Preventing Deadlocks: Tips for Pessimistic Locking in Java
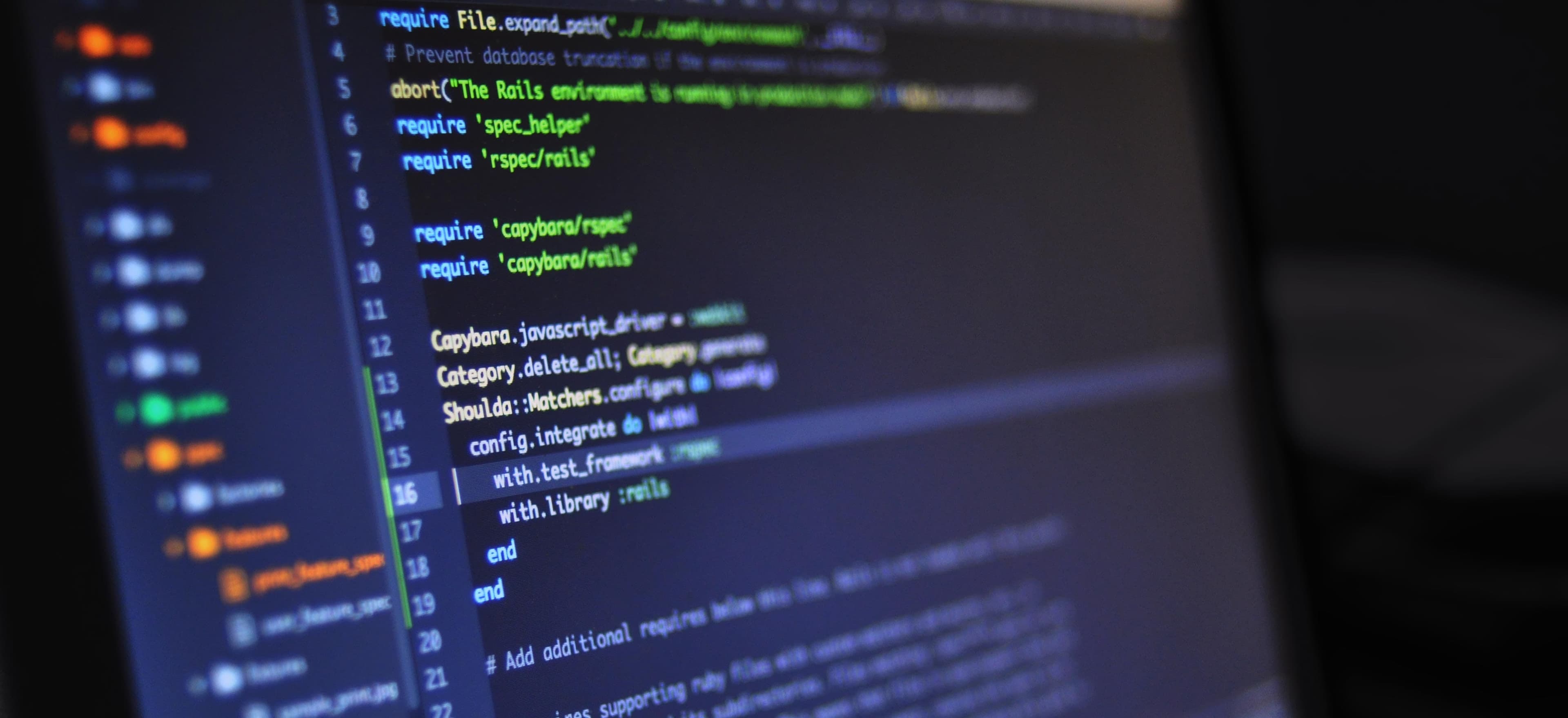
- Published on
Preventing Deadlocks: Tips for Pessimistic Locking in Java
Understanding deadlocks is crucial for any developer working with multithreaded applications in Java. A deadlock occurs when two or more threads are blocked forever, resulting in a halt of application progress. Deadlocks typically happen when two or more threads wait indefinitely for resources held by each other, leading to a system freeze.
In this blog post, we will explore the concept of pessimistic locking in Java and how it can be effectively utilized to prevent deadlocks. We will also delve into best practices while implementing these locking strategies.
Understanding Pessimistic Locking
Pessimistic locking is a concurrency control mechanism that assumes conflicts will occur among threads accessing shared resources. In this approach, a thread locks a resource before using it and holds that lock until its operation is completed.
