Breaking Down Dependency Inversion for Cleaner Code
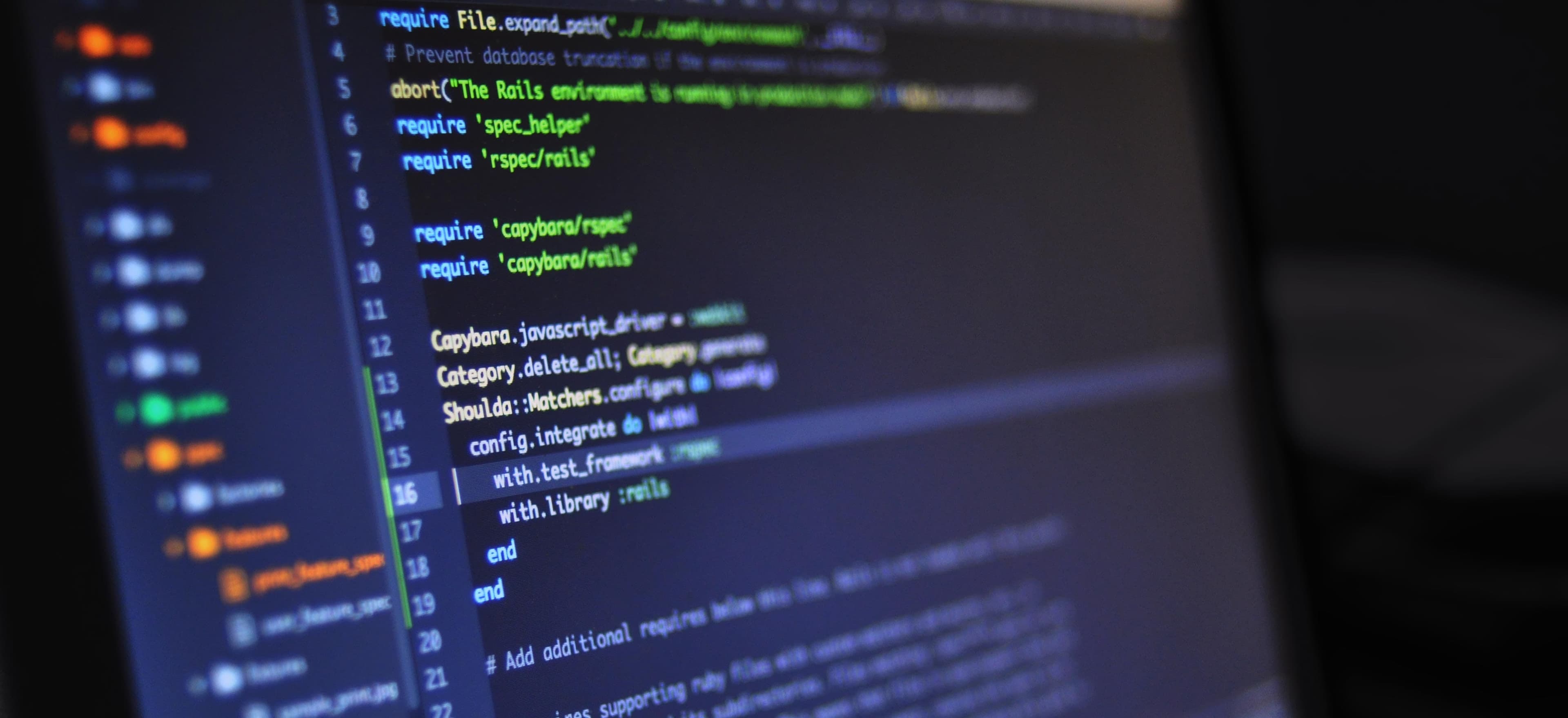
- Published on
Breaking Down Dependency Inversion for Cleaner Code
Stepping into the Topic
In the world of software development, code quality often determines the success and scalability of an application. One of the fundamental principles that help developers achieve cleaner, more maintainable code is the Dependency Inversion Principle (DIP), part of the SOLID principles introduced by Robert C. Martin. This article will delve into the nuances of the Dependency Inversion Principle, its significance, and how to effectively implement it in your Java projects.
What Is the Dependency Inversion Principle?
The Dependency Inversion Principle asserts that:
- High-level modules should not depend on low-level modules. Both should depend on abstractions.
- Abstractions should not depend on details. Details should depend on abstractions.
