query requirements, consider utilizing DynamoDB’s Query operation if you have a primary key or secondary index defined. This operation is typically faster and consumes fewer resources.
By following best practices, such as implementing efficient pagination and using filters, you’ll harness the full power of DynamoDB without incurring unnecessary costs.
Feel free to explore further on how to optimize your DynamoDB usage strategies through the AWS Developer Guide and unlock the full potential of this extraordinary NoSQL database!
Happy coding!