Mastering Bug Fixing: The Hidden Cost of Ignoring Issues
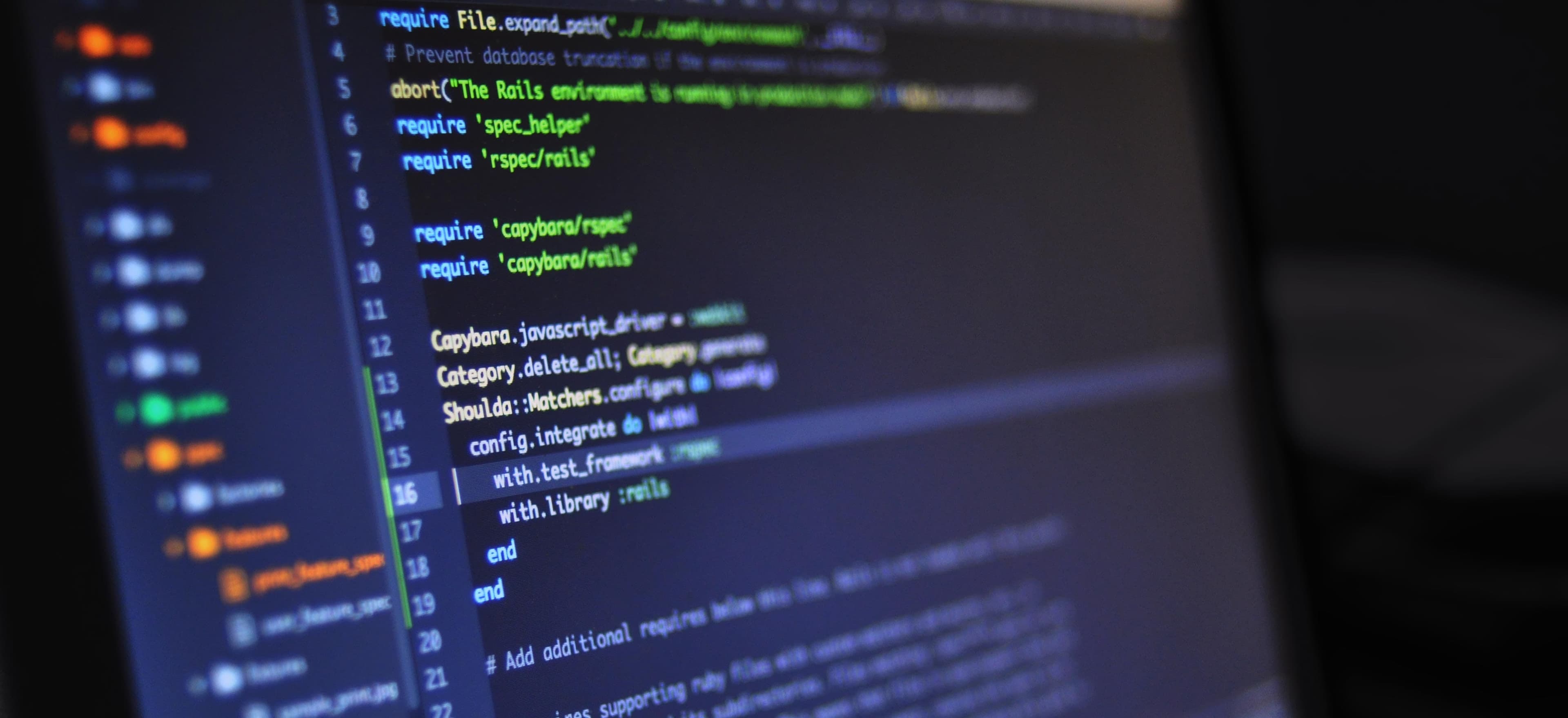
- Published on
Mastering Bug Fixing: The Hidden Cost of Ignoring Issues
In the world of software development, bugs are inevitable. Every developer encounters them, yet not all bugs are treated equally. The true cost of ignoring a bug can extend far beyond the immediate frustration of a faulty code. In this blog post, we will delve into why fixing bugs promptly is crucial, and explore effective strategies for dealing with them in Java development.
