Common Spring MVC Errors and How to Fix Them
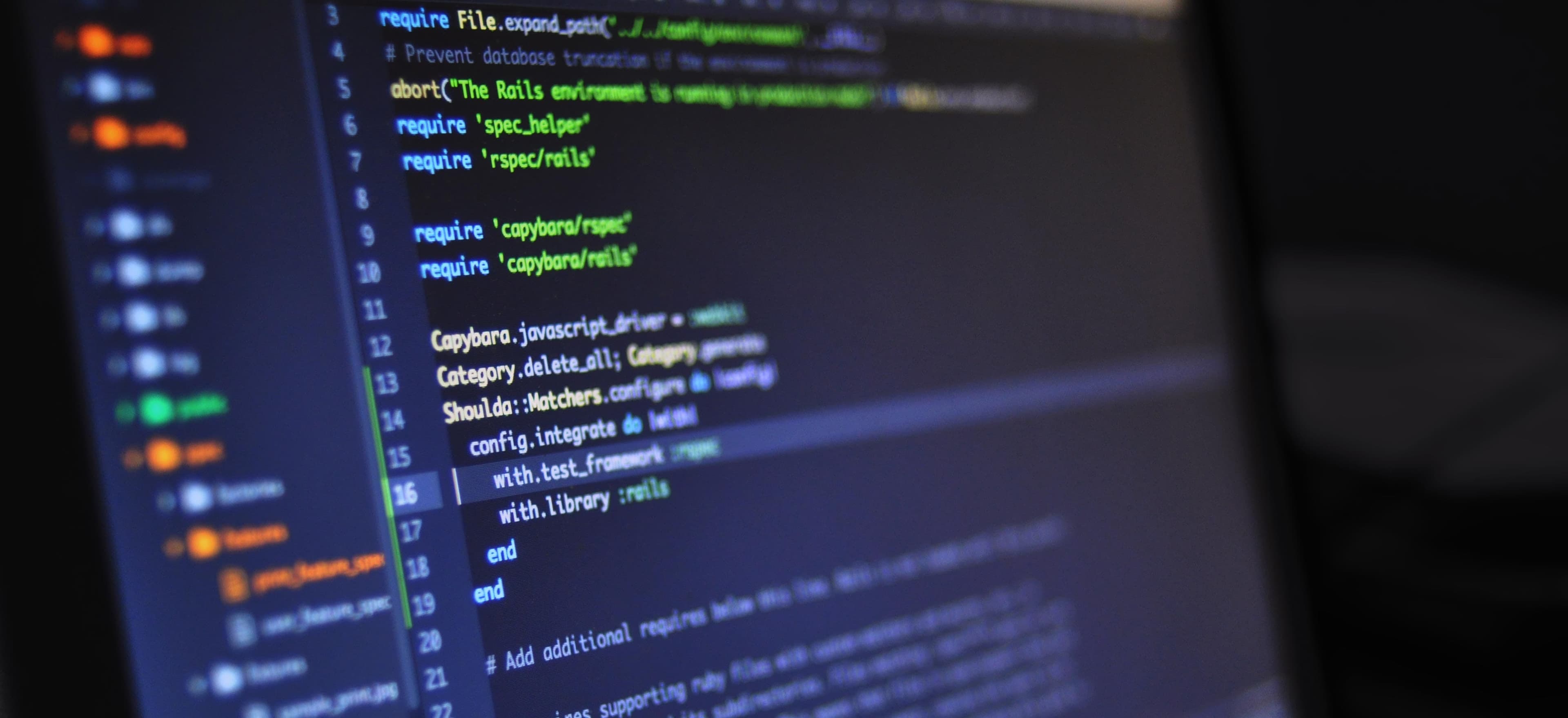
- Published on
Common Spring MVC Errors and How to Fix Them
Spring MVC is a powerful framework for building web applications in Java. However, as developers dive into this powerful toolkit, they often encounter a variety of errors that can hinder development. In this article, we'll explore the most common errors in Spring MVC, their causes, and provide effective solutions to overcome them.
Table of Contents
The Roadmap to Spring MVC
Spring MVC is part of the larger Spring Framework, designed to handle web requests and responses in an efficient manner. It follows the Model-View-Controller design pattern, which separates the application logic from the user interface. From its ability to integrate with other technologies to its extensive configuration options, Spring MVC makes it easier to build robust and scalable web applications.
Despite its strengths, beginners and even seasoned developers may face challenges. Understanding and effectively resolving common errors can significantly streamline your development process.
