Struggling to Fetch Version String in Maven Web Apps?
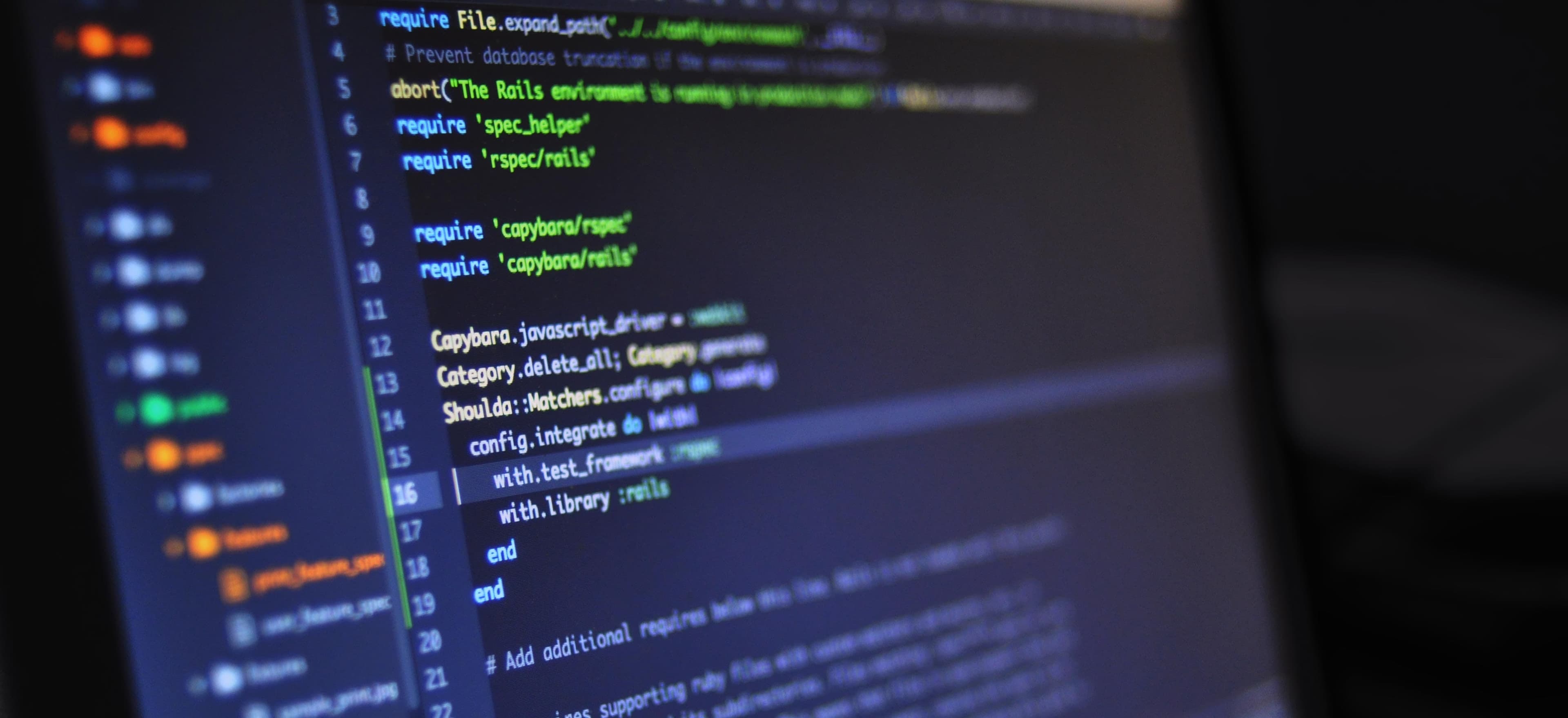
- Published on
Struggling to Fetch Version String in Maven Web Apps?
In the world of Java development, Maven is one of the most widely used tools for managing project dependencies and automating builds. Understanding how to efficiently extract version strings within a Maven web application can save developers time and streamline multiple aspects of the development lifecycle. In this blog post, we will break down how to fetch the version string in your Maven web applications, the common issues you might encounter, and practical solutions to those.