The Real Cost of Ignoring Bugs
Ignoring bugs may seem like an appealing option in the short term—after all, there are always new features to develop and deadlines to meet. However, this mentality can lead to astronomical costs down the line. Here are a few implications:
-
Increased Technical Debt: When bugs are ignored, they accumulate. Each bug ignored adds to the technical debt, leading to a codebase that is increasingly harder to maintain and understand.
-
Impact on Performance: Bugs can degrade performance. A missing null check, for instance, can cause a null pointer exception, leading to application crashes.
-
User Dissatisfaction: Users expect a smooth experience. Bugs that lead to crashes or unexpected behavior can erode trust and prevent user adoption.
-
Challenging Future Development: On top of this, unresolved bugs can complicate future development. New features may inherently become dependent on problematic areas of code.
According to Pyramid of Bug Fixing, addressing bugs in the early stages is less costly than fixing them during later stages of development or post-deployment.
Prioritizing Bugs: The Severity Spectrum
Understanding the severity of a bug is crucial to effective management. Bugs can be categorized as:
- Critical: These cause system failures or crashes and need immediate attention.
- Major: These are significant but do not halt operations entirely. They should be addressed soon.
- Minor: These issues cause inconvenience but do not significantly impact functionality. They can be scheduled for later resolution.
By prioritizing bugs efficiently, developers can allocate resources where they are needed most.
Strategies for Effective Bug Fixing in Java
1. Understanding Stack Traces
One of the first steps in bug fixing is understanding the stack trace produced during an exception. Here's a simple code snippet:
public class BuggyCode {
public static void main(String[] args) {
String text = null; // This will create a NullPointerException
System.out.println(text.length());
}
}
Why this matters: The stack trace helps pinpoint where the issue occurred. Before running the code, it’s essential to understand the potential for a null pointer exception in this scenario.
2. Unit Testing
Implementing unit tests can dramatically reduce bug rates. Take the below function as an example:
public int add(int a, int b) {
return a + b; // Simple addition logic
}
A corresponding unit test would look like this:
@Test
public void testAdd() {
assertEquals(5, add(2, 3)); // basic test case
assertEquals(-1, add(-2, 1)); // edge case
}
Why unit tests are important: With a robust suite of unit tests, you catch bugs during development before they reach production.
3. Code Reviews and Pair Programming
Peer reviews and pair programming foster a culture of shared responsibility. You ensure that no bug goes unnoticed by having another set of eyes on the code.
Practical Tip: During code reviews, focus not only on style but also logic and potential pitfalls that could result in bugs.
4. Utilizing Debugging Tools
Java comes with powerful debugging tools integrated in environments like Eclipse and IntelliJ IDEA. Stepping through code can reveal issues that aren’t immediately apparent.
Example:
// Given a method potentially causing issues:
public void processOrder(Order order) {
if (order == null) {
throw new IllegalArgumentException("Order cannot be null");
}
// Process order code here
}
Why debugging is essential: Stepping through your code while it executes can allow you to check the flow and state at crucial points, which is often where bugs lurk.
5. Continuous Integration / Continuous Delivery (CI/CD)
Implementing CI/CD pipelines can automate the testing and deployment process. Tools like Jenkins can help run your suite of tests every time a change is made, ensuring that bugs are caught early.
Further Reading: For a deeper understanding of CI/CD in Java, consider checking out CI/CD Strategies.
Key Takeaways for Bug Fixing
- Don’t ignore bugs: The short-term relief isn’t worth the long-term pain.
- Prioritize effectively: Classify bugs by severity.
- Write tests: It saves time and headaches later on.
- Collaborate: Code reviews and pair programming bring extra insight.
- Leverage tools: Keep your debugging toolkit close and utilize your IDE’s capabilities.
- Automate: Utilize CI/CD pipelines to catch issues as soon as they arise.
To Wrap Things Up
Mastering the art of bug fixing is a crucial skill for any Java developer. By understanding the hidden costs of ignoring bugs, prioritizing effectively, and utilizing practical strategies, you can not only improve the quality of your code but also foster a healthier development process. Remember, every bug has a lesson to teach—don’t let them go unlearned.
By embracing a proactive approach to fixing bugs, you ensure that your project evolves smoothly, user satisfaction remains high, and the cost of development stays manageable. Happy coding!
Checkout our other articles