Mastering takeWhile and dropWhile: Common Pitfalls in Java Streams
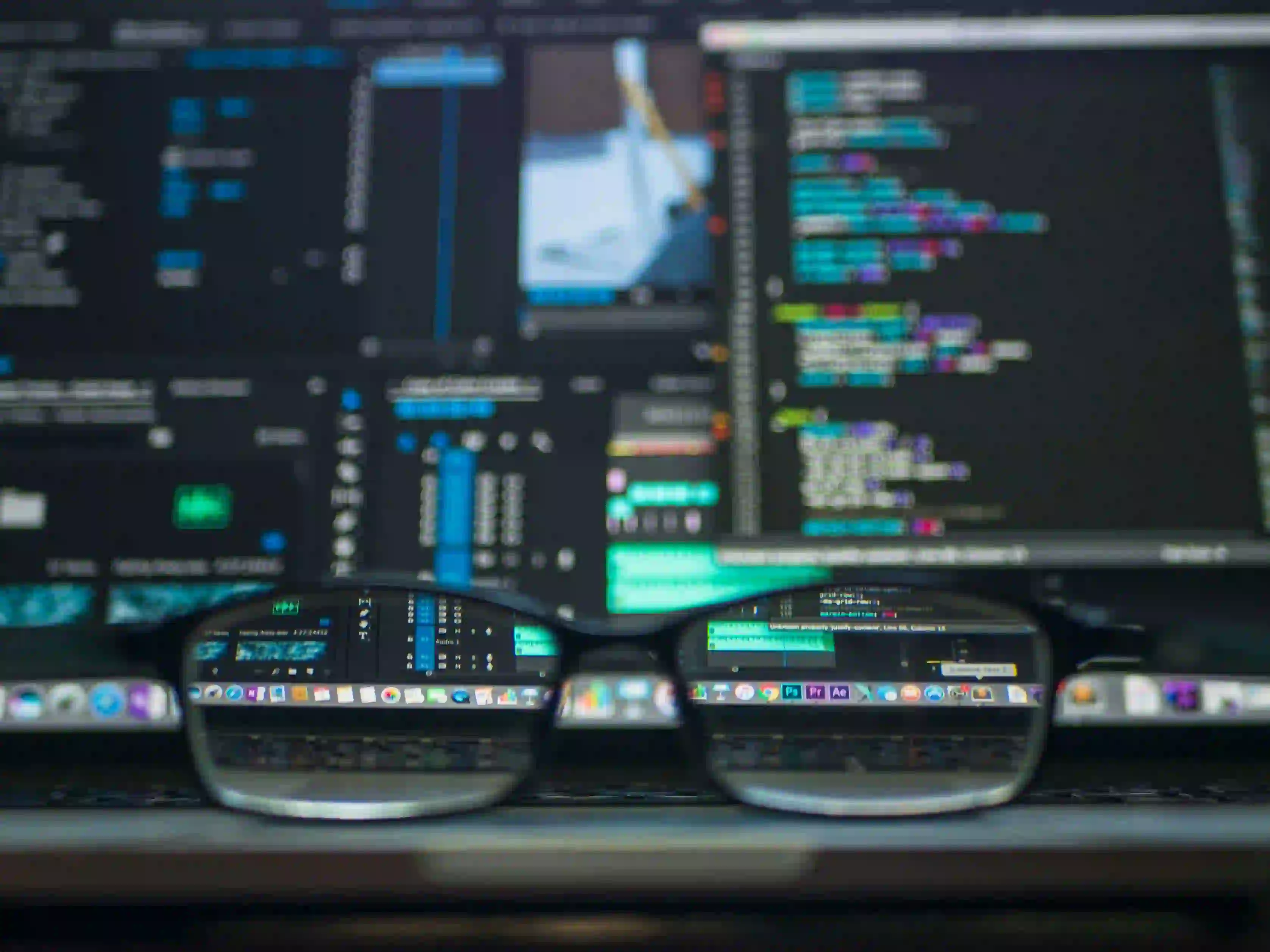
Mastering takeWhile and dropWhile: Common Pitfalls in Java Streams
Java Streams were introduced in Java 8 to facilitate functional-style operations on sequences of elements. Among the many useful methods that Streams offer, takeWhile
and dropWhile
are two powerful tools that can lead to confusion if not understood correctly. This blog post will explore these methods, highlighting their usage, common pitfalls, and best practices.
Understanding takeWhile
and dropWhile
Before diving into pitfalls, let’s establish a clear understanding of what takeWhile
and dropWhile
do.
The takeWhile
Method
The takeWhile
method is used to return a Stream consisting of the longest prefix of elements that match a given predicate. This means it will continue to take elements from the Stream as long as the predicate is true.
