Common Pitfalls in Implementing Vigenere Cipher in Java
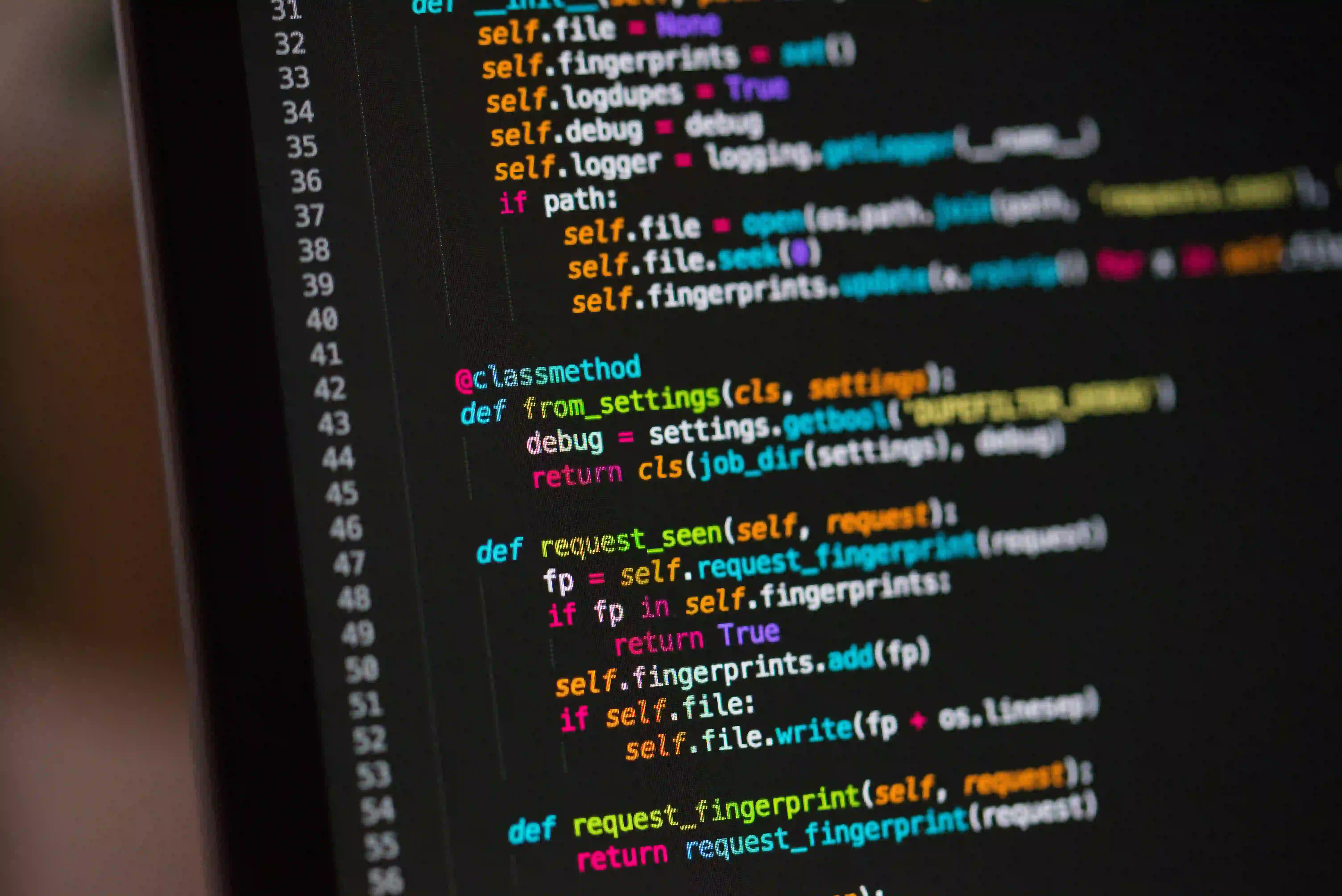
Common Pitfalls in Implementing Vigenère Cipher in Java
The Vigenère cipher is a classic encryption technique that has fascinated cryptographers and hobbyists alike for centuries. Unlike the simple Caesar cipher, the Vigenère cipher uses a keyword to enhance security, making it more challenging to crack. However, implementing this cipher in Java can introduce a host of common pitfalls, especially for those less experienced. In this article, we will discuss these pitfalls and provide clean Java code examples, along with clear commentary to guide you through the correct implementation of the Vigenère cipher.
Understanding the Vigenère Cipher
Before we dive into the pitfalls, let's briefly outline how the Vigenère cipher works:
- Keyword: A keyword is chosen, which repeats itself to match the length of the plain text.
