Troubleshooting Common Issues with Hibernate Mapped Collections
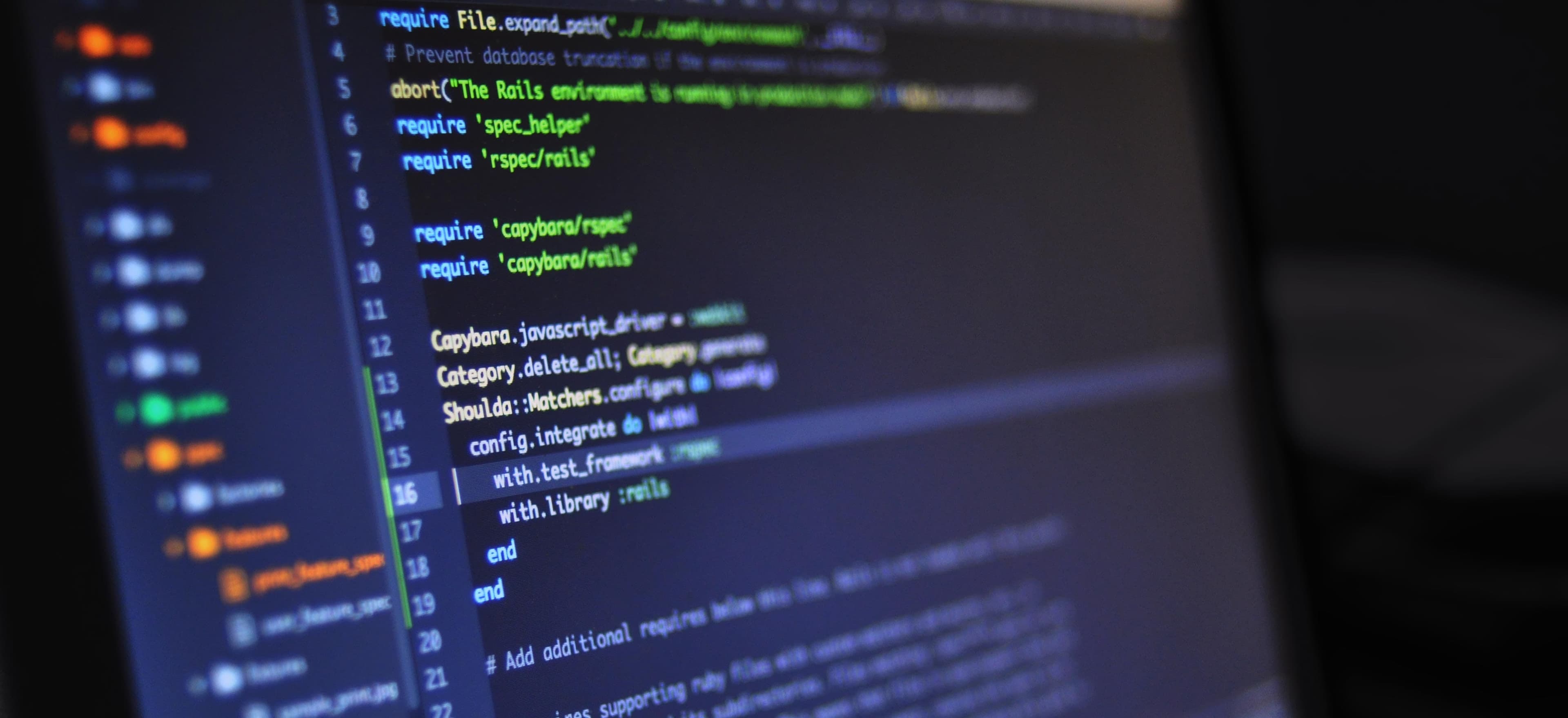
- Published on
Troubleshooting Common Issues with Hibernate Mapped Collections
Hibernate is a popular ORM (Object-Relational Mapping) framework for Java. It allows developers to interact with relational databases in a more object-oriented manner. One of its powerful features, mapped collections, provides an elegant solution for managing collections of entities. However, beginners and even experienced developers may encounter some common issues while using mapped collections. In this article, we will address these issues and provide practical solutions to troubleshoot them effectively.
