Decoding NullPointerException Messages in Java
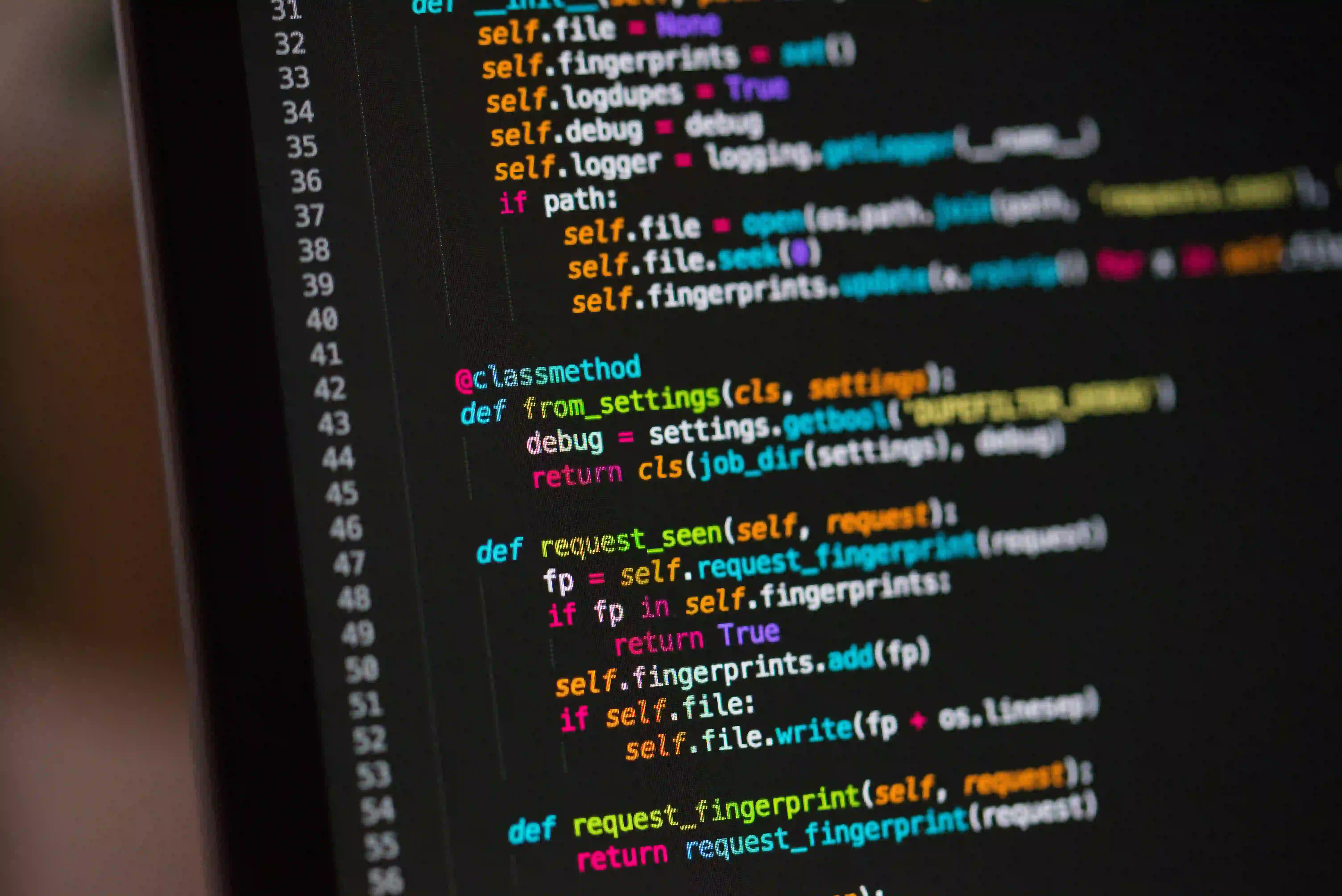
Decoding NullPointerException Messages in Java
The NullPointerException (NPE) is one of the most commonly encountered runtime exceptions in Java programming. For both novice and professional developers, understanding and diagnosing this exception can sometimes feel like navigating a maze. In this blog post, we will unravel NullPointerException messages, explore common causes, provide strategies for prevention, and offer actionable examples.
What is a NullPointerException?
