Overcoming Common Pitfalls in Code Migration Projects
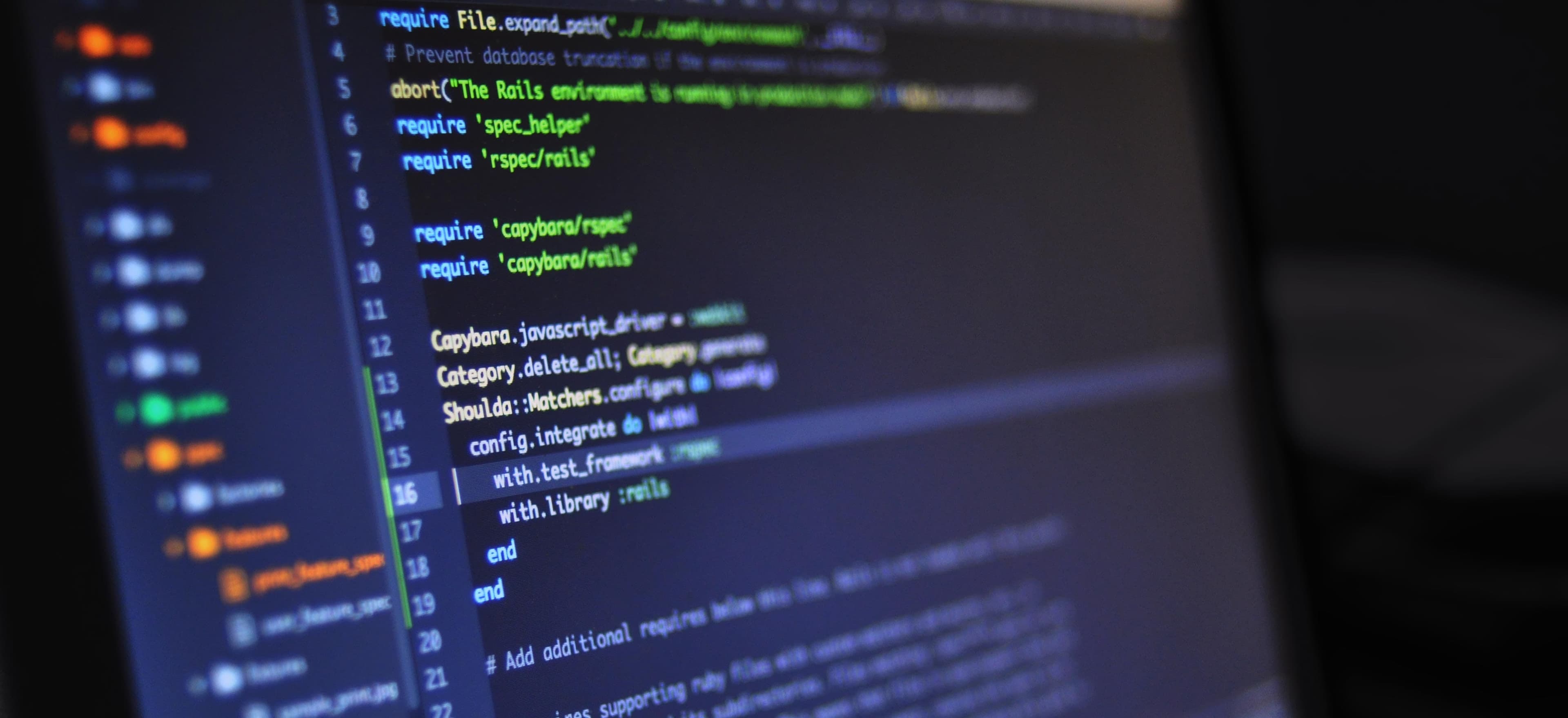
- Published on
Overcoming Common Pitfalls in Code Migration Projects
In the ever-evolving landscape of software development, code migration projects become increasingly necessary. Whether you are moving a monolithic application to microservices or transitioning from one tech stack to another, these projects can be riddled with challenges. In this blog post, we'll explore common pitfalls associated with code migration and how to overcome them effectively.
Understanding Code Migration
Before diving into potential challenges, it is essential to understand what code migration entails. Code migration involves relocating an application from one environment, platform, or technology stack to another. This can include changing programming languages, frameworks, or even databases.