Understanding Hibernate Mapped Collections
Before diving into troubleshooting, let's clarify what mapped collections are. Generally, they allow you to map a collection of elements to a database table. For example, if you have a User
entity that has a collection of PhoneNumber
entities, you can use a mapped collection to store and manage these numbers effectively.
Here's an example of how to define a one-to-many relationship using Hibernate:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ElementCollection
@CollectionTable(name = "user_phone_numbers", joinColumns = @JoinColumn(name = "user_id"))
@Column(name = "phone_number")
private Set<String> phoneNumbers = new HashSet<>();
// getters and setters
}
In this snippet, the User
class contains a set of phone numbers mapped to a new table called user_phone_numbers
. This is a simple example, but it highlights the foundational concept of mapped collections.
Common Issues with Mapped Collections
Issue 1: LazyInitializationException
One of the most common problems is the LazyInitializationException
. This occurs when you attempt to access a lazily-loaded collection outside of an active Hibernate session.
Solution
To solve this, ensure that the collection is initialized while the session is active, or you can use the FetchType.EAGER
strategy.
@OneToMany(fetch = FetchType.EAGER)
private Set<PhoneNumber> phoneNumbers = new HashSet<>();
Using FetchType.EAGER
retrieves the collection immediately with the parent entity but may impact performance with larger datasets.
Issue 2: Duplicate Values in Collections
Due to the nature of how collections are managed, developers sometimes experience unexpected duplicates when saving entities.
Solution
Ensure the collection type is appropriate. For unique entries, you should use a Set
instead of a List
. The Set
interface enforces uniqueness, causing Hibernate to manage duplicates correctly.
@ElementCollection
@CollectionTable(name = "user_phone_numbers")
private Set<String> phoneNumbers = new HashSet<>();
Issue 3: Unidirectional vs. Bidirectional Relationships
Sometimes, developers make design decisions about the relationship that can lead to complications. A unidirectional relationship may lead to issues when trying to save related entities.
Solution
If an entity requires navigation in both directions, consider using a bidirectional relationship.
@Entity
public class PhoneNumber {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String number;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
// getters and setters
}
By having both sides represented in your model, you can maintain integrity more easily during CRUD operations.
Issue 4: Hibernate not Generating the Collection Table
Another issue encountered is the absence of the collection table being generated in the database.
Solution
Ensure that your annotated classes are scanned by Hibernate. If using Spring Boot, ensure that the @EntityScan
annotation includes your package:
@SpringBootApplication
@EntityScan(basePackages = "com.example.models")
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Additionally, ensure that the hibernate.hbm2ddl.auto
property is set to update
or create
.
Issue 5: Not Persisting or Updating Collections
If you are facing issues where changes to collections are not being persisted into the database, ensure that the collections are properly managed.
Solution
Always maintain the entity's lifecycle management. When dealing with collections, add or remove elements from the collection, rather than creating new instances. For example:
public void addPhoneNumber(User user, String phoneNumber) {
user.getPhoneNumbers().add(phoneNumber);
// Ensure the user object is managed by the context and flush changes
session.saveOrUpdate(user);
}
Using user.getPhoneNumbers().add(phoneNumber);
ensures that Hibernate tracks the change accurately.
Tips for Debugging Hibernate Mapped Collections
- Check Hibernate SQL Logs: Enable SQL logging to see the executed queries that Hibernate generates. This can be done in the Hibernate properties:
hibernate.show_sql=true
hibernate.format_sql=true
-
Use Debugging Tools: Modern IDEs provide features to debug Hibernate sessions. Use breakpoints and inspect the session, objects, and collections during runtime.
-
Understand Cascade Types: Familiarize yourself with the different cascade types (CascadeType.ALL, PERSIST, MERGE, REMOVE, etc.) as they dictate how operations propagate to child entities.
-
Review Collection Types: Select the correct collection type (Set, List, Map) based on your use case to avoid issues related to duplicates or ordering.
Key Takeaways
Hibernate mapped collections are a robust way to manage relationships between entities efficiently. However, as outlined in this article, common problems can arise. By applying these solutions and tips, you can troubleshoot issues effectively.
For further reading on managing Hibernate collections, check out the Hibernate Documentation or Baeldung's Guide to Hibernate for an in-depth look at collections in Hibernate.
Solid understanding and proper implementation of mapped collections can significantly improve the data access layer of your Java applications. Happy coding!