In contrast, optimistic locking assumes that conflicts are rare and allows threads to proceed without immediate locks, checking for conflicts when completing transactions.
When to Use Pessimistic Locking
Pessimistic locking is an ideal choice when:
- High contention: If multiple threads are likely to request the same resource simultaneously.
- Critical operations: When the accuracy of operations on shared data structures is paramount.
- Risk of inconsistent states: If not using locks may lead to inconsistency due to uncoordinated access.
Key Strategies to Prevent Deadlocks
Here are essential tips on how to prevent deadlocks when using pessimistic locking in Java.
1. Lock Ordering
One of the most effective ways to prevent deadlocks is to establish a global order in which locks must be acquired by threads. By ensuring that all threads acquire locks in the same predefined order, you can minimize the chances of deadlocks.
public class LockOrderingExample {
private final Object lockA = new Object();
private final Object lockB = new Object();
public void thread1() {
synchronized (lockA) {
System.out.println("Thread 1: Holding lock A...");
try { Thread.sleep(100); } catch (InterruptedException e) {}
synchronized (lockB) {
System.out.println("Thread 1: Acquired lock B!");
}
}
}
public void thread2() {
synchronized (lockA) { // Incorrect order -> potential deadlock if thread1 locks lockB first
System.out.println("Thread 2: Holding lock A...");
}
}
}
In the above code, both threads acquire lockA
before lockB
. This ordering leads to the potential of a deadlock if they attempt to lock in any other sequence, illustrating the importance of a consistent locking order.
2. Use Timeout Mechanisms
Another essential strategy is to use timeouts when acquiring locks. This way, if a thread cannot acquire a lock within a specified interval, it can give up and release the locks it holds, reducing the chances of a deadlock.
public void threadWithTimeout() {
boolean gotLockA = false;
boolean gotLockB = false;
try {
gotLockA = lockA.tryLock(100, TimeUnit.MILLISECONDS);
gotLockB = lockB.tryLock(100, TimeUnit.MILLISECONDS);
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Restore the interrupted status
}
try {
if (gotLockA && gotLockB) {
// Perform critical operations
} else {
// Handle failure to acquire locks
}
} finally {
if (gotLockA) lockA.unlock();
if (gotLockB) lockB.unlock();
}
}
In this code snippet, we attempt to acquire lockA
and lockB
with a timeout of 100 milliseconds. If the locks are not acquired in time, the thread can manage the situation gracefully rather than leading to a deadlock.
3. Minimize Lock Scope
Reducing the scope of locks is another effective method. This involves keeping the locked section of your code as small as possible, thus minimizing the time that other threads are waiting for the locks.
public void process() {
synchronized (sharedResource) {
// Perform minimal interactions with shared resource
updateResource();
}
// Other independent operations can occur here without holding the lock
furtherProcess();
}
By narrowing the actions performed within the synchronized block, we decrease the time the lock is held, allowing other threads quick access and reducing blocking time.
4. Lock-free Algorithms
Whenever feasible, consider using lock-free data structures and algorithms. Java provides several concurrent collections such as ConcurrentHashMap
or CopyOnWriteArrayList
, which can help avoid explicit locking.
import java.util.concurrent.ConcurrentHashMap;
public class LockFreeExample {
private ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
public void safeUpdate(String key, Integer value) {
map.merge(key, value, Integer::sum);
// No explicit locking, reducing deadlock risk
}
}
These data structures handle concurrency and avoid deadlocks by managing internal locking mechanisms efficiently. Using them can significantly simplify your code and increase performance.
5. Detecting Deadlocks
Implementing deadlock detection is another approach, although proactive measures are more effective. You could either log the states of threads and resources or leverage tools like Java VisualVM to perform deadlock detection.
6. Review Your Design
Sometimes, the architecture or design of your application can contribute to deadlocks. An essential part of your programming approach should involve reviewing your code structure, dependencies, and thread interactions.
Summary
Preventing deadlocks in Java through pessimistic locking involves a series of strategies and best practices. By implementing lock ordering, using timeouts, minimizing lock scope, considering lock-free algorithms, and monitoring your design, you can significantly mitigate the risk of deadlocks in your applications.
Understanding these strategies not only improves the robustness of your application but also enhances its performance and reliability. For further reading, check out resources like Java Concurrency in Practice and explore the Java documentation on java.util.concurrent.
Now, what strategies will you implement to prevent deadlocks in your next Java project? Happy coding!