- Character Shifting: Each character in the plain text is shifted by an amount determined by the corresponding character in the keyword.
- Encryption: For a given character in the plain text
P
and a corresponding character in the keywordK
, the encrypted characterC
is calculated as follows:📄snippet.txtC = (P + K) mod 26
This results in a stronger form of encryption that can withstand frequency analysis.
Key Pitfall #1: Ignoring Non-Alphabetic Characters
Problem
One of the most common mistakes is to create an implementation that does not handle non-alphabetic characters. In many cryptographic applications, it is essential to leave spaces and punctuation unchanged.
Solution
We need to modify our encryption and decryption methods to skip non-alphabetic characters. Here's how you might do it:
public static String encrypt(String plainText, String keyword) {
StringBuilder encryptedText = new StringBuilder();
int keywordIndex = 0;
for (int i = 0; i < plainText.length(); i++) {
char currentChar = plainText.charAt(i);
// Check if the character is an alphabet
if (Character.isLetter(currentChar)) {
char keywordChar = keyword.charAt(keywordIndex % keyword.length());
int shift = Character.toLowerCase(keywordChar) - 'a';
char encryptedChar = (char) (((Character.toLowerCase(currentChar) - 'a' + shift) % 26) + 'a');
encryptedText.append(Character.isUpperCase(currentChar) ? Character.toUpperCase(encryptedChar) : encryptedChar);
keywordIndex++;
} else {
encryptedText.append(currentChar); // Append non-alphabetical characters unchanged
}
}
return encryptedText.toString();
}
Commentary
In this implementation, we:
- Check if the character is an alphabet before applying any shifts.
- Use the
Character.isLetter()
method to determine whether to encrypt or skip the character. - Preserve the case of the original character using a simple check with
Character.isUpperCase()
.
Key Pitfall #2: Keyword Length Management
Problem
Another common issue arises when the keyword is shorter than the plain text. New developers might forget to repeat the keyword appropriately, leading to index out of bounds or incorrect encryption.
Solution
The keyword should repeat itself to match the length of the plain text during processing. This was addressed in the code above by using the modulus operator %
to cycle through the keyword.
A Related Link
For a more in-depth understanding of string handling in Java, Java Strings - Null Pointer Exception provides a solid foundation.
Key Pitfall #3: Not Implementing Decryption
Problem
Sometimes, developers only implement encryption, forgetting that decryption is also necessary for a fully functional cipher.
Solution
Decryption follows a similar logic, but instead of shifting to the right, we shift to the left. Here's how to implement the decryption method:
public static String decrypt(String encryptedText, String keyword) {
StringBuilder decryptedText = new StringBuilder();
int keywordIndex = 0;
for (int i = 0; i < encryptedText.length(); i++) {
char currentChar = encryptedText.charAt(i);
if (Character.isLetter(currentChar)) {
char keywordChar = keyword.charAt(keywordIndex % keyword.length());
int shift = Character.toLowerCase(keywordChar) - 'a';
char decryptedChar = (char) (((Character.toLowerCase(currentChar) - 'a' - shift + 26) % 26) + 'a');
decryptedText.append(Character.isUpperCase(currentChar) ? Character.toUpperCase(decryptedChar) : decryptedChar);
keywordIndex++;
} else {
decryptedText.append(currentChar); // Append non-alphabetic characters unchanged
}
}
return decryptedText.toString();
}
Commentary
In the decryption code:
- We subtract
shift
instead of adding it, effectively reversing the encryption process. - The addition of 26 in the shift calculation ensures that the result remains positive, preventing negative indexes during the modulus operation.
Key Pitfall #4: Case Sensitivity
Problem
Some implementations fail to handle uppercase and lowercase letters properly, leading to unexpected outcomes in the ciphertext.
Solution
Always account for character cases. The previous implementation adequately handles this, but it's vital to emphasize maintaining the case throughout encryption and decryption.
Final Thoughts
The Vigenère cipher serves as an intriguing study into the world of classical cryptography. By avoiding these common pitfalls — failing to handle non-alphabetic characters, mismanaging keyword length, neglecting decryption, and not addressing case sensitivity — you can create a robust implementation in Java.
A Final Look
When correctly implemented, the Vigenère cipher not only secures messages but also enhances your understanding of cryptographic concepts. Remember to thoroughly test your code for edge cases, and don't shy away from leveraging resources like Oracle's Java Tutorials for further guidance.
As you experiment and iterate on your implementation, consider sharing your findings with the community. Understanding both the intricacies of this cipher and the pitfalls in its implementation can lead to a deeper appreciation of computer science and cryptography. Happy coding!