Overcoming Command Pattern Complexity in Software Design
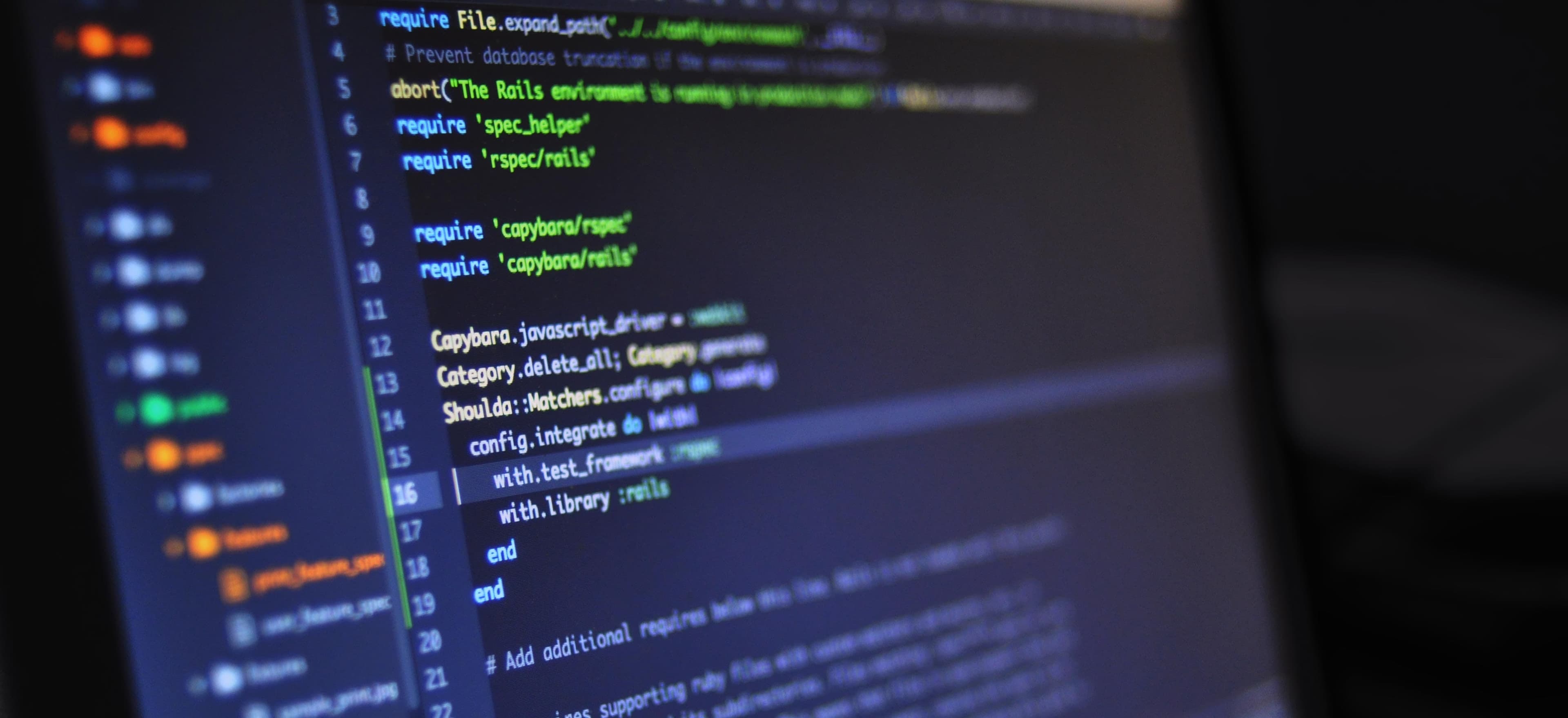
- Published on
Overcoming Command Pattern Complexity in Software Design
When dealing with complex software systems, maintaining clarity and functionality is crucial. One common design pattern that often leads to complexity, yet offers tremendous benefits when appropriately applied, is the Command Pattern. In this blog post, we will explore what the Command Pattern is, its advantages and disadvantages, and how to overcome the complexity associated with using it. We'll also delve into practical examples and code snippets in Java to illustrate our points effectively.
What is the Command Pattern?
The Command Pattern is a behavioral design pattern that encapsulates a request as an object. It allows you to parameterize clients with queues, requests, and operations—effectively decoupling the sender from the receiver of the request.
Why Use the Command Pattern?
