Converting API Futures to Reactive Types: A Java Guide
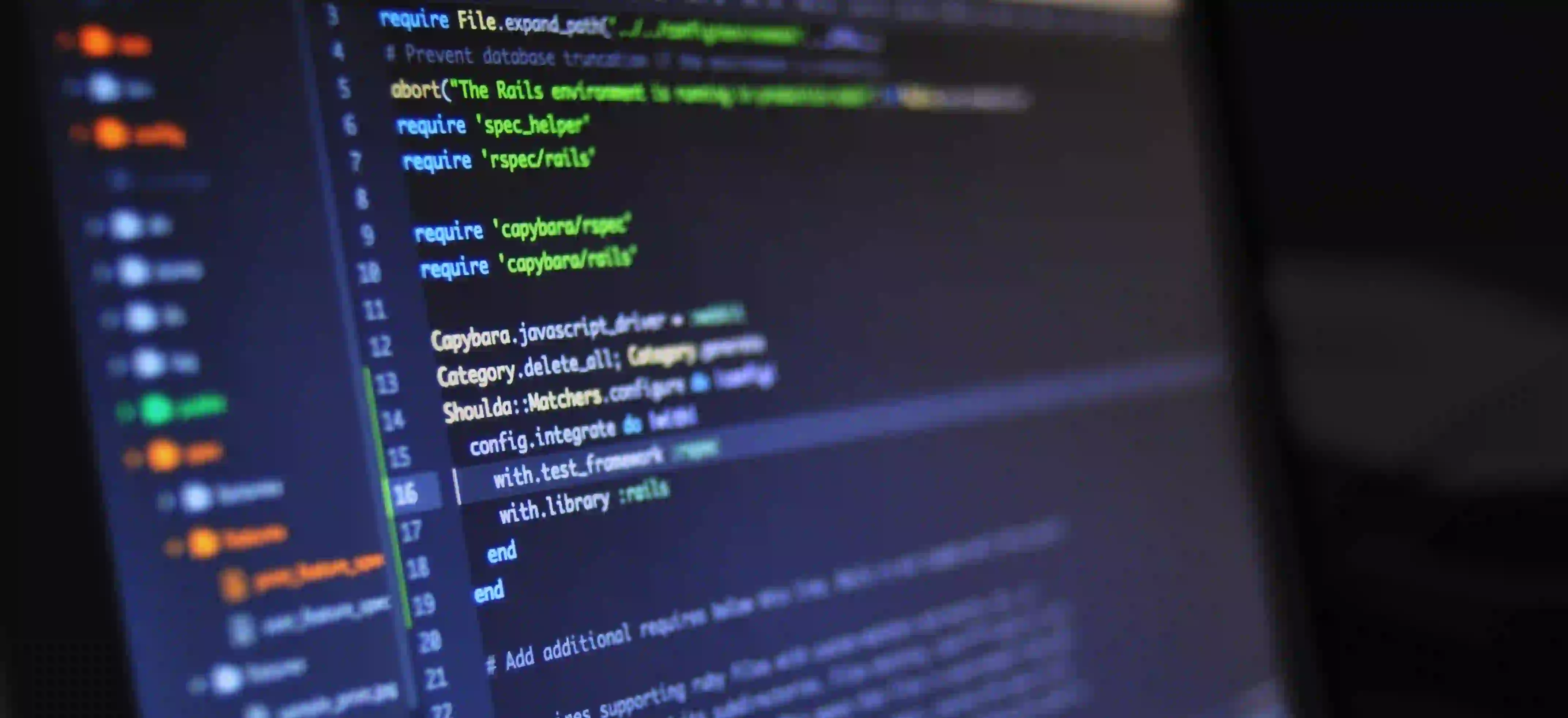
Converting API Futures to Reactive Types: A Java Guide
In the realm of Java development, application programming interfaces (APIs) often return results through Future
objects. While Future
provides a way to perform asynchronous computations, it can sometimes lack the flexibility and richness found in reactive programming. With the rise of reactive frameworks like Project Reactor and RxJava, converting Future
into reactive types can enhance the application's responsiveness and make your code more elegant.
In this blog post, we'll explore how to convert API Future
results into reactive types using Java. We will dive into the differences between Future
and reactive types, followed by practical implementation examples.
Understanding the Basics
What is a Future?
In Java, the Future
interface represents the result of an asynchronous computation. A Future
object allows you to retrieve the result of a computation after it's completed and helps in managing tasks in concurrent programming. It is a part of java.util.concurrent
package, introduced in Java 5.
