Troubleshooting Thread Starvation in Java Concurrency
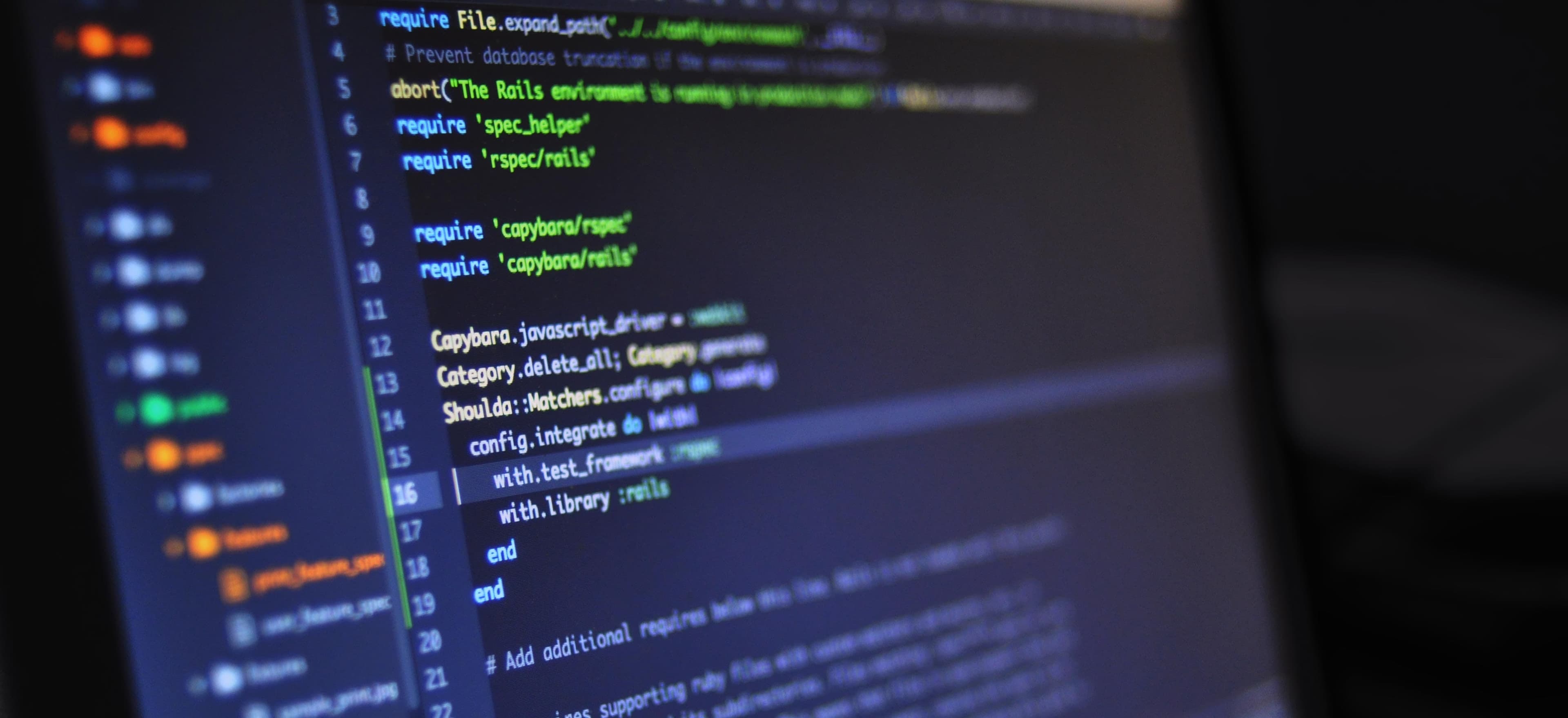
- Published on
Troubleshooting Thread Starvation in Java Concurrency
Thread starvation is a common issue in concurrent programming, especially when dealing with multi-threaded Java applications. It occurs when a thread is unable to gain access to the system resources it needs to execute, often due to other threads monopolizing those resources. This can lead to performance degradation and even deadlock scenarios if left unaddressed. In this article, we will explore the concept of thread starvation in Java concurrency, discuss its common causes, and provide strategies for troubleshooting and mitigating this issue.
Understanding Thread Starvation
In Java concurrency, thread starvation occurs when a thread is unable to make progress because other threads are continuously taking precedence over it in accessing shared resources such as CPU, memory, or locks. This can happen due to poor resource management, inefficient thread scheduling, or contention for shared resources.
