Solving NPEs: Understanding Java 14's Enhanced Messages
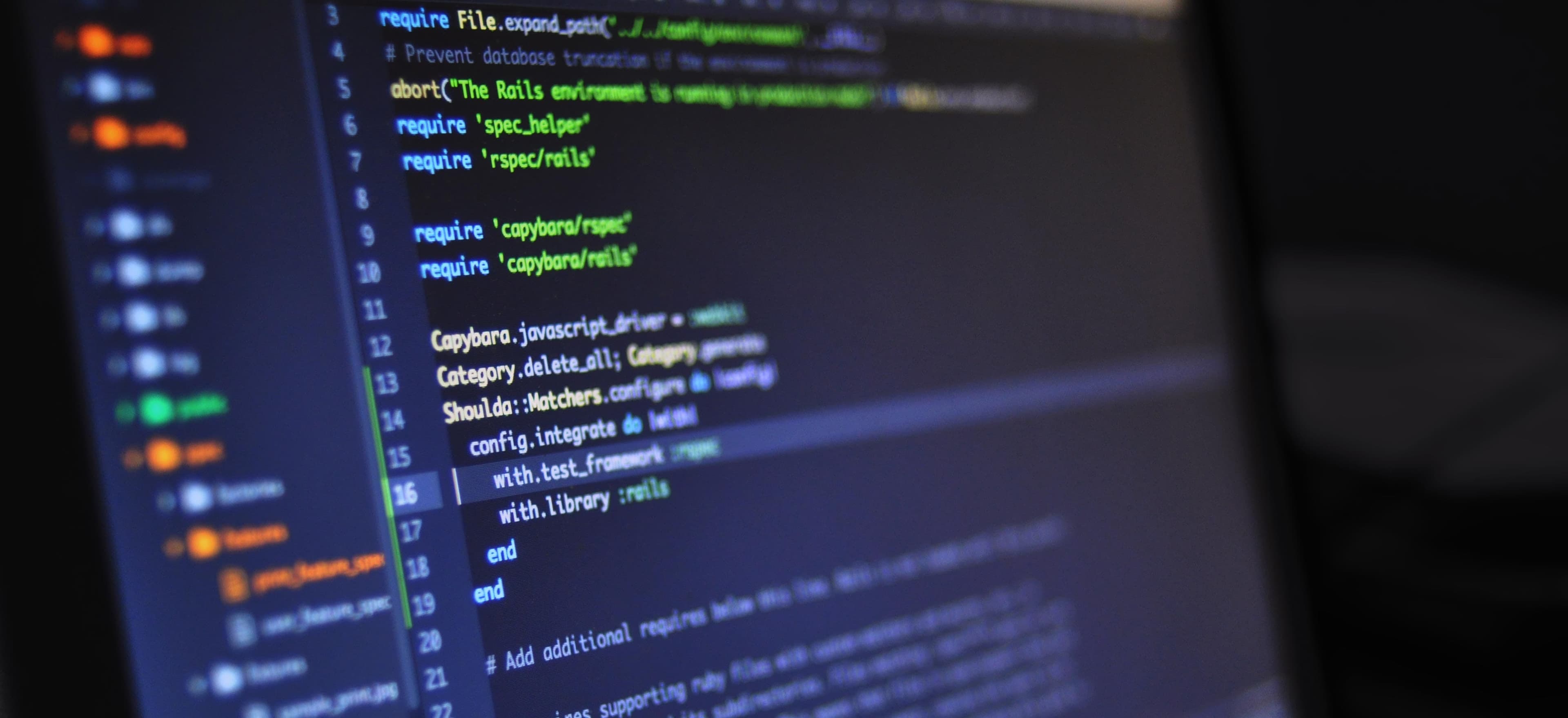
- Published on
Solving NPEs: Understanding Java 14's Enhanced Messages
In the development world, few things are as frustrating as encountering a NullPointerException (NPE). Java developers, whether seasoned or beginners, have met this error and pondered how to troubleshoot it effectively. Thankfully, Java 14 introduced an essential improvement: enhanced messages for NPEs. This feature not only aids in debugging but also promotes cleaner and more readable code.