In simpler terms, this principle encourages developers to depend on interfaces or abstract classes rather than concrete implementations. This approach facilitates more flexible and reusable code architectures.
Why is Dependency Inversion Important?
Implementing Dependency Inversion leads to several advantages:
- Decoupled Code: High-level components remain unaffected by changes in low-level components, making the system easier to understand and modify.
- Enhanced Testability: Code is more testable since you can easily inject mock dependencies while writing unit tests.
- Improved Maintainability: As requirements change, you can swap implementations without extensive rewrites.
Understanding Dependency Inversion in Java
To see the Dependency Inversion Principle in action, let’s work through a practical example.
Suppose you have a simple application that sends notifications. In the initial implementation, you might code it like this:
// Low-level module
public class EmailService {
public void sendEmail(String message) {
// Logic to send email
System.out.println("Sending email with message: " + message);
}
}
// High-level module
public class Notification {
private EmailService emailService;
public Notification() {
this.emailService = new EmailService();
}
public void notifyUser(String message) {
emailService.sendEmail(message);
}
}
Analyzing the Code
In this example, the Notification
class directly depends on the EmailService
class. If we decide to add SMS notifications or change our email service, we must modify the Notification
class, which violates the Dependency Inversion Principle.
Refactoring for Dependency Inversion
Now, let's refactor this code to comply with the Dependency Inversion Principle.
Step 1: Create an Interface
First, we create an interface that abstracts the notification logic:
// Abstraction
public interface NotificationService {
void sendNotification(String message);
}
Step 2: Implement the Interface in Concrete Classes
Next, we implement this interface in the concrete classes:
// Low-level module
public class EmailService implements NotificationService {
public void sendNotification(String message) {
System.out.println("Sending email with message: " + message);
}
}
// Another Low-level module
public class SMSService implements NotificationService {
public void sendNotification(String message) {
System.out.println("Sending SMS with message: " + message);
}
}
Step 3: Refactor the High-Level Module
Now we change the Notification
class to depend on the NotificationService
abstraction rather than a specific implementation:
// High-level module
public class Notification {
private NotificationService notificationService;
// Constructor injection for better flexibility
public Notification(NotificationService notificationService) {
this.notificationService = notificationService;
}
public void notifyUser(String message) {
notificationService.sendNotification(message);
}
}
Step 4: Dependency Injection
This is the crux of Dependency Inversion—using Dependency Injection (DI). We can create instances and inject our service:
public class Main {
public static void main(String[] args) {
NotificationService emailService = new EmailService();
Notification emailNotification = new Notification(emailService);
emailNotification.notifyUser("Hello via Email!");
NotificationService smsService = new SMSService();
Notification smsNotification = new Notification(smsService);
smsNotification.notifyUser("Hello via SMS!");
}
}
Explanation of Design Choices
1. Interface Definition: We defined an interface NotificationService
to represent the duty of sending notifications. This helps adhere to the Dependency Inversion Principle.
2. Concrete Implementations: Both EmailService
and SMSService
implement the NotificationService
, allowing the high-level class to work with any notification type.
3. Constructor Injection: This allows for an easy swap of the notification method without changing the Notification
class. Such flexibility is essential for maintaining a clean architecture.
Benefits Realized
By applying the Dependency Inversion Principle, you gain an architecture that can easily adapt to changes. Tomorrow, if you need a different notification method, such as push notifications, you can implement it easily:
// New Low-level module
public class PushNotificationService implements NotificationService {
public void sendNotification(String message) {
System.out.println("Sending push notification with message: " + message);
}
}
Then, simply replace the service used in the Main
class without altering the Notification
class.
Wrapping Up
The Dependency Inversion Principle significantly enhances the maintainability, testability, and scalability of your software applications. By decoupling high-level components from low-level details through the use of abstractions and interfaces, you build a robust framework that can easily adapt to changing requirements.
For more detailed explorations of these principles, consider checking SOLID Principles in Java and how Dependency Injection frameworks such as Spring can automate and standardize these practices.
Implementing the Dependency Inversion Principle is vital for anyone aiming to write clean and efficient Java code. The principle goes beyond mere compliance, fostering a mindset of flexibility and adaptability that is essential for 21st-century programming.
As you grow in your understanding of these concepts, remember that cleaner code isn't just about following rules—it's about cultivating best practices to enhance your development process and maintain the integrity of your applications over time.