Example Usage of takeWhile
import java.util.List;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<Integer> numbers = List.of(3, 5, 8, 10, 12, 15);
List<Integer> result = numbers.stream()
.takeWhile(n -> n < 10) // Takes elements while they are less than 10
.collect(Collectors.toList());
System.out.println(result); // Output: [3, 5, 8]
}
}
The dropWhile
Method
Conversely, dropWhile
skips elements from the Stream as long as the given predicate holds true, returning the rest of the elements once the predicate returns false.
Example Usage of dropWhile
import java.util.List;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<Integer> numbers = List.of(3, 5, 8, 10, 12, 15);
List<Integer> result = numbers.stream()
.dropWhile(n -> n < 10) // Drops elements while they are less than 10
.collect(Collectors.toList());
System.out.println(result); // Output: [10, 12, 15]
}
}
Common Pitfalls
Now that we've covered the basics, let’s discuss the most common pitfalls developers encounter when using takeWhile
and dropWhile
.
1. Not Understanding Short-Circuiting Behavior
One major pitfall is misunderstanding how short-circuiting works in takeWhile
and dropWhile
. Both methods stop processing elements as soon as the condition fails (for takeWhile
) or succeeds (for dropWhile
).
Example of Short-Circuiting
import java.util.List;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<Integer> numbers = List.of(5, 3, 8, 10, 7);
List<Integer> result = numbers.stream()
.dropWhile(n -> n < 6) // Will drop 5 and 3
.collect(Collectors.toList());
System.out.println(result); // Output: [8, 10, 7]
}
}
In this example, the Stream ceases any further checks once it encounters the first element that does not match the drop condition (in this case, the number 5).
2. Applying Unsuitable Conditions
Another common mistake is using conditions that do not apply to all elements of the Stream or failing to detect when the predicate never holds. This results in a Stream that unexpectedly returns an empty result.
Example of Unsuitable Conditions
import java.util.List;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<Integer> numbers = List.of(15, 20, 25);
List<Integer> result = numbers.stream()
.takeWhile(n -> n < 10) // No elements satisfy this condition
.collect(Collectors.toList());
System.out.println(result); // Output: []
}
}
3. Using Before Java 9
takeWhile
and dropWhile
were introduced in Java 9, so a common pitfall for developers is attempting to use these methods in earlier versions of Java. Always ensure your environment is updated to utilize these functionalities.
4. Being Unaware of State Side Effects
When using takeWhile
and dropWhile
, be cautious if you are depending on external mutable states. Non-deterministic behavior or concurrent modifications can lead to unpredictable results.
import java.util.ArrayList;
import java.util.List;
public class Example {
public static void main(String[] args) {
List<Integer> numbers = List.of(3, 5, 8, 10);
List<Integer> sideEffects = new ArrayList<>();
List<Integer> result = numbers.stream()
.takeWhile(n -> {
sideEffects.add(n); // Modifying an external collection
return n < 10;
})
.collect(Collectors.toList());
System.out.println(sideEffects); // Might be different than expected
System.out.println(result); // Output: [3, 5, 8]
}
}
5. Misusing with Infinite Streams
Workflow configurations involving infinite streams can yield confusing results, especially when combined with takeWhile
and dropWhile
. Be aware that the exit condition must ultimately be achievable.
Example of Infinite Stream Misuse
import java.util.stream.Stream;
public class Example {
public static void main(String[] args) {
Stream<Integer> infiniteNumbers = Stream.iterate(1, n -> n + 1); // Infinite stream
List<Integer> result = infiniteNumbers
.takeWhile(n -> n < 10) // This works, but...
.limit(5) // Use limit to prevent overly large collections
.collect(Collectors.toList());
System.out.println(result); // Output: [1, 2, 3, 4, 5] but can be resourced-intensive
}
}
Best Practices
To avoid pitfalls, follow these best practices:
-
Test Conditions Rigorously: Always validate that your predicate will behave as expected across the entire flow of your data.
-
Use Version Suitable Tools: Make sure you are running a Java version that supports these methods.
-
Limit Stream Size: When dealing with infinite streams, always define a limit or an end condition to prevent excessive resource usage.
-
Understand Short-Circuiting: Comprehend how
takeWhile
anddropWhile
handle termination to avoid misplaced expectations. -
Isolate Side Effects: Try to keep your predicates side-effect-free. It increases predictability and clarity in your Streams.
Final Thoughts
Understanding and mastering takeWhile
and dropWhile
is essential for any Java developer aiming to utilize the full power of Java Streams. While they offer elegant solutions to processing sequences, misapplication can lead to unexpected behaviors. By being aware of common pitfalls and following best practices, you can leverage these methods effectively in your applications.
For further reading, check out the official Java Documentation on Streams and explore more advanced concepts within the Java Streams API.
Happy coding!