Understanding Maven Basics
Maven is a powerful build automation tool designed primarily for Java projects. It uses an XML file, pom.xml
, to manage project dependencies, build configurations, and plugin integrations. Each Maven project has a defined structure, which makes it easier for developers to navigate and maintain applications.
What is the Version String?
The version string in a Maven project indicates the current version of the project. This is crucial in managing project releases and dependencies. It shows other developers (or consumers of the project) which version they are using.
By default, the version can be found in the header of the pom.xml
file, encapsulated as follows:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-web-app</artifactId>
<version>1.0.0</version> <!-- This is the version string -->
...
</project>
This article will focus on how to dynamically fetch this version string at runtime in your application.
The Problem: Fetching the Version String
When you need to access the version string within your Java code—say for logging or displaying it in the UI—it may not be immediately clear how to retrieve this information from the build process.
You might run into challenges because the pom.xml
file is not directly accessible during runtime. However, there are a few straightforward ways to achieve this.
Solution 1: Accessing the Version Using the Maven Properties Plugin
One common approach is to utilize the Maven Properties Plugin. This plugin allows you to define properties in your pom.xml
that can be accessed at runtime.
Step 1: Add Plugin to pom.xml
To start, include the following snippet in your pom.xml
:
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>properties-maven-plugin</artifactId>
<version>1.0.0</version>
<executions>
<execution>
<goals>
<goal>write-project-properties</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Step 2: Accessing the Version in Java Code
Once this is set up, you can easily access the project version using the @Value
annotation from Spring Framework or directly fetching the properties file generated by the plugin.
To illustrate the latter method, here is how you can read the version string at runtime.
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
public class VersionFetcher {
public String getVersion() {
Properties properties = new Properties();
try (InputStream input = getClass().getClassLoader().getResourceAsStream("project.properties")) {
if (input == null) {
System.out.println("Sorry, unable to find project.properties");
return "Version not found";
}
properties.load(input);
return properties.getProperty("project.version");
} catch (IOException ex) {
ex.printStackTrace();
return "Error occurred while fetching version";
}
}
}
Commentary
In this code snippet:
- We load the
project.properties
file that contains the version. - This prevents the dependency on hardcoding the version in multiple locations.
- If the properties file is not found or fails to load, it handles exceptions gracefully.
Solution 2: Using a Build-Time Resource File Inclusion
Another approach is to leverage the Maven Resources Plugin to copy the version information into a file during the build process.
Step 1: Modify pom.xml
Add the following code to the <build>
section of your pom.xml
:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-resources-plugin</artifactId>
<version>3.1.0</version>
<executions>
<execution>
<id>copy-version-file</id>
<goals>
<goal>copy-resources</goal>
</goals>
<configuration>
<outputDirectory>${project.build.directory}/classes</outputDirectory>
<resources>
<resource>
<directory>src/main/resources</directory>
<includes>
<include>version.txt</include>
</includes>
<filtering>true</filtering>
</resource>
</resources>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
Step 2: Create version.txt
Next, create a file named version.txt
in your src/main/resources
directory with the following content:
Project version: ${project.version}
Step 3: Fetch Version at Runtime
Here’s how to read the version string fetched in version.txt
:
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class VersionFetcher {
public String getVersion() {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(
getClass().getClassLoader().getResourceAsStream("version.txt")))) {
return reader.readLine();
} catch (Exception e) {
e.printStackTrace();
return "Version not found";
}
}
}
Commentary
- The version string is stored in a resource file that is included in the build output, making it easy to fetch at runtime.
- By utilizing Maven for replacing variables at build time, maintenance becomes simpler as you don’t have to worry about keeping it updated manually.
Closing Remarks
Fetching the version string in a Maven web application is crucial for both developer efficiency and application integrity. Whether you opt to use the Maven Properties Plugin or include version information in a resource file, each approach has its merits and can be tailored to better fit your project needs.
Navigating this can be daunting if you're new to Maven, but understanding these methods can streamline your workflow significantly. For further reading on Maven configurations, you can visit the official Maven Documentation.
Now that you're equipped with knowledge on how to manage version strings effectively, you should face fewer struggles in your future Maven endeavors. Happy coding!
Checkout our other articles