Here's an example of creating a Future
:
ExecutorService executor = Executors.newFixedThreadPool(2);
Future<Integer> futureResult = executor.submit(() -> {
Thread.sleep(2000);
return 42;
});
The Future
will hold the value 42
, which is returned after a 2-second delay.
What are Reactive Types?
Reactive types, on the other hand, represent asynchronous data streams. These streams allow you to compose complex asynchronous workflows with backpressure support, which can be crucial when dealing with high-volume data processing.
In Java, reactive programming is often accomplished using libraries such as RxJava or Project Reactor. They provide powerful abstractions such as Observable
or Mono
and Flux
for handling streams of data.
Why Convert Future to Reactive Types?
- Asynchronous Flow: Reactive types provide a more declarative style to handle asynchronous flows compared to the imperative style of
Future
. - Error Handling: Reactive programming frameworks come with built-in error-handling strategies.
- Chaining Operations: Reactive types enable you to chain multiple operations more seamlessly.
The Conversion Process
The best way to convert a Future
to a reactive type is by using the .fromFuture()
method in the reactive libraries. Below, we'll provide examples using both RxJava and Project Reactor.
Example: Converting Future to Mono using Project Reactor
First, add the dependency for Project Reactor to your pom.xml
:
<dependency>
<groupId>io.projectreactor</groupId>
<artifactId>reactor-core</artifactId>
<version>3.4.10</version>
</dependency>
Next, let’s convert a Future
into a Mono
:
import reactor.core.publisher.Mono;
import java.util.concurrent.*;
public class FutureToMonoExample {
public static void main(String[] args) throws InterruptedException, ExecutionException {
ExecutorService executorService = Executors.newSingleThreadExecutor();
Future<String> future = executorService.submit(() -> {
Thread.sleep(1000);
return "Hello, Reactive World!";
});
// Convert Future to Mono
Mono<String> monoResult = Mono.fromFuture(future);
// Subscribe and consume the result
monoResult.subscribe(
result -> System.out.println("Result: " + result),
error -> System.err.println("Error: " + error)
);
executorService.shutdown();
}
}
Explanation:
- Future Creation: We create a
Future
that sleeps for 1 second and then returns a greeting. - Mono Conversion: We convert the
Future
to aMono
usingMono.fromFuture()
. - Subscription: By subscribing, we can handle the result and any potential error that occurs during the computation.
Example: Converting Future to Observable using RxJava
For RxJava, include the following dependency in your pom.xml
:
<dependency>
<groupId>io.reactivex.rxjava3</groupId>
<artifactId>rxjava</artifactId>
<version>3.0.12</version>
</dependency>
Now, let’s convert a Future
to an Observable
:
import io.reactivex.rxjava3.core.Observable;
import java.util.concurrent.*;
public class FutureToObservableExample {
public static void main(String[] args) throws InterruptedException, ExecutionException {
ExecutorService executorService = Executors.newSingleThreadExecutor();
Future<String> future = executorService.submit(() -> {
Thread.sleep(1000);
return "Hello, Reactive World!";
});
// Convert Future to Observable
Observable<String> observableResult = Observable.fromFuture(future);
// Subscribe and consume the result
observableResult.subscribe(
result -> System.out.println("Result: " + result),
throwable -> System.err.println("Error: " + throwable)
);
executorService.shutdown();
}
}
Explanation:
- Future Creation: We create a
Future
, similar to the previous example. - Observable Conversion: The conversion is done using
Observable.fromFuture()
. - Subscription: Subscribing allows us to consume the result or handle potential errors asynchronously.
Benefits of Using Reactive Types
- Composability: You can easily chain multiple reactive operations.
- Backpressure Support: Reactive types can manage backpressure, which is critical in high-load situations.
- Thread Management: Reactive libraries help manage threads efficiently under the hood.
When to Use Reactive Programming
While reactive programming can offer numerous benefits, it is essential to recognize scenarios where it is most beneficial:
- Applications requiring high throughput and low latency.
- Systems where the user experience is paramount and responsiveness is critical.
- Applications that need to handle event-driven workflows and streams of data.
Lessons Learned
Converting Future
to reactive types represents a step towards modern Java application development. With the ability to chain operations and better error-handling strategies, embracing reactive programming can lead to more maintainable and performant applications.
Whether you choose Project Reactor or RxJava, leveraging reactive programming will enhance your application’s resilience and responsiveness. We hope this guide has provided you with crucial insights and practical examples to embark on converting your Future
s to reactive types in your Java applications.
Further Reading
- Reactive Programming with Java 8
- Project Reactor Documentation
- RxJava Documentation
By understanding and implementing the concepts provided in this blog, you can significantly improve the structure and performance of your Java applications. Happy coding!