Overcoming Common Pitfalls in Servlet 3.0 Implementation
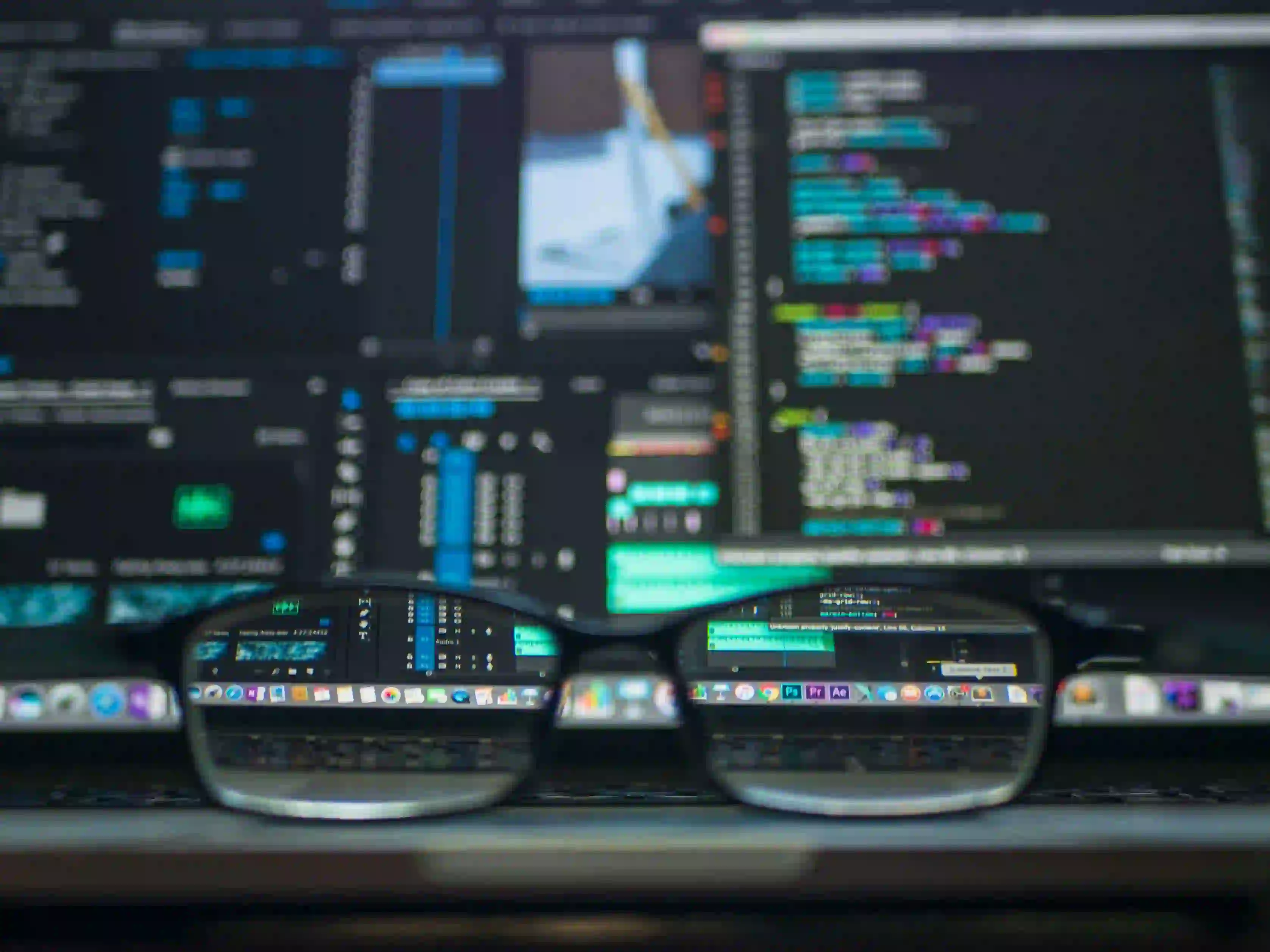
Overcoming Common Pitfalls in Servlet 3.0 Implementation
Servlets have long been a cornerstone of Java web application development. The release of Servlet 3.0 introduced several enhancements, including annotations and improved asynchronous processing. While these features empower developers to create more sophisticated applications, they can also lead to common pitfalls. This blog post will explore prevalent mistakes made during the implementation of Servlet 3.0 and how to avoid them.