In this blog post, we will explore what an NPE is, delve into Java 14's enhancements that can help mitigate these errors, and provide some practical code examples. Let’s embark on this journey to understand and resolve NPEs efficiently!
What is a NullPointerException?
A NullPointerException occurs when an application attempts to use null
in a case where an object is required. This might happen in various scenarios: calling a method on a null reference, accessing a field of a null object, or even attempting to calculate the length of an array that is null.
Example of NPE
Consider the following simple example:
public class NPEExample {
public static void main(String[] args) {
String testString = null;
int length = testString.length(); // This line will throw NPE
}
}
In the above code, when length
is computed, it leads to an NPE because testString
is null
.
Enhancements in Java 14
In March 2020, the release of Java 14 brought with it an important enhancement: the ability to provide more informative messages when a NullPointerException occurs. This functionality can significantly reduce the time developers spend debugging these issues.
New Message Format
Now, instead of the generic java.lang.NullPointerException
, you may see messages that specify exactly what was null. The improvement lies in how Java handles a null reference. With the enhanced messages, you can see a stack trace pointing out the variable that was null and the context of the reference.
Key Features
- Helpful Information: Java 14 provides a more detailed output.
- Ease of Debug: Helps locate the root cause efficiently.
- Reduced Development Time: With clear stack traces, fixing bugs becomes quicker.
Example of Enhanced NPE Messaging
Let’s modify the previous code to illustrate how Java 14 improves the output:
public class EnhancedNPEExample {
public static void main(String[] args) {
String testString = null;
printLength(testString); // Enhanced message will be thrown here
}
public static void printLength(String str) {
System.out.println("Length of the string is: " + str.length());
}
}
In the above code, when the printLength
method is called with a null argument, you will likely receive an output similar to this:
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "String.length()" because "str" is null
This message clearly indicates that it's the str
parameter that is holding a null value.
Benefits of Enhanced Messages
Improved Readability
The stack trace is much clearer, helping developers quickly understand which variable is causing the NPE, thereby streamlining the debugging process.
Avoiding Common Pitfalls
NPEs can often be caused by considering returns from APIs or libraries that may return null references. By adopting the enhanced messaging, developers can better analyze their assumptions about what should never be null.
Implementing Defensive Programming
You can further strengthen your code by implementing checks before dereferencing objects.
Example with Defensive Checks
public static void printLength(String str) {
if (str == null) {
throw new IllegalArgumentException("String must not be null");
}
System.out.println("Length of the string is: " + str.length());
}
In this adjusted example, if str
is null
, a clear error message is thrown, proactively catching potential NPEs before they can occur.
Incorporating Java 14
For those working with Java 14 or higher, enabling enhanced messages is straightforward. Ensure you are using the version when running your applications:
$ java --version
Be mindful that enhanced messages will only work on Java 14 or later. If you're using an IDE, ensure it’s configured to use Java 14 or higher.
The Closing Argument
Java 14's enhanced messages for NullPointerExceptions offer developers a useful tool for understanding and troubleshooting errors effectively. This improvement shifts the developer experience from piecemeal inspections to immediate clarity, significantly speeding up the debugging process.
Further Reading
For those interested in deeper dives into understanding NPEs and effective debugging techniques, consider exploring the Oracle Java Tutorials or the Java SE 14 Documentation.
As we wrap up, remember that while enhanced messages are a powerful ally, adopting defensive programming practices can help prevent NPEs from occurring altogether. With awareness and good coding practices, NPEs can become a minor bump in your programming journey rather than a colossal barrier.
With Java constantly evolving, keeping up with its enhancements can significantly ease the development process, guiding you toward robust, maintainable, and efficient code. Happy coding!