-
Decouples Sender and Receiver: The sender of a command does not need to know the specifics of how the command is handled or executed. This reduces coupling and increases flexibility.
-
Support for Undoable Operations: Encapsulating requests as objects allows you to implement features like undo and redo by maintaining a history of executed commands.
-
Queuing of Requests: Command objects can be stored in data structures like queues, making it easy to execute them later, facilitating asynchronous operations.
-
Composite Commands: You can aggregate multiple commands into a single command, allowing complex operations to be represented simply.
Advantages of the Command Pattern
Utilizing the Command Pattern offers several advantages:
- Flexibility: New commands can be added easily without altering existing code.
- Simplicity: By breaking down operations into separate objects, maintenance becomes more manageable.
- Composability: Commands can be composed into larger commands, promoting code reuse.
Disadvantages of the Command Pattern
However, there are pitfalls:
- Increased Complexity: The initial setup can be more complex due to the number of classes created for each command.
- Overuse: Not every operation requires this level of abstraction. Overusing the Command Pattern can lead to unnecessary complexity.
Overcoming Complexity
To effectively use the Command Pattern while mitigating complexity, here are strategies you can apply:
-
Limit Command Object Creation: Only create command objects that bring true benefits to your application. For instance, don’t create a separate command for trivial operations.
-
Use Command Factories: Create a command factory that generates command objects dynamically based on input parameters. This reduces clutter and centralizes command creation.
-
Parameter Defaults: Where applicable, leverage default parameters within command objects to minimize the need for multiple command variations.
-
Clear Naming Conventions: Use meaningful names for command classes to clarify their intentions and functionalities, thus making the code easier to read.
-
Unit Testing: Ensure that your command objects are well-tested. This can help catch issues arising from complex interactions.
Implementation Example
Let's delve into some Java code that demonstrates the Command Pattern within a simple text editor application.
Step 1: Command Interface
First, we define a command interface. This is foundational, as all commands will implement this interface.
public interface Command {
void execute();
void undo();
}
Step 2: Concrete Command Classes
Next, we'll create a concrete command for adding text to a document:
public class AddTextCommand implements Command {
private final Document document;
private final String text;
public AddTextCommand(Document document, String text) {
this.document = document;
this.text = text;
}
@Override
public void execute() {
document.addText(text); // Add the text to the document
}
@Override
public void undo() {
document.removeText(text.length()); // Remove the text by length
}
}
Step 3: The Receiver Class
The receiver in this example is the Document
class, which performs the actual operations:
public class Document {
private StringBuilder content = new StringBuilder();
public void addText(String text) {
content.append(text);
}
public void removeText(int length) {
if (length <= content.length()) {
content.delete(content.length() - length, content.length());
}
}
public String getContent() {
return content.toString();
}
}
Step 4: Invoker Class
Now we need a class to invoke the commands:
import java.util.Stack;
public class TextEditor {
private Stack<Command> commandHistory = new Stack<>();
public void executeCommand(Command command) {
command.execute();
commandHistory.push(command);
}
public void undoCommand() {
if (!commandHistory.isEmpty()) {
Command command = commandHistory.pop();
command.undo();
}
}
public void printContent(Document document) {
System.out.println(document.getContent());
}
}
Step 5: Putting It All Together
Finally, we can utilize these classes in our main program:
public class Main {
public static void main(String[] args) {
Document document = new Document();
TextEditor editor = new TextEditor();
// Adding text
Command addHello = new AddTextCommand(document, "Hello,");
editor.executeCommand(addHello);
Command addWorld = new AddTextCommand(document, " World!");
editor.executeCommand(addWorld);
// Print current document content
editor.printContent(document);
// Undo last command (removing " World!")
editor.undoCommand();
editor.printContent(document);
}
}
Explanation of Code
In this example, we defined a Command
interface with execute
and undo
methods. The AddTextCommand
class implements this interface, allowing users to add text to a Document
and also undo that action.
The TextEditor
acts as an invoker, maintaining the history of commands to enable undo operations. Users can add text, and can also roll back changes easily.
Wrapping Up
The Command Pattern encapsulates actions as objects, providing a lot of flexibility in software design. By understanding its advantages and disadvantages, and implementing strategies to overcome its complexities, this pattern can be a powerful tool in your programming toolbox.
By observing clear naming conventions, limiting command creation, and doing thorough unit testing, developers can streamline operations and make software easier to maintain.
For further information on other design patterns in Java, consider checking out this resource on Java Design Patterns which offers comprehensive insights and examples.
Happy coding!