Common Errors and Solutions
Let’s delve into the most frequent errors developers encounter while working with Spring MVC.
404 Not Found Error
Description:
A "404 Not Found" error occurs when the requested resource is not available on the server. This often happens if the URL is incorrectly mapped or if the resource does not exist.
Cause:
- Incorrect URL.
- Missing controller mappings.
- Application context path issues.
Solution: Begin by checking the URL in your browser. Ensure that it matches the URL patterns defined in your controller. To further troubleshoot, examine your controller code to confirm that you're using the appropriate annotations.
Example:
@Controller
@RequestMapping("/example")
public class ExampleController {
@GetMapping("/page")
public String showPage(Model model) {
model.addAttribute("message", "Hello World");
return "examplePage"; // view name
}
}
Why This Matters:
In this code, if you visit /example/page
, it will work. However, if you visit /example
or /example/pag
, you'll encounter a 404 error. Always verify your URL patterns.
405 Method Not Allowed
Description:
A "405 Method Not Allowed" error indicates that the HTTP method used in the request is not supported for the intended URL.
Cause:
- Incorrect HTTP methods (GET, POST) in request.
- Wrong mapping in controller.
Solution: Ensure that the method you are using corresponds to what's defined in the controller.
Example:
@PostMapping("/submit")
public String submitData(@ModelAttribute("data") DataObject data) {
// Handle the data submission logic
return "successPage";
}
Why This Matters:
If the above method expects a POST request, accessing /submit
via a GET request will lead to a 405 error. Always ensure that your client-side requests align with the specified method types.
Bean Creation Exception
Description:
A "Bean Creation Exception" occurs when the Spring container fails to create a necessary bean.
Cause:
- Misconfigured beans.
- Dependency issues.
Solution: Examine your Spring configuration files or annotations to ensure that all beans are correctly defined and dependencies are satisfied.
Example of configuration:
@Configuration
@ComponentScan(basePackages = "com.example")
public class AppConfig {
@Bean
public ExampleService exampleService() {
return new ExampleServiceImpl();
}
}
Why This Matters:
If ExampleServiceImpl
has dependencies that are not correctly defined, this will result in a creation exception. It is essential to understand the lifecycle of beans for effective management.
NoHandlerFound Exception
Description:
This exception indicates that the Spring MVC framework cannot find any handler methods for the incoming request.
Cause:
- Misconfigured view resolvers.
- Invalid URL mapping.
Solution: Double-check your request mappings and ensure that there's a corresponding method defined in the controller.
Example:
@GetMapping("/dashboard")
public String showDashboard() {
return "dashboardView"; // View must be defined in templates
}
Why This Matters:
If there’s no handler found for your requested URL (e.g., /dashboard
), you will encounter this exception. Review your configuration to ensure mappings are correct.
SessionExpired Exception
Description:
This occurs when a user tries to access a page that requires a session that's no longer valid or has expired.
Cause:
- Session timeout.
- Missing session management.
Solution: Implement session management logic and provide feedback for session expiration.
Example:
@Controller
public class SessionController {
@GetMapping("/profile")
public String showProfile(HttpSession session) {
if (session.getAttribute("user") == null) {
return "redirect:/login"; // Redirect to login if session expired
}
return "profileView";
}
}
Why This Matters:
Having robust session management ensures a better user experience. Redirect users to the login page instead of displaying an error if their session has expired.
Best Practices to Avoid Errors
- Organize Code: Keep your controllers, services, and repositories separated for better maintainability.
- Use Annotations Wisely: Familiarize yourself with Spring MVC annotations like
@Controller
,@RestController
,@GetMapping
, and others. Correct usage can prevent many errors. - Test Regularly: Write unit tests for your controllers and methods to catch errors before deployment using JUnit or Mockito.
- Maintain Proper Logging: Implement logging to help capture issues in production. Frameworks like SLF4J are widely used for logging in Spring applications.
Closing the Chapter
Working with Spring MVC can be incredibly rewarding, but it comes with its set of challenges. Encountering errors is part of every developer's journey, but knowing how to diagnose and fix them can dramatically enhance productivity. By understanding common mistakes and embracing best practices, you can carve out a smoother path in your development with Spring MVC.
For additional context, be sure to check out the Spring MVC documentation for detailed examples and further insights.
With a foundation in effective error management, your Spring MVC applications will be not only functional but also robust and maintainable. Happy coding!
Checkout our other articles