Common Pitfalls Java Developers Face in Elasticsearch
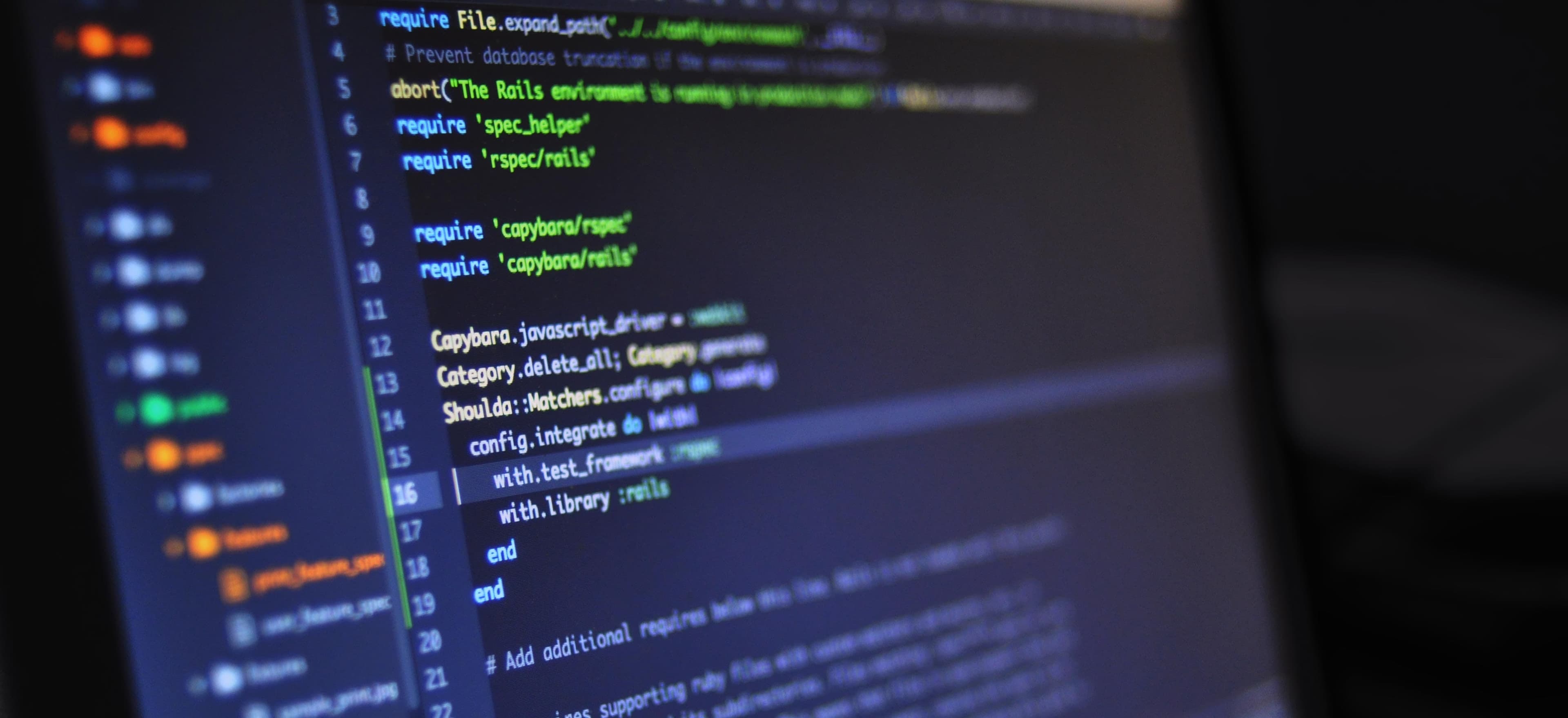
- Published on
Common Pitfalls Java Developers Face in Elasticsearch
Elasticsearch has rapidly become one of the most popular search and analytics engines in the world. Its ability to scale and provide real-time search capabilities makes it a preferred choice for developers. However, Java developers often encounter certain pitfalls when integrating Elasticsearch into their applications. This blog post delves into these common mistakes, providing clarity and solutions to help you navigate these challenges effectively.
Table of Contents
- Understanding Elasticsearch Basics
- Pitfall #1: Ignoring Indexing Strategies
- Pitfall #2: Misunderstanding Query Performance
- Pitfall #3: Improper Exception Handling
- Pitfall #4: Neglecting Data Mapping
- Pitfall #5: Failing to Monitor Elasticsearch Clusters
- Conclusion
Understanding Elasticsearch Basics
Before diving into pitfalls, it's essential to grasp Elasticsearch's foundational aspects. Elasticsearch is a distributed, RESTful search and analytics engine built on top of the Apache Lucene library. With its capability to perform full-text searches and analytics over vast quantities of structured and unstructured data, it is a favored tool in the Java ecosystem.