In Java, a NullPointerException occurs when your code attempts to use an object reference that hasn’t been initialized, or, in simpler terms, when the reference points to null
. This can happen in various scenarios, leading to app crashes, unexpected behavior, and frustrating debugging sessions.
Key Aspects of NullPointerException:
- It is an unchecked exception, meaning it does not need to be declared in a method's
throws
clause. - It indicates a bug in your program, as it arises from illegal usage of object references.
Common Causes of NullPointerException
Understanding the causes of NPE can help mitigate their occurrences. Here are some common scenarios where a NullPointerException might arise:
- Calling a method on a null object
- Accessing a field of a null object
- Using null in an array
- Improper dereferencing of an object
Each of these causes can be quite frustrating. Let’s dive into some coding examples to illustrate these points.
Example 1: Calling a Method on a Null Object
public class Main {
public static void main(String[] args) {
String str = null;
// Attempting to call a method on a null reference
System.out.println(str.length());
}
}
Why this Happens: In this example, the variable str
is declared to hold a String but is assigned null
. When we attempt to call str.length()
, Java throws a NullPointerException because it tries to access a method on a non-existent object.
Example 2: Accessing a Field of a Null Object
class Person {
String name;
}
public class Main {
public static void main(String[] args) {
Person person = null;
// Attempting to access a field on a null object
System.out.println(person.name);
}
}
Why this Happens: Here, a Person
object is supposed to hold a name. However, since person
is null
, accessing person.name
results in a NullPointerException. Always ensure that an object is initialized before accessing its fields.
Example 3: Using null in an Array
public class Main {
public static void main(String[] args) {
String[] names = new String[5];
names[0] = "Alice";
// Attempting to access an uninitialized array element
System.out.println(names[1].length());
}
}
Why this Happens: In this scenario, when we create an array of Strings, the elements are initialized to null
. Attempting to access names[1]
(which hasn’t been assigned) and calling .length()
results in a NullPointerException.
Example 4: Improper Dereferencing of an Object
class Employee {
String department;
public Employee(String department) {
this.department = department;
}
}
public class Main {
public static void main(String[] args) {
Employee emp = null;
// Dereferencing a null object
System.out.println(emp.department);
}
}
Why this Happens: Attempting to access emp.department
when emp
is null will cause the program to throw a NullPointerException, showcasing the importance of proper initialization.
Strategies for Preventing NullPointerExceptions
1. Use Java Optional
The Optional
class provides a way to express that a value can be absent. It prevents null
references and encourages developers to check for values.
import java.util.Optional;
public class Main {
public static void main(String[] args) {
Optional<String> optionalName = Optional.ofNullable(getName());
System.out.println(optionalName.orElse("Default Name"));
}
static String getName() {
return null; // or some name logic
}
}
This allows us to avoid potential NPEs by checking if the value is present before use.
2. Leverage Annotations
Annotations such as @NotNull
and @Nullable
can serve as documentation for your code. These annotations help IDEs and static analysis tools catch potential NPEs during development.
public class Main {
public static void main(@NotNull String name) {
// logic
}
}
By using @NotNull
, the code indicates that name
should never be null.
3. Defensive Programming
Always check for null
before using an object reference. This simple habit can save countless hours of debugging.
if (str != null) {
System.out.println(str.length());
} else {
System.out.println("String is null");
}
This defensive code checks if str
is null
before proceeding.
Utilizing assert Statements
In development environments, you can use assert
statements to enforce assumptions within your code. While these are not meant for production use, during development, they serve as useful checks for preventing NPE.
assert str != null : "String cannot be null!";
System.out.println(str.length());
Handling NullPointerException Gracefully
Despite your best efforts, you may still encounter a NullPointerException. You can catch it using a try-catch block to handle it gracefully.
try {
System.out.println(str.length());
} catch (NullPointerException e) {
System.out.println("Caught a NullPointerException!");
}
While this does not solve the root problem, it can prevent your application from crashing.
To Wrap Things Up
Understanding and preventing NullPointerExceptions in Java is vital for maintaining robust code. By familiarizing yourself with common pitfalls and employing strategies such as Optional
, annotations, and defensive programming, you can minimize the occurrence of NPE in your applications.
For further reading and deeper understanding:
- Java's Optional Class
- Effective Java by Joshua Bloch
- Defensive Programming: The Art of Writing Robust Code
Engage actively in your coding practices, and remember: understanding NullPointerException messages is a critical step towards mastering Java!
Happy coding!