Understanding the Servlet Lifecycle
Before diving into the pitfalls, it’s essential to grasp the lifecycle of a servlet. The servlet lifecycle consists of the following stages:
- Loading and Instantiation: The servlet class is loaded and instantiated by the web container.
- Initialization: The
init()
method is called, where you can perform initialization tasks like loading configuration settings. - Request Handling: For each request received, the
service()
method is invoked. This method decides how to respond based on the request type (GET, POST, etc.). - Destruction: Finally, the
destroy()
method is called before the servlet is removed from service.
Grasping this flow is vital for developing efficient and functional servlets.
Common Pitfalls in Servlet 3.0
1. Overutilizing Annotations
With Servlet 3.0, annotations like @WebServlet
and @WebFilter
simplify configuration. However, a common mistake is overusing them, which can lead to confusion and mismanagement.
Solution: Use annotations judiciously. Keep in mind the separation of concerns and maintain clear configuration files when necessary. This approach allows for easier debugging and maintainability.
Here is a simple example of using the @WebServlet
annotation:
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/hello")
public class HelloWorldServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException {
response.setContentType("text/plain");
response.getWriter().write("Hello, World!");
}
}
2. Failing to Implement the destroy()
Method
When a servlet is no longer needed, the destroy()
method is called. Leaving this method unimplemented is a common oversight. Not implementing it can lead to resource leaks, such as open database connections or lingering file handles.
Solution: Always implement the destroy()
method to release resources properly.
@Override
public void destroy() {
// Cleanup logic here
super.destroy(); // Always call the superclass method
}
3. Ignoring Asynchronous Processing
Servlet 3.0 introduced asynchronous processing to improve performance. Many developers fail to implement this feature, missing opportunities for scalability.
Solution: Utilize the AsyncContext
class to perform background tasks efficiently without holding up the request thread. Here’s a simplified example:
@WebServlet(urlPatterns = "/asyncServlet", asyncSupported = true)
public class AsyncServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException {
AsyncContext asyncContext = request.startAsync();
asyncContext.setTimeout(10000); // 10 seconds timeout
// Perform background task
new Thread(() -> {
// Simulate long-running task
try {
Thread.sleep(5000);
response.getWriter().write("Task Complete!");
} catch (InterruptedException | IOException e) {
e.printStackTrace();
} finally {
asyncContext.complete(); // Complete the async processing
}
}).start();
}
}
4. Mismanaging Session Scope
Session management is crucial in web applications. An improper understanding of session scope can lead to issues like session fixation and inefficient session storage.
Solution: Leverage the HttpSession
object wisely. Always validate sessions and avoid storing unnecessary data that could affect performance.
Example of how to retrieve and modify session attributes:
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException {
HttpSession session = request.getSession();
String user = (String) session.getAttribute("user");
if (user == null) {
session.setAttribute("user", "guest");
}
response.getWriter().write("User: " + session.getAttribute("user"));
}
5. Not Handling Exceptions Properly
Another common pitfall is neglecting to handle exceptions in servlets. Uncaught exceptions can lead to application-wide crashes or unintended exposure of sensitive error messages.
Solution: Implement error-handling mechanisms. You can define a custom error page in the web.xml
or use @WebServlet
annotations for handling specific HTTP error codes.
<error-page>
<error-code>404</error-code>
<location>/error404.jsp</location>
</error-page>
6. Ignoring Performance Considerations
Servlets should be efficient. Frequently, developers overlook best practices that affect performance, such as not caching responses or overusing synchronized resources.
Solution: Implement response caching using Cache-Control
headers and optimize data processing logic to reduce the overhead.
Here's how to set cache control in a servlet:
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException {
response.setHeader("Cache-Control", "max-age=3600"); // Cache for 1 hour
response.getWriter().write("Response Caching Implemented!");
}
Closing the Chapter
While Servlet 3.0 introduces exciting features and improvements, developers must be wary of common pitfalls. Understanding the servlet lifecycle, handling resources properly, and leveraging asynchronous processing can significantly enhance web application development.
For a deeper understanding of Java EE development, you can explore Java EE Documentation and Oracle's Servlet Specification.
As with any technology, experience is key. Learn from mistakes and keep improving your servlet skills to create robust Java web applications.