Thread starvation can manifest in various ways, including:
- Unresponsive or slow performance of a multi-threaded application
- Increased contention for synchronized resources
- Deadlock situations where threads are waiting indefinitely for access to resources
Causes of Thread Starvation
Thread starvation can be caused by a variety of factors, some of which include:
- Heavy contention: When multiple threads compete for a limited set of resources, it can lead to one or more threads being starved of those resources.
- Inefficient scheduling: The JVM's thread scheduler may not allocate CPU time fairly among threads, causing some threads to be starved of processing time.
- Unfair locking: The use of unfair locking mechanisms can lead to certain threads being continually denied access to critical sections of code.
- Blocking operations: Long-running or blocking operations within a thread can hinder the progress of other threads, resulting in starvation.
Identifying Thread Starvation
Detecting thread starvation can be challenging, as it may not always manifest as a clear error or exception. However, there are several indicators that can point to the presence of thread starvation in a Java application, such as:
- High CPU usage with low throughput
- Thread contention or deadlock warnings in application logs
- Unresponsive behavior or slow performance under concurrent load
Troubleshooting Thread Starvation
When troubleshooting thread starvation in a Java application, it is essential to employ various techniques to identify and address the root causes. Here are some strategies for troubleshooting thread starvation:
1. Thread Dump Analysis
Analyzing thread dumps can provide valuable insights into the state of threads within the application. Tools like jstack or visualVM can be used to capture and analyze thread dumps, helping to identify threads that are blocked or waiting for resources. By examining thread states and stack traces, you can pinpoint potential areas of contention or resource starvation.
2. Code Profiling
Using a Java profiler such as YourKit or Java Mission Control can help identify hotspots in the code where threads are contending for resources. Profiling the application under load can reveal inefficient synchronization, long-running operations, or bottlenecked resources that contribute to thread starvation.
3. Resource Pooling and Management
Implementing resource pooling strategies, such as connection pooling for database access or object pooling for expensive resources, can help alleviate contention and reduce the likelihood of thread starvation. By efficiently managing shared resources, you can ensure fair access for all threads and mitigate the impact of contention.
4. Optimizing Thread Synchronization
Reviewing the use of locks, synchronized blocks, and concurrent data structures in the code can uncover opportunities for improving thread synchronization. Using finer-grained locks, employing non-blocking algorithms, or re-evaluating the use of synchronized blocks can help reduce contention and minimize the risk of thread starvation.
5. Throttling and Backoff Mechanisms
Introducing throttling and backoff mechanisms in the application can help regulate the rate at which threads access resources. This can prevent resource exhaustion and alleviate contention, improving the overall fairness of resource allocation among threads.
Mitigating Thread Starvation
After identifying the root causes of thread starvation, it is crucial to implement strategies to mitigate this issue in Java concurrent applications. Some best practices for mitigating thread starvation include:
- Optimizing resource utilization: Ensuring efficient utilization of resources through proper resource management and allocation.
- Fair scheduling: Configuring the JVM's thread scheduler for fair allocation of CPU time among threads.
- Reducing contention: Minimizing contention for shared resources through efficient locking and synchronization mechanisms.
- Monitoring and alerting: Implementing monitoring tools and alerts to detect and address thread starvation proactively.
By addressing these areas, you can minimize the impact of thread starvation on the performance and reliability of your Java concurrent applications.
My Closing Thoughts on the Matter
Thread starvation poses a significant challenge in Java concurrent programming, leading to performance degradation and potential deadlock scenarios. By understanding the causes of thread starvation and employing effective troubleshooting and mitigation strategies, developers can ensure the smooth operation of multi-threaded Java applications. Through careful analysis, optimization, and proactive management of shared resources, thread starvation can be mitigated, resulting in improved application performance and reliability.
In conclusion, thread starvation is a common issue in Java concurrency, but with proper understanding and proactive measures, it can be effectively addressed and mitigated for robust and efficient multi-threaded applications.
Remember, identifying, troubleshooting, and mitigating thread starvation requires a deep understanding of Java concurrency and the tools and techniques available for analyzing and optimizing concurrent applications. By adhering to best practices and continuously monitoring and improving the performance of concurrent code, developers can minimize the impact of thread starvation and ensure the reliability and responsiveness of their Java applications.
By adopting a proactive approach to thread starvation, Java developers can build resilient and high-performance concurrent applications that deliver consistent and scalable performance under varying workloads.
For more in-depth understanding of Java concurrency and performance optimization, consider exploring Java Concurrency in Practice, a comprehensive guide to writing concurrent programs in Java.
As you continue to explore the intricacies of Java concurrency, remember that thread starvation is just one of the many challenges inherent in multi-threaded programming. With careful analysis, strategic optimization, and continuous improvement, you can build robust and efficient concurrent applications that fully leverage the power of Java's multi-threading capabilities.
Happy coding!