The Importance of Planning
Every successful code migration begins with meticulous planning. The absence of a clear strategy is often the root cause of many migration pitfalls. A well-structured plan outlines the scope of the migration, relevant teams, timelines, and expected outcomes.
Key Components of a Migration Plan:
- Assessment: Evaluate the existing codebase. What has been working, and what needs improvement?
- Compatibility: Determine if the new environment is compatible with existing tools and libraries.
- Documentation: Ensure all existing code is properly documented. This becomes vital during migration to prevent losing critical information.
- Testing Strategy: Devise a robust testing strategy to validate the migration at various stages.
To strengthen your planning phase, you might find resources like Atlassian’s Migration Guide exceptionally useful.
Common Pitfalls in Code Migration
1. Lack of Clear Objectives
A significant pitfall occurs when the migration project lacks defined goals. Without these, teams may find themselves working aimlessly, leading to wasted time and resources.
Solution: Clearly outline the objectives of the migration. Are you aiming for improved performance, scalability, or simply a more modern tech stack? Make sure the entire team is aligned with these objectives.
2. Incomplete Testing
Code migration inherently complicates the testing phase. Often, teams get caught up in the excitement of migrating to a new and trendy setup, neglecting comprehensive testing before launching the new system.
Example Code Snippet for Testing Migration:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MigrationTest {
@Test
public void testAddMethod() {
MyOldClass oldClass = new MyOldClass();
int result = oldClass.add(2, 3);
assertEquals(5, result, "The add method should return the correct sum.");
}
}
In this example, we implement a unit test for a basic addition method. This simple test helps ensure that fundamental functionalities remain intact during migration.
Tip: Extend your testing strategy to include integration and performance tests as well.
3. Underestimating Complexity
Codebases can have hidden complexities that become apparent only during migration. Dependencies, legacy code, and integrations can throw surprises your way.
Solution: Conduct a thorough analysis of dependencies before beginning the migration. Use tools like Apache Maven Dependency Plugin to map out these dependencies and understand their implications on your migration strategy.
4. Insufficient Communication
Migration projects often involve multiple teams - development, QA, operations, and product management. Poor communication can lead to misalignment, ultimately crippling the project.
Solution: Establish regular touchpoints among stakeholders to foster transparency. Utilize collaborative tools like Jira to keep everyone on the same page.
5. Ignoring User Feedback
User feedback can be a gold mine during a migration project. Yet, it is often overlooked. Failing to consider user experience can result in dissatisfaction post-migration.
Solution: Involve end-users during the migration process, allowing them to test the new version early. Consider their feedback to make necessary adjustments before going live.
6. Neglecting Post-Migration Support
Once the migration is complete, the work is not done. Neglecting to provide adequate post-migration support can jeopardize the project's success.
Solution: Prepare a support strategy that includes gathering user feedback, fixing potential bugs, and offering training if necessary. This phase is critical for ensuring that your migration project meets its goals.
Best Practices for Successful Code Migration
1. Establish a Feedback Loop
Implementing a feedback loop throughout the migration helps you identify problems early. Continuous improvement should be the mantra of any code migration project.
2. Prioritize Documentation
Updating documentation during migration is crucial. Ensure that every team member is aware of changes to the system architecture, new processes, and workflows.
3. User Training
Providing adequate training for end-users on the new system is essential for a smooth transition. Schedule training sessions and provide them with user manuals.
4. Leverage Automation Tools
Automation can help streamline many aspects of your migration. Tools like Jenkins for continuous integration and deployment can save time and reduce manual errors.
5. Incremental Migration
Opt for an incremental migration strategy. Instead of attempting to migrate the entire application at once, break it into smaller, manageable components. This makes it easier to isolate issues and reduce risk.
In Conclusion, Here is What Matters
Code migration can be a daunting task, filled with common pitfalls. However, with a structured plan, an understanding of potential challenges, and proactive communication, you can overcome these hurdles.
Successful migration not only enhances the application's performance but also sets your team up for future growth and innovation. Never forget: the effort you put into planning, communication, and post-migration support significantly impacts your long-term success.
By navigating these pitfalls wisely, you’re not just migrating code but paving the way for a future-ready digital solution.
For more insights into software migration and best practices, take a look at Martin Fowler's Blog which frequently covers best practices in software development.
Happy coding!
Checkout our other articles