Elasticsearch works with indexing, querying, and analyzing data. Interfacing with Elasticsearch can be accomplished through its REST API or through various client libraries, including official Elasticsearch Java clients.
Pitfall #1: Ignoring Indexing Strategies
When dealing with data in Elasticsearch, indexing is a crucial step. Developers often make the mistake of ignoring efficient indexing strategies.
Why This Matters
Efficient indexing helps in optimizing search performance and storage. A poor indexing strategy can lead to slow searches and excessive disk usage.
What To Do
-
Batch Indexing: Instead of sending individual documents for indexing, use bulk requests to improve indexing speed.
BulkRequest bulkRequest = new BulkRequest(); for (int i = 0; i < 1000; i++) { IndexRequest indexRequest = new IndexRequest("my_index") .id(String.valueOf(i)) .source(XContentType.JSON, "field", "value" + i); bulkRequest.add(indexRequest); } BulkResponse bulkResponse = client.bulk(bulkRequest, RequestOptions.DEFAULT);
By batching requests, you minimize the overhead associated with network communication, making indexing faster and more efficient.
-
Use Appropriate Refresh Intervals: Understand the implications of refresh rates. If your application doesn't need real-time indexing data, consider increasing the refresh interval to improve performance.
Pitfall #2: Misunderstanding Query Performance
Elasticsearch offers powerful querying tools, but poor query design can lead to performance issues.
Why This Matters
Inefficient queries not only slow down performance but can also strain network and server resources.
What To Do
-
Use Filters Instead of Queries: When appropriate, utilize filters, which are cached and can speed up performance in repeat searches.
{ "query": { "bool": { "filter": [ { "term": { "status": "active" } } ] } } }
-
Profile Your Queries: Use the Profile API to identify bottlenecks in query performance. This will help you adjust accordingly.
Pitfall #3: Improper Exception Handling
Integrating with Elasticsearch may present various types of exceptions. Neglecting proper exception handling can lead to unexpected outcomes.
Why This Matters
Ignoring exceptions can lead to data inconsistency and application crashes.
What To Do
-
Catch Specific Exceptions: Always catch specific Elasticsearch exceptions rather than generic exceptions.
try { IndexResponse response = client.index(indexRequest, RequestOptions.DEFAULT); } catch (ElasticsearchException e) { System.out.println("Elasticsearch Exception: " + e.status()); }
-
Implement Retry Logic: Establish a retry mechanism for transient errors that may occasionally occur due to network issues or unavailability of the Elasticsearch service.
Pitfall #4: Neglecting Data Mapping
Failing to define proper mappings for your data can create a host of issues down the line, including inaccurate searches and wasted storage.
Why This Matters
Mapping determines how documents are stored and indexed. Without it, Elasticsearch may guess types, which can lead to inefficiencies.
What To Do
-
Define Mappings Explicitly: Ensure you outline a mapping that fits your data model. Use
PUT
requests to define these mappings clearly.PUT /my_index { "mappings": { "properties": { "title": { "type": "text" }, "created_at": { "type": "date" }, "price": { "type": "float" } } } }
-
Review and Update Mappings Regularly: As your application evolves, so should your mappings. Regular reviews will ensure relevancy and optimization.
Pitfall #5: Failing to Monitor Elasticsearch Clusters
Monitoring is often an overlooked aspect in the life cycle of managing Elasticsearch.
Why This Matters
Without monitoring, you may face downtimes or performance degradation without any warning.
What To Do
-
Utilize Monitoring Tools: Use the Elastic Stack Monitoring to keep track of cluster health, performance metrics, and resource utilization.
-
Set Up Alerting Systems: Configure alerts for critical metrics like CPU usage, memory usage, and query response times. This can help you stay ahead of potential outages or performance issues.
A Final Look
Navigating Elasticsearch can be challenging, but by being aware of these common pitfalls, Java developers can build more robust and efficient applications.
Always invest time in understanding the architecture, optimize your indexing and querying strategies, handle exceptions properly, ensure proper data mapping, and implement monitoring tools for your Elasticsearch clusters.
For further reading, consider checking out Elastic's Official Documentation to get more detailed information on improving your Elasticsearch integration.
By taking these steps, you will not only improve the performance of your applications but also enhance user experiences through faster and more reliable search capabilities.